How Can I Effectively Search Text Using Stored Procedures in MS SQL?
In the world of data management, the ability to efficiently search and retrieve information is paramount, especially when dealing with large volumes of text. Microsoft SQL Server, a powerful relational database management system, offers a variety of tools and techniques to enhance text searching capabilities. Among these, stored procedures stand out as a robust solution for encapsulating complex queries and operations, allowing developers to streamline their database interactions. This article delves into the intricacies of using stored procedures for text searching in MS SQL, providing you with the knowledge to optimize your data retrieval processes.
When it comes to searching for text within SQL Server, the challenge often lies in balancing performance with accuracy. Stored procedures can be crafted to implement full-text search features, enabling users to perform sophisticated queries that go beyond simple keyword matching. By leveraging these procedures, developers can create reusable code that not only simplifies the search process but also enhances the overall efficiency of database operations. Understanding how to design and utilize these stored procedures effectively can significantly improve your application’s responsiveness and user experience.
Moreover, the integration of full-text indexes with stored procedures opens up a world of possibilities for advanced text searching. This combination allows for more nuanced queries, such as searching for phrases, proximity searches, and even ranking results based on relevance. As we explore the various strategies and
Searching Text in MS SQL Server
In Microsoft SQL Server, searching for text within stored procedures can be achieved using a combination of system views and functions. The `sys.sql_modules` view contains the definitions of the SQL objects, including stored procedures, while the `sys.objects` view provides metadata about the objects. By querying these views, you can find specific text within stored procedures.
To search for a keyword or phrase within stored procedures, you can use the following SQL query:
“`sql
SELECT
o.name AS ProcedureName,
m.definition AS ProcedureDefinition
FROM
sys.sql_modules m
JOIN
sys.objects o ON m.object_id = o.object_id
WHERE
o.type = ‘P’ AND
m.definition LIKE ‘%your_keyword%’
“`
In this query, replace `your_keyword` with the text you are searching for. The result will give you the names and definitions of the stored procedures containing the specified keyword.
Understanding the Components
When searching through stored procedures, it’s essential to understand the components involved:
- sys.sql_modules: This system view contains the definitions for all SQL objects that have definitions stored in them.
- sys.objects: This view provides a list of all objects in the database, including tables, views, and stored procedures.
The combination of these views allows for effective searching across various SQL objects.
Example Query Explained
The SQL query provided above does the following:
- Selects the name of the procedure and its definition.
- Joins `sys.sql_modules` with `sys.objects` to ensure that only stored procedures are retrieved.
- Filters results to only include entries where the definition contains the specified keyword.
This approach is efficient for identifying stored procedures that include specific text, which can be particularly useful for code reviews or debugging.
Performance Considerations
When executing text searches, keep in mind the following performance considerations:
- Database Size: Large databases with many stored procedures may take longer to search through.
- Keyword Specificity: Using more specific keywords can help reduce the search space and improve performance.
- Execution Plan: Monitor the execution plan for the query to ensure it is optimized.
Sample Results Table
Here is an example of what the results might look like after executing the search query:
ProcedureName | ProcedureDefinition |
---|---|
usp_GetCustomerData | CREATE PROCEDURE usp_GetCustomerData AS … |
usp_UpdateCustomerInfo | CREATE PROCEDURE usp_UpdateCustomerInfo AS … |
This table illustrates how the results could be structured, providing a clear view of which stored procedures contain the specified text.
By effectively using these queries, you can streamline your development process and enhance your ability to manage and maintain SQL Server stored procedures.
Understanding Full-Text Search in MS SQL
Full-text search in Microsoft SQL Server allows for sophisticated querying of text data stored in tables. Utilizing full-text indexes, it facilitates searching for words or phrases within large volumes of text efficiently.
- Key Features of Full-Text Search:
- Ability to search for words and phrases within text columns.
- Support for complex queries using operators like `AND`, `OR`, and `NOT`.
- Capability to handle linguistic variations with inflectional forms and thesaurus support.
Creating Full-Text Indexes
Before you can perform a full-text search, you must create a full-text index on the target table. The following steps outline the process:
- Create a Full-Text Catalog:
“`sql
CREATE FULLTEXT CATALOG MyFullTextCatalog AS DEFAULT;
“`
- Create a Full-Text Index:
“`sql
CREATE FULLTEXT INDEX ON MyTable(TextColumn)
KEY INDEX MyPrimaryKeyIndex
WITH CHANGE_TRACKING AUTO;
“`
Important Considerations:
- Ensure that the table has a primary key index, as full-text indexes depend on it.
- Full-text indexes can only be created on columns of type `CHAR`, `VARCHAR`, `NCHAR`, `NVARCHAR`, `TEXT`, or `NTEXT`.
Utilizing Full-Text Search Queries
Once the full-text index is established, you can execute queries using the `CONTAINS` and `FREETEXT` predicates.
