How Can I Resolve ‘Malformed Input to a URL Function’ Errors?
In the vast and intricate world of web development, the ability to manipulate URLs is a fundamental skill that can make or break an application. However, even the most seasoned developers can encounter frustrating errors, particularly when faced with the dreaded “malformed input to a URL function.” This seemingly cryptic message can halt progress and leave developers scratching their heads, wondering what went wrong. Understanding the nuances of URL functions and the common pitfalls associated with malformed inputs is essential for anyone looking to create seamless web experiences.
A malformed URL input can arise from various sources, including improper formatting, unsupported characters, or even logical errors in the way URLs are constructed. These issues not only disrupt the flow of data but can also lead to security vulnerabilities if not addressed properly. As developers strive to build robust applications, recognizing the signs of malformed inputs and learning how to troubleshoot them is crucial for maintaining functionality and user trust.
In this article, we will delve into the intricacies of URL functions, exploring the common causes of malformed inputs and offering insights into best practices for URL management. By equipping yourself with this knowledge, you can enhance your web development skills and ensure that your applications run smoothly, providing users with the reliable experience they expect. Whether you’re a novice programmer or a seasoned expert, understanding how to navigate the
Understanding Malformed URLs
Malformed URLs are a common issue encountered in web development and API integration. A malformed URL can arise from various factors, including incorrect syntax, invalid characters, or improper encoding. When a URL is not formatted correctly, it can lead to errors such as “400 Bad Request” or “404 Not Found”. Understanding the structure of a URL can help in identifying and correcting these issues.
A standard URL consists of several components:
- Scheme: Indicates the protocol used (e.g., `http`, `https`, `ftp`).
- Host: The domain name or IP address of the server.
- Port: Optional; specifies the port number (default is 80 for HTTP, 443 for HTTPS).
- Path: Indicates the specific resource on the server.
- Query String: Optional; provides additional parameters (e.g., `?key=value`).
- Fragment: Optional; points to a specific part of the resource (e.g., `section`).
Here’s a breakdown of a URL for clarity:
Component | Example |
---|---|
Scheme | https |
Host | www.example.com |
Port | 443 |
Path | /path/to/resource |
Query String | ?id=123&sort=asc |
Fragment | section1 |
Common Causes of Malformed URLs
Several factors can contribute to the creation of malformed URLs:
- Incorrect Encoding: URLs must be properly encoded to ensure special characters are represented correctly. For example, spaces should be encoded as `%20`.
- Typographical Errors: Simple mistakes such as missing slashes or typos in domain names can result in a malformed URL.
- Improper Query Parameters: Unescaped characters in query parameters can lead to issues. For example, using `&` without encoding it can break the query string.
- Missing Components: URLs that omit necessary components like the scheme or host will fail to resolve.
Identifying and Fixing Malformed URLs
To effectively address malformed URLs, consider the following steps:
- Validation: Use tools or libraries to validate URLs against standard formats.
- Encoding: Ensure that all characters in the URL are properly encoded. Utilize functions like `encodeURIComponent()` in JavaScript for encoding query parameters.
- Testing: Regularly test URLs in different environments to catch issues early.
- Logging Errors: Implement logging to capture and analyze malformed URLs encountered by users or systems.
By adhering to these practices, developers can minimize the occurrence of malformed URLs and enhance the reliability of their web applications.
Understanding Malformed Input in URL Functions
Malformed input to a URL function typically occurs when the input provided does not conform to the expected format or structure that the function requires. This can result in errors or unexpected behavior when the URL is processed. Understanding the common causes and how to address them is crucial for developers and users alike.
Common Causes of Malformed Input
The following are frequent reasons why input may be considered malformed:
- Improper Encoding: Special characters in URLs must be encoded. For instance, spaces should be represented as `%20`, and characters like “, `&`, or `?` have specific meanings in URLs.
- Missing Components: A valid URL typically includes a scheme (e.g., `http://`), host (e.g., `example.com`), and optionally a path and query string. Omitting any of these can lead to malformed input.
- Excessive or Insufficient Slashes: URLs may not have the correct number of slashes. For example, `http:///example.com` is malformed due to an excess of slashes.
- Invalid Characters: URLs can only contain certain characters. For example, using characters like `<`, `>`, or `{` in a URL will lead to malformation.
- Incorrect Structure: The overall structure must follow the standard format: `scheme://host:port/path?queryfragment`.
Identifying Malformed Input
To identify malformed input, consider the following approaches:
- Validation Functions: Use built-in URL validation functions in programming languages. For example, in JavaScript, the `URL` constructor can throw errors when provided with malformed URLs.
