Why Am I Getting ‘ModuleNotFoundError: No Module Named Crypto’ and How Can I Fix It?
In the world of programming, encountering errors is an inevitable part of the journey. Among the myriad of issues that developers face, the `ModuleNotFoundError: No module named ‘crypto’` stands out as a common yet perplexing hurdle, particularly for those venturing into the realms of cryptography and secure communications. This error not only halts progress but can also lead to confusion, especially for newcomers who may not fully grasp the intricacies of Python’s module management. Understanding the root causes of this error and how to resolve it is crucial for anyone looking to harness the power of cryptographic libraries in their projects.
As we delve into the topic, we will explore the significance of the ‘crypto’ module within Python’s ecosystem, shedding light on its role in enabling secure data handling and encryption. We will discuss the common scenarios that lead to the `ModuleNotFoundError`, including misconfigurations, missing installations, and the distinction between various cryptography libraries. By the end of this article, you will not only be equipped with the knowledge to troubleshoot this specific error but also gain insights into best practices for managing Python modules effectively.
Join us as we unravel the complexities surrounding the `ModuleNotFoundError` and empower you to navigate the challenges of incorporating cryptographic
Troubleshooting the ModuleNotFoundError
When encountering the `ModuleNotFoundError: No module named ‘crypto’`, it typically indicates that the Python interpreter cannot find the specified module in its environment. This can happen for several reasons, including installation issues, virtual environment misconfigurations, or naming conflicts.
To resolve this error, follow these steps:
- Check Installation: Ensure that the required module is installed. The `crypto` module is often confused with `pycryptodome` or `pycrypto`. Verify which one you intend to use and install it accordingly.
- Install the Correct Module: Use pip to install the required library. Open your terminal or command prompt and enter:
“`bash
pip install pycryptodome
“`
or
“`bash
pip install pycrypto
“`
Note that `pycryptodome` is a more actively maintained fork of `pycrypto`.
- Verify the Installation: After installation, you can verify whether the module is available by running:
“`python
import Crypto
“`
- Check Python Environment: If you are using a virtual environment, ensure it is activated. If you are working in a Jupyter notebook, verify that the kernel corresponds to the environment where the module is installed.
- Examine Python Path: Sometimes, the module might be installed, but the Python path is misconfigured. You can check the paths where Python looks for modules by:
“`python
import sys
print(sys.path)
“`
- Avoid Naming Conflicts: If you have a file named `crypto.py` in your project directory, it may shadow the actual library. Rename your file to avoid conflicts.
Common Solutions and Workarounds
If the above steps do not resolve the issue, consider the following solutions:
Solution | Description |
---|---|
Upgrade pip | Ensure you are using the latest version of pip: `pip install –upgrade pip` |
Reinstall the module | Sometimes, reinstalling can help: `pip uninstall pycryptodome` followed by `pip install pycryptodome` |
Use a requirements file | If you are working on a project, using a `requirements.txt` file can help maintain consistent installations across environments. Create it using `pip freeze > requirements.txt` and install with `pip install -r requirements.txt`. |
Check for dependency issues | Some modules may depend on others. Review the documentation for any prerequisites that need to be installed. |
By following these steps and utilizing the table of solutions, you can effectively troubleshoot and resolve the `ModuleNotFoundError: No module named ‘crypto’` in your Python environment.
Understanding the Error
The `ModuleNotFoundError: No module named ‘crypto’` typically indicates that Python cannot locate the specified module. This can arise from several factors, including:
- The module is not installed in the current Python environment.
- The module is part of a package that has been incorrectly referenced.
- The Python environment used to run the script is different from the one where the module is installed.
Common Causes
Identifying the root cause of the error is essential. Here are some common reasons:
- Missing Installation: The `crypto` module is not installed. This module is often confused with `pycryptodome`, which provides cryptographic services.
- Virtual Environment Issues: The script may be executed in a different virtual environment than where the module is installed.
- Incorrect Import Statement: The wrong import statement is used in the script, leading to a failure in locating the module.
Installation Steps
To resolve the `ModuleNotFoundError`, follow these installation steps:
- Using pip: Ensure that you have the correct package installed by running:
“`bash
pip install pycryptodome
“`
- Verify Installation: After installation, check if the module is available:
“`bash
pip show pycryptodome
“`
- Python Version: Ensure that you are using the correct version of Python. You can check this using:
“`bash
python –version
“`
Checking Virtual Environments
If you are using virtual environments, ensure you are operating in the correct one:
- Activate the virtual environment:
“`bash
source /path/to/venv/bin/activate On macOS/Linux
.\path\to\venv\Scripts\activate On Windows
“`
- After activation, repeat the installation command if necessary.
