How to Resolve the ‘ModuleNotFoundError: No Module Named ‘Click” in Your Python Projects?
In the world of Python programming, encountering errors is an inevitable part of the development journey. Among these, the `ModuleNotFoundError: No module named ‘click’` stands out as a common yet perplexing issue for many developers. Whether you’re a seasoned coder or a newcomer to the Python ecosystem, this error can disrupt your workflow and leave you scratching your head. As Python’s popularity continues to soar, understanding the nuances of its libraries and modules becomes increasingly essential. This article aims to demystify this particular error, guiding you through its causes and providing you with practical solutions to get back on track.
When you see the `ModuleNotFoundError`, it serves as a reminder that the Python interpreter is unable to locate a specified module—in this case, ‘click’. The ‘click’ library is a popular package used for creating command-line interfaces, and its absence can halt your project in its tracks. This error often arises due to various factors, such as missing installations, virtual environment misconfigurations, or even typos in your import statements. Understanding these underlying causes is crucial for troubleshooting effectively and ensuring a smooth development experience.
As we delve deeper into the topic, we will explore the common scenarios that lead to this error, as well as the steps you
Understanding the Error
The `ModuleNotFoundError: No module named ‘click’` indicates that the Python interpreter cannot find the `click` module in your current environment. This module is often used to create command-line interfaces and is a dependency for various packages. The error typically arises under the following circumstances:
- The module is not installed in your Python environment.
- You are using a virtual environment where the module has not been installed.
- There are issues with your Python path or environment variables.
Checking Your Python Environment
To troubleshoot this issue, you should first confirm that you are working in the correct Python environment. You can do this by checking the active environment and the installed packages. Here’s how you can check:
- Activate your virtual environment (if applicable):
- For Windows:
“`bash
.\venv\Scripts\activate
“`
- For macOS/Linux:
“`bash
source venv/bin/activate
“`
- Check the installed packages:
Run the following command to list the installed packages:
“`bash
pip list
“`
If `click` is not listed, you will need to install it.
Installing the Click Module
If the `click` module is missing, you can easily install it using pip. The installation command is straightforward:
“`bash
pip install click
“`
After running the command, you can verify the installation by checking the list of installed packages again. If the installation completes successfully, you should see `click` in the output.
Common Issues and Solutions
Even after installing, you might encounter issues related to the `click` module. Below is a table summarizing common issues and their solutions:
Issue | Solution |
---|---|
ModuleNotFoundError after installation | Ensure you are in the correct virtual environment and that the installation was successful. |
Multiple Python versions | Use the correct pip version corresponding to your Python interpreter, e.g., `pip3 install click` for Python 3. |
Permission denied on installation | Try using `pip install –user click` to install in the user directory or use a virtual environment. |
Conflicts with other packages | Check for version conflicts and consider upgrading or reinstalling related packages. |
Verifying Installation
To confirm that the `click` module is installed correctly, you can run a simple test script. Create a Python file (e.g., `test_click.py`) and add the following code:
“`python
import click
@click.command()
def hello():
click.echo(‘Hello, World!’)
if __name__ == ‘__main__’:
hello()
“`
Run the script using:
“`bash
python test_click.py
“`
If you see “Hello, World!” printed to the console, the `click` module is functioning correctly. If you still encounter errors, revisit the installation steps or check your environment settings.
Understanding the Error
The error message `ModuleNotFoundError: No module named ‘click’` typically indicates that Python cannot locate the `click` module in your current environment. This issue may arise due to several reasons:
- The `click` module is not installed.
- The module is installed in a different Python environment.
- There is a typo in the import statement.
To resolve this issue, it is essential to first diagnose the underlying cause effectively.
Installation of the Click Module
To install the `click` module, you can use the following methods depending on your environment:
- Using pip:
“`bash
pip install click
“`
- Using pip3 (for Python 3.x):
“`bash
pip3 install click
“`
- Using conda (for Anaconda environments):
“`bash
conda install click
“`
After running the appropriate command, verify the installation using:
“`bash
pip show click
“`
This command should display details about the `click` package if it is installed correctly.
Checking Python Environment
It is crucial to ensure that you are operating in the correct Python environment where `click` is installed. Here are some steps to verify your environment:
- Check the Python version:
“`bash
python –version
“`
- List installed packages:
“`bash
pip list
“`
- Check the active environment (if using virtual environments):
“`bash
which python
“`
This will provide the path to the Python executable, confirming the active environment.
