Why Am I Seeing ‘No QueryClient Set’? How to Use QueryClientProvider to Fix This!
In the ever-evolving landscape of web development, managing state and data fetching efficiently is crucial for building responsive and performant applications. As developers increasingly turn to libraries like React Query for their data management needs, understanding the nuances of configuration becomes paramount. One common question that arises is: “What does it mean to have no QueryClient set, and how can I use QueryClientProvider to establish one?” This inquiry not only highlights the importance of proper setup but also opens the door to a deeper exploration of how these tools can enhance your application’s performance and user experience.
At its core, the QueryClient serves as the backbone of React Query, enabling developers to manage server state seamlessly. When you encounter a scenario where no QueryClient is set, it can lead to unexpected behaviors and hinder your ability to fetch and cache data effectively. This is where the QueryClientProvider comes into play, acting as a vital component that wraps your application and provides the necessary context for the QueryClient. By understanding how to implement this provider, developers can unlock the full potential of React Query, ensuring that their applications are not only functional but also optimized for speed and reliability.
As we delve deeper into this topic, we will explore the significance of the QueryClient, the role of the QueryClientProvider, and best practices
Understanding QueryClient and QueryClientProvider
The QueryClient and QueryClientProvider are core components in the React Query library, which facilitates data fetching, caching, and synchronization in React applications. The QueryClient serves as the central configuration object, managing the cache and the state of your data fetching. Conversely, the QueryClientProvider acts as a context provider, making the QueryClient accessible throughout your component tree.
To properly set up your application, you typically initialize a QueryClient instance and wrap your application with QueryClientProvider. This ensures that all nested components have access to the QueryClient and can utilize its functionalities.
Setting Up QueryClient
To create a QueryClient, you simply instantiate it in your application’s entry point. The following example demonstrates how to do this:
“`javascript
import { QueryClient } from ‘react-query’;
const queryClient = new QueryClient();
“`
This instance can then be passed to the QueryClientProvider, as shown below:
“`javascript
import { QueryClientProvider } from ‘react-query’;
function App() {
return (
{/* Your application components */}
);
}
“`
Benefits of Using QueryClientProvider
Utilizing QueryClientProvider provides several advantages:
- Centralized Data Management: It maintains a single source of truth for all data fetching logic and cache.
- Global Configuration: You can configure global defaults for queries and mutations, such as stale times, retries, and refetch intervals.
- Improved Performance: By managing caching effectively, it minimizes unnecessary network requests and enhances application responsiveness.
Configuration Options
The QueryClient can be configured with various options to customize its behavior. Here is a table summarizing some common configuration options:
Option | Description | Default Value |
---|---|---|
defaultOptions | Sets default options for queries and mutations. | { queries: { staleTime: 0 }, mutations: { onError: () => {} } } |
logger | Custom logger for logging query events. | Console log |
queryCache | Custom cache object for storing query results. | Default cache |
By adjusting these options, developers can fine-tune how their application interacts with the server and manages data locally, leading to a more efficient user experience.
Conclusion on Setting Use QueryClientProvider
The use of QueryClient and QueryClientProvider is integral to effectively managing server state in React applications. By following the setup and configuration guidelines, developers can ensure that their applications are robust, maintainable, and performant. The QueryClientProvider not only simplifies access to query functionalities but also enhances the overall architecture of data management within the application.
Understanding QueryClient and QueryClientProvider
In React Query, `QueryClient` and `QueryClientProvider` are integral components for managing server state and caching. Here’s a closer examination of their functionality:
- QueryClient: This is the core object that manages the cache for your queries. It holds configurations and provides methods to interact with data fetching.
- QueryClientProvider: This component wraps your application and provides the `QueryClient` instance to all child components, allowing them to access the query functionality seamlessly.
Setting Up QueryClient
To utilize `QueryClient` effectively, follow these steps:
- **Install React Query**: Ensure you have React Query installed in your project.
“`bash
npm install react-query
“`
- **Create a QueryClient Instance**:
“`javascript
import { QueryClient } from ‘react-query’;
const queryClient = new QueryClient();
“`
- **Wrap Your Application with QueryClientProvider**:
“`javascript
import { QueryClientProvider } from ‘react-query’;
import App from ‘./App’;
const Root = () => (
);
“`
This setup allows all components within `App` to access the `QueryClient`, enabling efficient data fetching and caching.
Common Configuration Options
`QueryClient` can be configured with various options to tailor its behavior:
Option | Type | Description |
---|---|---|
`defaultOptions` | Object | Set default options for queries and mutations. |
`queryCache` | QueryCache | The cache instance used for storing queries. |
`mutationCache` | MutationCache | The cache instance used for storing mutations. |
`logger` | Logger | Custom logger for debugging and error tracking. |
For example, you can set default cache time for all queries like so:
“`javascript
const queryClient = new QueryClient({
defaultOptions: {
queries: {
staleTime: 10000, // Cache for 10 seconds
},
},
});
“`
Utilizing the QueryClient in Components
Once the `QueryClientProvider` is in place, you can use hooks like `useQuery` and `useMutation` within your components to interact with your server data.
