Why Am I Getting ‘Module Not Found: Error: Can’t Resolve ‘./app’?’ and How Can I Fix It?
In the world of software development, encountering errors is an inevitable part of the journey. One particularly perplexing issue that many developers face is the dreaded “module not found: error: can’t resolve ‘./app’.” This error can halt progress and lead to frustration, especially when you’re deep into coding and everything seems to be functioning smoothly—until it isn’t. Understanding the root causes of this error and how to effectively troubleshoot it can save you valuable time and effort, allowing you to focus on building innovative solutions rather than getting bogged down by technical hiccups.
As you dive deeper into the intricacies of module resolution in your projects, you’ll discover that this error often stems from a variety of factors, including incorrect file paths, misconfigured settings, or even issues with the development environment itself. Whether you’re working with a JavaScript framework like React or a backend system using Node.js, the principles behind resolving modules remain critical. Gaining insight into these underlying principles will not only help you address the “module not found” error but also enhance your overall coding proficiency.
In this article, we will explore the common scenarios that lead to this frustrating error and provide you with practical strategies to troubleshoot and resolve it. By the end, you’ll be equipped with the knowledge to tackle this issue head
Understanding the Error Message
The error message `module not found: error: can’t resolve ‘./app’` typically indicates that the module loader, such as Webpack, is unable to locate a specified module or file. This can occur due to several reasons, including incorrect paths, missing files, or misconfigurations in the project setup.
Common causes for this error include:
- Incorrect File Path: The specified path may not accurately reflect the location of the file. Pay attention to capitalization and relative paths.
- Missing File: The file you are trying to import may not exist in the specified directory.
- Configuration Issues: The module resolution settings in your build tool may not be properly configured.
- File Extensions: The file extension may not be specified correctly, or the loader may not be configured to handle that type of file.
Troubleshooting Steps
To effectively troubleshoot this error, follow these systematic steps:
- Check the File Path: Ensure that the path used in the import statement matches the actual file structure.
- Verify File Existence: Confirm that the file you are trying to import is present in the specified directory.
- Inspect Import Statements: Look at your import statements for typos or incorrect syntax.
- Review Project Configuration: Examine your project’s configuration files (e.g., `webpack.config.js`, `tsconfig.json`) to ensure that module resolution settings are correct.
- Rebuild the Project: Sometimes, clearing the cache or rebuilding the project can resolve transient issues.
Common Fixes
In addressing the `module not found` error, consider implementing the following fixes:
- Correct the Import Path: If you find that the import path is incorrect, adjust it accordingly. For example, if your file structure looks like this:
“`
src/
├── components/
│ └── app.js
└── index.js
“`
Your import statement in `index.js` should be:
“`javascript
import App from ‘./components/app’;
“`
- Add Missing Files: If a file is missing, create the necessary file or restore it from version control.
- Modify Configuration: Update your module resolution settings in your configuration file. For instance, ensure that you are using the correct file extensions in Webpack:
“`javascript
resolve: {
extensions: [‘.js’, ‘.jsx’, ‘.ts’, ‘.tsx’]
}
“`
Example of a Proper File Structure
Below is an example of a typical file structure that can help avoid such errors:
Directory | File |
---|---|
src | index.js |
src/components | app.js |
src/styles | styles.css |
By adhering to a clear and consistent directory structure, you can minimize the occurrence of module resolution errors.
Resolving the `module not found: error: can’t resolve ‘./app’` error involves understanding the underlying causes and methodically addressing them through careful examination of file paths, project configurations, and overall file management. By following best practices in project organization and configuration, developers can effectively mitigate such errors in their workflow.
Common Causes of the Error
The error message “module not found: error: can’t resolve ‘./app'” typically arises from various issues in a JavaScript project. Understanding these causes can help diagnose and resolve the problem effectively.
- Incorrect Import Path: The file path specified in the import statement may be incorrect. Ensure that the path accurately reflects the file structure.
- File Name Mismatch: JavaScript is case-sensitive. A discrepancy in the filename or its extension can lead to this error.
- Missing Module: The module might not be installed or included in the project. Verify that all dependencies are correctly installed in the `node_modules` directory.
- Build Issues: Sometimes, the build process might not pick up new files. This often occurs in environments using hot module reloading or when files are added after the server starts.
- Configuration Errors: In some cases, configuration files (like Webpack, Babel, or TypeScript) may not be set up correctly to resolve modules.
Steps to Resolve the Issue
Addressing the “module not found” error involves several troubleshooting steps. Follow these recommendations to identify and fix the underlying problem.
- Check Import Statements:
- Review all import paths in your code.
- Ensure the paths are relative to the file structure.
- Example: Change `import App from ‘./App’` to `import App from ‘./app’` if the file is named `app.js`.
- Verify File Existence:
- Confirm that the file you are trying to import exists in the specified location.
- Use terminal commands such as `ls` (Unix-based) or `dir` (Windows) to list files in the directory.
