Why Am I Seeing ‘No Suitable Driver Found for JDBC’ and How Can I Fix It?
In the world of database management and application development, establishing a reliable connection between your application and the database is crucial. However, encountering the error message “no suitable driver found for jdbc” can be a frustrating roadblock for developers and database administrators alike. This issue often arises when attempting to connect to a database using Java Database Connectivity (JDBC), and it can stem from various underlying causes. Understanding this error not only helps in troubleshooting but also enhances your overall proficiency in managing database connections effectively.
When you see the “no suitable driver found for jdbc” error, it typically indicates that the JDBC driver required for your specific database is either missing, not properly configured, or incompatible with your current setup. This situation can arise due to a variety of reasons, including incorrect classpath settings, outdated drivers, or even simple typographical errors in the connection URL. For developers, this error serves as a reminder of the importance of ensuring that all components of their database connection are correctly aligned.
Moreover, resolving this error involves a systematic approach to diagnosing the problem, which can include checking the driver installation, verifying connection strings, and ensuring that the appropriate dependencies are included in your project. By delving deeper into the nuances of JDBC connections, developers can not only fix the immediate issue
Common Causes of the “No Suitable Driver Found” Error
The “No suitable driver found for JDBC” error is typically encountered when the Java application fails to establish a connection to the database. This issue arises from several common causes, which include:
- Missing JDBC Driver: The most frequent reason for this error is that the JDBC driver for the specific database is not included in the application’s classpath.
- Incorrect Driver Class Name: If the driver class name is misspelled or incorrect, the application will not be able to load the driver.
- Incompatible Driver Version: Using a driver version that is incompatible with the Java version or the database can lead to connection issues.
- Driver Initialization Failure: Problems during the initialization of the driver, such as exceptions thrown in static blocks, can prevent it from being registered with the DriverManager.
Steps to Resolve the Error
To resolve the “No suitable driver found” error, follow these systematic steps:
- Verify Driver Inclusion:
- Ensure that the correct JDBC driver JAR file is added to your project’s dependencies.
- For Maven projects, check the `pom.xml` for the relevant dependency.
- Check Driver Class Name:
- Confirm that the driver class name in your connection code is correct.
- For example, the class name for MySQL is typically `com.mysql.cj.jdbc.Driver`.
- Confirm Classpath Configuration:
- Make sure that the JDBC driver JAR is included in the classpath of your application.
- In IDEs like Eclipse or IntelliJ, check the project settings to ensure the JAR is properly referenced.
- Test Compatibility:
- Verify that the version of the JDBC driver is compatible with both the Java version and the database version you are using.
- Consult the driver documentation for compatibility information.
- Initialize Driver Manually:
- As a workaround, you can manually load the driver using `Class.forName(“com.mysql.cj.jdbc.Driver”);` before establishing the connection.
Example Table of Common JDBC Drivers
Database | JDBC Driver Class | Driver Version |
---|---|---|
MySQL | com.mysql.cj.jdbc.Driver | 8.0.x |
PostgreSQL | org.postgresql.Driver | 42.x |
Oracle | oracle.jdbc.driver.OracleDriver | 19.x |
SQL Server | com.microsoft.sqlserver.jdbc.SQLServerDriver | 9.2.x |
Ensuring that you have the correct JDBC driver and that it is properly configured will typically resolve the “No suitable driver found” error.
Understanding the “No Suitable Driver Found” Error
The “No suitable driver found for JDBC” error typically occurs when the Java application fails to connect to a database due to the absence of the appropriate JDBC driver. This can stem from several factors, including driver compatibility, classpath configuration, or incorrect connection strings.
Common Causes
Identifying the root cause is essential for resolving this issue. Here are some common reasons:
- Missing JDBC Driver: The JDBC driver is not included in the classpath.
- Incorrect Driver Class Name: The specified driver class name does not match the actual driver.
- Driver Version Mismatch: The JDBC driver version is incompatible with the database version.
- Database URL Format: The connection string is not formatted correctly.
