Why Am I Getting ‘ModuleNotFoundError: No Module Named ‘Jinja2′?’ – Troubleshooting Tips
In the world of Python programming, encountering errors is an inevitable part of the development journey. One such common stumbling block is the dreaded `ModuleNotFoundError: No module named ‘jinja2’`. This error can leave developers puzzled, especially when they believe they have set up their environment correctly. Jinja2, a powerful templating engine for Python, is widely used in web development frameworks like Flask and Django. When this module goes missing, it can halt your project in its tracks, leading to frustration and wasted time. But fear not—understanding the root causes and solutions to this error can empower you to get back on track swiftly.
As you delve into the intricacies of this error, you’ll discover that it often arises from issues related to environment configuration, package installation, or even version mismatches. For beginners and seasoned developers alike, recognizing the signs of a missing module is crucial. The good news is that resolving this error typically involves straightforward steps, which can be easily implemented with a little guidance.
In the following sections, we will explore the common scenarios that lead to the `ModuleNotFoundError`, practical troubleshooting techniques, and best practices for managing your Python environments. Whether you’re building a new application or maintaining an existing one, understanding how to
Understanding the Error
The error message `ModuleNotFoundError: No module named ‘jinja2’` indicates that Python cannot find the Jinja2 library in your environment. Jinja2 is a templating engine for Python, widely used for rendering templates in web frameworks like Flask and Django. This error typically arises due to several common issues, including:
- Jinja2 is not installed in your current Python environment.
- The installation of Jinja2 is corrupted or incomplete.
- You are using a virtual environment, but it is not activated.
- There is a mismatch between the Python version and the installed package.
Installation Steps
To resolve the `ModuleNotFoundError`, follow these installation steps based on your environment:
- **Using pip**:
- Open your command line interface (CLI).
- Execute the following command:
“`bash
pip install Jinja2
“`
- **Using a virtual environment**:
- If you are using a virtual environment, ensure it is activated:
“`bash
For Windows
.\venv\Scripts\activate
For macOS/Linux
source venv/bin/activate
“`
- After activation, install Jinja2:
“`bash
pip install Jinja2
“`
- **Using requirements.txt**:
- If your project has a `requirements.txt` file, include Jinja2:
“`
Jinja2>=3.0.0
“`
- Install using:
“`bash
pip install -r requirements.txt
“`
Verifying Installation
Once installed, you can verify that Jinja2 is correctly installed by running the following command in Python’s interactive shell:
“`python
import jinja2
print(jinja2.__version__)
“`
This should display the version of Jinja2 that is installed, confirming successful installation.
Troubleshooting Common Issues
If the error persists, consider the following troubleshooting steps:
- Check Python version: Ensure that Jinja2 is compatible with your version of Python.
- Reinstall Jinja2: Sometimes, a clean installation resolves underlying issues:
“`bash
pip uninstall Jinja2
pip install Jinja2
“`
- Use Python’s package manager: If using a specific Python version, ensure you use the correct pip version:
“`bash
python3 -m pip install Jinja2
“`
Additional Resources
For further assistance, consider the following resources:
Resource | Description |
---|---|
Jinja2 Documentation | Official documentation for Jinja2, detailing usage and features. |
Stack Overflow | A community-driven Q&A platform for troubleshooting and best practices. |
PyPI Jinja2 | The Python Package Index page for Jinja2, for version history and source code. |
By following these guidelines, you should be able to effectively resolve the `ModuleNotFoundError` for Jinja2 and continue your development without disruption.
Understanding the Error
The `ModuleNotFoundError: No module named ‘jinja2’` error indicates that Python cannot locate the Jinja2 module, which is a templating engine used in web development. This error can arise from several situations:
- The Jinja2 library is not installed.
- The installed version of Jinja2 is not accessible in the current Python environment.
- There is a typo in the import statement.
It is essential to confirm the correct installation and accessibility of the Jinja2 module to resolve this issue.
Installing Jinja2
To install Jinja2, you can use pip, which is the package installer for Python. The installation can be done via the command line or terminal. Here are the steps to follow:
- Open your command line interface (CLI).
- Run the following command:
“`bash
pip install Jinja2
“`
For specific Python versions, use `pip3` if necessary:
“`bash
pip3 install Jinja2
“`
Checking Installation
After installation, verify that Jinja2 is correctly installed. You can do this by running the following command:
“`bash
pip show Jinja2
“`
This command will provide you with details such as the version number and the installation location. Ensure that the output reflects the expected installation.