- Regular Expressions: Implement regex patterns to validate URL formats. A basic pattern could look something like this:
“`regex
^(https?:\/\/)?([a-z0-9-]+\.)+[a-z]{2,}(:[0-9]{1,5})?(\/.*)?$
“`
- Error Handling: Implement robust error handling to capture exceptions thrown by URL functions. This can provide insight into what part of the input is malformed.
Best Practices for Avoiding Malformed Input
To mitigate issues with malformed URLs, consider the following best practices:
- Use Libraries: Rely on established libraries for URL manipulation, such as `urllib` in Python or `URL` in JavaScript, which handle encoding and validation.
- Sanitize Inputs: Always sanitize user inputs before processing them as URLs. This includes encoding special characters and validating against a whitelist.
- Testing and Logging: Implement comprehensive testing for URL functions and log malformed inputs for further analysis.
- User Feedback: Provide clear error messages to users when they input malformed URLs, guiding them on how to correct their input.
Example of Malformed Input Handling
Consider the following example in Python:
“`python
from urllib.parse import urlparse
def validate_url(url):
try:
parsed = urlparse(url)
if not all([parsed.scheme, parsed.netloc]):
raise ValueError(“Malformed URL: Missing scheme or netloc”)
return True
except Exception as e:
return str(e)
url_input = “http//example.com”
print(validate_url(url_input)) Output: “Malformed URL: Missing scheme or netloc”
“`
This code demonstrates a simple validation function that checks for the necessary components of a URL and returns an error message when the input is malformed.
Understanding the nuances of malformed input in URL functions is essential for effective web development and API integration. By implementing validation techniques, adhering to best practices, and utilizing robust libraries, developers can significantly reduce the incidence of malformed URLs in their applications.
Understanding Malformed Input in URL Functions
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Malformed input to a URL function can lead to significant vulnerabilities in web applications. It is crucial for developers to implement robust validation and sanitization processes to prevent injection attacks and ensure the integrity of the data being processed.”
James Lin (Cybersecurity Analyst, SecureWeb Solutions). “When handling URLs, malformed inputs can disrupt the expected flow of data and cause unexpected behavior in applications. Proper error handling and logging mechanisms are essential to identify and rectify these issues before they escalate into security breaches.”
Lisa Tran (Web Development Consultant, CodeSmart Agency). “In my experience, malformed input to URL functions often arises from user-generated content. Implementing strict input validation and encoding strategies can mitigate the risks associated with such inputs, ensuring a smoother user experience and maintaining application stability.”
Frequently Asked Questions (FAQs)
What does “malformed input to a URL function” mean?
Malformed input to a URL function refers to an incorrect or improperly formatted string that is intended to be processed as a URL. This can occur due to invalid characters, incorrect syntax, or missing components in the URL.
What are common causes of malformed URL inputs?
Common causes include the presence of spaces, unsupported characters, incorrect encoding, or missing protocol identifiers (e.g., “http://”). Additionally, typos or incomplete URLs can also lead to this error.
How can I fix a malformed URL input?
To fix a malformed URL input, ensure that the URL is properly formatted by checking for correct syntax, encoding special characters, and including all necessary components such as the protocol, domain, and path.
What tools can help identify malformed URLs?
Various tools can help identify malformed URLs, including online URL validators, web development tools in browsers, and programming libraries that provide URL parsing and validation functions.
Are there security implications of malformed URL inputs?
Yes, malformed URL inputs can pose security risks, such as injection attacks or exploitation of vulnerabilities in web applications. Proper validation and sanitization of URLs are essential to mitigate these risks.
Can malformed URL inputs affect web application performance?
Malformed URL inputs can negatively impact web application performance by causing errors, leading to failed requests, and increasing server load due to repeated attempts to process invalid URLs.
The issue of malformed input to a URL function typically arises when the input provided does not conform to the expected format or structure of a valid URL. This can lead to errors in web applications, where the URL is crucial for navigation and resource retrieval. Common causes of malformed URLs include incorrect encoding, missing components such as the protocol or domain, and the presence of illegal characters. Understanding these factors is essential for developers to ensure robust URL handling in their applications.
To mitigate the risks associated with malformed URLs, developers should implement comprehensive validation and sanitization processes. This includes using built-in functions or libraries that can automatically encode or decode URLs, ensuring that any user-generated input is properly formatted before being processed. Additionally, thorough testing should be conducted to identify potential edge cases that could lead to malformed URLs, which can help in preemptively addressing issues that may arise in production environments.
addressing malformed input to URL functions is a critical aspect of web development. By adhering to best practices in URL validation and encoding, developers can enhance the reliability and security of their applications. Ultimately, a proactive approach to handling URLs can prevent errors, improve user experience, and safeguard against potential vulnerabilities that may arise from improperly formatted URLs.
Author Profile
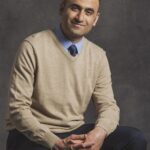
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?