Why Am I Getting a ModuleNotFoundError: No Module Named ‘dotenv’?
In the world of Python programming, encountering errors is an inevitable part of the development process. One common hurdle that many developers face is the dreaded `ModuleNotFoundError: No module named ‘dotenv’`. This error can be particularly frustrating, especially when you’re trying to manage environment variables for your applications. Whether you’re a seasoned developer or a newcomer to the Python ecosystem, understanding how to troubleshoot and resolve this issue is crucial for maintaining efficient workflows and ensuring that your applications run smoothly.
The `dotenv` module is a popular tool used for loading environment variables from a `.env` file, which helps keep sensitive information like API keys and database credentials secure. However, when Python cannot locate this module, it can halt your progress and lead to confusion. This article will guide you through the reasons behind this error, common scenarios where it occurs, and the steps you can take to fix it. By the end, you’ll be equipped with the knowledge to tackle this issue head-on, allowing you to focus on building robust applications without the hassle of unresolved dependencies.
As we delve into the intricacies of the `ModuleNotFoundError`, we will explore various factors that contribute to this error, including installation problems, virtual environment configurations, and even potential typos in your code. With practical solutions and
Understanding the Error
The error message “ModuleNotFoundError: No module named ‘dotenv'” typically indicates that the Python interpreter cannot locate the `dotenv` module, which is often used to manage environment variables in a project. This can occur for several reasons, including the module not being installed, being installed in a different Python environment, or the script being executed in an environment where the module is not accessible.
Common causes of this error include:
- The `python-dotenv` package is not installed.
- The package is installed in a different Python environment (e.g., system Python vs. virtual environment).
- The Python interpreter is not correctly configured to recognize the package.
Installing the `python-dotenv` Package
To resolve the “ModuleNotFoundError,” you must ensure that the `python-dotenv` package is installed in your current Python environment. You can do this using the following command:
“`bash
pip install python-dotenv
“`
If you are using a specific Python version, you may want to specify the version in the command. For example:
“`bash
pip3 install python-dotenv for Python 3
“`
In case you are using a virtual environment, ensure that it is activated before running the installation command. You can activate the virtual environment with:
“`bash
On Windows
.\venv\Scripts\activate
On macOS and Linux
source venv/bin/activate
“`
Verifying Installation
After installation, you can verify that the `dotenv` module is correctly installed by running the following commands in your Python interpreter:
“`python
import dotenv
print(dotenv.__version__)
“`
If the module imports successfully and the version prints, the installation was successful. If the error persists, you may need to check your Python environment configuration.
Common Python Environments
Understanding the environment you are working in is crucial for resolving module-related issues. Below is a table summarizing different Python environments and their characteristics:
Environment | Description | Usage |
---|---|---|
System Python | The default Python installation on your system. | General use, but may require administrative rights to install packages. |
Virtual Environment | An isolated Python environment that can have its own dependencies. | Best for project-specific installations, avoiding conflicts. |
Conda Environment | An environment managed by the Anaconda package manager. | Useful for data science projects, allows for easy package management. |
Ensuring Correct Python Interpreter
If you are still encountering the “ModuleNotFoundError,” it may be due to the Python interpreter used to run your script. You can check which Python interpreter is being used by executing:
“`bash
which python
or for Windows
where python
“`
Make sure this matches the interpreter where `python-dotenv` is installed. If not, you can specify the correct interpreter when running your script:
“`bash
/path/to/correct/python your_script.py
“`
By following these steps, you can effectively troubleshoot and resolve the “ModuleNotFoundError: No module named ‘dotenv'” issue in your Python projects.
Understanding the Error
The error message `ModuleNotFoundError: No module named ‘dotenv’` indicates that Python cannot locate the `dotenv` module in your current environment. This module is typically part of the `python-dotenv` package, which is used to read key-value pairs from a `.env` file and set them as environment variables.
Common reasons for encountering this error include:
- The `python-dotenv` package is not installed.
- The virtual environment is not activated.
- The package is installed in a different Python environment.
Installing the `python-dotenv` Package
To resolve this error, ensure the `python-dotenv` package is installed in your Python environment. You can install it via pip with the following command:
“`bash
pip install python-dotenv
“`
For users who are utilizing a virtual environment, make sure that the environment is activated before running the installation command:
“`bash
For Windows
.\venv\Scripts\activate
For macOS/Linux
source venv/bin/activate
“`
After activating your virtual environment, run the installation command again.
Verifying Installation
To confirm that `python-dotenv` is installed correctly, you can use the following Python commands:
“`python
import pkg_resources
installed_packages = pkg_resources.working_set
installed_packages_list = sorted([“%s==%s” % (i.key, i.version) for i in installed_packages])
print(installed_packages_list)
“`
Look for `python-dotenv` in the printed list. If it is not present, the installation was unsuccessful.