- Inspect Case Sensitivity:
- Ensure the casing of the filename matches exactly with the import statement.
- For example, `import App from ‘./App’` will not work if the file is named `app.js`.
- Reinstall Dependencies:
- If the module is missing, reinstalling your dependencies may help.
- Run the command:
“`bash
npm install
“`
- or, if using Yarn:
“`bash
yarn install
“`
- Clear Build Cache:
- Build tools may cache files. Clear the cache to force a fresh build.
- For example, with Webpack, you might need to delete the `dist` folder or run a clean command.
- Check Configuration Files:
- Review your configuration files (e.g., Webpack, Babel, TypeScript) for any misconfigurations that could affect module resolution.
- Ensure that aliasing or module resolution paths are correctly set.
Example Scenarios
The following table outlines common scenarios that can trigger the “module not found” error, along with corrective actions:
Scenario | Potential Cause | Corrective Action |
---|---|---|
Incorrect path in import | Typo in the file path | Correct the import statement |
File named differently | Case sensitivity issue | Match the filename’s case |
File not present | Module not installed or missing | Install the module or check file presence |
Cached build artifacts | Build process not recognizing changes | Clear build cache and rebuild |
Misconfigured module resolution | Improper settings in configuration | Update configuration files |
Preventive Measures
To avoid encountering the “module not found” error in the future, consider the following practices:
- Consistent Naming Conventions: Adopt a clear and consistent naming convention for files and directories.
- Automated Testing: Implement unit tests to check for import errors during the development phase.
- Code Reviews: Regularly review code changes with peers to catch potential path issues early.
- Version Control: Use version control (e.g., Git) to track changes and maintain a stable codebase.
By adhering to these practices, developers can significantly reduce the likelihood of running into module resolution errors.
Resolving the ‘Module Not Found’ Error: Expert Insights
Jessica Lin (Senior Software Engineer, CodeCraft Solutions). “The ‘module not found: error: can’t resolve ‘./app” typically indicates that the specified module cannot be located in the expected directory. It is crucial to verify the file path and ensure that the module is correctly exported in the respective file.”
Michael Chen (Lead Developer, Tech Innovations). “This error often arises from incorrect relative paths in import statements. Developers should double-check their directory structure and ensure that the path to the module is accurately defined, taking into account any changes in file locations.”
Sarah Patel (Frontend Architect, Web Solutions Inc.). “In many cases, the ‘module not found’ error can also be resolved by clearing the cache or reinstalling the node modules. A fresh installation can often fix path resolution issues that arise from outdated or corrupted dependencies.”
Frequently Asked Questions (FAQs)
What does the error “module not found: error: can’t resolve ‘./app'” indicate?
This error indicates that the module loader cannot locate the specified module or file named ‘./app’ in your project directory. This typically occurs due to incorrect file paths or missing files.
How can I resolve the “module not found” error?
To resolve this error, verify that the file ‘./app’ exists in the expected directory. Ensure the file path is correctly referenced in your import or require statement. Additionally, check for any typos in the file name or extension.
What should I check if the ‘./app’ file exists but the error persists?
If the ‘./app’ file exists, check the case sensitivity of the file name, as some operating systems are case-sensitive. Also, ensure that the file extension matches what you are trying to import, such as ‘.js’ or ‘.ts’.
Could this error be related to my project configuration?
Yes, this error can stem from misconfigurations in your project settings, such as incorrect module resolution paths in your build tool (e.g., Webpack, Babel). Review your configuration files to ensure they are set up correctly.
Are there any tools that can help diagnose this issue?
Yes, you can use tools like ESLint or TypeScript to identify module resolution issues. Additionally, running your build process with verbose logging may provide further insights into the source of the error.
What should I do if I recently moved or renamed the ‘./app’ file?
If you recently moved or renamed the ‘./app’ file, update all import statements throughout your project to reflect the new path or name. This will ensure that the module loader can find the file correctly.
The error message “module not found: error: can’t resolve ‘./app'” typically indicates that the module loader, such as Webpack or Node.js, is unable to locate the specified module within the project’s directory structure. This issue can arise from a variety of reasons, including incorrect file paths, missing files, or misconfigured module resolution settings. Developers often encounter this error during the build process or when attempting to import a module that does not exist in the expected location.
To effectively troubleshoot this error, it is essential to verify the file path specified in the import statement. Ensuring that the file exists in the correct directory and that the path is accurately referenced can resolve the issue. Additionally, developers should check for typos in the file name or extension, as these can lead to the module not being found. In some cases, the problem may stem from configuration settings in the module bundler, which may require adjustments to the resolve options.
Another key takeaway is the importance of maintaining an organized project structure. A well-structured directory can minimize the likelihood of encountering such errors. Furthermore, utilizing tools like TypeScript or ESLint can help catch these issues early in the development process. By adhering to best practices in coding and project organization, developers
Author Profile
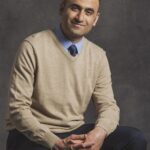
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?