Why Am I Getting a ModuleNotFoundError: No Module Named ‘fcntl’ and How Can I Fix It?
In the ever-evolving world of programming, encountering errors is an inevitable part of the journey. One such common yet perplexing error is the `ModuleNotFoundError: No module named ‘fcntl’`. This error can leave developers scratching their heads, particularly those who are new to Python or transitioning between different operating systems. Understanding the root causes of this error is crucial for troubleshooting and ensuring smooth development processes. In this article, we will delve into the intricacies of the `fcntl` module, the environments in which this error typically arises, and the best practices for resolving it.
The `fcntl` module is a powerful tool in Python, primarily used for file control operations on Unix-like systems. However, its absence in certain environments, particularly on Windows, can lead to the dreaded `ModuleNotFoundError`. This error signifies that the Python interpreter cannot locate the specified module, often due to compatibility issues or incorrect environment configurations. As developers navigate through their projects, recognizing the implications of this error is essential for maintaining productivity and efficiency.
By exploring the reasons behind the `ModuleNotFoundError` related to `fcntl`, developers can better understand how to adapt their code and environments accordingly. Whether you’re a seasoned programmer or just starting out, this article aims to equip you with
Understanding the Error
The error message `ModuleNotFoundError: No module named ‘fcntl’` typically arises when a Python script attempts to import the `fcntl` module, which is not available in the current environment. This module is primarily used for file control operations on Unix-like operating systems, including Linux and macOS. The absence of this module on non-Unix platforms, such as Windows, is a common reason for this error.
When working in a cross-platform environment, developers should be aware of modules that are not universally available. The `fcntl` module is one such example, as it provides functionality that is specific to Unix systems.
Possible Causes
Several factors can lead to the `ModuleNotFoundError` for `fcntl`:
- Platform Compatibility: The `fcntl` module is not implemented on Windows. If you attempt to run a script that imports `fcntl` on this platform, the error will occur.
- Virtual Environment Issues: If you are using a virtual environment, ensure that the environment is set up correctly and that it is not inadvertently restricting access to modules.
- Incorrect Python Version: Sometimes, using an incompatible version of Python could lead to the unavailability of certain modules.
Resolving the Error
To address the `ModuleNotFoundError`, consider the following approaches:
- Check Operating System: Verify that you are running your script on a Unix-like operating system if you intend to use the `fcntl` module.
- Conditional Imports: Implement conditional imports in your code to handle different operating systems.
Operating System | Action |
---|---|
Linux | Proceed with using fcntl |
macOS | Proceed with using fcntl |
Windows | Remove fcntl or use alternatives |
- Use Alternatives: If you are working in a Windows environment and require similar functionality, explore alternative modules or libraries that provide analogous features without relying on `fcntl`. For instance, consider using `os` or `subprocess` for file handling and process management.
Best Practices for Cross-Platform Development
To minimize issues like `ModuleNotFoundError`, adhere to the following best practices when developing cross-platform applications:
- Environment Detection: Use environment detection techniques to check the operating system before importing platform-specific modules.
“`python
import os
if os.name == ‘posix’:
import fcntl
“`
- Documentation: Clearly document dependencies and compatibility requirements for your code. This can aid users in understanding the limitations and necessary configurations.
- Testing: Regularly test your code on multiple platforms to catch compatibility issues early in the development process. Use continuous integration systems to automate this testing.
By following these guidelines, you can reduce the likelihood of encountering the `ModuleNotFoundError` and ensure a smoother development experience across different operating systems.
Understanding the Error
The error `ModuleNotFoundError: No module named ‘fcntl’` typically occurs when attempting to run Python code that imports the `fcntl` module on operating systems that do not support it, particularly Windows. The `fcntl` module is part of the standard library in Unix-like operating systems, allowing for file control operations.
Key Characteristics of the Error:
- Operating System Dependency: The `fcntl` module is not available on Windows, which leads to the error when code that relies on it is executed.
- Module Usage: It is commonly used in scenarios involving file locking, non-blocking I/O, and other low-level operations.
Common Scenarios Leading to the Error
Several development scenarios can trigger this error:
- Cross-Platform Development: Code written for Unix-based systems may include imports for `fcntl` which will fail on Windows.
- Third-Party Libraries: Libraries designed to work across multiple platforms may attempt to use `fcntl`, leading to compatibility issues.
- Misconfigured Environments: An environment set up for Unix-like development but mistakenly run on Windows.
Solutions to Resolve the Error
To address the `ModuleNotFoundError: No module named ‘fcntl’`, several strategies can be employed:
Modify Code for Cross-Platform Compatibility
- Use conditional imports based on the operating system:
“`python
import sys
if sys.platform == ‘win32’:
alternative code for Windows
else:
import fcntl
“`
Use Alternative Libraries
- For file locking and similar functionalities, consider using:
- `portalocker`: A cross-platform file locking library.
