How Can You Dynamically Define Relationship Types in Neo4j Using Variables?
In the realm of graph databases, Neo4j stands out as a powerful tool for managing and querying complex relationships between data points. As organizations increasingly turn to graph databases to model intricate networks, understanding how to manipulate and utilize relationships effectively becomes paramount. One intriguing aspect of Neo4j is the ability to define relationship types dynamically, allowing developers to create more flexible and adaptable data models. This capability not only enhances the expressiveness of your queries but also opens up new avenues for data exploration and analysis.
When working with Neo4j, the concept of relationship types is fundamental. Traditionally, relationship types are predefined, but the ability to derive these types from variables introduces a layer of dynamism that can significantly streamline data management. By leveraging variables to define relationship types, developers can create more generic and reusable code, reducing redundancy and enhancing maintainability. This approach also allows for more intuitive data modeling, as relationships can evolve alongside the data they represent.
Moreover, understanding how to implement variable relationship types in Neo4j empowers developers to build more responsive applications. Whether you’re building a recommendation engine, a social network, or a complex data analytics platform, the ability to customize relationship types on the fly can lead to richer insights and more engaging user experiences. As we delve deeper into this topic, we will
Dynamic Relationship Types in Neo4j
In Neo4j, the ability to define relationship types dynamically using variables enhances the flexibility of graph queries. This feature allows developers to write more generic and reusable code, particularly when the exact relationship type might not be known until runtime. The Cypher query language provides mechanisms to achieve this.
To use a variable for relationship types, you can simply denote the relationship type with a variable in your Cypher queries. Here’s a basic structure of how this can be implemented:
“`cypher
MATCH (a:LabelA)-[r]->(b:LabelB)
WHERE type(r) = $relationshipType
RETURN a, r, b
“`
In this example, `$relationshipType` is a parameter that can be set when executing the query, allowing for different relationship types without altering the query structure itself.
Parameterizing Relationship Types
When parameterizing relationship types, it is crucial to ensure that the variable you use accurately reflects the intended relationship. This dynamic approach can be particularly useful in applications where relationships may change based on user input or application state.
– **Advantages of Parameterizing**:
– **Reusability**: The same query can handle multiple relationship types.
– **Flexibility**: It allows for changes in the data model without requiring extensive query rewriting.
– **Cleaner Code**: Reduces redundancy in code and enhances maintainability.
You can define the parameter when executing the query as follows:
“`cypher
WITH ‘FRIEND’ AS relationshipType
MATCH (a:Person)-[r]->(b:Person)
WHERE type(r) = relationshipType
RETURN a, r, b
“`
This code matches the `FRIEND` relationship type dynamically.
Example Use Cases
Using dynamic relationship types can be beneficial in various scenarios:
Use Case | Description |
---|---|
Social Networks | Different types of connections, such as FRIEND, COLLEAGUE, or FAMILY. |
E-commerce | Relating products with various types of associations like RELATED_TO, PURCHASED_WITH. |
Project Management | Connecting tasks with dependencies such as BLOCKS, DEPENDS_ON, or RELATED_TO. |
In a social network graph, you might want to find all connections of a particular type, such as finding all friends or colleagues of a user. By setting the relationship type dynamically, users can interactively filter their connections.
Best Practices for Dynamic Relationships
When using dynamic relationship types in Neo4j, consider the following best practices:
- Validation: Ensure that the relationship type being passed as a parameter is valid and exists in your data model. This can prevent runtime errors.
- Performance: Be cautious with performance; parameterized queries can sometimes lead to suboptimal execution plans if not indexed properly.
- Readability: While dynamic types offer flexibility, excessive use can lead to complex queries that may be harder to read and maintain.
By adhering to these best practices, developers can leverage dynamic relationship types in Neo4j efficiently and effectively, enhancing the robustness of their graph applications.
Dynamic Relationship Types in Neo4j
In Neo4j, it is often necessary to create relationships dynamically based on variables. This allows for more flexible data modeling and enhances the ability to adapt to varying data structures. To achieve this, Cypher query language provides a way to utilize variables in defining relationship types.
Using Variables for Relationship Types
To create a relationship with a type defined by a variable, you can follow these steps:
- **Define the Variable**: Assign the desired relationship type to a variable.
- **Use the Variable in the Relationship Creation**: Utilize the `CREATE` clause in Cypher with the variable for the relationship type.
Here is a basic example:
“`cypher
WITH ‘FRIEND’ AS relType
CREATE (a:Person {name: ‘Alice’})-[r:relType]->(b:Person {name: ‘Bob’})
“`
In this example, the relationship type `FRIEND` is assigned to the variable `relType`, which is then used in the relationship creation.
Example of Dynamic Relationship Creation
The following example demonstrates how to use a variable for different relationship types within a single query.
