Why Am I Getting ‘ModuleNotFoundError: No Module Named YAML’ and How Can I Fix It?
In the ever-evolving world of programming, encountering errors is an inevitable part of the journey. One such common hurdle that many Python developers face is the dreaded `ModuleNotFoundError: No module named ‘yaml’`. This error can be particularly frustrating, especially when it halts your progress and leaves you scratching your head. Whether you’re a seasoned coder or a curious beginner, understanding how to navigate this issue is essential for smooth development. In this article, we will delve into the reasons behind this error, explore its implications, and provide you with the tools you need to resolve it effectively.
Overview
At its core, the `ModuleNotFoundError: No module named ‘yaml’` signifies that Python is unable to locate the PyYAML library, which is crucial for working with YAML files. YAML, a human-readable data serialization format, is widely used for configuration files and data exchange between languages. When this error arises, it often indicates that the library is either not installed or not accessible in your current Python environment.
Understanding the context of this error is vital for troubleshooting. Factors such as virtual environments, Python versions, and package management systems can all contribute to the issue. By familiarizing yourself with these elements, you can not only resolve the error
Understanding the Error
The error `ModuleNotFoundError: No module named ‘yaml’` indicates that Python cannot find the PyYAML package, which is necessary for parsing YAML files. YAML (YAML Ain’t Markup Language) is widely used for configuration files and data serialization. This error typically arises when the PyYAML module is not installed in the current Python environment.
Common scenarios where this error may occur include:
- Attempting to run a script that imports the `yaml` module without having PyYAML installed.
- Using a virtual environment that does not have the PyYAML package installed, even if it exists in the global Python environment.
- Typos in the import statement, such as incorrect casing or spelling.
Installing PyYAML
To resolve the `ModuleNotFoundError`, you need to install the PyYAML package. This can be accomplished through several methods, depending on your environment.
- Using pip: The most common method is to use pip, Python’s package installer. You can install PyYAML by running the following command in your terminal or command prompt:
“`
pip install pyyaml
“`
- Using conda: If you are using Anaconda or Miniconda, you can install PyYAML using conda:
“`
conda install pyyaml
“`
- Verifying the installation: After installation, verify that PyYAML is correctly installed by running:
“`python
import yaml
print(yaml.__version__)
“`
Troubleshooting Installation Issues
If you encounter issues during installation, consider the following troubleshooting steps:
- Ensure pip is updated: An outdated version of pip can cause installation problems. Update pip with:
“`
pip install –upgrade pip
“`
- Check Python Environment: Ensure you are working in the correct Python environment. To check your active environment, use:
“`
which python On macOS/Linux
where python On Windows
“`
- Virtual Environment: If you are using a virtual environment, make sure it is activated before running the installation commands.
- Permissions: If you face permission issues, you can use `–user` to install PyYAML for the current user:
“`
pip install –user pyyaml
“`
Command | Description |
---|---|
pip install pyyaml | Installs PyYAML using pip. |
conda install pyyaml | Installs PyYAML using conda. |
pip install –upgrade pip | Upgrades pip to the latest version. |
pip install –user pyyaml | Installs PyYAML for the current user to avoid permission issues. |
By following these steps, you should be able to successfully resolve the `ModuleNotFoundError: No module named ‘yaml’` and work with YAML files in your Python projects.
Understanding the Error
The error message `ModuleNotFoundError: No module named ‘yaml’` indicates that Python cannot find the PyYAML library, which is commonly used for parsing YAML files. This situation may arise due to various reasons, including but not limited to:
- The library is not installed in the current Python environment.
- The Python environment being used is different from the one where PyYAML is installed.
- There is a typo in the import statement.
To effectively address this error, it is crucial to determine the underlying cause.
Installing PyYAML
To resolve the error, you need to install the PyYAML module. This can be achieved using the following methods, depending on your environment:
Using pip:
Open your command line or terminal and run:
“`bash
pip install pyyaml
“`
Using conda (if you are using Anaconda):
If you are working within an Anaconda environment, you can install PyYAML by executing:
“`bash
conda install pyyaml
“`
Verifying Installation:
After installation, verify that PyYAML is correctly installed by running:
“`bash
pip show pyyaml
“`
This command will display information about the installed version of PyYAML.
Checking Python Environment
If the error persists after installation, it may be due to using the wrong Python environment. Here are steps to check your environment:
- Verify Python version: Check which version of Python is being used:
“`bash
python –version
“`
- Check pip version: Ensure that pip corresponds to the Python version:
“`bash
pip –version
“`
- List installed packages: To see if PyYAML is installed in the current environment, execute:
“`bash
pip list
“`
If PyYAML is not listed, reinstall it using the commands provided earlier.
