How Can You Use MongoTemplate to Aggregate and Group Multiple Fields Effectively?
In the world of data management and analysis, MongoDB stands out as a powerful NoSQL database that provides flexibility and scalability for modern applications. Among its many features, the aggregation framework is a game-changer, allowing developers to perform complex data transformations and computations. For those working with Spring Data MongoDB, the `MongoTemplate` class offers a convenient way to harness this capability. One of the most powerful aspects of aggregation is the ability to group data by multiple fields, enabling users to derive insights from their datasets in a granular and efficient manner. In this article, we will delve into the intricacies of using `MongoTemplate` to aggregate and group data across multiple fields, unlocking the potential of your MongoDB collections.
Understanding how to effectively group data is essential for anyone looking to perform in-depth analysis or reporting in MongoDB. The aggregation framework allows you to specify multiple fields for grouping, which can lead to richer insights and more nuanced data representations. By leveraging `MongoTemplate`, developers can seamlessly integrate these aggregation operations into their Spring applications, making it easier to work with complex datasets and derive meaningful conclusions. This approach not only enhances the performance of data queries but also simplifies the codebase, allowing for cleaner and more maintainable applications.
As we explore the techniques and best practices for
Understanding MongoTemplate Aggregation
MongoTemplate is a powerful tool within the Spring Data MongoDB framework that allows for complex queries and aggregations on MongoDB collections. One of the key functionalities of MongoTemplate is its ability to perform aggregation operations, which can manipulate and transform data in various ways. This includes grouping data based on multiple fields, enabling developers to analyze datasets more effectively.
Grouping Data by Multiple Fields
When aggregating data, it is often necessary to group documents based on multiple fields. This can be achieved using the `$group` stage in the aggregation pipeline, where you can specify multiple keys to group by. The result will be a set of documents, each representing a unique combination of the specified fields.
To group by multiple fields using MongoTemplate, you typically define a `GroupOperation` and specify the fields you want to include in the grouping. Here is how you can structure the operation:
“`java
Aggregation aggregation = Aggregation.newAggregation(
Aggregation.group(“field1”, “field2”)
.sum(“amount”).as(“totalAmount”)
);
“`
In this example, the documents are grouped by `field1` and `field2`, while simultaneously calculating the sum of the `amount` field for each group.
Example: Grouping and Aggregating Data
Let’s consider a practical example where we have a collection of sales data. Each document contains fields such as `productId`, `region`, and `amount`. To analyze the total sales by product and region, you can use the following aggregation:
“`java
Aggregation aggregation = Aggregation.newAggregation(
Aggregation.group(“productId”, “region”)
.sum(“amount”).as(“totalSales”)
);
“`
This aggregation will yield a result set that combines sales data based on both `productId` and `region`. The resulting documents will look something like this:
productId | region | totalSales |
---|---|---|
101 | North | 5000 |
102 | South | 3000 |
101 | South | 7000 |
103 | North | 2000 |
Advanced Grouping Techniques
In addition to basic grouping, MongoTemplate allows for more advanced aggregation techniques. These can include:
- Adding Multiple Accumulators: You can use multiple accumulators in the same `$group` stage.
“`java
Aggregation.group(“field1”)
.sum(“amount”).as(“totalAmount”)
.avg(“amount”).as(“averageAmount”);
“`
- Using Conditionals: You can apply conditions to your aggregations using the `$cond` operator, enabling more dynamic grouping.
- Sorting Results: After grouping, you may want to sort the results. This can be done with the `$sort` stage following the `$group`.
“`java
Aggregation aggregation = Aggregation.newAggregation(
Aggregation.group(“field1”).sum(“amount”).as(“totalAmount”),
Aggregation.sort(Sort.by(Sort.Order.desc(“totalAmount”)))
);
“`
MongoTemplate provides a robust framework for performing complex aggregations in MongoDB. By leveraging the `$group` stage effectively, developers can extract meaningful insights from their data through multi-field grouping and advanced aggregation techniques. Understanding these concepts enables better data analysis and reporting capabilities within applications utilizing MongoDB.
Using MongoTemplate for Aggregation
MongoTemplate is a powerful tool in Spring Data MongoDB that allows developers to perform complex queries and aggregations. When aggregating data, particularly when grouping by multiple fields, it’s crucial to understand the structure and syntax of the aggregation framework.
Aggregation Framework Basics
The MongoDB aggregation framework processes data records and returns computed results. It operates on the following stages:
- $match: Filters documents to pass only the documents that match the specified condition to the next pipeline stage.
- $group: Groups documents by a specified identifier and performs accumulations on grouped data.
- $sort: Sorts the documents.
- $project: Reshapes each document in the stream, adding new fields or removing existing fields.
Group by Multiple Fields
To group by multiple fields in MongoTemplate, you must define the fields you wish to group on in the `$group` stage. Here’s how you can do that:
“`java
Aggregation aggregation = Aggregation.newAggregation(
Aggregation.group(“field1”, “field2”) // specify multiple fields here
.count().as(“count”) // example of an accumulator
.sum(“someValue”).as(“totalValue”), // summing another field
Aggregation.sort(Sort.by(Sort.Order.desc(“count”))) // optional sorting
);
“`
Example: Grouping Sales Data
Assuming you have a collection of sales records, you can group by `productId` and `region` to get the count of sales per product per region.