Virtual Environments
If you are working within a virtual environment, ensure that Jinja2 is installed in the correct environment. To create and activate a virtual environment, follow these steps:
- Create a virtual environment:
“`bash
python -m venv myenv
“`
- Activate the virtual environment:
- On Windows:
“`bash
myenv\Scripts\activate
“`
- On macOS/Linux:
“`bash
source myenv/bin/activate
“`
- Install Jinja2 within the virtual environment:
“`bash
pip install Jinja2
“`
Common Troubleshooting Steps
If the error persists after installation, consider the following troubleshooting steps:
- Check Python Version: Ensure you are using the correct version of Python where Jinja2 is installed. Use:
“`bash
python –version
“`
- Inspect Import Statement: Verify that your import statement is correctly written:
“`python
import jinja2
“`
- Check for Typos: Ensure there are no typos in the module name or path.
- Reinstall Jinja2: If issues remain, try uninstalling and reinstalling the module:
“`bash
pip uninstall Jinja2
pip install Jinja2
“`
Using Jinja2 in Your Project
Once Jinja2 is correctly installed and the error is resolved, you can start using it in your project. Below is a simple example of how to render a template:
“`python
from jinja2 import Template
template = Template(“Hello, {{ name }}!”)
rendered = template.render(name=”World”)
print(rendered) Output: Hello, World!
“`
This example demonstrates creating a basic template and rendering it with a variable. Such functionality allows for dynamic content generation, making Jinja2 a powerful tool in web applications.
Understanding the Jinja2 Module Error in Python
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “The ‘ModuleNotFoundError: No module named ‘jinja2” typically arises when the Jinja2 package is not installed in your Python environment. It is essential to ensure that the package is correctly installed using pip, and that you are operating in the correct virtual environment.”
Michael Chen (Software Engineer, Open Source Solutions). “When encountering this error, it is vital to check your Python version and the environment settings. Jinja2 may be installed in a different Python environment than the one you are currently using, leading to this confusion.”
Sarah Patel (DevOps Specialist, CloudTech Corp.). “In addition to ensuring Jinja2 is installed, it is advisable to verify the integrity of your Python installation. Sometimes, conflicts with other packages or a corrupted installation can lead to module errors, including the one with Jinja2.”
Frequently Asked Questions (FAQs)
What does the error “ModuleNotFoundError: No module named ‘jinja2′” indicate?
This error indicates that the Python interpreter cannot find the Jinja2 module in the current environment, suggesting that it may not be installed.
How can I install the Jinja2 module to resolve this error?
You can install the Jinja2 module using pip by running the command `pip install Jinja2` in your terminal or command prompt.
Are there any specific Python versions that require a different installation method for Jinja2?
Jinja2 is compatible with Python 2.7 and 3.5 or later. Ensure you are using a version of pip that corresponds to your Python version.
What should I do if Jinja2 is already installed but I still encounter this error?
If Jinja2 is installed but the error persists, verify that you are using the correct Python environment and check for any virtual environments that may not have Jinja2 installed.
How can I check if Jinja2 is installed in my environment?
You can check if Jinja2 is installed by running the command `pip show Jinja2` in your terminal. This will display the module’s details if it is installed.
What are the potential reasons for the “ModuleNotFoundError” aside from Jinja2 not being installed?
Other reasons may include incorrect Python environment activation, typos in the import statement, or issues with the Python path configuration.
The error message “ModuleNotFoundError: No module named ‘jinja2′” indicates that the Python interpreter is unable to locate the Jinja2 module, which is a popular templating engine used in web development. This issue typically arises when the module is not installed in the current Python environment or when there are discrepancies in the Python path settings. Understanding the context of this error is crucial for developers working with frameworks such as Flask or Django, which often rely on Jinja2 for rendering HTML templates.
To resolve this error, users can take several steps. First, they should ensure that Jinja2 is installed in the active Python environment. This can be done using package management tools like pip, where the command `pip install Jinja2` can be executed. Additionally, developers should verify that they are working within the correct virtual environment, as modules installed in one environment will not be accessible in another. Checking for typos in the import statement is also essential, as a simple misspelling can lead to this error.
In summary, encountering the “ModuleNotFoundError: No module named ‘jinja2′” is a common issue that can be easily rectified by ensuring proper installation and environment management. By following the outlined
Author Profile
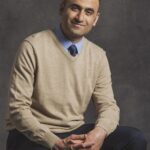
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?