Common Issues and Troubleshooting
If you continue to encounter the `ModuleNotFoundError` after installation, consider the following troubleshooting steps:
- Check Python Version: Ensure that the pip used for installation corresponds to the Python version being executed. Use `python –version` and `pip –version` to check.
- Reinstall the Package: There may have been an issue during installation. Try reinstalling it:
“`bash
pip uninstall python-dotenv
pip install python-dotenv
“`
- Check for Typos: Verify that the import statement in your Python code is correctly spelled:
“`python
from dotenv import load_dotenv
“`
- Virtual Environment Conflicts: If you have multiple virtual environments, ensure you are in the correct one where `python-dotenv` is installed.
Using `dotenv` in Your Code
Once the package is installed successfully, you can use it in your project. Here’s a simple example of how to load environment variables from a `.env` file:
- Create a `.env` file in your project directory with the following content:
“`
API_KEY=your_api_key_here
DEBUG=True
“`
- In your Python script, use the following code:
“`python
import os
from dotenv import load_dotenv
load_dotenv() Load environment variables from .env file
api_key = os.getenv(‘API_KEY’)
debug_mode = os.getenv(‘DEBUG’)
“`
This snippet loads the variables defined in your `.env` file and makes them accessible via `os.getenv()`.
Addressing the `ModuleNotFoundError: No module named ‘dotenv’` involves verifying installation, ensuring the correct environment is activated, and properly using the module in your code. Following the outlined steps will help resolve the issue efficiently.
Resolving the ModuleNotFoundError for Dotenv in Python
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “The error ‘ModuleNotFoundError: No module named dotenv’ typically indicates that the Python environment does not have the ‘python-dotenv’ package installed. To resolve this, ensure that you install the package using pip with the command ‘pip install python-dotenv’ in your terminal.”
Michael Chen (Software Engineer, Code Solutions LLC). “In many cases, users encounter this error due to using a virtual environment that hasn’t been activated. It is crucial to activate your virtual environment before running your Python scripts to ensure all dependencies are correctly recognized.”
Sarah Johnson (DevOps Specialist, CloudOps Group). “If you are still facing issues after installing the package, check your Python path and ensure that the interpreter you are using is the same one where ‘python-dotenv’ was installed. Misconfigurations in the environment can often lead to this error.”
Frequently Asked Questions (FAQs)
What does the error “ModuleNotFoundError: No module named ‘dotenv'” mean?
This error indicates that the Python interpreter cannot find the ‘dotenv’ module, which is typically used for loading environment variables from a `.env` file.
How can I resolve the “ModuleNotFoundError: No module named ‘dotenv'” error?
To resolve this error, you need to install the `python-dotenv` package using pip. You can do this by running the command `pip install python-dotenv` in your terminal or command prompt.
Is the ‘dotenv’ module included in the standard Python library?
No, the ‘dotenv’ module is not part of the standard Python library. It must be installed separately using a package manager like pip.
Can I use ‘dotenv’ in a virtual environment?
Yes, you can use ‘dotenv’ in a virtual environment. Ensure that you activate your virtual environment before installing the `python-dotenv` package.
What should I do if I have installed ‘dotenv’ but still see the error?
If you have installed ‘dotenv’ but still encounter the error, verify that you are using the correct Python interpreter and that the package is installed in the environment you are currently using.
Are there alternatives to using ‘dotenv’ for managing environment variables in Python?
Yes, alternatives include using the built-in `os` module to access environment variables or using other libraries like `environs` or `configparser` for configuration management.
The error message “ModuleNotFoundError: No module named ‘dotenv'” indicates that the Python interpreter cannot locate the ‘dotenv’ module, which is commonly used to manage environment variables in applications. This issue typically arises when the ‘python-dotenv’ package is not installed in the current Python environment. To resolve this, users can install the package using pip, which is the standard package manager for Python. The command `pip install python-dotenv` can be executed in the terminal or command prompt to successfully install the module.
Another common reason for encountering this error is that the Python interpreter being used does not match the environment where the module is installed. It is essential to verify that the correct Python environment is activated, especially when using virtual environments or multiple Python installations. Users should ensure they are operating within the intended environment where ‘dotenv’ is installed to avoid this error.
Additionally, it is advisable to check for any typos in the import statement. The correct way to import the ‘dotenv’ module is by using `from dotenv import load_dotenv`. Any discrepancies in the module name or import syntax can lead to the ModuleNotFoundError. Ensuring that the code is correctly written and that the module is properly installed will help in
Author Profile
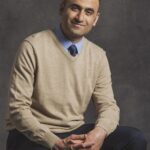
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?