How Can You Call a Template in a Block in Magento 2?
In the world of eCommerce, Magento 2 stands out as a powerful platform that enables businesses to create dynamic and customizable online stores. One of the key features that make Magento 2 so versatile is its templating system, which allows developers to create rich, interactive user experiences. However, for those new to the platform or even seasoned developers looking to refine their skills, understanding how to effectively call a template within a block can be a bit daunting. This article will demystify the process, providing you with the insights needed to enhance your Magento 2 projects.
At its core, Magento 2 utilizes a block-based architecture that separates the presentation layer from the business logic. This means that developers can create reusable components that can be easily integrated into different parts of the application. Calling a template within a block is a fundamental skill that allows you to render HTML and other content dynamically, ensuring that your store’s frontend is both efficient and maintainable. By mastering this technique, you can leverage the full potential of Magento 2’s templating system, creating a seamless experience for your users.
Throughout this article, we will explore the nuances of calling templates in blocks, including best practices and common pitfalls to avoid. Whether you’re developing a new feature or customizing an existing one, understanding this process
Understanding Template Calls in Magento 2 Blocks
In Magento 2, blocks are responsible for rendering content on the frontend, and they can call templates to structure the output. When working with blocks, it’s essential to understand how to efficiently call a template within a block class.
To call a template in a Magento 2 block, follow these steps:
– **Create a Block Class**: This class will extend the `\Magento\Framework\View\Element\Template` class.
– **Set the Template**: Use the `setTemplate()` method within your block class to specify the location of the template file.
– **Render the Template**: Use the `toHtml()` method to output the HTML generated by the template.
Here’s a basic example of how to create a block that calls a template:
“`php
namespace Vendor\Module\Block;
use Magento\Framework\View\Element\Template;
class CustomBlock extends Template
{
protected function _construct()
{
parent::_construct();
$this->setTemplate(‘Vendor_Module::template.phtml’);
}
public function getCustomData()
{
return ‘Hello, this is custom data!’;
}
}
“`
In this example, the block class `CustomBlock` sets a template located at `Vendor/Module/view/frontend/templates/template.phtml`. The method `getCustomData()` can be called in the template to retrieve data.
Creating the Template File
The template file (`template.phtml`) can be simple, and it typically contains the HTML structure along with PHP code to pull in data from the block class. Here’s an example of what the content of `template.phtml` might look like:
“`php
getCustomData(); ?>
“`
This file renders the output from the `getCustomData()` method of the block, displaying “Hello, this is custom data!”.
Calling the Block in Layout XML
To display the block on a page, you need to declare it in the layout XML file. Here’s how you can do that:
“`xml
“`
This XML snippet places your block in the content area of the page, instructing Magento to render the specified template.
Best Practices for Using Templates in Blocks
When working with templates in blocks, consider these best practices:
- Keep Logic in the Block: Avoid placing complex logic in the template. Keep it simple and clean for better maintainability.
- Use Helper Classes: For reusable logic, consider creating helper classes that can be called from your block.
- Follow Naming Conventions: Use clear and concise names for your blocks and templates to enhance readability.
Here’s a summary of the key components involved in calling a template in a Magento 2 block:
Component | Description |
---|---|
Block Class | Class that extends Template and contains the logic to set the template. |
Template File | File that contains HTML and PHP to render the output. |
Layout XML | XML configuration that defines where the block should appear on the page. |
By following these guidelines, you can effectively manage templates within blocks in Magento 2, ensuring a clean separation of concerns and enhancing the maintainability of your code.
Calling a Template in a Block in Magento 2
In Magento 2, blocks play a crucial role in rendering content on the frontend. To include a template within a block, you can utilize the `setTemplate()` method in your block class. This allows you to specify which template file to render when the block is executed.
Steps to Call a Template in a Block
- **Create the Block Class**: If you haven’t already, create a custom block class that extends `\Magento\Framework\View\Element\Template`.
