What Are the Multiple Definitions of ‘First’ Defined Here?
In the world of programming, encountering errors is an inevitable part of the development process. Among the myriad of error messages that can arise, few are as perplexing as the dreaded “multiple definition of first defined here.” This cryptic phrase often leaves developers scratching their heads, wondering what went wrong and how to rectify the situation. Understanding this error is crucial for anyone looking to write clean, efficient code and maintain a smooth workflow. In this article, we will delve into the intricacies of this error, exploring its common causes, implications, and effective solutions.
Overview
The “multiple definition” error typically occurs in languages like C or C++ when the same variable or function is defined in multiple places within a program. This redundancy can lead to confusion for the compiler, which struggles to determine which definition to prioritize. As a result, developers may find themselves facing compilation failures, hindering their progress and productivity. The error not only highlights the importance of proper code organization but also underscores the need for a solid understanding of scope and linkage.
Navigating this error requires a keen eye for detail and a methodical approach to code management. By recognizing the scenarios that lead to multiple definitions, programmers can implement best practices that minimize the risk of encountering this issue. Through careful struct
Understanding the Error Message
The error message “multiple definition of first defined here” commonly occurs during the compilation phase of programming, particularly in C and C++. It indicates that the linker has found multiple definitions of a function or variable that it cannot resolve to a single instance. This situation typically arises when the same function or variable is defined in multiple source files or within a single source file, leading to ambiguity.
Common Causes of the Error
Several scenarios can trigger this error. Recognizing these can help prevent it:
- Multiple Definitions in Source Files: When a function or variable is implemented in more than one source file without proper declaration.
- Header File Mismanagement: Including a header file in multiple source files without using include guards or `pragma once`.
- Static Variables: Defining a static variable in a header file that is included in multiple source files.
- Inconsistent Linkage: Using different linkage specifications (e.g., `extern` vs. non-extern) across different files.
Best Practices to Avoid the Error
To mitigate the risk of encountering this error, consider the following practices:
- Use Header Guards: Always wrap your header files in include guards to prevent multiple inclusions.
- Define in One Source File: Ensure that functions and variables are defined only once in a single source file.
- Use `extern` for Global Variables: Declare global variables as `extern` in header files and define them in only one source file.
- Organize Code Structure: Group related functions into the same source file to maintain clarity and prevent duplication.
Example of Proper Usage
The following table illustrates the correct approach to defining a variable in a header and a source file.
File | Code | Explanation |
---|---|---|
header.h | “`c ifndef HEADER_H define HEADER_H extern int globalVar; endif“` |
Declares the variable as external to prevent multiple definitions. |
source.c | “`c include “header.h” int globalVar = 0;“` |
Defines the variable in one source file, ensuring only one definition exists. |
By following these guidelines and understanding the roots of the error message, developers can avoid the pitfalls of multiple definitions in their projects.
Understanding Multiple Definition Errors
When encountering the error message “multiple definition of first defined here,” it indicates a conflict in your code where the same function, variable, or object is defined in multiple places. This situation typically arises in C or C++ programming, where the linker finds multiple definitions during the linking phase.
Common Causes of Multiple Definition Errors
- Global Variables: Defining a global variable in multiple source files without using the `extern` keyword can lead to this error.
- Function Definitions: Having the same function defined in different source files results in a conflict during the linking process.
- Static Variables: Using static variables in header files included by multiple source files without proper guarding mechanisms.
- Templates: In C++, if template implementations are placed in headers without appropriate inline specifications, it can cause multiple definitions.
Example Scenarios
Global Variable Example:
“`c
// File1.c
int globalVar = 10;
// File2.c
int globalVar = 20; // Error: multiple definition
“`
Function Definition Example:
“`c
// File1.c
void myFunction() {
// Function implementation
}
// File2.c
void myFunction() { // Error: multiple definition
// Another implementation
}
“`
Best Practices to Avoid Multiple Definition Errors
- Use `extern` for Global Variables: Declare global variables in header files as `extern` and define them in one source file.
- Implement Functions in One Source File: Define functions in a single source file; use `extern` declarations in headers.
- Include Guards in Headers: Use include guards or `pragma once` in header files to prevent multiple inclusions.
