How Can You Check if MUI DataGrid Has Finished Re-rendering?
When working with data-heavy applications, ensuring a smooth user experience is paramount. One of the most popular tools for displaying and managing data in React applications is the MUI DataGrid. However, developers often face a common challenge: determining when the grid has finished re-rendering. This seemingly simple task can significantly impact the performance and responsiveness of your application. In this article, we will explore effective strategies for detecting the completion of re-renders in MUI DataGrid, empowering you to create more efficient and user-friendly interfaces.
Understanding the re-rendering lifecycle of the MUI DataGrid is crucial for optimizing your application’s performance. The grid is designed to handle large datasets and complex operations, but with great power comes the need for careful management. By knowing when the grid has completed its rendering process, developers can implement additional logic, such as triggering animations, updating related components, or fetching new data. This knowledge not only enhances user experience but also helps in maintaining the integrity of data displayed in the grid.
In the following sections, we will delve into various techniques and best practices to monitor the rendering state of the MUI DataGrid. From leveraging built-in lifecycle methods to utilizing custom hooks, we will provide you with the tools you need to ensure that your application remains responsive and efficient. Whether
Understanding Re-rendering in MUI DataGrid
MUI’s DataGrid component is designed for handling large datasets efficiently. However, determining when the grid has completed its re-rendering can be crucial for implementing features such as loading indicators or triggering dependent actions. The re-rendering process can be influenced by various factors, including state changes, data updates, and configuration alterations.
To check if the grid has finished re-rendering, you can leverage the component’s lifecycle methods or hooks. Here are some approaches to consider:
– **Using `useEffect` Hook**: If you’re managing the grid’s data through React state, you can use the `useEffect` hook to listen for changes in the data. By doing so, you can determine when the grid has updated.
“`javascript
useEffect(() => {
// Code to execute after the grid has re-rendered
}, [gridData]); // Dependency on your grid data
“`
- Grid Callbacks: MUI DataGrid provides several callback props that can help you track changes. For instance, you can use the `onProcessRowUpdate` or `onRowEditComplete` callbacks to know when a user interaction has completed a row update.
Implementing a Loading Indicator
When dealing with asynchronous data fetching, displaying a loading indicator while the DataGrid is updating can enhance user experience. Here’s how you can implement it:
– **State Management**: Utilize a loading state in your component to indicate when data is being fetched.
“`javascript
const [loading, setLoading] = useState();
const fetchData = async () => {
setLoading(true);
// Fetch data logic
setLoading();
};
“`
- Conditional Rendering: Display a loading spinner based on the loading state.
“`javascript
return (
<>
{loading ?
>
);
“`
Performance Considerations
Optimizing the performance of the MUI DataGrid can lead to smoother interactions, especially during re-rendering. Consider the following strategies:
- Row Virtualization: Enable row virtualization to ensure only the visible rows are rendered, reducing the load on the browser.
- Memoization: Use `React.memo` for row components to prevent unnecessary re-renders.
- Debouncing Data Changes: If your application involves frequent updates to the grid data, implement debouncing techniques to limit the number of re-renders.
Example Configuration Table
The following table illustrates some configuration options that can impact the rendering behavior of the MUI DataGrid:
Property | Description | Default Value |
---|---|---|
rows | Data to be displayed in the grid | [] |
columns | Configuration for the grid columns | [] |
autoHeight | Automatically adjust grid height based on data | |
pagination | Enable pagination for large datasets |
By understanding how to monitor and optimize the re-rendering process in MUI’s DataGrid, you can create a more responsive and efficient user experience.
Detecting Re-Render Completion in MUI DataGrid
In MUI DataGrid, determining when the grid has finished re-rendering can be crucial for implementing features that depend on the final state of the grid. While there isn’t a built-in event specifically for detecting the completion of a re-render, developers can utilize a few strategies to achieve this.
Using State Management and Effect Hooks
One effective approach involves leveraging React’s state management in conjunction with the `useEffect` hook. By tracking the data and configuration changes, you can ascertain when the grid is finished updating.
– **Track State Changes**: Use state variables to track the data and configuration of the grid.
– **Implement `useEffect`**: This hook can be set to trigger when the state variables change, providing a callback to run code after the re-render.
“`javascript
import React, { useEffect, useState } from ‘react’;
import { DataGrid } from ‘@mui/x-data-grid’;
const MyDataGrid = () => {
const [rows, setRows] = useState([]);
const [columns, setColumns] = useState([]);
// Effect to run after rows or columns change
useEffect(() => {
// Code to execute after re-render
console.log(‘Grid has re-rendered’);
}, [rows, columns]);
// Assume fetchData is a function to fetch rows
const fetchData = () => {
// Fetch and set rows and columns
setRows(newData);
setColumns(newColumns);
};
return
};
“`
Custom Callback Function
Another method to check if the grid is finished rendering is to create a custom callback function that executes once the data is set. This can be called after updating the state.
