How Can You Use the Meta Box View Function to Retrieve Information from wp_terms?
In the world of WordPress development, customizations and enhancements are the lifeblood of creating unique user experiences. One powerful tool at a developer’s disposal is the meta box, which allows for the addition of custom fields in the WordPress admin area. These fields can be used to gather and display a wide range of information, making them invaluable for tailoring content management to specific needs. But what if you want to pull in data from the taxonomy system, specifically the `wp_terms` table? Understanding how to leverage the meta box view function to access and manipulate this data can elevate your WordPress projects to new heights.
The `wp_terms` table is a core component of WordPress’s taxonomy system, storing essential information about categories, tags, and custom taxonomies. By integrating this data into your custom meta boxes, you can create a seamless interface that allows users to select or display terms directly from the admin panel. This not only streamlines the content creation process but also enhances the overall functionality of your site. Whether you’re building a custom post type or simply looking to enrich existing content, tapping into the `wp_terms` data can provide a wealth of possibilities.
In this article, we will explore the intricacies of using the meta box view function to access information from the `
Understanding the Meta Box View Function
In WordPress development, particularly when working with custom post types and taxonomies, the meta box view function serves as a crucial tool for retrieving and displaying information associated with `wp_terms`. This function allows developers to create a user-friendly interface in the WordPress admin area, enabling the management of term data effectively.
To create a meta box view function that retrieves information from `wp_terms`, follow these key steps:
- Register the Meta Box: Use the `add_meta_box` function to add a meta box to the desired admin page.
- Define the Callback Function: This function will fetch data from the `wp_terms` table.
- Display the Retrieved Data: Format the output in a way that is easy for users to read and interact with.
Example of a Meta Box View Function
Here’s a simple example of a meta box view function that retrieves and displays terms associated with a custom taxonomy.
“`php
function custom_meta_box_view($post) {
$terms = get_terms(array(
‘taxonomy’ => ‘your_custom_taxonomy’,
‘hide_empty’ => ,
));
if (!empty($terms) && !is_wp_error($terms)) {
echo ‘
Term ID | Term Name |
---|---|
‘ . esc_html($term->term_id) . ‘ | ‘ . esc_html($term->name) . ‘ |
‘;
} else {
echo ‘No terms found.’;
}
}
“`
The above code registers a meta box and retrieves terms from a custom taxonomy. It checks for errors and formats the output in a table for clarity.
Key Functions for Term Information Retrieval
When working with `wp_terms`, several key functions are essential for efficient data retrieval:
- get_terms(): Retrieves terms from a specified taxonomy.
- get_term(): Fetches a single term by its ID.
- wp_get_post_terms(): Gets the terms associated with a particular post.
Best Practices for Implementing Meta Box View Functions
To ensure the effectiveness of your meta box view functions, consider the following best practices:
- Sanitize Output: Always use escaping functions such as `esc_html()` to prevent XSS vulnerabilities.
- User Experience: Ensure that the display is clean and intuitive, allowing users to easily understand the information presented.
- Conditional Logic: Implement checks to handle cases when no terms are found or when an error occurs.
Table of Common Functions for Working with Terms
Function | Description |
---|---|
get_terms() | Retrieves an array of term objects from a specified taxonomy. |
get_term() | Fetches a single term object by its ID. |
wp_get_post_terms() | Gets terms associated with a given post ID. |
wp_insert_term() | Adds a new term to a taxonomy. |
By following these guidelines, developers can create effective meta box view functions that enhance the WordPress admin experience, facilitating better management of terms and associated data.
Understanding the Meta Box View Function
The meta box view function is a crucial component for developers looking to retrieve information from the `wp_terms` table in WordPress. This function allows for the efficient display and management of taxonomy terms within the WordPress admin area.
Retrieving Term Information
To access term information from the `wp_terms` table, the following methods can be employed:
– **Using `get_terms()` Function**: This function retrieves terms from a specific taxonomy.
“`php
$terms = get_terms(array(
‘taxonomy’ => ‘category’,
‘hide_empty’ => ,
));
“`
- Using `get_term()` Function: This function fetches a single term by its ID.
“`php
$term = get_term($term_id, ‘category’);
“`
- Using `wp_get_post_terms()`: This function is useful for retrieving terms associated with a specific post.
“`php
$post_terms = wp_get_post_terms($post_id, ‘category’);
“`
Creating a Meta Box
To create a meta box that displays terms, you can utilize the following code structure. This includes registering the meta box and rendering the terms within it.
“`php
function my_custom_meta_box() {
add_meta_box(
‘my_meta_box_id’,
‘My Meta Box’,
‘render_my_meta_box’,
‘post’,
‘side’,
‘high’
);
}
add_action(‘add_meta_boxes’, ‘my_custom_meta_box’);
function render_my_meta_box($post) {
$terms = get_terms(array(
‘taxonomy’ => ‘category’,
‘hide_empty’ => ,
));
if (!empty($terms) && !is_wp_error($terms)) {
echo ‘
- ‘;
- ‘ . esc_html($term->name) . ‘
foreach ($terms as $term) {
echo ‘
‘;
}
echo ‘
‘;
}
}
“`
Displaying Terms in the Meta Box
When rendering the terms in your meta box, consider the following:
- HTML Structure: Use lists or tables for better readability.
