Why Am I Getting a ModuleNotFoundError: No Module Named ‘nltk’ and How Can I Fix It?
In the world of Python programming, few errors are as frustrating as the `ModuleNotFoundError: No module named ‘nltk’`. For those venturing into the realms of natural language processing (NLP), the Natural Language Toolkit (NLTK) is an indispensable library that provides tools for working with human language data. However, encountering this error can halt your progress and leave you scratching your head. Whether you’re a seasoned developer or a curious beginner, understanding the roots of this error and how to resolve it is crucial for harnessing the full potential of NLTK in your projects.
As you dive deeper into the intricacies of Python and its libraries, it’s essential to grasp the foundational aspects of module management. The `ModuleNotFoundError` typically indicates that Python cannot locate the specified module, which in this case is NLTK. This can stem from a variety of issues, including improper installation, virtual environment misconfigurations, or even simple typos in your import statements. Navigating these challenges not only enhances your coding skills but also enriches your understanding of Python’s ecosystem.
In this article, we will explore the common pitfalls that lead to the `ModuleNotFoundError` and provide practical solutions to ensure that NLTK is correctly installed
Understanding the Error
The error `ModuleNotFoundError: No module named ‘nltk’` indicates that Python cannot locate the Natural Language Toolkit (NLTK) module in your current environment. This is a common issue encountered by users who are either new to Python or have not installed the NLTK library correctly.
There are several reasons why this error may occur:
- NLTK Not Installed: The most straightforward cause is that the NLTK library is not installed in your Python environment.
- Virtual Environment Issues: If you are using a virtual environment, NLTK may not be installed in that specific environment.
- Incorrect Python Version: NLTK may be installed, but it might not be accessible due to using a different Python interpreter.
- Corrupted Installation: Occasionally, the installation of NLTK may become corrupted or incomplete.
Installing NLTK
To resolve the `ModuleNotFoundError`, you need to ensure that NLTK is installed properly. The installation can be completed using pip, the Python package manager. Here are the steps to install NLTK:
- Open your command line interface (CLI).
- Enter the following command:
“`
pip install nltk
“`
- Once the installation is complete, you can verify it by running a Python shell and executing:
“`python
import nltk
“`
If no error occurs, the installation was successful.
Working with Virtual Environments
When working in a virtual environment, ensure that you have activated it before installing NLTK. Here’s how to manage virtual environments effectively:
- Creating a Virtual Environment:
“`bash
python -m venv myenv
“`
- Activating the Virtual Environment:
- On Windows:
“`bash
myenv\Scripts\activate
“`
- On macOS/Linux:
“`bash
source myenv/bin/activate
“`
- Installing NLTK in the Activated Environment:
After activation, use the pip command as shown above.
Troubleshooting Installation Issues
If you still encounter the `ModuleNotFoundError` after installation, consider the following troubleshooting tips:
- Check Python Version: Ensure that you are using the correct version of Python where NLTK is installed. You can check the version by executing:
“`bash
python –version
“`
- Reinstall NLTK: If there are issues with the installation, uninstall NLTK and reinstall it:
“`bash
pip uninstall nltk
pip install nltk
“`
- Check PATH Variables: Ensure that your Python and Scripts directories are included in your system’s PATH variable.
Issue | Solution |
---|---|
NLTK Not Installed | Run `pip install nltk` |
Using Wrong Python Interpreter | Check and activate the correct environment |
Corrupted Installation | Uninstall and reinstall NLTK |
By following these guidelines, you should be able to resolve the `ModuleNotFoundError` related to NLTK and successfully utilize the library for your natural language processing tasks.
Understanding the Error
The error `ModuleNotFoundError: No module named ‘nltk’` indicates that the Python interpreter cannot find the Natural Language Toolkit (NLTK) module in your environment. This often occurs due to one of the following reasons:
- The NLTK library is not installed.
- The Python environment you are using does not have NLTK installed.
- There is a typo in the module name when importing.
- The script is being executed in a different environment than where NLTK was installed.
Installing NLTK
To resolve this error, you need to ensure that NLTK is properly installed in your Python environment. Follow these steps:
- Open your command line interface (CLI):
- For Windows: Command Prompt or PowerShell
- For macOS/Linux: Terminal
- Install NLTK using pip. Run the following command:
“`bash
pip install nltk
“`
- Verify the installation by starting a Python shell and importing NLTK:
“`python
import nltk
“`
If there are no errors, the installation was successful.
