How Can I Resolve the ‘No Main Manifest Attribute in JAR’ Error?
When working with Java applications, encountering the error message “no main manifest attribute in jar” can be a frustrating roadblock for developers. This seemingly cryptic message often appears when trying to execute a JAR file that lacks the necessary configuration to identify the starting point of the application. As Java developers know, the manifest file plays a crucial role in defining the structure and behavior of a JAR, including specifying the main class that should be executed. Understanding this error is essential for anyone looking to streamline their Java development process and ensure their applications run smoothly.
At its core, the “no main manifest attribute” error indicates that the Java Runtime Environment (JRE) cannot find the entry point of the application within the JAR file. This typically occurs when the manifest file, which is a special file within the JAR, does not include the `Main-Class` attribute. Without this attribute, the JRE is left in the dark about which class to execute, leading to confusion and halted progress. Developers may encounter this issue during various stages of their project, whether they are packaging their code for distribution or simply trying to run a local build.
To resolve this error, it is essential to understand how to properly configure the manifest file during the JAR creation process. By ensuring that the
Understanding the Manifest File
A Java Archive (JAR) file is a package that typically contains Java classes, metadata, and resources. One crucial component of a JAR file is the manifest file, located in the `META-INF` directory. The manifest file, named `MANIFEST.MF`, provides essential information about the JAR file, including versioning and entry points for execution.
To run a JAR file directly using the command line, it must specify a main class in the manifest file. The absence of the main class declaration leads to the error “no main manifest attribute in jar.” This error indicates that the Java Runtime Environment (JRE) cannot find the entry point for execution.
Creating a Manifest File
To resolve the “no main manifest attribute in jar” error, you must ensure that your JAR file includes a properly configured manifest file. Here’s how to create one:
- Create a Manifest File: Open a text editor and create a file named `MANIFEST.MF`.
- Specify Main Class: Include the following lines in the manifest file, replacing `YourMainClass` with the fully qualified name of your main class.
“`
Manifest-Version: 1.0
Main-Class: YourMainClass
“`
- Ensure Proper Formatting: Make sure there is a newline at the end of the manifest file. This is crucial for the file to be recognized correctly.
Packing the JAR File with Manifest
When you create a JAR file, you can include the manifest file using the `jar` command. Here’s the syntax:
“`
jar cfm YourJarFile.jar MANIFEST.MF -C path/to/classes .
“`
Where:
- `c` creates a new archive.
- `f` specifies the archive file name.
- `m` indicates that a manifest file should be included.
- `-C` changes to the specified directory before adding files.
Common Issues and Solutions
Below are common issues associated with the manifest file and their solutions:
Issue | Solution |
---|---|
Missing Main-Class entry | Add `Main-Class: YourMainClass` in the manifest file. |
No newline at EOF | Add a newline character at the end of the manifest file. |
Incorrect classpath | Ensure all dependencies are included in the JAR or specified in the manifest. |
Wrong package structure | Verify that the path to the main class matches the package structure. |
By addressing these common issues, you can successfully package your Java application into a JAR file with a working manifest.
Understanding the Main Manifest Attribute
In Java, a JAR (Java ARchive) file is a package file format that aggregates multiple files into one. It often includes Java classes and associated metadata. The main manifest attribute is essential for defining the entry point of a Java application within a JAR file. This attribute specifies the class that contains the `main` method to be executed when the JAR is run.
The main manifest attribute is defined in the `MANIFEST.MF` file, which is located in the `META-INF` directory of the JAR. The typical format for specifying the main class looks like this:
“`
Main-Class: com.example.MainClass
“`
Causes of “No Main Manifest Attribute” Error
The error message “no main manifest attribute in jar” indicates that the Java Runtime Environment (JRE) cannot find the entry point for execution. This issue can arise due to several reasons:
- Missing Manifest File: The `MANIFEST.MF` file may not be present in the JAR.
- Incorrect Manifest Format: The format of the `MANIFEST.MF` file may be incorrect or improperly structured.
- Omitted Main-Class Entry: The `Main-Class` entry may be missing or misspelled.
- Packaging Errors: Issues during the packaging process can lead to a corrupted JAR file.
How to Resolve the Error
To fix the “no main manifest attribute in jar” error, consider the following steps:
- Check the JAR File: Use the following command to list the contents and verify if the `META-INF/MANIFEST.MF` file exists:
“`bash
jar tf yourfile.jar
“`
- Inspect the Manifest File: Open the `MANIFEST.MF` file and ensure it contains the `Main-Class` attribute:
“`plaintext
Main-Class: com.example.MainClass
“`
- Recreate the JAR File: If the manifest file is missing or incorrect, recreate the JAR file with the proper manifest. Use the following command:
“`bash
jar cfm yourfile.jar MANIFEST.MF -C your/classes/directory .