- `pywin32`: Provides Windows-specific functionalities.
Check Third-Party Dependencies
- Review the libraries your project uses to ensure they are compatible with your operating system.
- Update or replace any library that relies on `fcntl`.
Best Practices for Avoiding Such Errors
To minimize the risk of encountering the `ModuleNotFoundError` related to `fcntl`, consider the following best practices:
- Environment Testing: Regularly test your code in all target environments.
- Use Virtual Environments: Create isolated environments for each project to manage dependencies effectively.
- Documentation: Maintain clear documentation regarding platform-specific dependencies within your codebase.
Table of Alternatives to fcntl
Functionality | Alternative Library | Description |
---|---|---|
File Locking | `portalocker` | Cross-platform file locking for Python. |
Asynchronous I/O | `asyncio` | Asynchronous programming library for Python. |
Process Management | `subprocess` | A module for spawning new processes. |
By following these guidelines and utilizing alternative libraries where appropriate, developers can effectively manage cross-platform compatibility and avoid the `ModuleNotFoundError: No module named ‘fcntl’`.
Understanding the ‘modulenotfounderror: no module named ‘fcntl” Issue
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “The ‘modulenotfounderror: no module named ‘fcntl” typically arises when attempting to run Python code on a non-Unix platform. The fcntl module is specific to Unix-like operating systems, and its absence on Windows systems can lead to this error. Developers must ensure they are using the correct environment or consider alternative libraries that provide similar functionality.”
James Liu (Software Engineer, Cross-Platform Solutions). “When encountering the ‘modulenotfounderror: no module named ‘fcntl”, it is crucial to assess the operating system in use. For Windows users, this error indicates that the code relies on Unix-specific functionality. In such cases, refactoring the code to eliminate dependencies on fcntl or using conditional imports can be effective strategies.”
Linda Martinez (Technical Consultant, Open Source Projects). “Dealing with the ‘modulenotfounderror: no module named ‘fcntl” error requires a thorough understanding of the project’s dependencies. If the project is intended to be cross-platform, developers should implement platform checks and provide fallbacks for Windows environments, ensuring that the application remains functional regardless of the operating system.”
Frequently Asked Questions (FAQs)
What does the error “modulenotfounderror: no module named ‘fcntl'” indicate?
This error indicates that the Python interpreter cannot find the ‘fcntl’ module, which is typically used for file control operations on Unix-like systems. It is not available on Windows platforms.
On which operating systems is the ‘fcntl’ module available?
The ‘fcntl’ module is available primarily on Unix-based operating systems, including Linux and macOS. It is not supported on Windows.
How can I resolve the “modulenotfounderror: no module named ‘fcntl'” error?
To resolve this error, ensure that your code is running on a compatible Unix-based operating system. If you are on Windows, consider using a virtual machine or a Linux subsystem to run your code.
Are there alternatives to the ‘fcntl’ module for Windows users?
Yes, Windows users can utilize the ‘msvcrt’ module, which provides similar functionality for file control operations, though the specific features may differ.
Is it possible to run code that uses ‘fcntl’ on Windows?
While it is not directly possible to run code that uses ‘fcntl’ on Windows, you can modify the code to use platform-specific modules or run it in a Linux environment using tools like WSL (Windows Subsystem for Linux).
What should I do if my code is meant to be cross-platform but requires ‘fcntl’?
For cross-platform compatibility, consider using conditional imports to check the operating system and implement alternative logic using modules like ‘msvcrt’ for Windows or ‘fcntl’ for Unix-based systems.
The error message “ModuleNotFoundError: No module named ‘fcntl'” typically arises in Python environments when a script attempts to import the `fcntl` module, which is not available on the platform being used. The `fcntl` module is specific to Unix-like operating systems, including Linux and macOS, and is used for file control and locking operations. Consequently, this error is commonly encountered by developers working in Windows environments, as the `fcntl` module does not exist in Windows’ Python installations.
To resolve this issue, developers can explore several workarounds. One approach is to modify the code to avoid using `fcntl` when running on non-Unix systems. This may involve implementing alternative methods for file locking or control that are compatible with Windows, such as using the `msvcrt` module. Additionally, developers can check for cross-platform libraries that abstract these functionalities, allowing for smoother execution across different operating systems.
In summary, understanding the platform-specific nature of Python modules is crucial for effective development. The “ModuleNotFoundError: No module named ‘fcntl'” serves as a reminder for developers to consider the operating system compatibility of their code. By adopting platform-agnostic coding practices or utilizing appropriate libraries, developers can mitigate
Author Profile
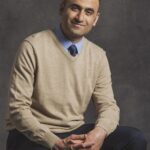
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?