- Using `CONTAINS`:
“`sql
SELECT * FROM MyTable
WHERE CONTAINS(TextColumn, ‘search term’);
“`
- Using `FREETEXT`:
“`sql
SELECT * FROM MyTable
WHERE FREETEXT(TextColumn, ‘search term’);
“`
Examples of Search Queries:
- To find rows containing the exact phrase:
“`sql
WHERE CONTAINS(TextColumn, ‘”exact phrase”‘);
“`
- To search for synonyms or variations:
“`sql
WHERE FREETEXT(TextColumn, ‘search term’);
“`
Advanced Full-Text Search Options
SQL Server supports various advanced searching capabilities to refine results further.
- Using `CONTAINS` with Wildcards:
“`sql
WHERE CONTAINS(TextColumn, ‘term*’);
“`
- Combining Searches:
“`sql
WHERE CONTAINS(TextColumn, ‘(“first term” AND “second term”) OR “third term”‘);
“`
- Ranking Results: Full-text queries can return a relevance ranking, which can be useful for displaying results.
“`sql
SELECT *, CONTAINS(TextColumn, ‘term’) AS Rank
FROM MyTable
WHERE CONTAINS(TextColumn, ‘term’)
ORDER BY Rank DESC;
“`
Performance Considerations
Implementing full-text search can enhance query performance, but it requires careful planning.
- Index Maintenance: Regularly update and rebuild full-text indexes to optimize performance.
- Monitoring Usage: Use SQL Server’s dynamic management views (DMVs) to monitor the effectiveness of full-text indexes.
- Resource Management: Ensure that the server has adequate memory and processing power to handle full-text search operations, especially with large datasets.
Incorporating full-text search capabilities into your SQL Server database provides powerful tools for searching and analyzing textual data efficiently. By understanding how to create and utilize full-text indexes and queries, you can significantly enhance the search functionality within your applications.
Expert Insights on MS SQL Search Stored Procedures for Text
Dr. Emily Carter (Database Architect, Tech Innovations Inc.). “When designing stored procedures for text search in MS SQL, it is crucial to leverage Full-Text Search capabilities. This allows for more efficient querying of large text fields, enhancing performance and accuracy in retrieving relevant data.”
Mark Thompson (Senior SQL Developer, Data Solutions Group). “Implementing stored procedures that utilize the CONTAINS and FREETEXT predicates can significantly improve the search functionality within your SQL Server database. These predicates allow for more natural language processing, making it easier for users to find the information they need.”
Linda Zhang (Data Analyst, Analytics Pro). “It’s essential to index the text columns properly when creating stored procedures for text searches. Without appropriate indexing, even the best-written procedures can lead to slow performance and unresponsive queries, especially as the dataset grows.”
Frequently Asked Questions (FAQs)
What are stored procedures in MS SQL?
Stored procedures in MS SQL are precompiled collections of one or more SQL statements that can be executed as a single unit. They enhance performance, promote code reuse, and encapsulate business logic.
How can I create a stored procedure for text search in MS SQL?
To create a stored procedure for text search, use the `CREATE PROCEDURE` statement along with the `SELECT` statement incorporating `LIKE`, `CHARINDEX`, or full-text search functions such as `CONTAINS` or `FREETEXT` depending on the search requirements.
What is full-text search in MS SQL?
Full-text search in MS SQL allows for sophisticated querying of text-based data. It enables searching for words or phrases in large text columns using the `CONTAINS` and `FREETEXT` predicates, offering better performance and accuracy compared to standard SQL `LIKE` queries.
How do I optimize a stored procedure for text searching?
To optimize a stored procedure for text searching, ensure proper indexing, utilize full-text indexes for large text columns, avoid unnecessary complexity in queries, and consider filtering results to reduce the dataset being processed.
Can I pass parameters to a stored procedure for text search?
Yes, you can pass parameters to a stored procedure for text search. Define input parameters in the stored procedure declaration and use them in your SQL queries to dynamically filter results based on user input.
What are common performance issues with text searches in MS SQL?
Common performance issues with text searches include lack of proper indexing, using non-optimized queries, and searching large datasets without filtering. Implementing full-text indexing and refining search queries can mitigate these issues.
In the realm of Microsoft SQL Server, searching for text within stored procedures is a critical task for database administrators and developers. The ability to efficiently locate specific text strings or keywords within stored procedures can significantly enhance code maintenance and debugging processes. SQL Server provides various methods to accomplish this, including querying system catalog views such as `sys.sql_modules` and `sys.objects`, which contain the definitions of stored procedures. Utilizing these catalog views allows users to filter and retrieve relevant stored procedures based on specific text criteria.
Moreover, employing dynamic SQL can further streamline the search process. By constructing a query that dynamically searches through the definitions of stored procedures, users can automate the identification of procedures containing particular text. This approach not only saves time but also reduces the potential for human error during manual searches. Additionally, leveraging built-in functions such as `CHARINDEX` or `PATINDEX` can enhance the search capabilities, allowing for more complex pattern matching and string manipulation.
mastering the techniques for searching text within stored procedures in MS SQL Server is essential for effective database management. By utilizing system catalog views and dynamic SQL, along with built-in string functions, users can efficiently locate and analyze stored procedures, leading to improved database performance and maintenance. As organizations continue
Author Profile
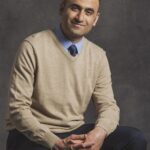
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?