How Can You Effectively Merge Two Columns in SQL?
In the world of databases, data manipulation is a crucial skill that can significantly enhance the efficiency and clarity of your data management processes. One common task that many SQL users encounter is the need to merge two columns into a single column. Whether you’re looking to combine first and last names into a full name, concatenate address components, or unify disparate data points, mastering this technique can streamline your queries and improve data presentation. In this article, we will explore the various methods and functions available in SQL to effectively merge columns, providing you with the tools to transform your data in meaningful ways.
Merging two columns in SQL is not only a practical necessity but also a powerful way to create more readable and usable datasets. This task can be accomplished using various SQL functions, depending on the database system you’re working with. By understanding the syntax and options available, you can easily concatenate strings and format your output to suit your needs. Additionally, merging columns can help eliminate redundancy and enhance the overall structure of your data, making it easier to analyze and report on.
As we delve deeper into the intricacies of merging columns, we will examine the different approaches available in popular SQL dialects, including the use of the `CONCAT` function, string operators, and even case-specific methods. By the end of
Merging Two Columns in SQL
Merging two columns in SQL typically involves concatenating the values of those columns into a single output. This can be accomplished using various SQL functions, depending on the database system in use. The most common methods include the use of the `CONCAT` function, the `||` operator, or the `+` operator. Below are examples demonstrating how to achieve this in different SQL dialects.
Using the CONCAT Function
The `CONCAT` function is widely supported across various SQL databases such as MySQL, SQL Server, and PostgreSQL. This function allows you to merge multiple strings into one.
Example:
“`sql
SELECT CONCAT(first_name, ‘ ‘, last_name) AS full_name
FROM employees;
“`
In this example, `first_name` and `last_name` columns are merged, with a space added between them for clarity.
Using the || Operator
In databases like PostgreSQL and SQLite, the `||` operator can be used for string concatenation.
Example:
“`sql
SELECT first_name || ‘ ‘ || last_name AS full_name
FROM employees;
“`
This approach also merges the `first_name` and `last_name` columns with a space in between.
Using the + Operator
For SQL Server, the `+` operator is used for concatenation.
Example:
“`sql
SELECT first_name + ‘ ‘ + last_name AS full_name
FROM employees;
“`
Just like in the previous examples, this method concatenates the names with a space.
Handling NULL Values
When merging columns, handling `NULL` values is crucial to avoid unexpected results. The behavior differs depending on the method used:
- With `CONCAT`, `NULL` values are treated as empty strings.
- Using `||` or `+` will result in a `NULL` output if any operand is `NULL`.
To ensure that `NULL` values do not disrupt the concatenation, you can use the `COALESCE` function:
Example:
“`sql
SELECT COALESCE(first_name, ”) || ‘ ‘ || COALESCE(last_name, ”) AS full_name
FROM employees;
“`
This guarantees that if either name is `NULL`, it is treated as an empty string.
Performance Considerations
Merging columns in SQL can have performance implications, especially when dealing with large datasets. Here are some considerations:
- Indexing: Ensure that the columns you are merging are indexed if you frequently perform queries on the result.
- Data Types: Be mindful of data types being merged. Implicit type conversion can add overhead.
- Query Optimization: Analyze the execution plan to identify any bottlenecks that might arise from concatenation operations.
Example Table
The following table illustrates a simple dataset for merging columns:
first_name | last_name |
---|---|
John | Doe |
Jane | Smith |
NULL | Brown |
Using the examples and considerations outlined, you can effectively merge columns in SQL while taking care of potential issues like `NULL` values and performance.
Merging Two Columns in SQL
Merging two columns in SQL can be accomplished using various functions depending on the SQL dialect in use. The most common approach involves utilizing the `CONCAT()` function or the concatenation operator (`||` or `+`), depending on the database system.
Using CONCAT() Function
The `CONCAT()` function allows you to merge two or more strings into a single string. Below is the syntax and an example of how to use it:
“`sql
SELECT CONCAT(column1, ‘ ‘, column2) AS merged_column
FROM table_name;
“`
- Parameters:
- `column1`: First column to merge.
- `column2`: Second column to merge.
- `’ ‘` (space): Optional, used to add a space between the two merged columns.
Example:
“`sql
SELECT CONCAT(first_name, ‘ ‘, last_name) AS full_name
FROM employees;
“`
This query combines the `first_name` and `last_name` columns from the `employees` table to produce a `full_name`.
