Why Does the Module java.base Not Open java.lang to Unnamed Modules?
In the ever-evolving landscape of Java development, the of the Java Platform Module System (JPMS) in Java 9 brought about a paradigm shift in how developers structure and manage their applications. While this modular system offers numerous benefits, such as improved encapsulation and better dependency management, it also introduces a set of challenges that can leave even seasoned developers scratching their heads. One such challenge is the perplexing error message: “module java.base does not open java.lang to unnamed module.” This cryptic notification can halt progress and provoke confusion, but understanding its implications is essential for navigating the intricacies of Java’s module system.
At its core, this error message highlights the strict access controls enforced by JPMS, which aim to enhance security and maintainability in Java applications. The “java.base” module, which is fundamental to the Java platform, contains essential classes, including the ubiquitous “java.lang” package. However, these classes are not automatically accessible to all modules, particularly unnamed modules—those that do not have a defined module descriptor. This restriction can lead to frustrating roadblocks when developers attempt to access core Java functionality without the appropriate permissions.
As we delve deeper into this topic, we will explore the underlying principles of Java’s module system, the significance of access modifiers,
Understanding the Module System in Java
The Java Platform Module System (JPMS), introduced in Java 9, allows developers to modularize their applications, encapsulating code and controlling access. A significant aspect of this system is the strong encapsulation it enforces, which can lead to issues when existing codebases that rely on reflection or access to internal APIs are migrated to a modular architecture.
When you encounter the error message `module java.base does not open java.lang to unnamed module`, it indicates that the unnamed module, which usually refers to code that is not part of a defined module, is trying to access a package that is not open for reflection. By default, the `java.base` module does not open its internal packages, which includes `java.lang`, to unnamed modules, thus preventing unauthorized access.
Common Causes of the Error
Several scenarios can lead to this error, including:
- Reflection Usage: Code attempting to use reflection to access classes or members in the `java.lang` package.
- Legacy Code: Older libraries or applications that were not designed with the module system in mind.
- Third-party Libraries: Some libraries may attempt to access internal Java APIs without proper module declarations.
How to Resolve the Issue
To resolve the `module java.base does not open java.lang to unnamed module` error, consider the following approaches:
- Modify Command-Line Options: You can open specific packages for reflection by using the `–add-opens` option when launching your application. For example:
“`
–add-opens java.base/java.lang=ALL-UNNAMED
“`
- Update Dependencies: Check if there are newer versions of libraries you are using that are compatible with the module system and do not require access to internal packages.
- Refactor Code: If feasible, refactor your code to avoid reflection or direct access to internal Java APIs.
Using the `–add-opens` Option
The `–add-opens` option is a powerful tool to control access to modules. Here’s how it works:
Option | Description |
---|---|
–add-opens | Allows specified packages in a module to be accessible for reflection by unnamed modules. |
java.base/java.lang=ALL-UNNAMED | Specifies that all unnamed modules can access the `java.lang` package for reflection. |
When using the `–add-opens` option, ensure you are aware of the security implications, as it may expose internal APIs that could lead to unstable code if modified.
Best Practices
To avoid running into module-related issues in the future, consider adhering to these best practices:
- Adopt Module System Early: Transition to JPMS early in your project lifecycle to identify and resolve module-related issues sooner.
- Use Descriptive Module Names: Clearly define module names and dependencies to maintain clarity within your project structure.
- Encapsulate Properly: Ensure that public APIs are clearly defined and encapsulated, reducing the need for reflection.
- Stay Updated: Regularly update your libraries and frameworks to ensure compatibility with the latest Java versions and module system enhancements.
By following these guidelines, you can mitigate the risks associated with the Java module system and enhance the maintainability and security of your applications.
Understanding the Issue
The error message `module java.base does not opens java.lang to unnamed module` typically arises in Java applications that leverage the module system introduced in Java 9. This issue indicates that the `java.base` module does not allow reflection on the `java.lang` package from unnamed modules.
Key Points:
- Java Module System: The module system, known as Project Jigsaw, encapsulates packages within modules, providing strong encapsulation.
- Unnamed Modules: An unnamed module includes classes that are not explicitly part of a defined module. This often happens with classpath-based applications.
- Reflection Restrictions: By default, Java restricts reflective access to the internals of modules to enhance security and maintainability.
Common Scenarios for the Error
The error can occur in various scenarios, including but not limited to:
- Using Libraries: Third-party libraries attempting to use reflection on core Java classes.
- Testing Frameworks: Testing tools that require access to internal APIs of Java classes.
- Legacy Code: Migrating older Java applications that rely heavily on reflection.
Resolving the Issue
Several approaches can be taken to mitigate the `module java.base does not opens java.lang to unnamed module` error.
Options for Resolution:
- Add Command-Line Arguments:
- Use the `–add-opens` option when launching the JVM to allow specific packages to be opened for reflection.