– **Define a Callback**: Create a function that runs actions after the data is updated.
– **Invoke Post-State Update**: Call this function right after setting the state.
“`javascript
const onDataUpdate = () => {
console.log(‘Data has been updated, grid may have re-rendered.’);
};
const fetchData = () => {
setRows(newData);
onDataUpdate();
};
“`
Listening to Events
MUI DataGrid provides several events that can also be utilized to monitor grid behavior. While these do not directly indicate a re-render, they can provide context about user interactions.
- Row Events: Listen for events like `onRowSelected` or `onRowEdit` to gauge user interaction.
- Page Events: Use `onPageChange` or `onPageSizeChange` to determine when the grid’s pagination changes.
“`javascript
onRowSelected={() => console.log(‘Row selected’)}
/>
“`
Performance Optimization Strategies
To ensure that the grid operates smoothly while managing re-renders, consider the following strategies:
- Memoization: Use `React.memo` for row components to prevent unnecessary re-renders.
- Virtualization: Ensure that large datasets are efficiently rendered by utilizing virtualization features in DataGrid.
- Debouncing State Updates: When fetching or updating data, debounce the updates to reduce the frequency of state changes and subsequent re-renders.
By implementing these methods, you can effectively manage and detect the completion of re-renders in MUI DataGrid, leading to a more responsive and interactive user experience.
Expert Insights on Detecting Re-rendering in MUI DataGrid
Dr. Emily Carter (Frontend Development Specialist, UI Innovations Inc.). “To determine if the MUI DataGrid has finished re-rendering, developers can utilize the `onStateChange` event, which allows tracking state updates. This event can signal when the grid has completed its rendering cycle, enabling developers to execute subsequent logic only after the grid is fully updated.”
Michael Chen (Senior Software Engineer, React Solutions Group). “One effective method to check if the MUI DataGrid is finished re-rendering is by leveraging the `useEffect` hook in combination with a state variable that tracks loading status. By setting this state variable to after the grid’s data has been updated, you can ensure that any dependent processes only run once the grid is fully rendered.”
Lisa Tran (UI/UX Architect, Digital Design Labs). “Implementing a loading spinner or similar visual cue during the rendering process can provide insights into the grid’s state. Additionally, using the `loading` prop of the DataGrid can help manage the user experience by indicating when the grid is still processing data, thus allowing for better control over subsequent actions.”
Frequently Asked Questions (FAQs)
How can I determine when the MUI DataGrid has finished re-rendering?
You can utilize the `onStateChange` event provided by the MUI DataGrid. This event triggers whenever the grid’s state changes, allowing you to implement logic to check if the re-rendering process is complete.
Is there a built-in event in MUI DataGrid for detecting re-render completion?
MUI DataGrid does not have a specific built-in event for detecting the completion of re-rendering. However, you can leverage the `onStateChange` or `onRowsScrollEnd` events to monitor changes and infer when rendering is complete.
Can I use a timeout to check if the DataGrid has finished rendering?
While using a timeout can be a workaround, it is not a reliable method. Instead, it is advisable to use event-driven approaches like `onStateChange` to accurately determine when rendering is complete.
What performance considerations should I keep in mind when checking for re-rendering?
Frequent checks or heavy computations during rendering can degrade performance. It is recommended to debounce your checks or limit the frequency of state checks to ensure optimal performance.
How can I manage state updates effectively during DataGrid re-renders?
To manage state updates effectively, consider using React’s `useEffect` hook in conjunction with the DataGrid’s state change events. This approach allows you to respond to changes without unnecessary re-renders.
Are there any best practices for optimizing rendering in MUI DataGrid?
Best practices include minimizing the number of rows rendered, using virtualization, and optimizing column definitions. Additionally, avoid unnecessary state updates that can trigger re-renders.
The MUI DataGrid is a powerful component for displaying and managing data in a tabular format within React applications. One common concern developers face is determining when the grid has finished re-rendering, especially after updates to the data or configuration. Understanding the lifecycle of the DataGrid and its rendering behavior is crucial for optimizing performance and ensuring a smooth user experience.
To check if the DataGrid has finished re-rendering, developers can utilize various lifecycle methods and event handlers provided by the component. The `onStateChange` event is particularly useful, as it triggers whenever the state of the DataGrid changes, allowing developers to implement custom logic after the grid updates. Additionally, leveraging React’s built-in hooks, such as `useEffect`, can help monitor changes in the data and respond accordingly when the grid completes its re-rendering process.
Key takeaways include the importance of understanding the rendering lifecycle of the MUI DataGrid and effectively utilizing the available event handlers to manage state changes. By implementing these strategies, developers can enhance the performance of their applications and provide a better user experience. Monitoring re-renders not only aids in debugging but also ensures that any dependent components or side effects are executed at the appropriate time.
Author Profile
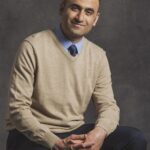
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?