Using Virtual Environments
If you are working in a project, it is advisable to use a virtual environment to manage dependencies. This prevents conflicts between packages required by different projects. Here’s how to set up a virtual environment:
- Install the `virtualenv` package if you haven’t already:
“`bash
pip install virtualenv
“`
- Create a new virtual environment:
“`bash
virtualenv myenv
“`
- Activate the virtual environment:
- For Windows:
“`bash
myenv\Scripts\activate
“`
- For macOS/Linux:
“`bash
source myenv/bin/activate
“`
- Install NLTK within the activated environment:
“`bash
pip install nltk
“`
- Again, check if the installation was successful:
“`python
import nltk
“`
Common Pitfalls
When dealing with module import errors, consider the following common pitfalls:
- Multiple Python installations: Ensure that you are using the correct Python installation where NLTK is installed.
- Jupyter Notebooks: If you are using Jupyter, ensure that the kernel is associated with the Python environment where NLTK is installed. You can install the kernel with:
“`bash
python -m ipykernel install –user –name=myenv
“`
- Permissions issues: On some systems, you may need administrator privileges to install packages. Use `pip install –user nltk` to install it just for your user account.
Testing Your Installation
After installation, you can run a simple test to ensure that NLTK is functioning correctly. Execute the following code snippet:
“`python
import nltk
nltk.download(‘punkt’)
from nltk.tokenize import word_tokenize
text = “Hello, world! This is a test.”
tokens = word_tokenize(text)
print(tokens)
“`
This code downloads the necessary resources and tokenizes a simple string. If you see the tokenized output, your installation is confirmed to be operational.
Troubleshooting Further Issues
If you continue to experience issues, consider the following troubleshooting steps:
- Reinstall NLTK:
“`bash
pip uninstall nltk
pip install nltk
“`
- Check for Python version compatibility. NLTK requires Python 3.5 or higher.
- Consult the NLTK documentation for additional dependencies or installation instructions.
By following these guidelines, you should be able to resolve the `ModuleNotFoundError` and successfully utilize the NLTK library in your projects.
Resolving the NLTK Module Not Found Error: Expert Insights
Dr. Emily Carter (Data Scientist, AI Solutions Inc.). “The `ModuleNotFoundError: No module named ‘nltk’` typically indicates that the Natural Language Toolkit (NLTK) library is not installed in your Python environment. Users should ensure they have executed `pip install nltk` in the command line to resolve this issue.”
James Liu (Python Developer, CodeCrafters). “In some cases, the error may arise due to the use of virtual environments. It is crucial to activate the appropriate environment where NLTK is installed. Running `pip list` can help verify if NLTK is indeed present in the current environment.”
Sarah Thompson (Software Engineer, Data Insights Corp). “If the error persists even after installation, it may be beneficial to check for multiple Python installations on your system. Using `python -m pip install nltk` can help ensure that you are installing the module in the correct Python version.”
Frequently Asked Questions (FAQs)
What does the error “ModuleNotFoundError: No module named ‘nltk'” indicate?
This error indicates that the Python interpreter cannot locate the Natural Language Toolkit (NLTK) module in your environment, suggesting that it is either not installed or not properly configured.
How can I install the NLTK module to resolve this error?
You can install the NLTK module using pip by running the command `pip install nltk` in your terminal or command prompt. Ensure that you are using the correct Python environment where your project resides.
What should I do if I receive a permission error while installing NLTK?
If you encounter a permission error, try using `pip install –user nltk` to install the module for your user account only. Alternatively, you can run the command with administrative privileges.
How can I verify if NLTK is installed correctly?
You can verify the installation by running `import nltk` in a Python shell or script. If there are no errors, NLTK is installed correctly. You can also check the installed version with `nltk.__version__`.
What if I have multiple Python versions installed and still see the error?
Ensure that you are installing NLTK in the same Python environment where you are running your script. You can specify the Python version explicitly by using `python3 -m pip install nltk` or the corresponding command for your setup.
Can I use NLTK in a virtual environment?
Yes, using NLTK in a virtual environment is recommended to avoid conflicts with other packages. Create a virtual environment using `python -m venv myenv`, activate it, and then install NLTK within that environment.
In summary, the error message “ModuleNotFoundError: No module named ‘nltk'” indicates that the Natural Language Toolkit (NLTK) library is not installed in the Python environment being used. This is a common issue that can arise when attempting to import the library without having it properly set up. Users may encounter this error in various development environments, including local setups, virtual environments, or cloud-based platforms.
To resolve this issue, users should first ensure that they have the correct Python environment activated. Following this, they can install NLTK using package management tools such as pip. The command `pip install nltk` will typically suffice for most users. Additionally, it is important to verify that the installation was successful and that the library is accessible within the intended environment.
Moreover, users should consider the implications of using virtual environments. By creating isolated environments, developers can manage dependencies more effectively and avoid conflicts between different projects. This practice not only helps in resolving module-related errors but also enhances overall project organization and maintainability.
encountering the “ModuleNotFoundError: No module named ‘nltk'” error serves as a reminder of the importance of proper library installation and environment management in Python development.
Author Profile
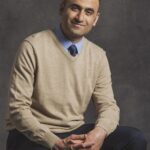
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?