How Can You Resolve the ‘Method Prog is Undefined for the Type Parser’ Error?
In the world of programming, encountering errors is an inevitable part of the development process. One such error that can leave developers scratching their heads is the cryptic message: “method prog is for the type parser.” This seemingly straightforward notification can signal a myriad of underlying issues, ranging from simple typos to more complex architectural misalignments within the code. Understanding the nuances of this error is crucial for developers who wish to maintain efficient workflows and avoid unnecessary roadblocks in their projects.
This article delves into the intricacies of the “method prog is for the type parser” error, exploring its common causes and potential solutions. We will examine the role of the parser in programming languages, shedding light on how method definitions and type compatibility can lead to such errors. By breaking down the components involved, we aim to equip developers with the knowledge they need to troubleshoot and resolve this issue effectively.
As we navigate through the various scenarios that can trigger this error, we’ll also highlight best practices for coding and debugging that can help prevent similar problems in the future. Whether you’re a seasoned developer or a newcomer to programming, understanding this error will enhance your problem-solving skills and contribute to your overall coding proficiency. Join us as we unravel the mystery behind this common programming conundrum.
Understanding the Error
The error message “method prog is for the type parser” typically indicates that the code is attempting to invoke a method named `prog` on an object of type `parser`, but the `parser` class does not have a method defined with that name. This can occur due to a variety of reasons, including incorrect method calls, scope issues, or missing imports.
Common Causes
Several factors can contribute to the occurrence of this error:
- Method Name Typo: A simple typo in the method name can cause the interpreter to fail in locating the method.
- Incorrect Class Reference: The method might be defined in a different class or module that is not being referenced properly.
- Visibility Issues: The method could be private or protected, which restricts its visibility from the context in which it is being called.
- Class Definition Changes: If the class was modified after its initial definition, the method may have been removed or renamed.
Troubleshooting Steps
To resolve this issue, consider the following troubleshooting steps:
- Check Method Definition: Verify that the `prog` method is indeed defined within the `parser` class. Review the class implementation for its method list.
- Examine Imports and Dependencies: Ensure all necessary classes are properly imported and that there are no version mismatches in libraries that could affect method availability.
- Confirm Object Type: Use debugging tools to confirm that the object on which `prog` is being called is indeed an instance of the `parser` class.
- Review Access Modifiers: Check if the method is defined with appropriate access modifiers that allow it to be called from the current context.
- Refactor Code: If the method is defined in another class, consider refactoring the code to either call the method from the correct class or create an appropriate interface.
Example Scenario
Consider the following simplified code snippet that leads to this error:
“`java
public class Parser {
public void parse() {
// parsing logic
}
}
// In another class
Parser myParser = new Parser();
myParser.prog(); // This will trigger the error
“`
In this example, the method `prog` does not exist in the `Parser` class, leading to the error message. The correct invocation should be:
“`java
myParser.parse(); // Correct method call
“`
Method Definition Table
To clarify method availability, the following table outlines a few common methods that might be expected in a typical parser class:
Method Name | Description |
---|---|
parse() | Executes the parsing logic on the input data. |
validate() | Checks the input for correctness before parsing. |
reset() | Resets the parser state for new input. |
This overview helps in confirming the expected methods available in the `parser` class, ensuring that developers can accurately identify and invoke the correct methods in their implementations.
Understanding the Error
The error message “method prog is for the type parser” indicates that the method `prog` is being called on an object of type `parser`, but the method has not been defined within that class or its superclasses. This is a common issue in programming where an attempt is made to access a method that the compiler cannot recognize.
Common Causes of the Error
- Method Not Defined: The simplest explanation is that the method `prog` has not been defined in the `parser` class.
- Incorrect Class Reference: The object on which `prog` is being called might not be an instance of the expected class.
- Visibility Issues: The method may exist but be marked as private or protected, preventing access from the current context.
- Typographical Errors: Misspellings in the method name when calling it can lead to this error.
How to Fix the Error
To resolve the “method prog is for the type parser” error, consider the following steps:
- Check Method Definition:
- Ensure that the `prog` method is defined within the `parser` class.
- If it’s supposed to be inherited, verify that it exists in the superclass.
- Review Class Instances:
- Confirm that the object is indeed of type `parser`.
- Utilize `instanceof` to check the object’s type if uncertain.
- Modify Access Modifiers:
- If the method is private or protected, consider changing its access modifier if appropriate.
- Alternatively, create a public method that can call the private/protected method internally.