Correct Import Statements
Verify that you are using the proper import statement in your code. The following are recommended for using `pycryptodome`:
“`python
from Crypto.Cipher import AES For AES encryption
from Crypto.PublicKey import RSA For RSA keys
“`
Module Alternatives
If you are looking for alternatives to `crypto`, consider the following libraries:
Library | Description |
---|---|
`cryptography` | A robust library for both high-level recipes and low-level interfaces. |
`PyCryptodome` | A self-contained Python package of low-level cryptographic primitives. |
`hashlib` | A built-in Python library for secure hash functions. |
Using these libraries may provide additional functionalities or simplify your cryptographic operations.
To resolve the `ModuleNotFoundError: No module named ‘crypto’`, ensure correct installation, verify your virtual environment, and use the appropriate import statements. By following these guidelines, you can effectively troubleshoot and overcome this common error in Python development.
Expert Insights on Resolving ModuleNotFoundError: No Module Named Crypto
Dr. Emily Chen (Lead Software Engineer, CyberSecure Solutions). “The error ‘ModuleNotFoundError: No module named crypto’ typically indicates that the required library is not installed in your Python environment. It is crucial to ensure that you have the correct package installed, which is often ‘pycryptodome’ or ‘cryptography’, depending on your needs.”
James Patel (Python Developer, Tech Innovators Inc.). “When encountering this error, I recommend checking your virtual environment. Sometimes, the module may be installed globally but not available in your active environment. Utilizing pip to install the necessary packages within the right context is essential.”
Sarah Thompson (Data Security Analyst, SecureTech Labs). “In addition to installation issues, this error can arise from naming conflicts with other modules. It is advisable to review your import statements and ensure that there are no conflicting names that could lead to this confusion.”
Frequently Asked Questions (FAQs)
What does the error “ModuleNotFoundError: No module named ‘crypto'” mean?
This error indicates that Python cannot find the ‘crypto’ module, which is typically required for cryptographic operations. This may occur if the module is not installed or if there is a typo in the import statement.
How can I resolve the “ModuleNotFoundError: No module named ‘crypto'” error?
To resolve this error, you should install the ‘pycryptodome’ library, which provides the ‘Crypto’ module. You can do this by running the command `pip install pycryptodome` in your terminal or command prompt.
Is ‘crypto’ the correct name of the module I should import?
No, the correct module name to import is ‘Crypto’ (with an uppercase ‘C’). Ensure your import statement reads `from Crypto import …` instead of `from crypto import …`.
What are the common libraries that provide cryptographic functionalities in Python?
Common libraries include ‘pycryptodome’, ‘cryptography’, and ‘hashlib’. Each library has its own features and use cases, so choose based on your specific requirements.
Can I use ‘pycrypto’ instead of ‘pycryptodome’?
While ‘pycrypto’ was a popular library for cryptography in Python, it is no longer actively maintained. It is advisable to use ‘pycryptodome’ as it is a drop-in replacement and offers better security and support.
What should I do if I have installed ‘pycryptodome’ but still encounter the error?
If you have installed ‘pycryptodome’ and still see the error, check your Python environment. Ensure you are using the correct interpreter and that the installation was successful. You can verify the installation by running `pip show pycryptodome`.
The error message “ModuleNotFoundError: No module named ‘crypto'” typically indicates that the Python interpreter is unable to locate the specified module. This issue often arises when the module has not been installed in the current Python environment or when there is a typographical error in the import statement. It is essential to ensure that the correct module name is used, as there are multiple libraries related to cryptography, such as ‘pycryptodome’ and ‘cryptography’, which may lead to confusion.
To resolve this error, users should first verify that the required module is installed. This can be accomplished using package management tools like pip. For instance, running the command `pip install pycryptodome` or `pip install cryptography` in the terminal can effectively install the necessary library. Additionally, users should check their Python environment and ensure that they are working within the correct virtual environment, as modules installed in one environment may not be accessible in another.
encountering a “ModuleNotFoundError” related to ‘crypto’ is a common issue that can be easily addressed by confirming the installation of the appropriate cryptographic library and ensuring the correct environment is being utilized. By following these steps, users can effectively eliminate this error and
Author Profile
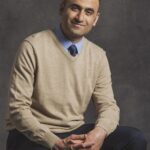
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?