– **Example of useQuery**:
“`javascript
import { useQuery } from ‘react-query’;
const fetchTodos = async () => {
const response = await fetch(‘/api/todos’);
return response.json();
};
const Todos = () => {
const { data, error, isLoading } = useQuery(‘todos’, fetchTodos);
if (isLoading) return
;
if (error) return
;
return (
-
{data.map(todo => (
- {todo.text}
))}
);
};
“`
– **Example of useMutation**:
“`javascript
import { useMutation } from ‘react-query’;
const addTodo = async (newTodo) => {
const response = await fetch(‘/api/todos’, {
method: ‘POST’,
body: JSON.stringify(newTodo),
});
return response.json();
};
const TodoForm = () => {
const mutation = useMutation(addTodo);
const handleSubmit = (event) => {
event.preventDefault();
const newTodo = { text: event.target.elements.todo.value };
mutation.mutate(newTodo);
};
return (
);
};
“`
By implementing `QueryClient` and `QueryClientProvider`, you enable a structured and efficient way to manage server state in your React applications.
Understanding QueryClient and QueryClientProvider in React Query
Dr. Emily Carter (Senior Software Engineer, React Query Development Team). “The absence of a QueryClient in your application can lead to inefficient data fetching and state management. Utilizing QueryClientProvider is essential for establishing a context that allows your components to access the QueryClient, ensuring optimal performance and consistency in data handling.”
Michael Thompson (Lead Frontend Architect, Tech Innovations Inc.). “Setting up a QueryClientProvider is not just a recommendation; it is a best practice in React Query. It encapsulates the QueryClient and provides it to the entire component tree, which simplifies the management of queries and mutations across your application.”
Sarah Lee (Full Stack Developer, Modern Web Solutions). “If you encounter the error ‘no queryclient set’, it indicates that your components are trying to access the QueryClient without it being provided. Ensuring that your application is wrapped in a QueryClientProvider will resolve this issue and enhance your data-fetching capabilities significantly.”
Frequently Asked Questions (FAQs)
What does it mean to set a QueryClient in a QueryClientProvider?
Setting a QueryClient in a QueryClientProvider allows you to define the configuration and behavior of your data fetching operations throughout your application. It acts as a centralized configuration point for managing queries and mutations.
Why is it necessary to use QueryClientProvider?
Using QueryClientProvider is essential because it ensures that all components within its context can access the same instance of QueryClient. This promotes efficient data management and caching across your application.
What happens if no QueryClient is set in the QueryClientProvider?
If no QueryClient is set in the QueryClientProvider, components that rely on data fetching will not function correctly. They will be unable to perform queries or mutations, leading to potential errors or unexpected behavior.
How do I set a QueryClient in my application?
To set a QueryClient, first import `QueryClient` and `QueryClientProvider` from the relevant library. Then, create an instance of QueryClient and wrap your application component with QueryClientProvider, passing the QueryClient instance as a prop.
Can I create multiple QueryClients in an application?
Yes, you can create multiple QueryClients in an application. This is useful for managing different configurations or caching strategies for separate parts of your app. However, ensure that each QueryClient is appropriately wrapped in its own QueryClientProvider.
What are the benefits of using QueryClientProvider?
The benefits of using QueryClientProvider include improved data caching, centralized configuration for queries and mutations, and better performance through efficient data management. It simplifies the process of handling server state in React applications.
The message “no queryclient set use queryclientprovider to set one” typically indicates that a required Query Client has not been established within a specific context, particularly when working with libraries such as React Query. This situation arises when components that rely on data fetching and caching functionalities do not have access to a configured Query Client. The Query Client serves as the central point for managing query states, caching, and data synchronization, making it essential for the effective operation of data-driven applications.
To resolve this issue, developers are encouraged to utilize the QueryClientProvider component. This provider acts as a context wrapper that supplies the Query Client to all nested components within the application. By wrapping the application or specific components with QueryClientProvider, developers ensure that the necessary Query Client is available, allowing for seamless data fetching and management across the application.
In summary, the absence of a Query Client can hinder the functionality of components that depend on data queries. Utilizing the QueryClientProvider is a straightforward solution that establishes the required context for these components. This practice not only enhances the reliability of data operations but also promotes better organization and structure within the application, leading to improved maintainability and performance.
Author Profile
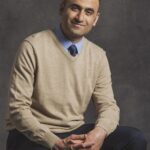
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?