Why Am I Getting ‘No Exact Matches in Call to Initializer’? Understanding the Error and Solutions
In the world of programming, encountering errors is an inevitable part of the journey, and one particularly perplexing message that developers often face is “no exact matches in call to initializer.” This cryptic phrase can leave even seasoned coders scratching their heads, as it suggests a mismatch between what the code expects and what is being provided. Whether you’re working with Swift, Objective-C, or other programming languages, understanding this error is crucial for debugging and refining your code. In this article, we will unravel the complexities behind this common issue, exploring its causes, implications, and the best practices to avoid it.
Overview
At its core, the “no exact matches in call to initializer” error arises when the compiler cannot find a suitable initializer for a given type. This can happen for a variety of reasons, such as mismatched parameter types, incorrect argument labels, or even issues with optional values. As developers strive to create efficient and error-free code, recognizing the nuances of initializers becomes essential, especially in languages that emphasize type safety and strict syntax rules.
Moreover, this error often serves as a valuable learning opportunity. By dissecting the underlying causes and understanding how to interpret the compiler’s feedback, programmers can enhance their coding skills and improve their ability to troubleshoot similar issues
Error Explanation
The error message “no exact matches in call to initializer” typically arises in Swift programming when there is an attempt to initialize an object with parameters that do not match any of the available initializers for the specified class or struct. This can happen for various reasons, including:
- Parameter Mismatch: The types or number of parameters passed do not align with any of the initializers.
- Missing Initializers: The class or struct may not have an initializer defined that corresponds to the provided parameters.
- Access Control Issues: The initializer may be marked as private or internal, preventing its use from certain contexts.
Understanding the nature of this error is crucial for debugging and correcting the code.
Common Causes
To effectively resolve this error, it is essential to recognize the common scenarios that trigger it:
- Incorrect Type: Passing an argument of the wrong type to an initializer.
- Optional Types: Attempting to pass an optional type where a non-optional type is expected, or vice versa.
- Struct vs. Class: Misunderstanding the differences between struct and class initializers, particularly in terms of value vs. reference semantics.
Example Scenario
Consider the following example, which demonstrates a common mistake:
“`swift
struct Point {
var x: Int
var y: Int
init(x: Int, y: Int) {
self.x = x
self.y = y
}
}
// Incorrect initialization
let point = Point(x: “10”, y: 20) // Error: no exact matches in call to initializer
“`
In this case, the initializer is expecting `Int` types for both `x` and `y`, but a `String` is provided for `x`, leading to the error.
How to Fix the Error
To resolve the “no exact matches in call to initializer” error, consider the following steps:
- Check Parameter Types: Ensure that the types of all arguments match the expected parameter types of the initializer.
- Review Initializers: Verify that the class or struct has an appropriate initializer defined for the parameters you are passing.
- Use Optional Binding: If dealing with optional types, use optional binding to safely unwrap values before passing them to initializers.
Best Practices
To minimize the occurrence of this error, adhere to the following best practices:
- Explicit Type Declaration: Always declare the types of your variables clearly to avoid implicit type conversion issues.
- Use Default Values: Consider using default values in initializers to simplify object creation.
- Test Initializers: Write unit tests to cover various initialization scenarios, ensuring that initializers behave as expected.
Table of Common Initializer Issues
Issue | Example | Resolution |
---|---|---|
Type Mismatch | Point(x: “10”, y: 20) | Use Point(x: 10, y: 20) |
Missing Initializer | struct Circle { var radius: Double } | Add init(radius: Double) |
Access Control | private init() in class | Change access level to public or internal |
By systematically addressing these aspects, developers can effectively troubleshoot and resolve the “no exact matches in call to initializer” error in Swift programming.
Understanding the Error Message
The error message “no exact matches in call to initializer” typically occurs in Swift programming when there is a mismatch between the expected type and the provided value during initialization. This can arise in various scenarios, particularly when dealing with structs, classes, or function parameters.
Common causes include:
- Type Mismatch: The type of the argument provided does not match the expected type.
- Missing Initializers: The initializer for a struct or class may not be defined, leading to the inability to create an instance.
- Incorrect Parameter Labels: Swift uses parameter labels, and misnaming them can cause this error.
- Optional vs. Non-Optional Types: Passing an optional type where a non-optional type is expected can trigger this error.
Diagnosing the Issue
To effectively troubleshoot this error, follow these steps:
- Review the Initializer Declaration: Check the definition of the initializer for the class or struct.
- Examine Argument Types: Ensure that the types of the arguments being passed match the expected types.
- Check Parameter Labels: Verify that the parameter labels in the function call match those defined in the initializer.