- Example:
“`bash
java –add-opens java.base/java.lang=ALL-UNNAMED -jar yourapplication.jar
“`
- Modifying the Module Descriptor:
- If you have control over the module, update the `module-info.java` file to specify which packages should be opened.
- Example:
“`java
module your.module {
opens java.lang to other.module;
}
“`
- Refactoring Code:
- Where possible, refactor the code to eliminate the need for reflection on internal Java classes. Utilize public APIs instead.
Example of Using `–add-opens`
Consider a scenario where a testing framework needs access to internal Java classes. The following command demonstrates how to use `–add-opens` effectively:
“`bash
java –add-opens java.base/java.lang=ALL-UNNAMED -cp your-test-framework.jar your.package.MainClass
“`
Command Component | Description |
---|---|
`–add-opens` | Specifies the package to be opened for reflection. |
`java.base/java.lang` | The module and package that are being opened. |
`ALL-UNNAMED` | Allows all unnamed modules to access the opened package. |
`-cp` | Sets the classpath for required libraries. |
Best Practices
To maintain compatibility and security when working with the Java module system:
- Limit Use of Reflection: Avoid using reflection unless absolutely necessary.
- Use Public APIs: Prefer public APIs over internal classes whenever possible.
- Stay Updated: Keep your dependencies and the JDK up-to-date to benefit from improvements and fixes related to module handling.
Understanding Java Module System Challenges
Dr. Emily Carter (Java Platform Architect, Tech Innovations Inc.). “The error message ‘module java.base does not opens java.lang to unnamed module’ typically arises due to the encapsulation introduced in Java 9. This encapsulation is designed to enhance security and modularity, but it can lead to challenges when legacy code or libraries attempt to access internal APIs that are no longer accessible without explicit permissions.”
Michael Thompson (Senior Software Engineer, OpenSource Solutions). “To resolve this issue, developers should consider using the `–add-opens` JVM argument to grant access to specific packages. However, this should be approached with caution, as it can undermine the modularity benefits that the Java Platform Module System aims to provide. It’s crucial to assess whether modifying module accessibility is truly necessary for the application.”
Lisa Chen (Java Development Consultant, CodeCraft Consulting). “Understanding the implications of the module system is vital for modern Java development. The ‘unnamed module’ refers to code that does not belong to a named module, which can lead to accessibility issues. Developers should refactor their code to explicitly define modules where possible, thus adhering to best practices in modular programming and avoiding such access issues in the future.”
Frequently Asked Questions (FAQs)
What does the error “module java.base does not opens java.lang to unnamed module” mean?
This error indicates that the unnamed module (typically a non-modularized code or legacy code) is attempting to access internal classes or packages in the `java.base` module, which are not exposed for reflective access.
Why is access to `java.lang` restricted in Java modules?
Java modules were introduced to encapsulate packages and control access, enhancing security and maintainability. The `java.base` module restricts access to its internal packages to prevent unintended interactions and maintain a clean API.
How can I resolve the “module java.base does not opens java.lang to unnamed module” error?
To resolve this error, you can either refactor your code to avoid accessing internal classes or use the `–add-opens` JVM argument to explicitly allow access, such as `–add-opens java.base/java.lang=ALL-UNNAMED`.
What is the impact of using `–add-opens` on application security?
Using `–add-opens` can expose internal APIs to unnamed modules, potentially compromising security by allowing unintended access to sensitive classes. It is advisable to use this option cautiously and only when absolutely necessary.
Are there alternatives to using `–add-opens` for accessing internal classes?
Yes, alternatives include refactoring the code to use public APIs or libraries that provide the necessary functionality without requiring access to internal classes. This approach promotes better design and compatibility with future Java versions.
What are unnamed modules in Java?
Unnamed modules are a default category for classes that are not part of any defined module. They typically include legacy code or applications that do not use the Java Platform Module System (JPMS).
The error message “module java.base does not open java.lang to unnamed module” typically arises in Java applications that utilize the Java Platform Module System (JPMS), introduced in Java 9. This message indicates that the unnamed module, which represents code not part of any named module, is attempting to access internal classes or packages in the `java.base` module, specifically the `java.lang` package. The JPMS enforces strong encapsulation, restricting access to certain packages and classes unless explicitly allowed by the module declaration.
To resolve this issue, developers can take several approaches. One common solution is to refactor the application to use named modules, which allows for more control over package accessibility through the `module-info.java` file. By defining the necessary exports and opens directives, developers can grant access to specific packages for other modules. Alternatively, for quick testing or legacy applications, the `–add-opens` JVM argument can be used to bypass the encapsulation for specific packages, though this should be approached with caution as it undermines the modularity principles.
In summary, understanding the implications of the JPMS and the error message regarding module access is crucial for Java developers. It highlights the importance of adhering to modular design principles and the
Author Profile
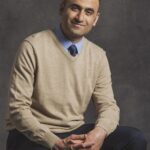
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?