“`c
// Example of include guard
ifndef MY_HEADER_H
define MY_HEADER_H
// Declarations
endif // MY_HEADER_H
“`
Debugging Multiple Definition Errors
- Check Linker Error Messages: Read the error messages carefully to identify the conflicting symbols.
- Review Your Includes: Ensure that header files are correctly guarded and not causing multiple inclusions.
- Search for Duplicate Definitions: Use search functionalities in your IDE to find where symbols are defined.
- Refactor Code: If necessary, refactor your code structure to segregate definitions from declarations.
Using Tools to Identify Issues
Employing tools like `nm`, `objdump`, or IDE features can help locate multiple definitions. For example:
- Using `nm`:
“`bash
nm -g
“`
- Using `objdump`:
“`bash
objdump -t
“`
These commands can help identify where multiple definitions exist across your compiled object files.
Addressing multiple definition errors requires careful management of your code’s structure and definitions. By following best practices and utilizing debugging tools, you can effectively resolve these conflicts and prevent them in future projects.
Understanding Multiple Definitions in Programming Contexts
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The error message ‘multiple definition of first defined here’ typically arises during the linking stage of compilation in C and C++. This indicates that the same symbol has been defined in multiple translation units, leading to ambiguity. It is crucial for developers to ensure that function and variable definitions are consistent across files to prevent such conflicts.”
Michael Chen (Lead Compiler Developer, CodeCraft Solutions). “When encountering the ‘multiple definition of first defined here’ error, it is essential to review your header files and the use of include guards or `pragma once`. Properly managing declarations and definitions can significantly reduce the likelihood of this error, ensuring that each symbol is defined only once in the entire program.”
Sarah Thompson (Software Development Instructor, Code Academy). “Students often face the ‘multiple definition’ error when they are new to C/C++ programming. I emphasize the importance of understanding the difference between declarations and definitions. By teaching proper scoping and modular programming practices, students can avoid this common pitfall and write cleaner, more maintainable code.”
Frequently Asked Questions (FAQs)
What does “multiple definition of first defined here” mean?
This error message indicates that a variable, function, or object has been defined more than once in the same scope, leading to ambiguity in the code.
What causes the “multiple definition” error in programming?
The error typically arises from including the same header file multiple times in different source files without proper include guards, or from defining functions or variables in header files instead of declaring them.
How can I resolve the “multiple definition” error?
To resolve this error, ensure that you use include guards in header files, declare functions and variables in headers, and define them in corresponding source files to avoid multiple definitions.
Are there specific programming languages where this error is common?
Yes, this error is particularly common in languages like C and C++ due to their compilation model, where separate compilation units can lead to multiple definitions if not managed correctly.
What are include guards, and how do they help?
Include guards are preprocessor directives that prevent a header file from being included multiple times in a single compilation unit, thereby avoiding multiple definitions of the same entities.
Can this error occur in object-oriented programming?
Yes, in object-oriented programming, this error can occur if class members are defined in header files without being declared as inline or if the same class is instantiated in multiple translation units without proper management.
The term “multiple definition” refers to the phenomenon where a single word or phrase can have several meanings, depending on the context in which it is used. This concept is crucial in linguistics, as it highlights the complexity of language and the importance of understanding context for accurate communication. Various fields, including law, literature, and everyday conversation, often encounter words with multiple definitions, which can lead to ambiguity or misinterpretation if not addressed properly.
One of the key insights from the discussion of multiple definitions is the necessity for clarity in communication. When a word possesses more than one meaning, it is essential for speakers and writers to provide sufficient context to guide the audience toward the intended interpretation. This can involve using additional descriptive language or rephrasing sentences to minimize confusion. Additionally, the audience’s familiarity with the subject matter plays a significant role in how effectively they can discern the intended meaning.
Furthermore, the exploration of multiple definitions underscores the dynamic nature of language. As society evolves, so do the meanings of words, which can change over time or develop new connotations. This adaptability reflects cultural shifts and the influence of technology on communication. Therefore, it is vital for individuals to remain aware of these changes and to continually refine their understanding of language
Author Profile
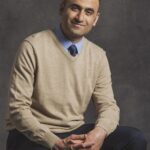
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?