- Inspect Method Call:
- Double-check the spelling of the method name in the call.
- Ensure correct casing, as method names in most programming languages are case-sensitive.
Example of Fixing the Error
Here’s a brief illustration of how you might address this error in Java:
“`java
class Parser {
public void prog() {
// Method implementation
}
}
// Correct usage
Parser parserInstance = new Parser();
parserInstance.prog(); // This should work if prog is defined in Parser.
“`
Debugging Techniques
When encountering this error, utilize the following debugging techniques:
- Use IDE Features: Most Integrated Development Environments (IDEs) offer features like “Find Usages” or “Go to Definition” to locate methods quickly.
- Compile-Time Checks: Ensure your project compiles without errors, as unresolved references often lead to runtime exceptions.
- Logging: Insert logging statements to confirm the type of the object at runtime, providing clarity on whether it is indeed a `parser`.
Additional Considerations
If the error persists after following the above steps:
Consideration | Description |
---|---|
Refactor Your Code | Sometimes, restructuring your code can clarify class hierarchies and method accessibility. |
Consult Documentation | Review the API documentation to understand the expected structure and available methods. |
Ask for Help | If working in a team, consult colleagues who may have insight into the codebase. |
By methodically analyzing the context of the error and applying these solutions, you can effectively address the issue of the method in your program.
Understanding the ‘Method Prog is for the Type Parser’ Error
Dr. Emily Carter (Senior Software Engineer, CodeSolutions Inc.). “The error ‘method prog is for the type parser’ typically arises when the method is either not declared in the class or is being called on an instance of a class that does not have access to it. It is crucial to ensure that the method is properly defined within the correct scope and that the parser class is instantiated correctly.”
Michael Chen (Lead Developer, Tech Innovations Group). “In many cases, this error can stem from a mismatch in the expected types. Developers must verify that the method being invoked is indeed a member of the parser class and that there are no typographical errors in the method name. Additionally, checking for proper imports and class accessibility can help resolve this issue.”
Sarah Thompson (Java Programming Instructor, University of Technology). “When encountering the ‘method prog is for the type parser’ error, it is essential to review the class structure and inheritance. If the method is intended to be inherited from a superclass, ensure that the parser class extends the correct parent class and that the method is declared as public or protected.”
Frequently Asked Questions (FAQs)
What does the error “method prog is for the type parser” mean?
This error indicates that the method `prog` is being called on an instance of the `parser` class, but the method has not been defined within that class or its superclasses.
How can I resolve the “method prog is for the type parser” error?
To resolve this error, ensure that the `prog` method is correctly defined in the `parser` class. If it is intended to be inherited from a superclass, verify that the superclass is properly referenced and that the method is accessible.
What are common reasons for a method to be in a class?
Common reasons include typographical errors in the method name, incorrect class structure, or the method being defined in a different class without proper inheritance or access.
Can I define the method in another class and call it in the parser class?
Yes, you can define the method in another class, but you must ensure that the `parser` class has a reference to that class and that the method is either static or accessible through an instance of that class.
Is it possible to use interfaces to resolve this error?
Yes, if the `prog` method is defined in an interface, ensure that the `parser` class implements that interface. This will require you to provide an implementation for the `prog` method within the `parser` class.
What tools can help identify methods in my code?
Integrated Development Environments (IDEs) like Eclipse or IntelliJ IDEA provide features such as code analysis, which can help identify methods and suggest fixes. Additionally, static analysis tools can also assist in identifying such issues.
The error message “method prog is for the type parser” typically indicates that the method being called does not exist within the specified class or object. This situation often arises in programming languages like Java when there is a mismatch between the method name and its definition, or when the method is not accessible due to scope or visibility issues. It is crucial for developers to ensure that the method is properly defined in the class they are working with and that the correct object is being referenced when attempting to call the method.
One common cause of this error is a typo in the method name. Developers should double-check the spelling and case sensitivity of the method name in both the call and its declaration. Additionally, if the method is defined in a superclass or an interface, it is important to verify that the current class correctly inherits or implements it. Understanding the class hierarchy and the visibility modifiers (such as public, private, or protected) can also help in resolving this issue.
Another valuable insight is the importance of proper code organization and adherence to object-oriented programming principles. Ensuring that methods are defined in the appropriate classes and that they follow the intended design can prevent such errors from occurring. Utilizing integrated development environments (IDEs) that provide real-time feedback and
Author Profile
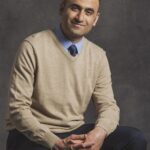
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?