“`java
Aggregation aggregation = Aggregation.newAggregation(
Aggregation.group(“productId”, “region”)
.count().as(“totalSales”)
.sum(“amount”).as(“totalRevenue”)
);
“`
Executing the Aggregation
To execute the aggregation operation, use the `aggregate` method of `MongoTemplate`. Here is an example:
“`java
AggregationResults
List
“`
Result Mapping
You will need a result class to map the output of the aggregation. For example:
“`java
public class MyResultType {
private String productId;
private String region;
private int totalSales;
private double totalRevenue;
// getters and setters
}
“`
Handling Complex Aggregations
Complex aggregations may involve additional stages, such as filtering or reshaping data after grouping. You can chain multiple aggregation operations as follows:
“`java
Aggregation aggregation = Aggregation.newAggregation(
Aggregation.match(Criteria.where(“status”).is(“completed”)),
Aggregation.group(“productId”, “region”)
.count().as(“totalSales”)
.sum(“amount”).as(“totalRevenue”),
Aggregation.project(“totalSales”, “totalRevenue”)
.and(“productId”).as(“productId”)
.and(“region”).as(“region”)
);
“`
Tips for Efficient Aggregation
- Indexing: Ensure the fields used in `$match` and `$group` stages are indexed for better performance.
- Pipeline Optimization: Arrange the stages to minimize the number of documents processed in later stages.
- Testing: Use MongoDB’s aggregation pipeline in the shell for testing before implementing it in MongoTemplate.
By leveraging these techniques, you can effectively utilize MongoTemplate to aggregate and group data across multiple fields, providing valuable insights and analytics for your applications.
Expert Insights on Aggregating and Grouping Multiple Fields with MongoTemplate
Dr. Emily Chen (Data Architect, Tech Innovations Inc.). “When using MongoTemplate to aggregate data, it is crucial to understand how to effectively group multiple fields. This allows for more complex queries and insights, enabling developers to harness the full potential of their data models.”
Michael Thompson (Senior Software Engineer, Cloud Solutions Corp.). “Aggregating multiple fields in MongoTemplate not only enhances data retrieval efficiency but also simplifies the analysis process. Properly structuring your aggregation pipeline is key to achieving optimal performance.”
Jessica Patel (Lead Database Consultant, DataWise Analytics). “Incorporating multiple fields in your aggregation queries with MongoTemplate can significantly improve your application’s analytical capabilities. It is essential to leverage the right operators to ensure accurate and meaningful results.”
Frequently Asked Questions (FAQs)
What is the purpose of using MongoTemplate for aggregation?
MongoTemplate is a Spring framework abstraction that simplifies interactions with MongoDB, particularly for complex queries like aggregation. It allows developers to execute aggregation pipelines, making it easier to manipulate and analyze data.
How can I group multiple fields in a MongoTemplate aggregation?
To group multiple fields, use the `group` method in your aggregation pipeline. Specify the fields to group by in a `GroupOperation`, and you can also define accumulators to compute values based on the grouped data.
Can I perform calculations on grouped fields using MongoTemplate?
Yes, you can perform calculations on grouped fields. Use accumulator operators like `$sum`, `$avg`, `$max`, or `$min` within the `group` stage to compute aggregate values based on the grouped fields.
What is the syntax for grouping multiple fields in a MongoTemplate aggregation?
The syntax involves creating a `GroupOperation` with the fields specified in the `group` method. For example:
“`java
Aggregation aggregation = Aggregation.newAggregation(
Aggregation.group(“field1”, “field2”)
.sum(“field3”).as(“totalField3”)
);
“`
How do I handle null values when grouping in MongoTemplate?
To handle null values, you can use the `$ifNull` operator within your aggregation pipeline. This allows you to specify a default value for null fields, ensuring that they are included in the results without causing errors.
Is it possible to sort the results after grouping in a MongoTemplate aggregation?
Yes, you can sort the results after grouping by adding a `sort` stage to your aggregation pipeline. Use the `sort` method to specify the fields and the order (ascending or descending) for sorting the aggregated results.
The use of MongoTemplate for aggregation operations in MongoDB allows developers to efficiently group multiple fields within a collection. This capability is essential for performing complex queries and data transformations, enabling users to derive meaningful insights from their datasets. By utilizing the aggregation framework, developers can combine various stages such as `$match`, `$group`, and `$project` to filter, group, and reshape the data as needed.
Grouping multiple fields in MongoTemplate requires a clear understanding of the aggregation pipeline. Each stage of the pipeline serves a specific purpose, and the `$group` stage is particularly crucial for consolidating documents based on specified criteria. By defining the fields to group by and the aggregation operations to perform, developers can generate summaries, counts, averages, and other statistical measures that are vital for data analysis.
Moreover, leveraging MongoTemplate’s fluent API enhances code readability and maintainability. This approach not only simplifies the construction of complex aggregation queries but also aligns with best practices in modern application development. As a result, developers can create robust data processing workflows that are both efficient and scalable, ultimately leading to better performance in data-driven applications.
Author Profile
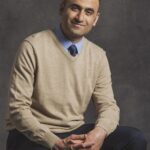
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?