Common Issues and Solutions
Issue | Solution |
---|---|
Module not installed | Install the `click` module using pip or conda. |
Using the wrong Python interpreter | Ensure you are using the correct Python version. |
Using a virtual environment without activation | Activate your virtual environment before running scripts. |
Typo in the import statement | Check for spelling errors in the import line. |
Verifying Installation in Different Environments
If you are using multiple Python environments, you may want to verify the installation in each one. Use the following commands:
- For a virtual environment:
- Activate the environment:
“`bash
source venv/bin/activate On macOS/Linux
.\venv\Scripts\activate On Windows
“`
- Then check for `click`:
“`bash
pip show click
“`
- For system Python:
Simply check using:
“`bash
pip show click
“`
Best Practices for Managing Python Packages
To avoid issues such as `ModuleNotFoundError`, consider the following best practices:
– **Use virtual environments**: Always create isolated environments for different projects to manage dependencies effectively.
– **Regularly update packages**: Run `pip list –outdated` to check for updates.
– **Document dependencies**: Maintain a `requirements.txt` file using:
“`bash
pip freeze > requirements.txt
“`
- Check compatibility: Ensure that the versions of your installed packages are compatible with your Python version.
Implementing these practices will help mitigate errors related to module imports in the future.
Resolving the ‘ModuleNotFoundError’ in Python: Expert Insights
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “The ‘ModuleNotFoundError: no module named ‘click” typically indicates that the Click library is not installed in your Python environment. It is essential to ensure that you are operating within the correct virtual environment and that Click is properly installed using pip.”
James Liu (Software Engineer, Open Source Advocate). “To troubleshoot this error effectively, I recommend checking your Python version and the environment where your script is running. Sometimes, a simple misalignment between the Python interpreter and the installed packages can lead to this issue.”
Sarah Thompson (Lead Python Instructor, Code Academy). “Understanding how to manage Python packages is crucial. If you encounter this error, try running ‘pip install click’ in your terminal. Additionally, using a requirements.txt file can help maintain package consistency across different environments.”
Frequently Asked Questions (FAQs)
What does the error “ModuleNotFoundError: No module named ‘click'” mean?
This error indicates that the Python interpreter cannot find the ‘click’ module in your environment, which is necessary for your script or application to run.
How can I resolve the “ModuleNotFoundError: No module named ‘click'” error?
To resolve this error, you should install the ‘click’ module using pip. Run the command `pip install click` in your terminal or command prompt.
Is ‘click’ a standard library module in Python?
No, ‘click’ is not part of the standard library. It is an external package used for creating command-line interfaces and must be installed separately.
How can I check if ‘click’ is installed in my Python environment?
You can check if ‘click’ is installed by running the command `pip show click` in your terminal. If it is installed, it will display information about the package.
What should I do if I have multiple Python versions installed and still get the error?
Ensure that you are installing ‘click’ in the correct Python environment. You may need to specify the version by using `pip3 install click` or by using a virtual environment.
Can I use ‘click’ in a virtual environment?
Yes, using ‘click’ in a virtual environment is recommended. It allows you to manage dependencies for different projects without conflicts. Create a virtual environment and install ‘click’ within it.
The error message “ModuleNotFoundError: No module named ‘click'” indicates that the Python interpreter is unable to locate the ‘click’ module in the current environment. This issue typically arises when the module has not been installed, or when there is a misconfiguration in the Python environment. The ‘click’ library is a popular package used for creating command-line interfaces, and its absence can hinder the functionality of applications that rely on it.
To resolve this issue, users should first ensure that the ‘click’ module is installed. This can be accomplished using package management tools such as pip. Running the command `pip install click` in the terminal will typically rectify the problem. Additionally, it is important to verify that the installation is being performed in the correct Python environment, especially when using virtual environments or multiple Python installations.
Another important consideration is to check for potential conflicts between different Python versions or environments. Users should confirm that the environment from which they are executing their scripts is the same one where ‘click’ is installed. Utilizing tools such as `pip list` can help in verifying the installed packages within the current environment.
In summary, the “ModuleNotFoundError: No module named ‘click'” error can be
Author Profile
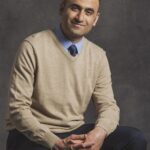
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?