How Do You Match RESTful API Methods to CRUD Functions?
In the ever-evolving landscape of web development, the integration of RESTful APIs has become a cornerstone for building robust and scalable applications. As developers strive to create seamless interactions between clients and servers, understanding the relationship between RESTful API methods and CRUD (Create, Read, Update, Delete) operations is essential. This connection not only enhances the efficiency of data management but also streamlines the development process, making it crucial for both novice and experienced programmers alike.
At the heart of RESTful architecture lies a set of standardized methods that correspond directly to the fundamental operations of data manipulation. These methods—GET, POST, PUT, PATCH, and DELETE—serve as the building blocks for CRUD functionality, each playing a unique role in how data is created, retrieved, modified, or deleted. By mapping these HTTP methods to their respective CRUD actions, developers can establish clear and intuitive interfaces that facilitate communication between the client and server.
As we delve deeper into this topic, we’ll explore how each RESTful method aligns with CRUD operations, providing practical insights and examples to illustrate their applications. Whether you’re looking to refine your API design skills or simply seeking to understand the underlying principles of RESTful services, this guide will equip you with the knowledge needed to harness the full potential of these powerful web technologies.
Mapping RESTful API Methods to CRUD Functions
In RESTful API design, the operations performed on resources are typically aligned with the standard CRUD (Create, Read, Update, Delete) functions. Understanding this mapping is crucial for developing and consuming APIs effectively. Below is a detailed overview of how each HTTP method corresponds to these CRUD operations.
HTTP Methods and Their Corresponding CRUD Functions
The primary HTTP methods used in RESTful APIs include GET, POST, PUT, PATCH, and DELETE. Each of these methods serves a specific purpose in relation to CRUD functionality:
HTTP Method | CRUD Function | Description |
---|---|---|
GET | Read | Retrieves data from the server. |
POST | Create | Submits data to be processed to the server, often resulting in a new resource. |
PUT | Update | Updates an existing resource with new data. |
PATCH | Update | Partially updates an existing resource. |
DELETE | Delete | Removes a resource from the server. |
Detailed Explanation of CRUD Operations
Creating, reading, updating, and deleting resources are the fundamental operations in a database, and they are mirrored in RESTful APIs through the aforementioned methods:
- Create (POST): This method is used when a client wants to create a new resource on the server. For example, submitting a form with user data could result in a new user being created in the database.
- Read (GET): The GET method is employed to retrieve data from the server without modifying it. For instance, retrieving a list of users or the details of a specific user.
- Update (PUT/PATCH):
- PUT is used when the client wants to replace the entire resource with new data. This is typically applied when a complete update is necessary.
- PATCH, on the other hand, is used for making partial updates to a resource, allowing clients to send only the fields that need to be updated.
- Delete (DELETE): This method is straightforward; it is used to remove a resource from the server. For example, deleting a user account.
Understanding the relationship between HTTP methods and CRUD operations is essential for effective API design and usage. By adhering to these conventions, developers can create APIs that are intuitive and easy to work with, enabling seamless interaction between clients and servers.
Mapping RESTful API Methods to CRUD Functions
RESTful APIs are designed around the principles of REST (Representational State Transfer), and they provide a structured way to interact with resources using standard HTTP methods. The CRUD operations—Create, Read, Update, and Delete—are fundamental to managing data within applications. Below is a detailed mapping of RESTful API methods to their corresponding CRUD functions.
CRUD Function | RESTful API Method | Description |
---|---|---|
Create | POST | Used to create a new resource on the server. |
Read | GET | Used to retrieve data from the server. |
Update | PUT/PATCH | PUT replaces the entire resource, while PATCH partially updates it. |
Delete | DELETE | Used to remove a resource from the server. |
Detailed Explanation of Each Method
POST (Create)
The POST method is used when a client wants to create a new resource on the server. This method sends data to the server, which then processes the request and creates the resource.
- Example: Sending a JSON object to create a new user in a database.
- URL Structure: `/api/users`
GET (Read)
GET is the method for retrieving data from the server. It is safe and idempotent, meaning it does not alter the state of the resource.
- Example: Fetching user information from a server.
- URL Structure: `/api/users/{id}` for a specific user, or `/api/users` for all users.
PUT/PATCH (Update)
The PUT method is primarily used to update an existing resource entirely, while PATCH is used for partial updates.