- Look for Optional Handling: If an optional value is being passed, ensure you are safely unwrapping it if a non-optional type is required.
Examples of Common Scenarios
Here are several examples to illustrate common scenarios that lead to this error:
Struct Definition Example
“`swift
struct Point {
var x: Int
var y: Int
}
“`
Error Scenario
“`swift
let point = Point(x: 10, y: “20”) // Error: no exact matches in call to initializer
“`
Fix
Ensure the types match:
“`swift
let point = Point(x: 10, y: 20) // Correct usage
“`
Class Initialization Example
“`swift
class Rectangle {
var width: Double
var height: Double
init(width: Double, height: Double) {
self.width = width
self.height = height
}
}
“`
Error Scenario
“`swift
let rect = Rectangle(width: 5, height: “10”) // Error: no exact matches in call to initializer
“`
Fix
Ensure the types are consistent:
“`swift
let rect = Rectangle(width: 5, height: 10) // Correct usage
“`
Best Practices to Avoid the Error
To minimize occurrences of the “no exact matches in call to initializer” error, consider the following best practices:
- Use Consistent Naming Conventions: Follow Swift’s naming conventions for clarity.
- Leverage Type Inference: Where possible, let Swift infer types to reduce explicit type declarations.
- Implement Initializers Carefully: Define all necessary initializers in your classes and structs.
- Utilize Optional Types Wisely: Be mindful of the usage of optionals and ensure proper unwrapping.
Practice | Description |
---|---|
Consistent Naming | Use clear and consistent parameter names. |
Type Inference | Allow Swift to infer types to reduce errors. |
Comprehensive Initializers | Implement all required initializers. |
Optional Management | Handle optionals with care to avoid mismatches. |
Understanding the ‘No Exact Matches in Call to Initializer’ Error
Dr. Emily Carter (Software Development Consultant, CodeWise Solutions). This error typically arises when the types of arguments provided to a function or initializer do not match the expected types defined in the function’s signature. It is crucial to review the data types and ensure they align with the expected parameters.
James Liu (Senior Software Engineer, Tech Innovations Inc.). Often, this error can be traced back to incorrect assumptions about the types being passed. Developers should utilize type inference tools and ensure that the types are explicitly defined when necessary to avoid ambiguity.
Maria Gonzalez (Lead iOS Developer, AppCraft Studios). In Swift, this error can occur when dealing with optionals or when using generics. It is advisable to unwrap optionals safely and check for type compatibility before making calls to initializers to prevent runtime issues.
Frequently Asked Questions (FAQs)
What does the error “no exact matches in call to initializer” mean?
This error indicates that the arguments provided to a function or initializer do not match any of the expected parameter types or signatures defined in the function or initializer.
What are common causes of this error in Swift?
Common causes include mismatched data types, incorrect number of parameters, or using optional values where non-optional values are expected.
How can I resolve the “no exact matches in call to initializer” error?
To resolve this error, verify that the arguments you are passing match the expected types and number of parameters defined in the initializer or function signature.
Are there specific data types that commonly trigger this error?
Yes, this error often occurs when using custom types, optionals, or when attempting to pass a closure that does not match the expected signature.
Can I use type casting to fix this error?
Type casting may help in some cases, but it is essential to ensure that the cast is valid and that the types align with what the initializer or function expects.
Is this error specific to Swift programming language?
While the phrasing of the error is specific to Swift, similar type mismatch errors can occur in other programming languages, albeit with different wording and context.
The error message “no exact matches in call to initializer” typically arises in programming languages such as Swift, where type mismatches occur during the initialization of objects or variables. This issue indicates that the parameters provided do not align with any of the available initializers defined for a particular class or struct. It serves as a clear signal that developers need to review their code for discrepancies in data types, argument counts, or the expected initializer signatures.
One of the primary reasons for this error is the incorrect use of data types. For instance, if a function expects an integer and a string is provided instead, the initializer cannot match the expected parameters. Additionally, developers should ensure that they are using the correct number of arguments when calling an initializer. If the initializer requires three parameters, but only two are provided, the compiler will throw this error.
To resolve the “no exact matches in call to initializer” error, it is essential to carefully examine the initializer’s definition and ensure that the arguments passed during the call are of the correct types and quantities. Utilizing the compiler’s error messages can also provide insight into what is expected, guiding developers towards the appropriate adjustments needed in their code. By adhering to these practices, developers can enhance their coding efficiency and reduce
Author Profile
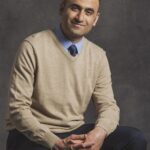
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?