Using the Concatenation Operator
Different SQL databases use different operators for concatenation. Here’s a brief overview:
Database | Concatenation Operator | ||
---|---|---|---|
MySQL | `CONCAT()` | ||
PostgreSQL | ` | ` | |
SQL Server | `+` | ||
Oracle | ` | ` |
Examples:
- PostgreSQL:
“`sql
SELECT column1 || ‘ ‘ || column2 AS merged_column
FROM table_name;
“`
- SQL Server:
“`sql
SELECT column1 + ‘ ‘ + column2 AS merged_column
FROM table_name;
“`
- Oracle:
“`sql
SELECT column1 || ‘ ‘ || column2 AS merged_column
FROM table_name;
“`
Handling NULL Values
When merging columns, it is important to consider how NULL values are treated, as they can affect the output. Most SQL functions return NULL if any concatenated value is NULL. To handle this, you can use the `COALESCE()` function.
Example:
“`sql
SELECT CONCAT(COALESCE(column1, ”), ‘ ‘, COALESCE(column2, ”)) AS merged_column
FROM table_name;
“`
This will replace NULL values with an empty string, ensuring that the concatenation does not result in NULL.
Practical Use Cases
Merging columns is useful in various scenarios, such as:
- Creating Full Names: Combining first and last names for user-friendly display.
- Address Formatting: Merging street, city, and state columns into a single address string.
- Generating Descriptive Labels: Creating more informative labels by combining various attributes.
Performance Considerations
While merging columns can enhance readability and usability, consider the following performance aspects:
- Indexing: Merging columns can complicate indexing strategies. Consider keeping the original columns indexed if needed.
- Query Complexity: Adding concatenation in SELECT statements can increase query complexity. Optimize where necessary.
- Data Types: Ensure proper data types are used for concatenation to avoid type conversion errors.
Utilizing the appropriate SQL functions and operators for merging columns will enhance data presentation and usability while maintaining performance.
Expert Insights on Merging Two Columns in SQL
Dr. Emily Chen (Database Architect, Tech Innovations Inc.). “Merging two columns in SQL can be efficiently accomplished using the CONCAT function or the concatenation operator, depending on the SQL dialect. It is crucial to handle NULL values appropriately to avoid unexpected results in your queries.”
Mark Thompson (Senior SQL Developer, Data Solutions Group). “When merging columns, especially in large datasets, performance considerations are vital. Utilizing indexes and ensuring that the merged columns are of compatible data types can significantly enhance query execution times.”
Lisa Patel (Data Analyst, Insight Analytics). “I recommend using the COALESCE function when merging columns that may contain NULLs. This approach allows you to specify fallback values, ensuring that your merged results are both meaningful and complete.”
Frequently Asked Questions (FAQs)
What is the SQL syntax for merging two columns?
To merge two columns in SQL, you can use the `CONCAT()` function or the `||` operator, depending on the database system. For example, `SELECT CONCAT(column1, column2) AS merged_column FROM table_name;` merges `column1` and `column2`.
Can I add a separator when merging two columns?
Yes, you can include a separator by modifying the `CONCAT()` function. For example, `SELECT CONCAT(column1, ‘ ‘, column2) AS merged_column FROM table_name;` adds a space between the merged values.
Is it possible to merge more than two columns in SQL?
Yes, you can merge multiple columns by including them all in the `CONCAT()` function. For instance, `SELECT CONCAT(column1, ‘ ‘, column2, ‘ ‘, column3) AS merged_column FROM table_name;` merges three columns with spaces in between.
What happens to NULL values when merging columns?
When using `CONCAT()`, NULL values are treated as empty strings. For example, if `column1` is NULL and `column2` contains a value, the result will be the value of `column2`. However, behavior may vary in different SQL implementations.
Can I update a column with the merged values of two other columns?
Yes, you can update a column with the merged values using the `UPDATE` statement. For example, `UPDATE table_name SET target_column = CONCAT(column1, ‘ ‘, column2);` updates `target_column` with the merged values of `column1` and `column2`.
Are there performance considerations when merging columns in SQL?
Merging columns can impact performance, especially with large datasets. It is advisable to limit the number of columns merged and to consider indexing strategies if necessary. Always test performance in your specific environment.
Merging two columns in SQL is a common task that can be accomplished through various methods, depending on the specific requirements of the database and the desired output. The most frequently used approach involves the use of the `CONCAT()` function or the concatenation operator (`||` or `+`, depending on the SQL dialect). This allows users to combine the values of two or more columns into a single output column, which can be particularly useful for formatting data or generating full names from separate first and last name columns.
Additionally, the `COALESCE()` function can be employed to handle NULL values effectively when merging columns. This function returns the first non-null value in the list of arguments, ensuring that the merged result does not produce unexpected NULLs when one of the columns is empty. Understanding how to manipulate and merge data is crucial for data analysis and reporting, as it enhances the clarity and usability of the information presented.
In summary, merging columns in SQL is a straightforward process that can significantly improve data presentation and usability. By leveraging functions like `CONCAT()` and `COALESCE()`, users can create more informative and comprehensive datasets. Mastery of these techniques not only streamlines data management but also empowers users to generate insights that drive
Author Profile
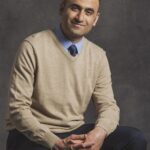
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?