Why Am I Seeing a ‘Missing Key Prop for Element in Iterator’ Warning in My React App?
In the world of web development, particularly when working with libraries like React, efficiency and clarity in rendering lists of elements can make all the difference. One common pitfall that developers encounter is the infamous warning about a “missing key prop for element in iterator.” This seemingly innocuous message can lead to a cascade of issues in your application, affecting performance and even causing unexpected behavior. Understanding the significance of the key prop is essential for anyone looking to create robust, scalable web applications.
When rendering lists of components, React requires a unique identifier for each item to optimize the rendering process. This is where the key prop comes into play. Without it, React struggles to track changes, leading to inefficient updates and potential bugs. The key prop not only helps React identify which items have changed, been added, or removed, but it also plays a crucial role in ensuring that your application runs smoothly and efficiently.
In this article, we will delve into the importance of the key prop, explore common mistakes developers make when using iterators, and provide best practices for implementing keys in your React applications. Whether you’re a seasoned developer or just starting your journey in web development, understanding this concept will empower you to build better, more performant applications.
Understanding the Importance of Key Props
When working with lists in React, the `key` prop is crucial for ensuring that components are efficiently updated. The key prop is a special attribute that helps React identify which items have changed, are added, or are removed. This identification process allows React to optimize rendering and improve performance.
Key props should be assigned to the elements in an array or iterator. Without unique keys, React will throw a warning indicating that a missing key prop is present. This can lead to unpredictable behavior in your application, including:
- Performance issues: React may not re-render components efficiently.
- Incorrect UI updates: Changes in the order of items may not reflect correctly.
- State loss: The state may not be preserved as expected when items are reordered.
Best Practices for Using Key Props
To avoid the warning about missing key props, follow these best practices:
– **Use unique identifiers**: Whenever possible, use unique IDs from your data, such as database IDs.
– **Avoid using array indexes**: Using indexes as keys can lead to performance issues and bugs when the list changes.
– **Ensure keys are stable**: Keys should not change between renders. If an item’s key changes, it can cause React to treat it as a new component.
Here is a simple example of how to implement keys correctly:
“`jsx
const items = [{ id: 1, name: ‘Item 1’ }, { id: 2, name: ‘Item 2’ }];
const ItemList = () => (
-
{items.map(item => (
- {item.name}
))}
);
“`
Common Errors Related to Missing Key Props
While developing React applications, you may encounter several common scenarios that lead to missing key prop warnings. Below are some examples:
Scenario | Description |
---|---|
Iterating over static data | Forgetting to add keys when rendering a static list. |
Using map without keys | Directly mapping over an array without assigning key props. |
Conditional rendering in lists | Failing to provide keys in lists that conditionally render items. |
To address these issues, make sure you always provide a key prop, especially in dynamic lists where data may change over time.
Debugging Key Prop Warnings
When you encounter a warning about a missing key prop, consider the following steps to debug:
- Locate the warning: Check the console for the exact component causing the warning.
- Review your map function: Ensure that you are providing a key for each child component.
- Consider the structure of your data: Verify that the data being iterated over has unique identifiers.
- Refactor if necessary: If your current data structure does not provide unique keys, consider restructuring the data to include them.
By following these guidelines and understanding the significance of key props, you can enhance the performance and reliability of your React applications.
Understanding the Warning
When using React, developers may encounter a warning about missing keys in iterators. This warning typically appears when rendering a list of elements, and it indicates that React requires a unique “key” prop for each child in a list. The purpose of the key prop is to help React identify which items have changed, are added, or are removed, enabling efficient updates to the UI.
Reasons for the Warning:
- Enhances performance during re-renders by allowing React to track elements.
- Prevents potential issues with component state and behavior when the list changes.
Key Prop Implementation
To effectively use the key prop, it is essential to provide a unique identifier for each item being rendered in a list. Here are some best practices for implementing the key prop:
– **Use Unique IDs**: Whenever possible, use a unique identifier from the data source.
– **Avoid Using Indexes**: Using array indexes as keys can lead to issues, especially when items are reordered or removed.
– **Generate Unique Keys**: If no unique ID is available, consider generating a unique key using a combination of properties.
