Why Am I Getting a ModuleNotFoundError: No Module Named ‘chardet’?
Have you ever been deep into a Python project, only to be jolted by the frustrating message: `ModuleNotFoundError: No module named ‘chardet’`? This error can halt your progress and leave you scratching your head, wondering what went wrong. For many developers, especially those working with data processing or web scraping, encountering this issue can be a common yet perplexing hurdle. Understanding the root causes and solutions to this error can not only save you time but also enhance your coding experience, allowing you to focus on what truly matters—building and innovating.
In the realm of Python programming, `chardet` is a popular library used for character encoding detection. Its absence can disrupt your workflow, particularly when handling diverse data sources. However, the `ModuleNotFoundError` is more than just a nuisance; it serves as a reminder of the importance of managing dependencies in your projects. Whether you’re a seasoned developer or a newcomer to the Python ecosystem, grappling with this error can provide valuable insights into package management and the intricacies of Python’s import system.
As we delve deeper into the causes of the `ModuleNotFoundError` related to `chardet`, we’ll explore practical solutions and best practices for ensuring your Python environment is
Understanding the Error
The error message `ModuleNotFoundError: No module named ‘chardet’` indicates that the Python interpreter cannot locate the `chardet` library in your current environment. This module is essential for detecting character encoding in text files, often used in web scraping, data processing, and other tasks involving text data.
Common scenarios that trigger this error include:
- The `chardet` module is not installed in the current Python environment.
- A virtual environment is active, but `chardet` is installed in the global Python environment instead.
- Typographical errors in the import statement.
Installation of Chardet
To resolve the `ModuleNotFoundError`, you need to install the `chardet` library. The installation can be performed easily using Python’s package manager, `pip`. Below are the steps for various environments:
- Using pip: Open your terminal or command prompt and run the following command:
“`bash
pip install chardet
“`
- For Python 3 specifically: If you are using Python 3, you may need to specify `pip3`:
“`bash
pip3 install chardet
“`
- Within a virtual environment: If you are working in a virtual environment, ensure it is activated before running the installation command.
Verifying Installation
After installation, you can verify that `chardet` has been installed correctly by executing the following command in a Python shell:
“`python
import chardet
print(chardet.__version__)
“`
If this command executes without any errors and displays the version number, the installation was successful.
Common Issues and Troubleshooting
In some cases, the installation might fail or the error may persist. Here are some common issues and their solutions:
Issue | Solution |
---|---|
Pip not recognized | Ensure Python and pip are added to your system’s PATH variable. |
Permission Denied | Use `sudo` (Linux/Mac) or run the command prompt as an administrator (Windows). |
Conflicting packages | Consider using `pip install –upgrade chardet` to upgrade to the latest version. |
Virtual Environment Issues | Check that you are in the correct virtual environment where `chardet` is installed. |
Alternative Libraries
If for any reason you cannot install `chardet`, consider using the following alternative libraries for character encoding detection:
- `cchardet`: A faster, C++ port of `chardet`.
- `charset-normalizer`: A library that can detect encoding and is more actively maintained.
These libraries can also be installed via `pip` in a similar manner:
“`bash
pip install cchardet
“`
or
“`bash
pip install charset-normalizer
“`
By utilizing these solutions, you can effectively address the `ModuleNotFoundError` for the `chardet` module and continue with your Python projects seamlessly.
Understanding the Error
The error `ModuleNotFoundError: No module named ‘chardet’` typically occurs when Python cannot locate the `chardet` module in your environment. This can happen for several reasons:
- The module is not installed in your current Python environment.
- The environment in which you are running the script is not the same as the one where the module was installed.
- There may be a typographical error in the import statement.
Installing Chardet
To resolve the error, the first step is to ensure that `chardet` is installed. You can install it using the following commands, depending on your environment:
- For pip (Python’s package installer):
“`bash
pip install chardet
“`
- For conda (if you are using Anaconda):
“`bash
conda install -c conda-forge chardet
“`
- If you are using Python 3 and want to ensure you’re installing for the correct version, use:
“`bash
pip3 install chardet
“`
Verifying Installation
After installation, verify that `chardet` is correctly installed in your environment. You can do this by executing the following commands in your Python interpreter:
“`python
import chardet
print(chardet.__version__)
“`
If this runs without errors and outputs the version number, the installation was successful.
