Why Must You Declare a Named Package in Eclipse?
Navigating the world of Java development can be both exciting and challenging, especially when using integrated development environments (IDEs) like Eclipse. One common hurdle that developers encounter is the error message: “must declare a named package.” This seemingly cryptic warning can halt progress and leave even seasoned programmers scratching their heads. Understanding this error is crucial for anyone looking to streamline their coding process and enhance their productivity in Eclipse. In this article, we will delve into the reasons behind this error, its implications for your Java projects, and how to effectively resolve it, ensuring that you can focus on what really matters—writing great code.
When working with Java, packages serve as a fundamental organizational tool, allowing developers to group related classes and interfaces. Eclipse, as a robust IDE, expects developers to adhere to certain conventions, including the declaration of named packages. Failing to do so can lead to confusion and disrupt the compilation process, resulting in frustrating errors that can derail even the simplest of projects. Understanding the significance of package declarations is essential for maintaining clean, manageable code and avoiding common pitfalls.
In this exploration, we will unpack the concept of packages in Java and how they relate to Eclipse’s structure. By examining the reasons behind the “must declare a named package” error,
Understanding Named Packages in Eclipse
When you encounter the error message “must declare a named package” in Eclipse, it typically indicates that your Java class file is not part of a defined package. In Java, packages serve as namespaces that organize classes and interfaces into a hierarchical structure, enhancing code modularity and manageability.
To declare a named package in your Java file, you should include the `package` statement at the very beginning of your source file, before any import statements or class definitions. The syntax is straightforward:
“`java
package com.example.myapp;
“`
This statement tells the Java compiler that the class belongs to the specified package.
Common Causes of the Error
Several factors can lead to the “must declare a named package” error:
- Missing Package Declaration: The most straightforward cause is simply forgetting to include the package declaration at the top of your Java file.
- File Location Mismatch: If your file structure does not match the package declaration, Eclipse may throw this error. For example, if your package is `com.example.myapp`, the file should be located in the directory structure `com/example/myapp/`.
- Default Package Usage: If you have not defined a package, your classes are placed in the default package, which is discouraged for larger projects. To avoid issues, always define a named package.
How to Fix the Error
To resolve the “must declare a named package” error, follow these steps:
- Add a Package Declaration: At the top of your Java file, add the appropriate `package` statement. Make sure it reflects the folder structure of your project.
- Correct Directory Structure: Ensure that your Java file is located in the correct directory corresponding to its package name. For instance, if your package is `com.example.myapp`, your file should be under the path `src/com/example/myapp/`.
- Refactor if Necessary: If you need to change the package name or structure, use Eclipse’s refactor tools to safely rename packages and classes.
Example Package Declaration
Here’s an example of how to properly declare a package in a Java file:
“`java
package com.example.myapp;
public class MyClass {
public void myMethod() {
System.out.println(“Hello, World!”);
}
}
“`
This class `MyClass` is part of the `com.example.myapp` package.
Package Declaration Best Practices
To ensure your Java packages are well-structured and avoid common pitfalls, consider the following best practices:
- Use a Reverse Domain Name: Structure your package names using a reverse domain name format to avoid naming conflicts.
- Keep Packages Small and Focused: Organize related classes into packages to enhance readability and maintainability.
- Avoid Default Package: Always declare a named package; avoid using the default package to prevent confusion and potential issues with class accessibility.
Action | Description |
---|---|
Add Package Declaration | Include the package name at the top of the Java file. |
Check Directory Structure | Ensure the file is located in the correct folder path corresponding to its package. |
Refactor Packages | Use Eclipse tools to rename packages safely. |
By adhering to these guidelines and resolving any errors related to package declarations, you can maintain a clean and efficient Java project within the Eclipse IDE.
Understanding the Error
The “must declare a named package” error in Eclipse typically arises when Java source files do not declare a package at the top of the file, or when the package structure does not align with the file’s location. This error highlights the importance of adhering to Java’s package conventions.
Common Causes
Several factors can lead to this error:
- Missing Package Declaration: If a Java file does not begin with a package declaration, it defaults to the unnamed package, which can lead to issues when trying to organize classes.
- Incorrect File Path: The location of the Java file must match the declared package structure. For example, a file in `src/com/example/` should declare `package com.example;`.
- Project Configuration Issues: Eclipse projects need to be set up correctly to recognize source folders. If the source folder is misconfigured, it can lead to package declaration problems.
