How Can I Search for Text in Stored Procedures in MSSQL?
In the world of database management, efficiency and precision are paramount, especially when working with Microsoft SQL Server (MSSQL). As databases grow in size and complexity, the need for effective search capabilities becomes increasingly critical. One of the powerful features that MSSQL offers is the ability to create and utilize stored procedures, which can encapsulate complex queries and business logic. However, as developers and database administrators navigate through numerous stored procedures, the challenge of searching for specific text or keywords within these procedures can become daunting. This article delves into the strategies and techniques for efficiently searching stored procedures in MSSQL, enabling you to streamline your workflow and enhance your database management practices.
When managing a large number of stored procedures, locating specific text or code snippets can be a time-consuming task. MSSQL provides various methods to facilitate this search, allowing developers to quickly identify relevant procedures that contain the desired text. From leveraging built-in system views to utilizing dynamic SQL scripts, there are several approaches that can help you navigate the complexities of your database with ease. Understanding these methods not only saves time but also improves overall productivity, making it easier to maintain and update your database systems.
In addition to the technical aspects, it’s essential to consider best practices for organizing and documenting stored procedures. By
Searching for Text in Stored Procedures
To effectively search for text within stored procedures in Microsoft SQL Server, you can utilize system catalog views and built-in functions. This process allows you to find specific keywords, phrases, or even patterns embedded within the definitions of your stored procedures.
One of the most common methods is to query the `sys.sql_modules` view, which contains the definitions of all SQL Server objects, including stored procedures. You can also join this with `sys.objects` to filter results to only include stored procedures.
Here’s an example of how to construct a SQL query to find stored procedures containing a specific text:
“`sql
SELECT
o.name AS ProcedureName,
m.definition AS ProcedureDefinition
FROM
sys.sql_modules AS m
JOIN
sys.objects AS o ON m.object_id = o.object_id
WHERE
o.type = ‘P’ — ‘P’ indicates stored procedures
AND m.definition LIKE ‘%YourSearchText%’
“`
Replace `YourSearchText` with the text you are looking for. This query will return the names and definitions of all stored procedures that include the specified text.
Utilizing the INFORMATION_SCHEMA
Another approach involves using the `INFORMATION_SCHEMA` views, which provide a standardized way to retrieve metadata about database objects. Although this method is slightly less direct than using `sys.sql_modules`, it is still effective.
You can execute the following query:
“`sql
SELECT
ROUTINE_NAME,
ROUTINE_DEFINITION
FROM
INFORMATION_SCHEMA.ROUTINES
WHERE
ROUTINE_TYPE = ‘PROCEDURE’
AND ROUTINE_DEFINITION LIKE ‘%YourSearchText%’
“`
This query offers similar results and is compliant with SQL standards, making it a good option for databases that might be accessed using multiple database systems.
Considerations for Large Objects
Be aware that if your stored procedures contain large amounts of code, the `ROUTINE_DEFINITION` field may not return the entire definition. In such cases, it is advisable to use the `sys.sql_modules` view for more reliable results.
Performance Implications
When executing searches, especially on larger databases, consider the performance impact. Queries that utilize `LIKE` with leading wildcards (`%`) can be less efficient, as they may result in full table scans. Here are some strategies to mitigate performance issues:
- Limit the scope by using additional filters (e.g., schema name).
- Run searches during off-peak hours to reduce the impact on database performance.
- Consider creating an index on the relevant columns if searches are frequent.
Method | Pros | Cons |
---|---|---|
sys.sql_modules | Direct access to stored procedure definitions | May return large results for complex procedures |
INFORMATION_SCHEMA | Standardized approach, cross-platform compatibility | Potential truncation of large procedure definitions |
Searching for Text in Stored Procedures
To search for specific text within stored procedures in Microsoft SQL Server (MSSQL), you can utilize system views such as `sys.sql_modules` and `sys.objects`. These views allow you to query the definition of stored procedures stored in the database.
Using T-SQL to Search
The following T-SQL query can be employed to search for a specific string within the definitions of all stored procedures in your database:
“`sql
SELECT
o.name AS ProcedureName,
m.definition AS ProcedureDefinition
FROM
sys.sql_modules m
JOIN
sys.objects o ON m.object_id = o.object_id
WHERE
o.type = ‘P’ — P indicates Stored Procedures
AND m.definition LIKE ‘%search_text%’ — Replace ‘search_text’ with your search term
ORDER BY
o.name;
“`
Explanation of the Query Components:
- sys.sql_modules: Contains the definitions of SQL Server objects.
- sys.objects: Contains a row for each object that is created within a database, including stored procedures.
- o.type = ‘P’: Filters results to include only stored procedures.
- LIKE ‘%search_text%’: Utilizes pattern matching to search for the specified text.
Considerations When Searching
- Case Sensitivity: The search is case-sensitive or case-insensitive depending on the collation settings of your database.
- Performance: Searching for text in large databases or in a significant number of stored procedures can impact performance.
- Permissions: Ensure that you have the necessary permissions to access the system views and stored procedure definitions.
