How to Fix ModuleNotFoundError: No Module Named ‘stdemo’?
In the world of programming, encountering errors can be both a frustrating and enlightening experience. One such error that often leaves developers scratching their heads is the infamous `ModuleNotFoundError: No module named ‘stdemo’`. This seemingly cryptic message can halt your coding progress and prompt a flurry of questions: What is ‘stdemo’? Why is it missing? And how can I resolve this issue? Whether you’re a seasoned developer or a novice just starting your coding journey, understanding the nuances of this error is essential for smooth sailing in your programming endeavors.
The `ModuleNotFoundError` is a common hurdle in Python, signaling that the interpreter cannot locate a specified module. This error can arise from various factors, including typos in the module name, incorrect installation paths, or even issues with your environment setup. Specifically, when the error references ‘stdemo’, it indicates that the Python interpreter is unable to find a module that may be crucial to your project. As you delve deeper into troubleshooting this issue, you’ll discover the importance of proper module management and the intricacies of Python’s import system.
In the following sections, we will explore the potential causes of the `ModuleNotFoundError` related to ‘stdemo’ and provide practical solutions to help you overcome
Understanding the Error Message
The error message `ModuleNotFoundError: No module named ‘stdemo’` indicates that Python is unable to locate the specified module named `stdemo`. This issue may arise due to several factors, including module installation, environment configuration, or naming discrepancies. Understanding the underlying causes can facilitate effective troubleshooting.
Common reasons for this error include:
- Module Not Installed: The most frequent cause is that the required module has not been installed in the current Python environment.
- Virtual Environment Issues: If you are using a virtual environment, the module may be installed in a different environment or not installed at all.
- Incorrect Module Name: There might be a typo in the module name, or the module may have been renamed or removed in newer versions.
- Path Issues: The module may not be included in the Python path, preventing the interpreter from locating it.
Troubleshooting Steps
To resolve the `ModuleNotFoundError`, consider the following troubleshooting steps:
- Verify Module Installation:
- Check if the module is installed by executing:
“`bash
pip show stdemo
“`
- If it is not installed, you can install it using:
“`bash
pip install stdemo
“`
- Check Python Environment:
- Ensure you are working in the correct Python environment. Activate your virtual environment if necessary:
“`bash
source venv/bin/activate On macOS/Linux
.\venv\Scripts\activate On Windows
“`
- Inspect the Module Name:
- Double-check the spelling of the module name in your import statement. Ensure it matches exactly with the installed package.
- Review PYTHONPATH:
- If the module is installed but not recognized, ensure that its installation directory is included in the `PYTHONPATH`. You can check this by running:
“`python
import sys
print(sys.path)
“`
- Reinstall the Module:
- If the module was previously installed but is still not found, try uninstalling and reinstalling it:
“`bash
pip uninstall stdemo
pip install stdemo
“`
Common Solutions Table
Issue | Solution |
---|---|
Module Not Installed | Run pip install stdemo |
Wrong Virtual Environment | Activate the correct environment |
Typo in Module Name | Correct the spelling in the import statement |
Module Not in PYTHONPATH | Add module path to PYTHONPATH |
Corrupted Installation | Reinstall the module |
By following these troubleshooting steps and utilizing the solutions outlined, you can effectively resolve the `ModuleNotFoundError` associated with the `stdemo` module.
Understanding the Error
The error message `ModuleNotFoundError: No module named ‘stdemo’` indicates that Python cannot locate the module named `stdemo`. This typically occurs for several reasons, which can be categorized as follows:
- Module Not Installed: The module may not be installed in your Python environment.
- Incorrect Module Name: There may be a typo in the module name.
- Virtual Environment Issues: The module might be installed in a different virtual environment than the one currently active.
- Python Path Issues: The directory containing the module may not be included in the Python path.
Common Causes and Solutions
Cause | Solution |
---|---|
Module Not Installed | Install the module using pip:pip install stdemo |
Incorrect Module Name | Verify the module name for typos. Check documentation for the correct name. |
Virtual Environment Issues | Activate the correct virtual environment where the module is installed:source venv/bin/activate (Linux/Mac) orvenv\Scripts\activate (Windows) |
Python Path Issues | Add the module’s directory to the Python path by including these lines in your script:import sys sys.path.append('/path/to/module') |
Verifying Installation
To ensure that `stdemo` is installed, you can run the following command in your terminal or command prompt:
“`bash
pip show stdemo
“`
This command will provide information about the installed module, including its version and location. If it returns nothing, the module is not installed.