- PUT Example: Replacing a user’s entire information.
- URL Structure: `/api/users/{id}`
- PATCH Example: Modifying only the user’s email.
- URL Structure: `/api/users/{id}`
DELETE (Delete)
The DELETE method is utilized to remove a resource from the server. This operation is idempotent, meaning that multiple identical requests should have the same effect as a single request.
- Example: Deleting a user from the system.
- URL Structure: `/api/users/{id}`
Best Practices for Using RESTful Methods
To ensure effective utilization of RESTful API methods, consider the following best practices:
- Use Appropriate Methods: Match the HTTP method to the action being performed (CRUD).
- Idempotency: Ensure that methods like PUT and DELETE are idempotent; repeated calls should yield the same result.
- Statelessness: Keep each request independent by not relying on the server’s state.
- Resource Naming: Use nouns for resource names in URLs, avoiding verbs.
- Versioning: Implement API versioning to manage changes over time without disrupting existing clients.
By adhering to these principles, developers can create robust and intuitive RESTful APIs that align closely with CRUD operations.
Aligning RESTful API Methods with CRUD Operations
Dr. Emily Tran (Lead Software Architect, Tech Innovations Inc.). “Understanding the relationship between RESTful API methods and CRUD operations is essential for effective software design. The HTTP methods GET, POST, PUT, and DELETE directly correspond to the operations of Read, Create, Update, and Delete, respectively. This alignment ensures that APIs are intuitive and adhere to standard practices, facilitating easier integration and maintenance.”
Michael Chen (Senior Backend Developer, Cloud Solutions Co.). “In my experience, mapping RESTful methods to CRUD functions not only enhances the clarity of API design but also improves the overall user experience. For instance, using GET for retrieving data ensures that clients can easily understand how to interact with the API. This consistency is crucial when developing scalable applications.”
Laura Martinez (API Specialist, Digital Services Group). “It’s vital to maintain a clear mapping of RESTful methods to CRUD operations to avoid confusion among developers. For example, using POST for creating resources and PUT for updating them helps maintain a predictable structure. This predictability is key in collaborative environments where multiple developers may be interacting with the same API.”
Frequently Asked Questions (FAQs)
What RESTful API method corresponds to the Create function in CRUD?
The RESTful API method that corresponds to the Create function in CRUD is the POST method. It is used to submit data to be processed to a specified resource.
Which RESTful API method is used for Read operations in CRUD?
The GET method is used for Read operations in CRUD. It retrieves data from a specified resource without modifying it.
What RESTful API method is associated with the Update function in CRUD?
The PUT method is typically associated with the Update function in CRUD. It is used to update an existing resource with new data.
Is there a specific RESTful API method for the Delete function in CRUD?
Yes, the DELETE method is specifically used for the Delete function in CRUD. It removes a specified resource from the server.
Can the PATCH method be used in CRUD operations?
Yes, the PATCH method can be used in CRUD operations as an alternative to the PUT method. It is used for partial updates to an existing resource.
How do RESTful API methods enhance CRUD operations?
RESTful API methods enhance CRUD operations by providing a standardized way to interact with resources over HTTP, ensuring clear and predictable behavior for data manipulation.
In the realm of web development, understanding the relationship between RESTful API methods and CRUD (Create, Read, Update, Delete) operations is fundamental. RESTful APIs utilize specific HTTP methods to perform corresponding CRUD functions, which facilitates the management of resources over the web. The primary methods include POST for Create, GET for Read, PUT/PATCH for Update, and DELETE for Delete. Each method serves a distinct purpose and adheres to the principles of stateless communication that define RESTful architecture.
The POST method is employed to create new resources on the server. When a client sends a POST request, it typically includes the necessary data for the new resource, which the server processes and stores. Conversely, the GET method retrieves existing resources, allowing clients to access data without altering it. This method is crucial for reading and displaying information stored on the server.
For updating resources, the PUT and PATCH methods are used. PUT replaces the entire resource with the new data provided, while PATCH allows for partial updates, modifying only specific fields of the resource. This distinction is essential for developers to choose the appropriate method based on the requirements of their application. Finally, the DELETE method is straightforward, as it removes resources from the server, completing the CRUD cycle.
Author Profile
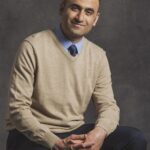
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?