Troubleshooting Common Issues
If the error persists after installation, consider the following troubleshooting steps:
- Check Python Environment:
- Ensure that you are using the correct Python interpreter. Use the command:
“`bash
which python
“`
- Compare it with the Python version in which `chardet` was installed.
- Virtual Environment:
- If you are using a virtual environment, make sure it is activated:
“`bash
source /path/to/your/venv/bin/activate
“`
- Reinstalling Chardet:
- Sometimes a clean install can resolve issues:
“`bash
pip uninstall chardet
pip install chardet
“`
- Dependency Conflicts:
- Check for any conflicts with other packages. Use:
“`bash
pip check
“`
Alternative Libraries
If you continue to experience issues with `chardet`, you may consider using alternative libraries for character encoding detection:
Library Name | Description |
---|---|
`cchardet` | A faster alternative to `chardet` that can provide improved performance. |
`charset-sniffer` | Another library that can detect character encodings. |
You can install these libraries using pip:
“`bash
pip install cchardet
“`
or
“`bash
pip install charset-sniffer
“`
By following these steps, you should be able to resolve the `ModuleNotFoundError` related to `chardet` efficiently.
Understanding the Chardet Module Error in Python
Dr. Emily Carter (Senior Software Engineer, Data Science Innovations). “The error ‘ModuleNotFoundError: No module named ‘chardet” typically arises when the Python environment lacks the specified module. Ensuring that the module is installed via pip or conda is crucial for resolving this issue.”
Michael Thompson (Python Developer, Tech Solutions Inc.). “This error often indicates that the chardet library, which is essential for character encoding detection, is either not installed or is installed in a different Python environment. Users should verify their environment and install the module accordingly.”
Lisa Nguyen (Lead Data Engineer, Cloud Analytics Group). “When encountering ‘ModuleNotFoundError: No module named ‘chardet”, it is advisable to check the requirements.txt file of your project. If chardet is missing, adding it and running the installation command can resolve the issue effectively.”
Frequently Asked Questions (FAQs)
What does the error “ModuleNotFoundError: No module named ‘chardet'” indicate?
This error indicates that Python cannot locate the ‘chardet’ module in your environment, which is required for character encoding detection in various libraries.
How can I resolve the “ModuleNotFoundError: No module named ‘chardet'” error?
To resolve this error, you should install the ‘chardet’ module using pip. Run the command `pip install chardet` in your terminal or command prompt.
Is ‘chardet’ a standard library in Python?
No, ‘chardet’ is not a standard library in Python. It is an external library that needs to be installed separately.
What are the common use cases for the ‘chardet’ module?
The ‘chardet’ module is commonly used for detecting the encoding of text files, especially when working with data from various sources that may not specify their encoding.
Can I use an alternative to ‘chardet’ for encoding detection?
Yes, alternatives such as ‘cchardet’ or the built-in ‘codecs’ module can be used for encoding detection, though they may have different performance characteristics and capabilities.
What should I do if ‘chardet’ is already installed but I still see the error?
If ‘chardet’ is installed but the error persists, check your Python environment and ensure you are using the correct interpreter. You may also want to verify the installation by running `pip show chardet` to confirm its presence.
The error message “ModuleNotFoundError: No module named ‘chardet'” indicates that the Python interpreter is unable to locate the ‘chardet’ module, which is commonly used for character encoding detection in text files. This issue typically arises when the module is not installed in the current Python environment or when there are discrepancies in the environment setup. Users may encounter this error while running scripts or applications that depend on ‘chardet’ for processing data, especially in web scraping or data analysis tasks.
To resolve this error, users can take several steps. The most straightforward solution is to install the ‘chardet’ module using package management tools such as pip. Executing the command `pip install chardet` in the terminal will download and install the module, making it available for import in Python scripts. Additionally, users should ensure that they are working within the correct virtual environment, as the module may be installed in a different environment than the one currently active.
In summary, the “ModuleNotFoundError: No module named ‘chardet'” error can be effectively addressed by ensuring the module is installed and verifying the active Python environment. Users should be mindful of their environment management practices and consider using virtual environments to
Author Profile
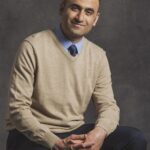
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?