Checking Virtual Environments
If you suspect that the issue is related to virtual environments, check the active environment by running:
“`bash
which python
“`
or
“`bash
where python
“`
This will display the path to the currently active Python interpreter. Ensure that this matches the location of your virtual environment.
Debugging Steps
If the error persists, follow these debugging steps:
- Check Python Version: Ensure that you are using the correct version of Python where `stdemo` is installed.
- Reinstall the Module: Sometimes, reinstalling the module can resolve issues:
“`bash
pip uninstall stdemo
pip install stdemo
“`
- Consult Documentation: Review the documentation for `stdemo` for any specific installation instructions or dependencies.
- Search for Alternatives: If `stdemo` is not maintained or unavailable, consider using alternative modules that provide similar functionality.
Following these guidelines should help resolve the `ModuleNotFoundError` and allow for successful importation of the `stdemo` module in your Python scripts.
Resolving the ‘ModuleNotFoundError’ in Python Development
Dr. Emily Chen (Senior Software Engineer, Tech Innovations Inc.). “The ‘ModuleNotFoundError: No module named ‘stdemo” typically indicates that the Python interpreter cannot locate the specified module. This often occurs due to an incorrect installation or a missing package in your Python environment. Ensuring that the module is installed and accessible in the current environment is crucial for resolving this error.”
Michael Thompson (Python Developer Advocate, CodeCraft). “When encountering the ‘ModuleNotFoundError’, it is essential to verify your Python path and ensure that the module is installed in the right environment. Using virtual environments can help manage dependencies effectively, preventing such errors from disrupting your workflow.”
Sarah Patel (Lead Data Scientist, Analytics Hub). “In many cases, the error may arise from a typo in the module name or an incorrect import statement. Double-checking your code for such discrepancies, along with confirming that the module is properly installed, can save significant debugging time.”
Frequently Asked Questions (FAQs)
What does the error “ModuleNotFoundError: No module named ‘stdemo'” mean?
This error indicates that Python cannot find the module named ‘stdemo’ in the current environment. It suggests that the module is either not installed or not accessible.
How can I resolve the “ModuleNotFoundError: No module named ‘stdemo'” error?
To resolve this error, ensure that the ‘stdemo’ module is installed. You can install it using pip with the command `pip install stdemo`, or verify that you are using the correct virtual environment.
Is ‘stdemo’ a standard Python module?
No, ‘stdemo’ is not a standard Python module. It may be a third-party library or a custom module that needs to be installed separately.
How can I check if ‘stdemo’ is installed in my Python environment?
You can check if ‘stdemo’ is installed by running the command `pip list` in your terminal or command prompt. If it is not listed, it is not installed in your current environment.
What should I do if ‘stdemo’ is installed but I still get the error?
If ‘stdemo’ is installed but you still encounter the error, ensure that your script is running in the same environment where ‘stdemo’ is installed. Additionally, check for any typos in the module name in your import statement.
Can I use a different version of Python to avoid this error?
Using a different version of Python may not resolve the error unless the ‘stdemo’ module is compatible with that version. Ensure that the module is supported in the Python version you are using.
The error message “ModuleNotFoundError: No module named ‘stdemo'” indicates that the Python interpreter is unable to locate the specified module named ‘stdemo’. This issue often arises due to various reasons, such as the module not being installed, incorrect naming, or the module being located in a directory that is not included in the Python path. Understanding the root cause of this error is essential for effective troubleshooting and resolution.
To resolve this error, users should first verify that the module is indeed installed in their Python environment. This can be done using package management tools like pip. If the module is not installed, it can be added by executing the command `pip install stdemo`. Additionally, checking for typos in the module name and ensuring that the script is being executed in the correct environment can help eliminate common sources of this error.
Another important aspect to consider is the Python path. If the module exists but is located in a non-standard directory, users may need to modify the PYTHONPATH environment variable or use relative imports to ensure that Python can locate the module. By following these steps, users can effectively troubleshoot and resolve the “ModuleNotFoundError: No module named ‘stdemo'” error, allowing for smoother execution of
Author Profile
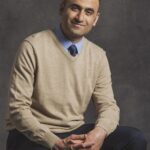
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?