Resolving the Error
To resolve the “must declare a named package” error, follow these steps:
- Add Package Declaration: Ensure that each Java file begins with a proper package declaration. For example:
“`java
package com.example;
“`
- Check File Location: Verify that the Java file is located in the appropriate directory that reflects the package structure. Adjust the file path if necessary.
- Configure Eclipse Project Settings:
- Right-click on the project in the Project Explorer.
- Select Properties.
- Navigate to Java Build Path and ensure that the Source tab includes the correct source folders.
- Clean and Rebuild the Project: Sometimes, Eclipse may not immediately recognize changes. Use the following steps:
- Click on Project in the menu.
- Select Clean and then rebuild the project.
Best Practices for Package Management
To avoid encountering the “must declare a named package” error in the future, consider the following best practices:
- Consistent Naming Conventions: Use lowercase letters for package names to prevent conflicts and confusion.
- Organize Classes Logically: Group related classes within the same package, making it easier to manage and maintain the code.
- Use Source Folders: Always define source folders in your Eclipse project settings to keep the package structure organized.
- Regularly Review Project Structure: Periodically check that all Java files are in the correct directories and that package declarations match.
Example of Proper Package Declaration
Here is an example of a correctly structured Java file:
“`java
package com.example.myapp;
public class MyClass {
public void myMethod() {
System.out.println(“Hello, World!”);
}
}
“`
- File Location: `src/com/example/myapp/MyClass.java`
- Package Declaration: Must match the folder structure.
By adhering to these guidelines and addressing the common causes of the error, developers can ensure a smoother experience when working with Java projects in Eclipse.
Understanding Package Declarations in Eclipse
Dr. Emily Carter (Software Development Consultant, CodeCraft Solutions). “In Java, every class must belong to a named package unless it is in the default package. Eclipse enforces this rule to maintain organization and prevent naming conflicts, which is crucial for large projects.”
Michael Thompson (Java Programming Instructor, Tech Academy). “When you encounter the ‘must declare a named package’ error in Eclipse, it typically indicates that your class files are not properly organized. Ensuring that each file has a corresponding package declaration at the top can resolve this issue swiftly.”
Sarah Jenkins (Senior Software Engineer, Innovative Tech Solutions). “Using named packages in Eclipse not only helps in avoiding conflicts but also enhances code readability and maintainability. Developers should adopt this practice from the outset to streamline their workflow.”
Frequently Asked Questions (FAQs)
What does it mean to declare a named package in Eclipse?
Declaring a named package in Eclipse involves specifying a package name at the beginning of your Java source file. This organizes your classes and interfaces into a namespace, preventing naming conflicts.
Why do I receive an error stating “must declare a named package” in Eclipse?
This error occurs when your Java file does not specify a package declaration at the top. Java requires that all classes be part of a package, unless they are in the default package, which is not recommended for larger projects.
How do I declare a named package in my Java file in Eclipse?
To declare a named package, add the line `package your.package.name;` at the very top of your Java source file, before any import statements or class definitions.
Can I use the default package in Eclipse?
While you can use the default package by omitting the package declaration, it is not advisable for larger applications. Using named packages enhances organization and maintainability.
What are the benefits of using named packages in Java?
Named packages help avoid class name conflicts, improve code organization, facilitate access control, and make it easier to manage large codebases by grouping related classes together.
How can I fix the “must declare a named package” error in an existing project?
To fix this error, open the affected Java file, add a package declaration at the top, and ensure that the file is located in the corresponding directory structure that matches the package name.
In Java programming, particularly when using an Integrated Development Environment (IDE) like Eclipse, declaring a named package is essential for organizing code effectively. A named package allows developers to group related classes and interfaces together, which enhances code maintainability and readability. By adhering to the package naming conventions, such as using the reverse domain name structure, developers can avoid naming conflicts and ensure their code is easily navigable within larger projects.
Furthermore, Eclipse requires that any Java file containing a class definition must declare a package at the beginning of the file if it is not placed in the default package. This declaration is crucial as it informs the Java compiler of the package to which the class belongs, facilitating proper compilation and execution of the program. Failing to declare a named package can lead to organizational issues and complications when integrating with other codebases.
In summary, declaring a named package in Eclipse is not merely a best practice but a requirement for structured Java programming. It fosters better collaboration among developers and contributes to a cleaner, more efficient codebase. By understanding and implementing named packages, developers can significantly improve their coding workflow and project organization.
Author Profile
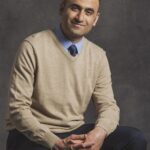
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?