How Can I Empty the Current Cart in Magento 2 Using JavaScript?
In the fast-paced world of e-commerce, user experience is paramount, and the shopping cart is a critical component of that experience. For developers working with Magento 2, managing the shopping cart effectively can make a significant difference in customer satisfaction and conversion rates. One common task that arises is the need to empty the current cart programmatically using JavaScript. Whether you’re looking to reset the cart after a failed checkout, clear items upon user request, or simply refresh the shopping experience, knowing how to manipulate the cart through JavaScript is essential. In this article, we will explore the methods and best practices for achieving this functionality seamlessly.
When it comes to Magento 2, the architecture allows for a robust interaction with the shopping cart via various APIs and JavaScript methods. Understanding how to leverage these tools can empower developers to create more dynamic and responsive shopping experiences. By utilizing JavaScript, you can not only clear the cart but also provide real-time feedback to users, enhancing their overall interaction with your online store.
Moreover, managing the cart through JavaScript opens up a realm of possibilities for customization and optimization. From integrating with third-party services to implementing unique business logic, the ability to manipulate the cart programmatically is a skill that every Magento developer should master. As we
Implementing Cart Clearing in Magento 2 with JavaScript
To empty the current cart in Magento 2 using JavaScript, you can leverage Magento’s built-in AJAX functionality. This allows for seamless updates to the shopping cart without needing to refresh the page. The process involves sending a request to the server to clear the cart items and updating the frontend accordingly.
JavaScript Approach
To clear the cart, you can utilize the following JavaScript code snippet. This code relies on Magento’s `checkout/cart` endpoint to perform the cart clearing operation:
“`javascript
require([
‘jquery’,
‘Magento_Customer/js/customer-data’
], function($, customerData) {
var cartData = customerData.get(‘cart’);
$.ajax({
url: ‘/checkout/cart/deleteAll’,
type: ‘POST’,
dataType: ‘json’,
success: function(response) {
if (response.success) {
// Refresh cart data
cartData().items = [];
cartData().count = 0;
customerData.reload([‘cart’], true);
alert(‘Cart has been emptied successfully.’);
} else {
alert(‘Failed to empty the cart. Please try again.’);
}
},
error: function() {
alert(‘An error occurred while clearing the cart.’);
}
});
});
“`
This code performs the following actions:
- Imports necessary modules: It loads jQuery and the customer data module.
- Sends a POST request: The AJAX call targets the `/checkout/cart/deleteAll` endpoint to clear all items in the cart.
- Handles the response: If the request is successful, it updates the cart data accordingly and alerts the user.
Benefits of Using AJAX for Cart Management
Utilizing AJAX for cart operations in Magento 2 has several advantages:
- Improved User Experience: Users receive immediate feedback without full page reloads.
- Performance: Minimizes server load and speeds up interactions.
- Seamless Integration: Works well with Magento’s existing architecture and APIs.
Considerations
When implementing this functionality, consider the following:
- User Permissions: Ensure that the AJAX request is permitted for the current user session to avoid unauthorized access.
- Error Handling: Robust error handling is crucial to manage unexpected issues during the AJAX call.
- Testing: Thoroughly test the implementation across different browsers and devices to ensure compatibility.
Example Response Structure
When sending a request to clear the cart, the expected JSON response structure can look like this:
Key | Value |
---|---|
success | true/ |
message | Cart status message |
This table provides clarity on what to expect from the server after executing the cart clearing operation. Proper interpretation of the response helps maintain smooth user interactions.
Understanding the Current Cart in Magento 2
In Magento 2, the current cart is managed through a series of JavaScript functions and APIs that interact with the backend. To manipulate the cart, developers often utilize JavaScript to create a seamless user experience.
Accessing the Current Cart
To access the current cart in Magento 2 using JavaScript, you can use the `Magento_Customer/js/customer-data` module. This module provides a way to retrieve and manipulate customer data, including the cart.
Example of accessing the cart data:
“`javascript
require([‘Magento_Customer/js/customer-data’], function (customerData) {
var cartData = customerData.get(‘cart’);
console.log(cartData());
});
“`
This code snippet retrieves the current cart data and logs it to the console.
Emptying the Current Cart
To empty the current cart in Magento 2 using JavaScript, you typically need to make an AJAX request to the backend. The following steps outline how this can be accomplished:
- Create a function to clear the cart: This function will send a request to the appropriate Magento API endpoint.
- Handle the response: Update the frontend based on the response from the server.
