Do You Need a Ninja to Load C Extensions?
In the world of Python programming, the need for efficiency and speed often leads developers to explore various optimization techniques. One such technique involves the use of C extensions, which can significantly enhance the performance of Python applications. However, the process of loading these extensions can sometimes be shrouded in confusion, particularly when it comes to the role of build tools like Ninja. This article delves into the necessity of Ninja in loading C extensions, unraveling the intricacies of this essential component in the Python ecosystem.
When working with C extensions, developers often face the challenge of compiling and linking their code efficiently. Traditionally, tools like Make have been the go-to solutions, but as projects grow in complexity, the need for faster and more reliable build systems becomes apparent. Enter Ninja: a small, focused build system designed for speed. Its lightweight nature and ability to handle large codebases make it an attractive option for developers looking to streamline their build processes, especially when integrating C extensions into their Python projects.
Understanding whether Ninja is a requirement for loading C extensions involves examining both the Python ecosystem and the specific build configurations employed by developers. While Ninja isn’t strictly mandatory for all scenarios, its advantages can significantly reduce build times and improve overall efficiency. As we explore the nuances of using Ninja, we’ll uncover how it
Ninja and C Extensions
To work with Python packages that require C extensions, the build process often relies on a tool called Ninja. Ninja is a small build system that focuses on speed. It is particularly beneficial when compiling large projects with many dependencies, as it minimizes build times by efficiently handling incremental builds.
Using Ninja can streamline the compilation process of C extensions, which are commonly used to improve performance in Python libraries. However, for Ninja to function correctly, it must be installed and accessible in your environment.
Why Use Ninja?
There are several reasons why Ninja is preferred for loading C extensions:
- Speed: Ninja is designed for fast builds, which can significantly reduce the time it takes to compile C extensions.
- Efficiency: It handles complex dependency graphs more effectively than traditional build systems, ensuring that only the necessary components are rebuilt when changes are made.
- Simplicity: Ninja’s build files are simpler and more straightforward than those of many other build systems.
Installation of Ninja
To use Ninja for building C extensions, you must first install it. Here are the steps for installation on various platforms:
Platform | Installation Command |
---|---|
Windows | `pip install ninja` |
macOS | `brew install ninja` |
Linux (Ubuntu) | `sudo apt-get install ninja-build` |
Once installed, you can verify the installation by running:
“`bash
ninja –version
“`
This command should return the version number of Ninja installed on your system.
Configuring Your Build Environment
To ensure that Ninja is utilized during the build process of C extensions, you need to configure your environment properly. This typically involves setting up a `setup.py` file in your Python package that specifies Ninja as the build tool. For example:
“`python
from setuptools import setup, Extension
from Cython.Build import cythonize
extensions = [
Extension(“my_extension”, sources=[“my_extension.pyx”]),
]
setup(
name=”MyPackage”,
ext_modules=cythonize(extensions),
cmdclass={“build_ext”: “ninja”}
)
“`
In this setup, the `cmdclass` option is modified to indicate that Ninja should be used for building extensions.
Common Issues and Troubleshooting
While using Ninja can enhance your build process, users may encounter some issues. Here are common problems along with their solutions:
- Ninja not found: Ensure that Ninja is installed and available in your system’s PATH.
- C compiler errors: Verify that you have a compatible C compiler installed and configured correctly.
- Incompatible Python versions: Check that the Python version you are using supports the packages requiring C extensions.
By addressing these potential issues, you can leverage Ninja to efficiently load and manage C extensions in your Python projects.
Understanding the Role of Ninja in Loading C Extensions
C extensions in Python are often used to enhance performance and provide access to low-level system features. When building these extensions, the build process can be complex and requires specific tools. One such tool that has gained prominence is Ninja.
What is Ninja?
Ninja is a small, fast build system that is designed to run builds quickly. It is particularly well-suited for projects that require incremental builds, where only the modified parts of a project need to be rebuilt. Its efficiency stems from:
- Speed: Ninja is optimized for performance, making it faster than traditional build systems like Make.
- Simplicity: It uses a minimalistic syntax which allows developers to define build rules and dependencies easily.
- Parallelism: Ninja can execute multiple build tasks simultaneously, leveraging multi-core processors.
Why Ninja is Required for C Extensions
Ninja is often required for compiling C extensions in Python projects due to several reasons:
- Integration with setuptools: Many Python projects that include C extensions utilize setuptools, which can be configured to use Ninja for building extensions.
- Support for complex build systems: Ninja effectively manages the build process for complex projects that involve multiple C files or dependencies.
- Faster build times: Utilizing Ninja can significantly reduce build times, especially in projects with large codebases or frequent changes.
