Why Am I Seeing ‘No Process is on the Other End of the Pipe’ and How Can I Fix It?
In the intricate world of computing, communication between processes is vital for executing tasks efficiently. However, developers and system administrators often encounter the perplexing error message: “no process is on the other end of the pipe.” This seemingly cryptic phrase can signal a range of issues related to inter-process communication, particularly when using pipes—a fundamental mechanism for data exchange in many operating systems. Understanding this error is crucial for anyone working with software that relies on process interaction, as it can lead to frustrating roadblocks in development and deployment.
At its core, the “no process is on the other end of the pipe” error indicates a breakdown in communication between two processes that are meant to share data through a pipe. Pipes serve as conduits for data, allowing one process to send output that another can read. When this error occurs, it typically means that one of the processes has terminated unexpectedly or that there is a misconfiguration in how the pipe was set up. This can happen in various scenarios, such as when a parent process tries to read from a pipe after its child process has already exited.
Delving deeper into this topic reveals the underlying mechanics of pipes and the common pitfalls that can lead to this error. By exploring the causes and potential solutions, developers can enhance their understanding of process management
Understanding the Error
The error message “no process is on the other end of the pipe” typically occurs in programming and system operations when a communication channel between two processes is disrupted. This can happen in various contexts, such as when using inter-process communication (IPC) mechanisms, where data is transmitted between different processes.
This error is indicative of a failure in the expected communication flow. When one end of the pipe (which can be a named or unnamed pipe) attempts to send or receive data, it expects the other end to be active and available. If the receiving process has terminated or is not listening, the error is triggered.
Common Causes
Several factors can lead to this error:
- Process Termination: If the receiving process has exited unexpectedly, the sending process will encounter this error.
- Incorrect Pipe Handling: Mismanagement of pipes, such as not closing them properly or attempting to write to a pipe that is no longer open, can result in this issue.
- Timing Issues: Situations where the sender and receiver are not properly synchronized can lead to one end being inactive when the other tries to communicate.
- Resource Limits: Hitting system resource limits, such as the maximum number of open file descriptors, can also trigger this error.
Troubleshooting Steps
To address the “no process is on the other end of the pipe” error, consider the following troubleshooting steps:
- Check Process Status: Ensure that the intended receiving process is still running and has not crashed or been terminated.
- Inspect Pipe Creation: Verify that the pipes are created correctly and that both ends are set up to read and write as intended.
- Review Code Logic: Examine the logic of your application to ensure proper synchronization between processes.
- Monitor Resource Usage: Use system monitoring tools to check for resource limits and ensure that your application isn’t exceeding them.
Example Scenario
Here’s a simple example of how this error might occur in a UNIX-like environment:
“`bash
Process A
echo “Hello, World!” > pipe.txt &
Process B
cat pipe.txt
“`
If Process A finishes and closes the pipe before Process B attempts to read from it, Process B will encounter the error.
Mitigation Strategies
To prevent this error from occurring, consider implementing the following strategies:
- Error Handling: Incorporate robust error handling in your code to gracefully manage communication failures.
- Keep Alive Signals: Use heartbeat or keep-alive signals to ensure that both processes are still active.
- Retry Logic: Implement retry mechanisms for communication attempts, allowing for transient failures to be handled smoothly.
Cause | Description |
---|---|
Process Termination | The receiving process has exited unexpectedly. |
Incorrect Pipe Handling | Improper management of pipe resources. |
Timing Issues | Desynchronization between sender and receiver. |
Resource Limits | Exceeding the maximum number of allowed open pipes. |
Understanding the Error Message
The error message “no process is on the other end of the pipe” typically arises in computing contexts, particularly when dealing with inter-process communication (IPC). This error indicates that one end of a pipe, a mechanism for passing data between processes, is closed or not available.
Key Points:
- Pipe Mechanism: Pipes are used for IPC, allowing data to flow from one process to another.
- Error Context: This error often occurs in scenarios where a process tries to read from or write to a pipe that has been closed by the other end.
- Common Scenarios:
- A child process terminates unexpectedly.
- A program attempts to communicate after the other end has been closed.
Troubleshooting Steps
When encountering the “no process is on the other end of the pipe” error, the following troubleshooting steps can be applied:
- Check Process Status:
- Ensure that the process on the other end of the pipe is still running.
- Use system monitoring tools (e.g., Task Manager, Activity Monitor) to confirm process status.
- Review Code Logic:
- Inspect the logic flow of your application to ensure that pipes are being opened and closed correctly.
- Validate that data is being sent before the receiving process attempts to read from the pipe.
- Handle Exceptions:
- Implement error handling in your code to gracefully manage situations where the pipe might be closed unexpectedly.
