Why Am I Seeing ‘Module Not Found: Error: Can’t Resolve’ and How Can I Fix It?
In the world of software development, encountering errors is as common as breathing. Among the myriad of issues developers face, few are as frustrating as the dreaded “module not found: error: can’t resolve.” This error can halt progress in its tracks, leaving developers scratching their heads and scouring forums for answers. Whether you’re a seasoned programmer or a newcomer to the coding landscape, understanding the nuances of this error is crucial for maintaining a smooth workflow and ensuring that your projects run seamlessly. In this article, we will delve into the causes behind this perplexing error and explore effective strategies for troubleshooting and resolution.
When you see the “module not found” error, it often signifies a breakdown in the connection between your code and the libraries or modules it relies on. This can stem from various issues, such as incorrect file paths, missing dependencies, or even configuration mishaps. As projects grow in complexity, the likelihood of encountering such errors increases, making it essential to grasp the underlying principles that govern module resolution in your development environment.
Navigating through the intricacies of this error can be daunting, but with the right approach, you can turn frustration into understanding. By examining common scenarios that lead to this error and learning how to methodically troubleshoot them, you’ll not only resolve
Understanding the Error Message
The error message “module not found: error: can’t resolve” typically indicates that the system is unable to locate a specific module or dependency that is required for your application to function properly. This can occur in various programming environments, particularly in JavaScript frameworks like Node.js, React, or Angular, where modules are essential for application structure.
Common reasons for this error include:
- Incorrect Module Name: The specified module name may contain typographical errors.
- Missing Installation: The module might not be installed in your project’s node_modules directory.
- Path Issues: The path to the module may be incorrectly defined in your import or require statements.
- Dependency Version Conflicts: Conflicting versions of dependencies can lead to resolution errors.
Resolving the Error
To effectively resolve the “module not found” error, consider the following steps:
- Verify Module Name: Double-check the name of the module you are trying to import. Ensure it matches the exact spelling and casing of the installed package.
- Check Installation: Confirm that the module is installed. You can run the following command in your terminal to check:
“`bash
npm list
“`
- Install Missing Modules: If the module is not listed, install it using npm or yarn:
“`bash
npm install
“`
or
“`bash
yarn add
“`
- Review Import Paths: Ensure that you are using the correct relative or absolute path in your import statements. For example:
“`javascript
import MyModule from ‘./path/to/MyModule’;
“`
- Clear Cache: Sometimes, cached data can lead to module resolution issues. Clear the npm cache with:
“`bash
npm cache clean –force
“`
- Check Configuration Files: Look at your project’s configuration files (like `webpack.config.js` or `tsconfig.json`) to ensure that the module resolution settings are correct.
Common Modules and Their Usage
Here is a table of commonly used modules in JavaScript applications along with their installation commands:
Module Name | Purpose | Installation Command |
---|---|---|
express | Web framework for Node.js | npm install express |
react | Library for building user interfaces | npm install react |
lodash | Utility library for JavaScript | npm install lodash |
axios | Promise-based HTTP client for the browser and Node.js | npm install axios |
Best Practices for Managing Modules
To minimize the occurrence of module resolution errors, implement the following best practices:
- Consistent Package Management: Use a package manager like npm or yarn consistently across your project to manage dependencies.
- Version Control: Maintain a lock file (`package-lock.json` or `yarn.lock`) to ensure consistent installations across environments.
- Documentation: Keep documentation for modules and their required versions up to date, especially when working in a team.
- Regular Updates: Regularly update your dependencies to benefit from bug fixes and improvements.
By following these guidelines, you can significantly reduce the likelihood of encountering “module not found” errors and streamline your development process.
Understanding the “Module Not Found” Error
The “Module Not Found” error typically occurs in programming environments when the system cannot locate a required module or package. This can arise from various issues, which can be categorized as follows:
- Incorrect Module Name: The specified name does not match the installed module.
- Missing Dependencies: The module is dependent on other modules that are not installed.
- File Path Issues: The file path specified is incorrect or the file does not exist.
- Environment Configuration: The project environment is not set up properly, leading to module visibility issues.
Common Causes and Solutions
Identifying the root cause of the error is crucial for resolving it effectively. Below are common causes along with their respective solutions:
Cause | Description | Solution |
---|---|---|
Typos in Module Name | Misspelled module names can lead to this error. | Verify the spelling and correct it. |
Module Not Installed | The module is not present in the project’s environment. | Install the module using package managers like npm or pip. |
Incorrect Import Statement | Using incorrect syntax or path in the import statement. | Check the import syntax and ensure the path is correct. |
Missing Node Modules | Node modules may not be installed or may be corrupted. | Run `npm install` to reinstall the modules. |
Virtual Environment Issues | The virtual environment may not be activated. | Activate the virtual environment before running the code. |
Global vs Local Packages | Confusion between globally and locally installed packages. | Ensure the package is installed in the correct scope. |
Debugging Steps
To effectively troubleshoot the “Module Not Found” error, follow these debugging steps:
- Check Module Installation:
- Use package management commands (e.g., `npm list`, `pip list`) to confirm the module is installed.