Common Mistakes
When working with Python and its packages, certain mistakes can lead to the `ModuleNotFoundError`. Here are some common pitfalls:
- Typographical errors in import statements: Ensure the import statement is correct:
“`python
import yaml
“`
- Using a virtual environment: If you are using a virtual environment, ensure that it is activated before running your script:
“`bash
source venv/bin/activate On macOS/Linux
.\venv\Scripts\activate On Windows
“`
- Multiple Python installations: If you have multiple installations of Python, make sure you are using the one where PyYAML is installed. You can check the path by running:
“`bash
which python On macOS/Linux
where python On Windows
“`
Alternative Solutions
If you continue to face issues, consider the following alternative solutions:
- Reinstall Python: This ensures all packages are correctly configured. Uninstall and reinstall Python, then reinstall PyYAML.
- Using a requirements file: For projects, maintain a `requirements.txt` file that lists all necessary packages, including PyYAML. You can install all packages at once with:
“`bash
pip install -r requirements.txt
“`
- Check for compatibility: Ensure that the version of PyYAML you are trying to install is compatible with your Python version.
By following these steps and guidelines, you should be able to resolve the `ModuleNotFoundError: No module named ‘yaml’` effectively.
Resolving the ModuleNotFoundError for YAML in Python
Dr. Emily Carter (Senior Software Engineer, Data Solutions Inc.). “The error ‘ModuleNotFoundError: No module named yaml’ typically indicates that the PyYAML library is not installed in your Python environment. To resolve this, you can install the library using pip with the command ‘pip install pyyaml’. It is crucial to ensure that you are working in the correct virtual environment if you are using one.”
Michael Thompson (Python Developer and Educator, Code Academy). “When encountering the ‘ModuleNotFoundError: No module named yaml’, it is important to check your Python version and the installed packages. Sometimes, the module might be installed in a different version of Python. Running ‘pip list’ can help you verify if PyYAML is installed in the active environment.”
Sarah Jenkins (Technical Lead, Open Source Projects). “In addition to installing PyYAML, users should also consider potential conflicts with other packages. If you are still facing the error after installation, try creating a new virtual environment and reinstalling the necessary packages to isolate the issue. This can often resolve hidden dependency problems.”
Frequently Asked Questions (FAQs)
What does the error “ModuleNotFoundError: No module named ‘yaml'” mean?
This error indicates that Python cannot find the PyYAML module, which is necessary for working with YAML files in your code.
How can I install the PyYAML module to resolve this error?
You can install PyYAML using pip by running the command `pip install pyyaml` in your terminal or command prompt.
Is PyYAML compatible with all versions of Python?
PyYAML is compatible with Python 2.7 and Python 3.x. However, it is recommended to use the latest version of Python for optimal support and features.
What should I do if I have installed PyYAML but still encounter this error?
Ensure that you are using the correct Python interpreter where PyYAML is installed. You can check the installed packages by running `pip list` in the terminal.
Can I use a virtual environment to manage Python packages like PyYAML?
Yes, using a virtual environment is a best practice for managing dependencies. Create a virtual environment using `python -m venv myenv` and activate it before installing PyYAML.
Are there alternative libraries to PyYAML for working with YAML files?
Yes, alternatives include `rueml` and `oyaml`, which also provide YAML parsing capabilities, although PyYAML is the most widely used and supported.
The error message “ModuleNotFoundError: No module named ‘yaml'” typically indicates that the Python interpreter cannot locate the PyYAML library, which is essential for working with YAML files in Python. This issue often arises when the library is not installed in the current Python environment. Users may encounter this error when attempting to import the ‘yaml’ module in their scripts or applications, highlighting the importance of ensuring that all necessary dependencies are correctly installed before executing code that relies on them.
To resolve this error, users can install the PyYAML library using package managers such as pip. The command `pip install PyYAML` is commonly used to install the library in the active Python environment. It is crucial to verify that the installation is performed in the correct environment, especially when using virtual environments or multiple Python installations. Additionally, checking for typos in the import statement can help avoid unnecessary troubleshooting.
In summary, the “ModuleNotFoundError: No module named ‘yaml'” error serves as a reminder of the importance of dependency management in Python programming. Proper installation and environment configuration are essential steps to ensure that all required libraries are available for use. By following best practices for managing Python packages, developers can minimize the occurrence of such errors and
Author Profile
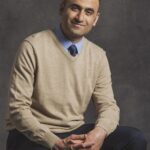
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?