Alternative Methods
If you prefer a graphical interface, tools such as SQL Server Management Studio (SSMS) provide functionalities to search within the object explorer.
Steps to Search in SSMS:
- Open SSMS and connect to your database.
- Right-click on the database name and select “View Dependencies”.
- Use the “Object Explorer” to manually locate stored procedures and view their definitions.
Using Third-Party Tools
Consider using third-party SQL Server management tools that offer enhanced search capabilities for stored procedures and other database objects. Some popular tools include:
Tool Name | Features |
---|---|
Redgate SQL Search | Fast search across all SQL Server objects. |
ApexSQL Search | Comprehensive search functionality with filters. |
dbForge Studio | Advanced search options and code navigation. |
These tools can simplify the search process and enhance productivity.
Automating the Search Process
For recurring searches or batch processing, consider writing a stored procedure to encapsulate the search logic. This can be scheduled as a job or called as needed, providing a reusable solution for text searches across stored procedures.
“`sql
CREATE PROCEDURE SearchStoredProcedures
@SearchText NVARCHAR(100)
AS
BEGIN
SELECT
o.name AS ProcedureName,
m.definition AS ProcedureDefinition
FROM
sys.sql_modules m
JOIN
sys.objects o ON m.object_id = o.object_id
WHERE
o.type = ‘P’
AND m.definition LIKE ‘%’ + @SearchText + ‘%’
ORDER BY
o.name;
END
“`
This stored procedure takes a search string as input and returns all matching stored procedures.
Expert Insights on Searching Stored Procedures in MSSQL
Dr. Emily Carter (Database Architect, Tech Solutions Inc.). “When searching for specific text within stored procedures in MSSQL, utilizing the system views such as sys.sql_modules and sys.objects can be highly effective. This method allows you to query the definition of stored procedures directly, enabling you to filter results based on the text you are interested in.”
James Thornton (Senior SQL Developer, Data Dynamics). “A common approach to finding text in stored procedures is to use dynamic SQL combined with the LIKE operator. This allows for flexible searching and can be tailored to look for specific keywords or phrases within the procedure definitions.”
Linda Chen (Database Management Consultant, Optimal Data Solutions). “For comprehensive analysis, consider creating a script that iterates through all stored procedures and captures their definitions. This not only aids in searching but also helps in maintaining documentation for auditing purposes.”
Frequently Asked Questions (FAQs)
How can I search for specific text within stored procedures in MSSQL?
You can search for specific text within stored procedures in MSSQL by querying the `sys.sql_modules` and `sys.objects` system views. Use a SQL query that filters the `definition` column for your desired text and joins it with the `sys.objects` view to get the names of the procedures.
What SQL query can I use to find stored procedures containing a particular keyword?
You can use the following SQL query:
“`sql
SELECT OBJECT_NAME(object_id) AS ProcedureName
FROM sys.sql_modules
WHERE definition LIKE ‘%your_keyword%’
AND OBJECTPROPERTY(object_id, ‘IsProcedure’) = 1;
“`
Replace `your_keyword` with the text you are searching for.
Is it possible to search for text in all types of objects in MSSQL?
Yes, you can search for text in all types of objects by modifying the query to include other object types, such as views or functions. You can adjust the `OBJECTPROPERTY` function to filter based on the specific object type you want to include.
What are the performance implications of searching through stored procedures in MSSQL?
Searching through stored procedures can impact performance, especially in databases with a large number of procedures or complex definitions. It is advisable to limit the search scope or run such queries during off-peak hours to minimize the impact on system performance.
Can I automate the process of searching for text in stored procedures?
Yes, you can automate the process by creating a SQL Server Agent job or a stored procedure that encapsulates the search logic. This allows for scheduled searches and can help maintain documentation or code standards.
Are there any third-party tools available for searching text in MSSQL stored procedures?
Yes, several third-party tools, such as Redgate SQL Search and ApexSQL Search, provide enhanced functionality for searching through stored procedures and other database objects. These tools often offer user-friendly interfaces and additional features for better code management.
In summary, searching for text within stored procedures in Microsoft SQL Server (MSSQL) can be a crucial task for database administrators and developers. This process typically involves querying system catalog views such as `sys.sql_modules` and `sys.objects` to locate specific text strings within the definition of stored procedures. By utilizing SQL queries that filter these system views, users can effectively identify and retrieve stored procedures containing the desired text.
Additionally, leveraging the power of SQL Server’s built-in functions, such as `CHARINDEX` or `PATINDEX`, can enhance the search capabilities. These functions allow for more complex search patterns and can help in locating text that may not be an exact match. Furthermore, understanding the structure of the database and the naming conventions used can significantly streamline the search process, making it more efficient and effective.
Ultimately, the ability to search stored procedures for specific text not only aids in code maintenance and debugging but also contributes to better documentation practices. By regularly auditing stored procedures and their contents, organizations can ensure that their database systems remain robust, secure, and aligned with best practices in software development.
Author Profile
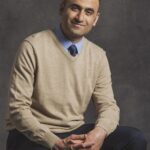
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?