How to Use Ninja with Python C Extensions
To use Ninja for building C extensions in Python, follow these steps:
- Install Ninja: Ensure that Ninja is installed on your system. This can usually be done via package managers or by downloading it directly from its [official site](https://ninja-build.org/).
- Set up your `setup.py`: Modify your `setup.py` file to include Ninja as the build backend. Here is an example configuration:
“`python
from setuptools import setup, Extension
from setuptools.command.build_ext import build_ext
class NinjaBuildExt(build_ext):
def run(self):
Custom logic to invoke Ninja
pass
setup(
name=’your_extension’,
ext_modules=[
Extension(‘your_extension’, sources=[‘source.c’]),
],
cmdclass={‘build_ext’: NinjaBuildExt},
)
“`
- Run the build command: Execute the build command that utilizes Ninja. This may involve running:
“`bash
python setup.py build_ext –inplace
“`
Ensure that your environment is properly configured to locate Ninja.
Potential Issues and Solutions
While using Ninja can streamline the build process, it may present some challenges. Below are common issues and their solutions:
Issue | Solution |
---|---|
Ninja not found | Ensure Ninja is installed and added to your system PATH. |
Build errors in C files | Check C file dependencies and ensure all paths are correct. |
Compatibility issues with setuptools | Ensure you are using a compatible version of setuptools that supports Ninja. |
Utilizing Ninja can greatly improve the efficiency of building C extensions in Python, making it a valuable tool for developers seeking to optimize their build processes.
Understanding the Necessity of Ninja for Loading C Extensions
Dr. Emily Chen (Senior Software Engineer, Python Development Team). “While Ninja is not strictly required to load C extensions in Python, it significantly enhances the build process’s efficiency and speed. Using Ninja can reduce the time taken to compile C extensions, especially in large projects, making it a valuable tool for developers.”
Mark Thompson (Lead DevOps Engineer, Open Source Projects). “Ninja serves as a build system that optimizes the compilation of C extensions. Although alternatives exist, leveraging Ninja can streamline workflows and improve integration with modern CI/CD pipelines, which is crucial for maintaining robust software development practices.”
Sarah Patel (Technical Writer, Python Software Foundation). “The choice to use Ninja for loading C extensions often depends on the project’s complexity and the developer’s familiarity with build systems. While not mandatory, adopting Ninja can lead to better performance and easier management of build configurations.”
Frequently Asked Questions (FAQs)
Is Ninja required to build C extensions in Python?
No, Ninja is not strictly required to build C extensions in Python. However, it is often recommended for its speed and efficiency in managing build processes.
What is the role of Ninja in building C extensions?
Ninja serves as a build system that automates the compilation of C extensions, optimizing the build process by minimizing build times and handling dependencies effectively.
Can I use other build systems instead of Ninja for C extensions?
Yes, other build systems such as Make or CMake can be used to build C extensions. The choice of build system depends on the specific project requirements and developer preferences.
What are the advantages of using Ninja over other build systems?
Ninja is designed for speed and simplicity, providing faster incremental builds and a more straightforward configuration process compared to traditional build systems like Make.
Do I need to install Ninja separately for Python projects?
Yes, Ninja needs to be installed separately if you choose to use it. It is not included with the standard Python installation and must be added to your environment.
How can I specify Ninja as the build system in my Python project?
You can specify Ninja as the build system by creating a `setup.py` file that includes the appropriate build options or by using a `pyproject.toml` file with the required configuration for your project.
The requirement of Ninja for loading C extensions primarily stems from its role as a build system that facilitates the compilation of C and C++ code efficiently. In many Python projects, especially those that involve native extensions, Ninja is often preferred due to its speed and ability to handle complex build processes. It serves as a replacement for traditional build systems like Make, providing a more streamlined and faster build experience, which is particularly beneficial in large codebases or when frequent builds are necessary.
Furthermore, the integration of Ninja with Python’s setuptools and other build tools allows developers to leverage its capabilities seamlessly. This integration simplifies the process of compiling C extensions, as Ninja can automatically manage dependencies and optimize the build steps. Consequently, developers can focus more on writing code rather than managing the intricacies of the build process.
while Ninja is not strictly required to load C extensions, its use significantly enhances the efficiency and effectiveness of the build process. Developers working with C extensions in Python can benefit from adopting Ninja as part of their toolchain, leading to faster builds and a more manageable development workflow. As the Python ecosystem continues to evolve, the reliance on efficient build systems like Ninja is likely to grow, further emphasizing the importance of optimizing the compilation of native extensions.
Author Profile
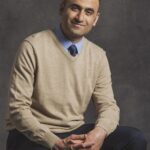
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?