How to Fix the ModuleNotFoundError: No Module Named ‘FastAPI’?
In the ever-evolving landscape of web development, FastAPI has emerged as a powerful tool for building APIs with speed and efficiency. Its popularity stems from its intuitive design, automatic generation of documentation, and seamless integration with modern Python features. However, even seasoned developers can encounter roadblocks when working with new frameworks. One common issue that can halt progress is the dreaded `ModuleNotFoundError: No module named ‘fastapi’`. This error can be frustrating and perplexing, especially when you’re eager to harness the capabilities of FastAPI for your next project.
Understanding the root causes of this error is crucial for anyone looking to streamline their development process. Often, it arises from simple oversights, such as missing installations or incorrect environment configurations. As we delve deeper into this topic, we will explore the common pitfalls that lead to this error, how to troubleshoot them effectively, and best practices to ensure a smooth setup for FastAPI. Whether you’re a beginner or an experienced developer, this guide will equip you with the knowledge to overcome this hurdle and unlock the full potential of FastAPI in your applications.
Join us as we navigate through the intricacies of module management in Python, shedding light on how to resolve the `ModuleNotFoundError` and set the
Understanding the Error
The `ModuleNotFoundError: No module named ‘fastapi’` indicates that Python cannot locate the FastAPI library in your environment. This error typically occurs when FastAPI is not installed or if it is installed in a different Python environment than the one being used to run your application.
There are several common reasons for this error:
- FastAPI is not installed: This is the most straightforward reason. If FastAPI hasn’t been added to your environment, Python will throw this error.
- Virtual environment issues: If you are using a virtual environment, you may not have installed FastAPI within that specific environment.
- Python version mismatch: If your script is being executed with a different Python interpreter than the one where FastAPI is installed, this error will arise.
- Incorrect module name: Ensure that you are importing FastAPI correctly in your code. Module names are case-sensitive in Python.
Installing FastAPI
To resolve the `ModuleNotFoundError`, you need to install FastAPI. Here’s how to do it using pip, the Python package installer.
- Open your terminal or command prompt.
- Activate your virtual environment (if you are using one) by running:
- For Windows:
“`
.\venv\Scripts\activate
“`
- For macOS/Linux:
“`
source venv/bin/activate
“`
- Install FastAPI by executing the following command:
“`bash
pip install fastapi
“`
- Verify installation: You can check if FastAPI is installed by running:
“`bash
pip show fastapi
“`
This command will provide details about the FastAPI installation, including the version and location.
Troubleshooting Common Issues
If you continue to encounter the `ModuleNotFoundError` after installation, consider the following troubleshooting steps:
- Check Python version: Ensure you are using Python 3.6 or above, as FastAPI requires at least this version to function correctly.
- Confirm active environment: Use the following command to confirm which Python interpreter you are using:
“`bash
which python
“`
Or for Windows:
“`bash
where python
“`
- Inspect installed packages: List all installed packages in your environment to check if FastAPI is present:
“`bash
pip list
“`
If FastAPI is not listed, repeat the installation steps.
Common Commands for Managing Python Packages
To further assist you in managing Python packages, the following table summarizes common pip commands:
Command | Description |
---|---|
`pip install |
Installs the specified package. |
`pip uninstall |
Uninstalls the specified package. |
`pip list` | Displays all installed packages. |
`pip show |
Shows detailed information about the specified package. |
`pip freeze` | Outputs installed packages in a format suitable for requirements.txt. |
By following these steps and utilizing the information provided, you should be able to resolve the `ModuleNotFoundError` related to FastAPI and successfully run your application.
Troubleshooting the ModuleNotFoundError
To resolve the `ModuleNotFoundError: No module named ‘fastapi’`, follow these troubleshooting steps:
- Verify Installation: Ensure FastAPI is installed in your Python environment.
- Run the command:
“`bash
pip show fastapi
“`
- If it’s not installed, install it using:
“`bash
pip install fastapi
“`
- Check Python Environment: Confirm that you are in the correct Python environment where FastAPI is installed.
- If using virtual environments, activate it with:
“`bash
source /path/to/venv/bin/activate On macOS/Linux
.\path\to\venv\Scripts\activate On Windows
“`
- Python Version Compatibility: Ensure that your Python version is compatible with FastAPI, typically Python 3.6 or later.
Common Causes of the Error
Understanding the common causes can help in effectively resolving the issue:
- Missing Installation: The FastAPI package may not be installed in the active environment.
- Incorrect Environment: The script may be executed in a different environment than where FastAPI is installed.