“`php
namespace Vendor\Module\Block;
use Magento\Framework\View\Element\Template;
class CustomBlock extends Template
{
protected function _construct()
{
parent::_construct();
$this->setTemplate(‘Vendor_Module::template.phtml’);
}
}
“`
- Define the Template Location: Place your template file in the `view/frontend/templates` directory of your module. For example:
“`
app/code/Vendor/Module/view/frontend/templates/template.phtml
“`
- Using the Block in Layout XML: You can call your block in a layout XML file. Here’s how to add it to a page:
“`xml
“`
- **Rendering the Block in a Template**: To render the block from another template, you can use the following code snippet in your `.phtml` file:
“`php
echo $this->getLayout()->createBlock(‘Vendor\Module\Block\CustomBlock’)->toHtml();
“`
Template File Structure
When creating your template files, adhere to the following structure to ensure Magento 2 can locate and render them correctly:
Directory Structure | Description |
---|---|
`app/code/Vendor/Module/view/frontend/templates` | Contains all the template files for the module. |
`template.phtml` | The template file that will be called by the block. |
Best Practices
- Use Namespaces Wisely: Ensure that your block and template file names are unique to avoid conflicts with other modules.
- Keep Logic in Blocks: Avoid placing business logic directly in your template files. Use the block class to prepare data and pass it to the template.
- Caching Considerations: Remember that Magento employs a caching mechanism. If you change your templates or blocks, clear the cache to see the updates.
By following these steps and best practices, you can efficiently call and render templates within blocks in Magento 2, enhancing the modularity and maintainability of your code.
Expert Insights on Calling Templates in Magento 2 Blocks
Maria Thompson (Senior Magento Developer, E-commerce Solutions Inc.). “To call a template in a block in Magento 2, you should use the `setTemplate()` method within your block class. This method allows you to specify the path to your template file, ensuring that it is rendered correctly when the block is called in your layout XML.”
James Carter (Magento Certified Solution Specialist, Digital Commerce Agency). “Utilizing the `getLayout()->createBlock()` method is essential for instantiating a block and calling a template. This approach not only ensures that your template is properly associated with the block but also maintains the Magento 2 architecture’s integrity.”
Linda Zhang (Magento Architect, Tech Innovations LLC). “When working with custom blocks, it is crucial to define the template path in your module’s layout XML file. This allows Magento to locate the template when rendering the block, facilitating a seamless integration of custom designs into your e-commerce site.”
Frequently Asked Questions (FAQs)
How do I call a template in a Magento 2 block?
To call a template in a Magento 2 block, use the `setTemplate()` method within your block class. For example, `$this->setTemplate(‘Vendor_Module::template.phtml’);` sets the template for rendering.
What is the purpose of the `getTemplate()` method in a block?
The `getTemplate()` method retrieves the currently assigned template path for the block. This method is essential for ensuring that the correct template is rendered when the block is displayed.
Can I call multiple templates from a single block?
While a single block can only have one active template at a time, you can include multiple templates within that template using the `getLayout()->createBlock()` method to instantiate additional blocks.
How do I pass data to a template in Magento 2?
To pass data to a template, you can use the `setData()` method in your block class. For instance, `$this->setData(‘key’, ‘value’);` allows you to access the data in your template using `$block->getData(‘key’);`.
What is the correct directory structure for custom templates in Magento 2?
Custom templates should be placed in the following directory structure: `app/code/Vendor/Module/view/frontend/templates/`. Ensure that the template file has a `.phtml` extension for proper rendering.
How can I override a core block template in Magento 2?
To override a core block template, create a new template file in your custom module and specify the override in your module’s `layout` XML file. Use the `referenceBlock` directive to point to the block you want to override.
In Magento 2, calling a template within a block is a fundamental aspect of customizing the frontend experience. Developers can achieve this by utilizing the `setTemplate` method in their block class, which allows them to specify the path to the desired template file. This method is typically invoked within the block’s constructor or any other appropriate method, ensuring that the block renders the correct template when it is called in a layout XML file or directly in a PHTML file.
Moreover, it is essential to understand the directory structure where templates are stored. Magento 2 follows a specific convention for organizing templates, typically located in the `view/frontend/templates` directory of a module. By adhering to this structure, developers can maintain clarity and consistency in their code, making it easier to manage and update templates as needed.
Another important aspect to consider is the use of layout XML files to define how blocks and templates are rendered on the page. By specifying the block and its associated template in the layout XML, developers can control the output and placement of content on the frontend. This integration of blocks and templates through layout XML is a powerful feature that enhances the flexibility and scalability of Magento 2 themes.
In summary, effectively calling a template in
Author Profile
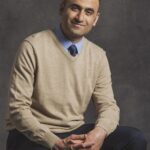
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?