- Styling: Use CSS classes to enhance the appearance of the meta box.
Example of a styled display:
“`html
- Term 1
- Term 2
“`
Saving Term Data
If you need to save selected terms when a post is saved, it is essential to implement a save function. Use `save_post` action to handle the saving process.
“`php
function save_my_meta_box_data($post_id) {
if (array_key_exists(‘my_meta_box_field’, $_POST)) {
wp_set_post_terms($post_id, $_POST[‘my_meta_box_field’], ‘category’);
}
}
add_action(‘save_post’, ‘save_my_meta_box_data’);
“`
Best Practices
When working with meta boxes and terms, adhere to the following best practices:
- Sanitize Input: Always sanitize user input to prevent security vulnerabilities.
- Use Nonce Fields: Implement nonce fields for verification during form submissions.
- Optimize Queries: Limit the number of terms retrieved to enhance performance.
- User Experience: Ensure the interface is user-friendly and intuitive for content editors.
By following the outlined methods and practices, you can effectively manage and display term information from the `wp_terms` table within WordPress meta boxes.
Expert Insights on Using Meta Box View Functions to Access WP_Terms Information
Jessica Harmon (WordPress Developer and Plugin Specialist). “Utilizing meta box view functions to retrieve information from wp_terms can significantly enhance the user experience in custom post types. By leveraging these functions, developers can create intuitive interfaces that allow for seamless term management directly within the WordPress admin.”
Michael Chen (Senior Software Engineer, WP Solutions Inc.). “The integration of meta box view functions with wp_terms is crucial for maintaining data integrity and improving performance. By implementing these functions correctly, developers can ensure that taxonomy data is efficiently accessed and displayed, which is vital for large-scale WordPress sites.”
Laura Green (Content Management Systems Consultant). “When working with meta boxes, understanding how to effectively pull information from wp_terms can streamline content categorization. This not only helps in organizing content but also plays a significant role in SEO, as it allows for better structuring of related content.”
Frequently Asked Questions (FAQs)
What is a meta box view function in WordPress?
A meta box view function in WordPress is a custom function that displays additional information or fields in the WordPress admin area, typically within the post or page edit screen. It allows developers to enhance the editing experience by providing structured data input.
How can I retrieve information from wp_terms using a meta box view function?
To retrieve information from `wp_terms`, you can use the `get_terms()` function within your meta box view function. This function allows you to query terms based on taxonomy, and you can then display the relevant information in your meta box.
What parameters can I use with get_terms() to filter results?
You can use parameters such as ‘taxonomy’, ‘hide_empty’, ‘orderby’, ‘order’, and ‘number’ to filter the results returned by `get_terms()`. This allows for precise control over which terms are retrieved based on your specific requirements.
Can I display custom fields associated with terms in my meta box?
Yes, you can display custom fields associated with terms in your meta box. You can retrieve custom field values using the `get_term_meta()` function, allowing you to present additional information alongside the term data.
Is it possible to save data from a meta box that interacts with wp_terms?
Yes, you can save data from a meta box that interacts with `wp_terms`. You will need to use the `save_post` action hook to process and store the data when the post is saved, ensuring that the information is linked to the appropriate terms.
How do I ensure my meta box is displayed on the appropriate admin pages?
You can specify the admin pages where your meta box should appear by using the `add_meta_box()` function with the correct screen identifier. This allows you to control the context and placement of your meta box within the WordPress admin interface.
The meta box view function in WordPress serves as a crucial tool for retrieving and displaying information from the `wp_terms` table. This table is fundamental to the taxonomy system in WordPress, as it stores the terms associated with taxonomies such as categories and tags. By utilizing a meta box view function, developers can effectively query and present this data within the WordPress admin interface, enhancing the user experience and providing valuable insights into the site’s taxonomy structure.
One of the key takeaways from the discussion on this topic is the importance of understanding the relationship between terms and their associated taxonomy. Developers can leverage this relationship to create custom meta boxes that provide users with relevant information about terms, including their descriptions, counts, and hierarchical structures. This not only aids in content management but also allows for more informed decision-making regarding term usage and organization.
Moreover, implementing a meta box view function can streamline the workflow for content creators and site administrators. By presenting term information directly within the post editing screen, users can quickly access and manage their taxonomies without navigating away from their current task. This integration fosters a more efficient content management process, ultimately leading to a more organized and user-friendly website.
Author Profile
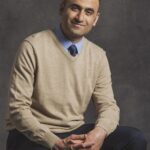
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?