- Use try-catch blocks to manage read/write operations.
Common Causes of the Error
Identifying the root cause of the error can assist in implementing effective solutions. Below are common causes:
Cause | Description |
---|---|
Process Termination | The writing or reading process has terminated prematurely. |
Incorrect Pipe Configuration | Pipes not correctly set up or permissions not granted. |
Timing Issues | Race conditions where one process closes the pipe too early. |
Resource Limitations | System resources exhausted, leading to unexpected behavior. |
Preventive Measures
To mitigate the risk of encountering this error in the future, consider the following preventive measures:
- Proper Resource Management:
- Ensure that all processes are designed to handle pipe communication efficiently.
- Use of Signal Handling:
- Implement signal handling to manage the closure of processes and notify other components accordingly.
- Testing and Debugging:
- Conduct thorough testing, particularly around IPC operations, to catch errors before deployment.
- Utilize debugging tools to monitor the state of processes and pipes during execution.
Best Practices for IPC with Pipes
To enhance the reliability of inter-process communication via pipes, adhere to the following best practices:
- Establish Clear Protocols:
- Define clear communication protocols between processes to ensure that both sides know when to read and write.
- Timeouts and Retries:
- Implement timeouts and retry mechanisms to handle transient issues when reading from or writing to pipes.
- Documentation:
- Maintain comprehensive documentation of the IPC mechanisms used, including expected behaviors and error handling strategies.
By following these guidelines, developers can minimize the occurrence of the “no process is on the other end of the pipe” error and improve the robustness of their applications.
Understanding the “No Process is on the Other End of the Pipe” Error
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The error message ‘no process is on the other end of the pipe’ typically indicates that a communication channel, such as a pipe or socket, has been closed unexpectedly. This often occurs in inter-process communication when one end of the pipe terminates before the other, leading to an inability to send or receive data.”
Mark Thompson (Systems Architect, Cloud Solutions Group). “In my experience, this error can arise during application development when a child process is expected to read data from a parent process, but the parent has already exited. Proper error handling and ensuring that processes are synchronized can mitigate this issue significantly.”
Linda Zhang (DevOps Specialist, AgileTech Solutions). “The ‘no process is on the other end of the pipe’ error is a common pitfall in containerized environments. It is crucial to monitor the lifecycle of processes and ensure that the dependencies are correctly managed. Implementing health checks can help avoid abrupt terminations that lead to this error.”
Frequently Asked Questions (FAQs)
What does the error “no process is on the other end of the pipe” indicate?
This error typically indicates that a program is attempting to communicate with another process through a pipe, but the receiving end of the pipe is not available or has been closed.
What causes the “no process is on the other end of the pipe” error?
The error can occur due to various reasons, including the termination of the receiving process, improper handling of pipe connections, or network issues in client-server applications.
How can I troubleshoot the “no process is on the other end of the pipe” error?
To troubleshoot, verify that both processes are running, check for proper error handling in your code, and ensure that the pipe is correctly established and not prematurely closed.
Is this error specific to a certain programming language?
No, this error can occur in various programming languages and environments that utilize inter-process communication (IPC) through pipes, including C, Python, and Java.
Can this error be resolved by restarting the application?
Restarting the application may temporarily resolve the issue by re-establishing the pipe connection; however, it is essential to identify and fix the underlying cause to prevent recurrence.
Are there any best practices to avoid the “no process is on the other end of the pipe” error?
Yes, best practices include implementing robust error handling, ensuring proper synchronization between processes, and regularly monitoring the status of all processes involved in communication.
The phrase “no process is on the other end of the pipe” typically refers to a situation in computing and programming where a process attempts to communicate through a pipe, but there is no receiving process available to handle the data. This can occur in various scenarios, such as when a program is designed to send output to another program’s input, but the receiving program has not been initiated or has terminated unexpectedly. Understanding this concept is crucial for developers and system administrators, as it can lead to errors and inefficiencies in data processing and inter-process communication.
One of the key takeaways from the discussion surrounding this issue is the importance of robust error handling in applications that rely on inter-process communication. Developers should implement checks to ensure that the receiving process is active before attempting to send data. This can prevent runtime errors and improve the overall reliability of the system. Additionally, logging mechanisms can be beneficial for diagnosing issues related to inter-process communication, allowing developers to trace back the source of the problem when it occurs.
Moreover, it is essential to consider the lifecycle of processes when designing applications that utilize pipes. Proper management of process creation and termination can help mitigate the risks associated with “no process is on the other end of the pipe.” Techniques such as using
Author Profile
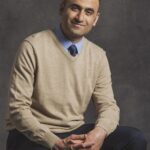
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?