How to Resolve the ‘ModuleNotFoundError: No Module Named ‘google.colab’?’ Issue in Your Python Environment
In the world of data science and machine learning, Google Colab has emerged as a powerful tool, allowing users to write and execute Python code in an interactive environment. However, as with any technology, users may encounter unexpected hurdles along the way. One common issue that many face is the dreaded `ModuleNotFoundError: No module named ‘google.colab’`. This error can be frustrating, especially when you’re in the midst of a project and need to leverage the unique features that Colab offers. Understanding the root causes of this error and how to resolve it is essential for anyone looking to harness the full potential of Google Colab.
The `ModuleNotFoundError` typically arises when the Python environment cannot locate the specified module, in this case, ‘google.colab’. This can happen for various reasons, such as running code outside of the Google Colab environment or issues with the installation of required packages. The error serves as a reminder of the importance of environment management in programming, particularly in collaborative and cloud-based platforms where dependencies can vary significantly.
Navigating this error not only involves troubleshooting but also gaining insights into the structure of Google Colab and its dependencies. By exploring the potential causes and solutions, users can enhance their coding experience and minimize disruptions. In the
Understanding the Error
The `ModuleNotFoundError: No module named ‘google.colab’` typically arises when attempting to run a script that is designed for Google Colab in a different environment, such as a local machine or another cloud platform. This error indicates that the Python interpreter cannot find the specified module, which is specific to Google Colab’s environment.
Key reasons for encountering this error include:
- Running Code Locally: Code snippets that utilize Google Colab libraries will fail if executed in a local Python environment.
- Incorrect Environment: If the script is being executed on a server or in a notebook that does not support Google Colab functionalities.
- Module Not Installed: In some instances, the environment may lack the installation of required libraries, although this is less common with Google Colab modules.
Common Solutions
To resolve the `ModuleNotFoundError`, consider the following solutions based on your specific situation:
- Switch to Google Colab: If your code is heavily reliant on Google Colab features, running the code in a Colab notebook is the most straightforward solution.
- Modify the Code: If you intend to run the code outside of Colab, you may need to refactor it to remove dependencies on Google Colab libraries. This could involve:
- Replacing Colab-specific functions with equivalent functions from other libraries.
- Adjusting the way data is loaded or processed without using Colab’s file management features.
- Use Alternative Libraries: For functionalities provided by Google Colab, you might find alternative libraries that serve similar purposes. Here’s a brief comparison:
Google Colab Feature | Alternative |
---|---|
File Uploads | Use `tkinter` or `Flask` for file uploads in local applications |
GPU Acceleration | Utilize `TensorFlow` or `PyTorch` locally with CUDA installed |
Collaborative Editing | Use GitHub or JupyterHub for collaboration |
- Install Required Packages: If the code can be adapted to run in a different environment, ensure that all necessary packages are installed. Use the following command to install required libraries:
“`bash
pip install
Debugging Tips
If you are still facing issues after trying the above solutions, consider the following debugging tips:
- Check Python Environment: Ensure you are in the correct virtual environment where the necessary libraries are installed.
- Verify Module Names: Double-check that the module name is correctly spelled and formatted.
- Use `pip freeze`: Run this command to list all installed packages and confirm that the required libraries are present.
- Look for Alternative Imports: Sometimes, certain functions can be imported from different libraries, so explore other options if you encounter issues.
By implementing these approaches, you can effectively address the `ModuleNotFoundError` and adapt your code for successful execution in your desired environment.
Understanding the Error
The error `ModuleNotFoundError: No module named ‘google.colab’` typically occurs when a script that is intended to run in the Google Colab environment is executed outside of it, such as in a local Python environment or other IDEs. Google Colab is a cloud-based platform that provides specific libraries and functionalities tailored for machine learning and data analysis, which may not be available in standard Python installations.
Common Scenarios for This Error
This error can arise in several contexts, including:
- Local Environment: Attempting to run a notebook that utilizes Google Colab-specific libraries on your local machine.
- Jupyter Notebook: Running a Jupyter Notebook that includes Google Colab imports without the necessary environment set up.
- Script Execution: Executing a Python script that imports Google Colab libraries without being in the Colab environment.
Resolving the Error
To resolve the `ModuleNotFoundError`, consider the following approaches:
- Use Google Colab: Run your code directly in Google Colab. This is the simplest solution if the code relies on Colab-specific libraries.