“`
- Use Correct Line Endings: Ensure that the `MANIFEST.MF` file uses the correct line endings (LF or CRLF) as required by the operating system.
- Verify Classpath: If your application depends on other libraries, ensure that the classpath is correctly set in the manifest file.
Example of a Correct Manifest File
Here is an example of a correctly structured `MANIFEST.MF` file:
“`
Manifest-Version: 1.0
Main-Class: com.example.MainClass
Class-Path: lib/dependency1.jar lib/dependency2.jar
“`
Ensure that there is a newline at the end of the file, as this is necessary for the JAR to read the attributes correctly.
Testing the JAR File
Once you have ensured that the manifest file is correct, test the JAR file by executing it with the following command:
“`bash
java -jar yourfile.jar
“`
If the setup is correct, the application should run without displaying the “no main manifest attribute in jar” error.
Understanding the ‘No Main Manifest Attribute in Jar’ Error
Dr. Emily Carter (Software Development Specialist, Tech Innovations Inc.). “The ‘no main manifest attribute in jar’ error typically arises when the JAR file does not specify an entry point for execution. This can be resolved by ensuring that the JAR’s manifest file includes the ‘Main-Class’ attribute, which designates the main class to be executed.”
Michael Chen (Java Architect, CodeCraft Solutions). “Many developers overlook the importance of the manifest file when packaging their applications. To avoid this error, it is crucial to configure the build process correctly, ensuring that the manifest file is generated with the required attributes during JAR creation.”
Sarah Thompson (Senior DevOps Engineer, CloudTech Systems). “Incorporating automated build tools can significantly reduce the chances of encountering the ‘no main manifest attribute in jar’ error. Tools like Maven or Gradle can automatically handle manifest generation, ensuring that the necessary attributes are included without manual intervention.”
Frequently Asked Questions (FAQs)
What does “no main manifest attribute in jar” mean?
The error “no main manifest attribute in jar” indicates that the Java Archive (JAR) file lacks a specified entry point. This means the JAR does not contain a `Main-Class` attribute in its manifest file, which is necessary for the Java Virtual Machine (JVM) to know which class to execute.
How can I fix the “no main manifest attribute in jar” error?
To resolve this error, you need to ensure that your JAR file includes a manifest file with the `Main-Class` attribute defined. You can create or modify the manifest file and then repackage the JAR using the `jar` command with the `m` option to include the updated manifest.
What is a manifest file in a JAR?
A manifest file is a special file within a JAR that contains metadata about the JAR file, including information about the classes it contains and the entry point for execution. It is typically located in the `META-INF` directory and named `MANIFEST.MF`.
How do I create a manifest file with a main class?
To create a manifest file with a main class, create a text file named `MANIFEST.MF` and include the line `Main-Class: YourMainClassName` (replace `YourMainClassName` with the actual class name). Ensure there is a newline at the end of the file, then package it with your JAR using the `jar` command.
Can I run a JAR without a main class specified?
You cannot run a JAR file without a main class specified in its manifest. The JVM requires the `Main-Class` attribute to determine which class contains the `main` method to start execution.
What tools can I use to inspect the contents of a JAR file?
You can use the `jar` command-line tool, which comes with the JDK, to inspect the contents of a JAR file. Additionally, tools like `unzip` or archive managers can also be used to explore the JAR file structure, including the manifest file.
The error message “no main manifest attribute in jar” typically indicates that the Java Archive (JAR) file being executed does not contain a specified entry point for the Java Virtual Machine (JVM). This entry point is defined in the JAR’s manifest file, specifically through the `Main-Class` attribute. Without this attribute, the JVM lacks the necessary information to identify which class to execute, resulting in the error when attempting to run the JAR file.
To resolve this issue, developers must ensure that the JAR file is packaged correctly. This includes creating a manifest file that specifies the `Main-Class` attribute, which points to the class containing the `main` method. Properly configuring the build process, whether through command line tools or integrated development environments (IDEs), is essential to avoid this common pitfall. Additionally, verifying the structure of the JAR file can help confirm that the manifest is included and correctly formatted.
Key takeaways from this discussion emphasize the importance of the manifest file in JAR packaging. Developers should pay close attention to the build configuration and ensure that the necessary attributes are included. Furthermore, understanding the structure of JAR files and the role of the manifest can prevent runtime errors and streamline the execution of
Author Profile
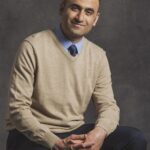
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?