**Example Implementation:**
“`jsx
const items = [
{ id: 1, name: ‘Item 1’ },
{ id: 2, name: ‘Item 2’ },
{ id: 3, name: ‘Item 3’ }
];
const ItemList = () => (
-
{items.map(item => (
- {item.name}
))}
);
“`
Common Mistakes
Several common pitfalls can lead to the key prop warning. Understanding these mistakes can help avoid the warning:
- Duplicating Keys: Ensure that each key is unique across the entire array.
- Not Specifying Keys: Always provide a key prop when rendering elements in a loop.
- Changing Keys: Avoid changing the key prop dynamically in a way that confuses React’s reconciliation process.
Debugging Key Prop Issues
When debugging the key prop warning, consider the following steps:
- Inspect the List: Review the list being rendered to ensure that each element has a unique key.
- Check Data Structure: Validate that the data structure used for rendering contains unique identifiers.
- Console Warnings: Pay attention to the console warnings during development, as they provide insights into which elements are missing keys.
Performance Implications
Not using keys properly can lead to performance degradation. When React does not have keys to track elements, it may resort to inefficient rendering strategies. This can result in:
- Increased rendering time.
- Loss of component state.
- Unintended behavior in dynamic lists.
By adhering to best practices for using keys, developers can ensure that their applications remain efficient and responsive.
Understanding the Importance of Key Props in React Iterators
Dr. Emily Carter (Senior Software Engineer, React Innovations). “The missing key prop in iterators can lead to performance issues and unexpected behavior in React applications. Each element in a list should have a unique key to help React identify which items have changed, are added, or are removed, thus optimizing rendering.”
Michael Chen (Frontend Development Specialist, CodeCraft). “Failing to provide a key prop when rendering lists can result in bugs that are difficult to trace. It is essential to ensure that each element has a stable identity, which is crucial for maintaining the integrity of the UI during updates.”
Sarah Thompson (React Consultant, UI/UX Solutions). “In my experience, ignoring the key prop can lead to issues like incorrect component states and performance degradation. Developers should always prioritize assigning unique keys to list items to ensure a smooth user experience.”
Frequently Asked Questions (FAQs)
What does “missing key prop for element in iterator” mean?
This warning indicates that a React component rendering a list of elements lacks a unique “key” prop for each item. The key prop helps React identify which items have changed, are added, or are removed, optimizing rendering performance.
Why is the key prop important in React?
The key prop is crucial for maintaining the identity of elements in a list. It allows React to efficiently update the UI by tracking changes between renders, thereby minimizing unnecessary re-renders and improving performance.
How do I fix the missing key prop warning?
To resolve this warning, ensure that each element in an iterator has a unique key prop. You can use a unique identifier from your data, such as an ID, or the index of the element as a last resort, although using indices can lead to issues in certain scenarios.
What happens if I ignore the missing key prop warning?
Ignoring the warning can lead to performance issues and bugs in your application. Without proper keys, React may not correctly identify changes in the list, leading to incorrect rendering or unexpected behavior.
Can I use the index of the array as a key prop?
While using the index as a key prop is possible, it is not recommended unless the list is static and does not change. Using indices can cause issues with component state and performance when the list is reordered or modified.
Where should I add the key prop in my code?
You should add the key prop directly to the element being rendered within the iterator. For example, in a map function, include the key prop in the returned JSX element, ensuring that it is unique for each item in the list.
The warning about a missing key prop for elements in an iterator is a common issue encountered in React applications. This warning arises when developers render lists of components without providing a unique ‘key’ prop for each element. The ‘key’ prop is essential for helping React identify which items have changed, been added, or removed, thus optimizing the rendering process and improving performance.
When a key prop is omitted, React defaults to using the index of the element in the array, which can lead to problems, especially when the list is modified. Using indices can result in incorrect component state and behavior during re-renders, as React may not accurately track the elements. Therefore, it is crucial to provide a stable and unique identifier for each item in the list, such as an ID from the data being rendered.
In summary, ensuring that each element in an iterator has a unique key prop is vital for maintaining the integrity of the component state and optimizing performance in React applications. Developers should prioritize implementing unique keys to avoid potential issues and enhance the user experience. By adhering to this best practice, developers can create more efficient and reliable React applications.
Author Profile
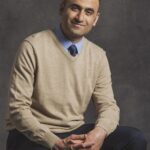
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?