- Verify Import Statements:
- Review the code to ensure import statements are correctly formatted and paths are accurate.
- Inspect Environment Configuration:
- Make sure the correct environment is activated, particularly in projects using virtual environments.
- Clear Cache:
- For Node.js, clearing the npm cache might help (`npm cache clean –force`).
- Reinstall Dependencies:
- If corruption is suspected, delete the `node_modules` directory and reinstall dependencies using `npm install`.
Best Practices to Avoid Module Issues
Implementing best practices can significantly reduce the likelihood of encountering module-related errors:
- Consistent Naming Conventions: Use standard naming conventions for modules to avoid typos.
- Documentation: Maintain clear documentation of dependencies and their versions in `package.json` or `requirements.txt`.
- Environment Management: Utilize tools like `venv` for Python or Docker for containerized environments to manage dependencies effectively.
- Regular Updates: Keep packages updated to the latest stable versions to avoid compatibility issues.
Understanding and addressing the “Module Not Found” error involves a systematic approach to identifying the cause and implementing appropriate solutions. By following troubleshooting steps and adhering to best practices, developers can minimize disruptions in their workflow.
Expert Insights on Resolving Module Not Found Errors
Dr. Emily Chen (Senior Software Engineer, Tech Innovations Corp). “The ‘module not found’ error often stems from incorrect paths or missing dependencies. It’s crucial to verify that the module is installed and that your project configuration accurately reflects its location.”
James Patel (Lead Developer, CodeCraft Solutions). “When encountering a ‘can’t resolve’ error, I recommend checking your package.json file for any discrepancies in versioning. Ensuring that all dependencies are properly listed can often resolve these issues quickly.”
Laura Simmons (Technical Consultant, DevOps Insights). “This error can also occur due to caching issues. Clearing your build cache and reinstalling modules can sometimes resolve the ‘module not found’ error, allowing for a fresh start in your development environment.”
Frequently Asked Questions (FAQs)
What does “module not found: error: can’t resolve” mean?
This error indicates that the system is unable to locate a specified module or package in your project. It typically arises when the module is not installed, the path is incorrect, or the module name is misspelled.
How can I resolve the “module not found” error?
To resolve this error, ensure that the module is installed correctly using package managers like npm or yarn. Verify the module’s name and check the import or require paths in your code for accuracy.
What should I check if the module is installed but still shows an error?
If the module is installed but the error persists, check for version compatibility issues, ensure that the module is included in the correct directory, and verify that your build configuration is set up to recognize the module.
Can this error occur due to case sensitivity?
Yes, this error can occur due to case sensitivity, especially in environments like Linux. Ensure that the module name matches exactly, including letter casing, in your import statements.
Is it possible to clear cache to fix this error?
Yes, clearing the cache can sometimes resolve the issue. For npm, use the command `npm cache clean –force`, and for yarn, use `yarn cache clean`. After clearing the cache, reinstall the dependencies.
What tools can help diagnose this error further?
Tools such as Webpack, Babel, or TypeScript can provide detailed error logs and insights. Additionally, using IDEs with built-in linting and error-checking features can help identify the source of the issue more effectively.
The error message “module not found: error: can’t resolve” typically indicates that a specific module or package required by a project cannot be located by the module bundler or runtime environment. This issue often arises in JavaScript environments, particularly when using tools like Webpack, Node.js, or React. The underlying causes can range from incorrect file paths, missing dependencies, to misconfigured module resolution settings. Understanding these factors is crucial for developers to effectively troubleshoot and resolve the issue.
Key takeaways from the discussion include the importance of verifying the existence of the module in question, ensuring that all dependencies are correctly installed, and checking for typos in import statements. Additionally, developers should be aware of the configuration settings in their build tools that dictate how modules are resolved. By systematically addressing these areas, one can often pinpoint the source of the error and implement a suitable fix.
Furthermore, adopting best practices such as maintaining a clear project structure, utilizing version control for dependencies, and regularly updating packages can mitigate the occurrence of such errors in the future. It is also beneficial to consult documentation and community forums for guidance, as many developers have encountered similar issues and can offer valuable insights. Overall, a proactive approach to module management can significantly enhance the development workflow
Author Profile
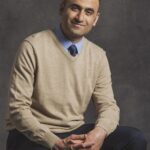
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?