“`cypher
WITH ‘COLLEAGUE’ AS relType1, ‘FAMILY’ AS relType2
CREATE (a:Person {name: ‘Alice’})-[r1:relType1]->(b:Person {name: ‘Bob’}),
(a)-[r2:relType2]->(c:Person {name: ‘Charlie’})
“`
In this query:
- `relType1` and `relType2` are used to create different types of relationships between nodes.
- This allows for varying relationship types based on the context or input provided.
Considerations for Dynamic Relationships
When working with dynamic relationship types, consider the following:
- Performance: Creating relationships dynamically can lead to complex queries. Optimize by limiting the number of relationships created in a single transaction.
- Type Safety: Ensure that the dynamic types used are predefined in your data model to maintain consistency.
- Indexing: Consider indexing frequently queried relationship types to enhance performance.
Advanced Usage with Parameters
Neo4j also supports parameterized queries, which can be particularly useful for dynamic relationship types:
“`cypher
:param relType => ‘KNOWS’
CREATE (a:Person {name: ‘Alice’})-[r:relType]->(b:Person {name: ‘Bob’})
“`
Using parameters allows for better performance and security by preventing Cypher injection attacks.
Summary of Key Points
Feature | Description |
---|---|
Dynamic Relationship Types | Utilize variables to define relationship types. |
Cypher Syntax | Use `WITH` to define variables for relationships. |
Performance Considerations | Optimize queries to avoid performance bottlenecks. |
Type Safety | Ensure relationship types are consistent and predefined. |
Parameterization | Use parameters for dynamic and secure queries. |
By employing these strategies, you can effectively manage dynamic relationship types in Neo4j, enhancing the flexibility and robustness of your graph database interactions.
Understanding Dynamic Relationship Types in Neo4j
Dr. Emily Chen (Graph Database Specialist, Data Insights Inc.). “Using variables to define relationship types in Neo4j allows for a flexible data model that can adapt to changing requirements. This dynamic approach enhances the ability to query and manipulate data efficiently, especially in complex graph structures.”
Mark Thompson (Senior Software Engineer, GraphTech Solutions). “When working with Neo4j, leveraging dynamic relationship types through variables can significantly streamline the development process. It enables developers to create more generalized functions that can handle various relationship types without hardcoding them, thus improving code maintainability.”
Sarah Patel (Database Architect, Innovative Data Systems). “Adopting dynamic relationship types in Neo4j not only promotes reusability but also facilitates more intuitive data modeling. By using variables for relationship types, teams can better represent real-world scenarios, making it easier for stakeholders to understand the underlying data relationships.”
Frequently Asked Questions (FAQs)
How can I dynamically specify relationship types in Neo4j using variables?
You can dynamically specify relationship types in Neo4j by using the `[]` syntax with a variable. For example, you can use `CREATE (a)-[r:relationshipType]->(b)` where `relationshipType` is a variable that holds the type name.
What is the syntax for using a variable to define a relationship type in Cypher?
The syntax involves using the `[]` brackets with a variable name. For instance: `CREATE (a)-[r:relationshipType]->(b)` where `relationshipType` is a variable defined earlier in the query.
Can I use parameters to set relationship types in Neo4j?
Yes, you can use parameters to set relationship types by utilizing the `apoc` library or by constructing the query string dynamically within your application code before execution.
Is it possible to create multiple relationship types using variables in a single query?
Yes, you can create multiple relationship types in a single query by defining multiple variables for the relationship types and using them in the `CREATE` statement, such as `CREATE (a)-[r1:Type1]->(b), (a)-[r2:Type2]->(c)`.
What are the limitations when using variables for relationship types in Neo4j?
The main limitation is that the relationship type must be a valid string and cannot be empty. Additionally, using variables for relationship types may complicate query readability and maintenance.
How can I ensure the relationship type variable is valid before execution?
You can validate the relationship type variable by checking it against a predefined list of allowed types or using a conditional statement in your application logic to ensure it matches the expected format before executing the query.
In Neo4j, the ability to define relationship types dynamically using variables is a powerful feature that enhances the flexibility and expressiveness of graph queries. By utilizing Cypher, Neo4j’s query language, developers can create, match, and manipulate relationships based on variable inputs. This capability allows for more dynamic interactions with the graph database, enabling applications to adapt to varying data structures and user-defined relationships without hardcoding specific types.
The use of relationship types from variables can significantly streamline the process of querying and updating graph data. It allows for greater abstraction, enabling users to write more generic and reusable queries. This is particularly beneficial in scenarios where the relationship types may not be known in advance or where the database schema may evolve over time. By leveraging this feature, developers can maintain cleaner code and improve the maintainability of their applications.
Moreover, understanding how to effectively implement variable relationship types can lead to improved performance and efficiency in graph operations. As relationships are a core component of graph databases, optimizing their definition and manipulation can result in faster query execution times and reduced complexity in data handling. Overall, mastering this aspect of Neo4j empowers developers to unlock the full potential of graph databases, facilitating more robust and adaptable data-driven applications.
Author Profile
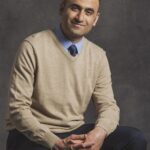
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?