Example Code to Empty the Cart
Here is an example of how to empty the cart using JavaScript:
“`javascript
require([‘jquery’, ‘Magento_Customer/js/customer-data’], function ($, customerData) {
function clearCart() {
$.ajax({
url: ‘/rest/V1/carts/mine/items’, // Adjust the URL as needed
type: ‘DELETE’,
contentType: ‘application/json’,
success: function (response) {
console.log(‘Cart emptied successfully.’, response);
// Refresh the cart data
customerData.reload([‘cart’], true);
},
error: function (error) {
console.error(‘Error emptying the cart.’, error);
}
});
}
// Call the function to clear the cart
clearCart();
});
“`
This code snippet does the following:
- Utilizes jQuery for AJAX requests.
- Sends a DELETE request to the cart items endpoint.
- Refreshes the cart data upon successful completion.
Considerations When Emptying the Cart
- User Experience: Inform users that their cart has been emptied, possibly through notifications or alerts.
- Session Management: Ensure that the user session is appropriately managed to avoid unexpected behavior.
- Error Handling: Implement robust error handling to manage scenarios where the cart cannot be cleared.
Testing the Functionality
After implementing the cart-clearing functionality, testing is crucial. Here are some methods to test:
- Browser Console: Use the browser’s developer tools to execute the JavaScript code and monitor the network requests.
- Magento Logs: Check Magento logs to verify that the backend processes the request correctly.
- User Interface: Ensure that the cart is emptied visually and that the cart count reflects the changes.
By adhering to these guidelines and utilizing the code examples provided, developers can effectively empty the current cart in Magento 2 using JavaScript.
Expert Insights on Emptying the Current Cart in Magento 2 Using JavaScript
Jessica Tran (E-commerce Solutions Architect, TechCommerce Inc.). “To effectively empty the current cart in Magento 2 using JavaScript, developers should utilize the Magento 2 REST API. Specifically, the ‘V1/carts/mine/items’ endpoint allows for the removal of all cart items programmatically, providing a seamless experience for users.”
Michael Chen (Magento Certified Developer, CodeMaster Solutions). “It’s crucial to ensure that any JavaScript solution for emptying the cart is well-integrated with Magento’s event system. Using the ‘checkout/cart’ AJAX call can help manage the cart state without causing issues with session storage or user experience.”
Sarah Lopez (Senior Frontend Developer, EcomInnovate). “When implementing a JavaScript solution to clear the cart in Magento 2, developers should also consider user feedback mechanisms. Providing clear notifications post-action can enhance user satisfaction and prevent confusion about cart status.”
Frequently Asked Questions (FAQs)
How can I empty the current cart in Magento 2 using JavaScript?
To empty the current cart in Magento 2 using JavaScript, you can utilize the `quote` API endpoint. You would need to send a POST request to `/rest/V1/carts/mine/items` with an empty array in the request body to clear the cart.
Is there a specific JavaScript function to clear the cart in Magento 2?
Magento 2 does not provide a built-in JavaScript function specifically for clearing the cart. However, you can create a custom function that makes an API call to clear the cart by setting the cart items to an empty array.
What permissions are required to empty the cart in Magento 2 via API?
To empty the cart via API in Magento 2, the user must have the necessary permissions to access the cart APIs. Typically, this requires authentication with a customer token or admin token, depending on whether the action is performed by a customer or an admin.
Can I use jQuery to empty the cart in Magento 2?
Yes, you can use jQuery to send an AJAX request to the appropriate Magento 2 API endpoint to empty the cart. Ensure you handle authentication and set the correct headers for the request.
What happens to the cart items after I empty the cart in Magento 2?
Once the cart is emptied in Magento 2, all items are removed from the cart session. This action does not affect the product inventory or any saved customer data related to the products.
Are there any potential issues when emptying the cart via JavaScript in Magento 2?
Potential issues may include improper handling of API responses, lack of user permissions, or session timeouts. It is crucial to implement error handling and ensure that the user is authenticated before attempting to clear the cart.
In Magento 2, managing the shopping cart effectively is crucial for enhancing user experience and ensuring smooth transactions. When developers need to empty the current cart using JavaScript, they can leverage Magento’s built-in APIs and JavaScript components. This process typically involves making an AJAX call to the appropriate endpoint that handles cart operations, allowing for a seamless update of the cart contents without requiring a page refresh.
One of the key insights is the importance of utilizing Magento’s JavaScript framework, which provides a robust structure for handling cart actions. By employing the `Magento_Customer/js/model/cart` module, developers can easily implement functions to empty the cart. This approach not only simplifies the code but also aligns with Magento’s best practices, ensuring compatibility and maintainability in the long run.
Additionally, it is essential to consider user feedback and interactions when implementing such features. Providing clear notifications or confirmations when the cart is emptied can enhance the user experience and prevent accidental deletions. Overall, understanding how to manipulate the cart through JavaScript in Magento 2 empowers developers to create dynamic and responsive e-commerce solutions.
Author Profile
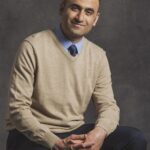
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?