- Typographical Errors: Check for spelling mistakes in the import statement. Correct usage is:
“`python
import fastapi
“`
- Conflicts with Other Packages: Sometimes, conflicts with other installed packages can cause issues. Check for any conflicting dependencies.
Using Virtual Environments
Utilizing virtual environments can prevent conflicts between project dependencies. Here’s how to create and use a virtual environment:
- Create a Virtual Environment:
“`bash
python -m venv myenv
“`
- Activate the Virtual Environment:
- For macOS/Linux:
“`bash
source myenv/bin/activate
“`
- For Windows:
“`bash
.\myenv\Scripts\activate
“`
- Install FastAPI within the Environment:
“`bash
pip install fastapi
“`
Checking Installed Packages
You can list all installed packages to verify FastAPI’s presence:
Command | Description |
---|---|
`pip list` | Lists all installed packages |
`pip freeze` | Provides installed packages in requirements format |
Ensure FastAPI appears in the list. If it does not, install it accordingly.
Testing Your Installation
After installation, test if FastAPI is correctly set up by running the following script:
“`python
from fastapi import FastAPI
app = FastAPI()
@app.get(“/”)
def read_root():
return {“Hello”: “World”}
“`
Run the application using:
“`bash
uvicorn your_script_name:app –reload
“`
Check the terminal output for any errors related to FastAPI. If the server starts without errors, your installation is successful.
Expert Insights on Resolving ModuleNotFoundError in FastAPI
Dr. Emily Carter (Software Development Consultant, Tech Innovations). “The ‘ModuleNotFoundError: No module named ‘fastapi” typically indicates that the FastAPI library has not been installed in your Python environment. It is essential to ensure that you are using the correct virtual environment and that FastAPI is installed via pip. Running ‘pip install fastapi’ should resolve the issue.”
Michael Chen (Senior Python Developer, CodeCrafters). “In many cases, this error arises when a user attempts to run a script without activating the virtual environment where FastAPI is installed. Always verify that your environment is activated before executing your application to avoid such import errors.”
Sarah Johnson (Lead Software Engineer, FutureTech Solutions). “Another common cause for this error is the presence of multiple Python installations on the system. It is crucial to check which Python interpreter is being used to run your script and ensure that FastAPI is installed in that specific interpreter’s site-packages.”
Frequently Asked Questions (FAQs)
What does the error “ModuleNotFoundError: No module named ‘fastapi'” mean?
This error indicates that the Python interpreter cannot find the FastAPI module in your environment. This typically occurs when FastAPI is not installed or the environment is not set up correctly.
How can I install FastAPI to resolve this error?
You can install FastAPI using pip by running the command `pip install fastapi` in your terminal or command prompt. Ensure that you are in the correct virtual environment if you are using one.
What should I do if I have already installed FastAPI but still see this error?
Check that you are using the correct Python interpreter and that the FastAPI installation is in the same environment. You can verify the installation by running `pip show fastapi`.
Can I use FastAPI without an ASGI server?
FastAPI requires an ASGI server to run, such as Uvicorn or Daphne. You can install Uvicorn with the command `pip install uvicorn` and then run your FastAPI application with it.
What are the common reasons for encountering this error in a virtual environment?
Common reasons include not activating the virtual environment, installing FastAPI in the global environment instead of the virtual one, or having multiple Python installations that can cause confusion about which one is being used.
Is it possible to resolve this error in a Jupyter Notebook environment?
Yes, ensure that FastAPI is installed in the same environment as your Jupyter Notebook kernel. You can install it directly in the notebook using `!pip install fastapi` if needed.
The error message “ModuleNotFoundError: No module named ‘fastapi'” indicates that the FastAPI library is not installed in the Python environment being used. FastAPI is a modern, fast web framework for building APIs with Python 3.6+ based on standard Python type hints. This error typically arises when developers attempt to import the FastAPI module without having it installed or when working in a virtual environment where FastAPI is not available.
To resolve this issue, users should ensure that FastAPI is installed. This can be accomplished by running the command `pip install fastapi` in the terminal or command prompt. It is also advisable to verify that the installation is occurring in the correct Python environment, especially when using virtual environments or conda environments. If the error persists after installation, checking the Python interpreter settings in the development environment may be necessary to ensure it points to the correct environment where FastAPI is installed.
Another important consideration is the compatibility of FastAPI with other dependencies and the Python version in use. FastAPI requires Python 3.6 or higher, so users should confirm that their Python version meets this requirement. Additionally, keeping the FastAPI library and other related packages updated can help avoid potential conflicts that may lead to
Author Profile
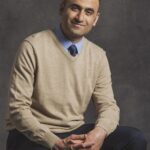
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?