- Modify Code: If you need to run your code locally, you can modify it to remove or replace the Google Colab imports. Here are some alternatives:
Colab Functionality | Alternative |
---|---|
`from google.colab import drive` | Use local file paths or `os` module. |
`!pip install` command | Use terminal commands or a requirements file. |
`from google.colab.widgets` | Use standard `ipywidgets` for interactivity. |
- Install Necessary Packages: If you are using functionality that can be replicated using other libraries, install those packages via pip. For example:
“`bash
pip install ipywidgets
“`
Verifying Your Environment
Before running your code, ensure you are in the correct environment. You can check your environment with the following commands:
- Check Python Version:
“`python
import sys
print(sys.version)
“`
- List Installed Packages:
“`python
pip list
“`
If you are in a Jupyter notebook, you can use the following command to check for installed packages:
“`python
!pip list
“`
Best Practices for Cross-Environment Compatibility
To avoid encountering this error in the future, consider adopting the following best practices:
- Environment Detection: Implement checks in your code to detect the environment and conditionally import modules. For instance:
“`python
try:
import google.colab
in_colab = True
except ImportError:
in_colab =
“`
- Abstract Environment-Specific Code: Encapsulate environment-specific code in functions that can be called based on the detected environment.
- Documentation: Clearly document the required environment for your notebooks or scripts to inform users about the dependencies.
By understanding the reasons behind the `ModuleNotFoundError` and implementing the recommended resolutions and practices, you can effectively manage and avoid this common issue when working with Google Colab and other Python environments.
Understanding the ‘ModuleNotFoundError’ in Google Colab
Dr. Emily Carter (Data Scientist, AI Research Institute). “The ‘ModuleNotFoundError: no module named ‘google.colab” typically arises when users attempt to run code outside of the Google Colab environment where the module is inherently available. It is essential to ensure that your code is executed in the correct platform to utilize Colab-specific libraries.”
Michael Chen (Software Engineer, Cloud Computing Solutions). “This error can also indicate that the Colab environment has not been properly initialized. Users should verify that they are using the correct runtime settings and that any necessary packages are installed in their Colab notebook.”
Sarah Thompson (Educational Technologist, Online Learning Hub). “For educators and students using Colab, encountering this error may signal a misunderstanding of the platform’s capabilities. It is crucial to familiarize oneself with the unique features of Colab to avoid such issues and enhance the learning experience.”
Frequently Asked Questions (FAQs)
What does the error “ModuleNotFoundError: No module named ‘google.colab'” mean?
This error indicates that the Python environment you are using does not have the ‘google.colab’ module installed or is not running in Google Colab. This module is specific to the Google Colab environment.
How can I resolve the “ModuleNotFoundError: No module named ‘google.colab'” error?
To resolve this error, ensure that you are running your code in Google Colab. If you are using a local environment, you will not have access to the ‘google.colab’ module as it is exclusive to Google Colab.
Can I use Google Colab features in a local Jupyter Notebook?
No, features specific to Google Colab, such as ‘google.colab’, are not available in local Jupyter Notebooks. You can, however, replicate some functionalities using alternative libraries.
Is there a way to simulate Google Colab functionality locally?
While you cannot directly use ‘google.colab’, you can simulate some functionalities by using libraries such as TensorFlow, PyTorch, or by setting up a cloud environment with similar capabilities.
What should I do if I need to run code that relies on ‘google.colab’ locally?
If your code relies on ‘google.colab’, consider modifying it to remove dependencies on this module or run it in the Google Colab environment to avoid compatibility issues.
Are there alternatives to Google Colab that support similar features?
Yes, alternatives like Kaggle Kernels, Microsoft Azure Notebooks, and IBM Watson Studio offer similar cloud-based environments with support for collaborative coding and access to GPUs.
The error message “ModuleNotFoundError: No module named ‘google.colab'” typically arises when users attempt to run code that is intended for Google Colab in a different environment, such as a local Python installation or another cloud-based platform. This error indicates that the specific module, which is integral to the functionalities provided by Google Colab, is not available in the current Python environment. Google Colab is designed to facilitate the execution of Python code in a cloud-based environment, offering unique features like GPU support and easy access to Google Drive, which are not present in standard Python installations.
To resolve this error, users should ensure they are executing their code within Google Colab itself. If the intention is to run similar code locally, alternatives must be implemented. For instance, one can use libraries such as Jupyter Notebook or set up a local environment that mimics the features of Google Colab. Additionally, users should be mindful of the specific libraries and modules they import, as not all code written for Colab is directly transferable to other platforms without modifications.
the “ModuleNotFoundError: No module named ‘google.colab'” serves as a reminder of the environment-specific dependencies that exist within Python programming. Users should
Author Profile
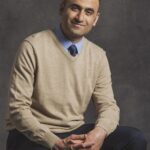
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?