- Classpath Configuration Issues: The classpath does not include the necessary libraries.
Resolving the Issue
To resolve the “No suitable driver found” error, consider the following steps:
- Include the JDBC Driver: Ensure that the appropriate JDBC driver JAR file is included in your project’s build path. Common drivers include:
- MySQL: `mysql-connector-java-x.x.x.jar`
- PostgreSQL: `postgresql-x.x.x.jar`
- Oracle: `ojdbc8.jar`
- Check the Driver Class Name: Verify that you are using the correct driver class name. Examples include:
- MySQL: `com.mysql.cj.jdbc.Driver`
- PostgreSQL: `org.postgresql.Driver`
- Oracle: `oracle.jdbc.driver.OracleDriver`
- Validate the Connection URL: Ensure your connection URL follows the correct syntax. Example formats are:
- MySQL: `jdbc:mysql://hostname:port/dbname`
- PostgreSQL: `jdbc:postgresql://hostname:port/dbname`
- Oracle: `jdbc:oracle:thin:@hostname:port:dbname`
- Update Classpath Configuration: For applications running on servers like Tomcat or GlassFish, ensure the JDBC driver is placed in the appropriate `lib` directory or included in the project’s `WEB-INF/lib` directory.
- Check for Dependency Management: If using build tools like Maven or Gradle, include the JDBC driver dependency in your `pom.xml` or `build.gradle` file. For example, in Maven:
“`xml
“`
Testing the Connection
Once you have made the necessary changes, test the database connection with the following snippet:
“`java
try {
Class.forName(“com.mysql.cj.jdbc.Driver”);
Connection connection = DriverManager.getConnection(“jdbc:mysql://hostname:port/dbname”, “username”, “password”);
System.out.println(“Connection successful!”);
} catch (SQLException e) {
e.printStackTrace();
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
“`
This code snippet verifies that the driver can be loaded and that the connection can be established. If the issue persists, review the stack trace for additional clues regarding the failure.
By systematically addressing the potential causes of the “No suitable driver found” error, developers can effectively troubleshoot and resolve JDBC connection issues.
Understanding the “No Suitable Driver Found for JDBC” Error
Dr. Emily Chen (Database Systems Analyst, Tech Innovations Inc.). “The ‘No suitable driver found for JDBC’ error typically arises when the JDBC driver is not included in the classpath. Ensuring that the correct driver is loaded and properly configured is crucial for establishing a successful database connection.”
Mark Thompson (Senior Java Developer, CodeCraft Solutions). “This error often indicates a mismatch between the JDBC URL and the driver being used. Developers should verify that the JDBC URL is correctly formatted and corresponds to the specific database driver intended for use.”
Linda Martinez (Software Quality Assurance Engineer, Reliable Systems). “In many cases, the absence of the driver in the project dependencies can lead to this error. Utilizing build tools like Maven or Gradle to manage dependencies can help prevent such issues by ensuring that the required JDBC driver is included.”
Frequently Asked Questions (FAQs)
What does “no suitable driver found for jdbc” mean?
This error indicates that the Java Database Connectivity (JDBC) driver required to establish a connection to the database is not found in the classpath. It typically occurs when the application cannot locate the necessary driver for the specified database.
How can I resolve the “no suitable driver found for jdbc” error?
To resolve this error, ensure that the appropriate JDBC driver is included in your project’s classpath. Verify that the driver is correctly installed and that the connection URL is formatted correctly for the specific database.
What are common reasons for the “no suitable driver found for jdbc” error?
Common reasons include missing JDBC driver JAR files, incorrect connection URL syntax, or using an unsupported database version. Additionally, ensure that the driver class is loaded correctly in the application.
How do I add a JDBC driver to my project?
You can add a JDBC driver by downloading the appropriate JAR file and including it in your project’s build path. In Maven projects, you can add the dependency in the `pom.xml` file. For Gradle, include it in the `build.gradle` file.
Is it necessary to load the JDBC driver class explicitly?
In many cases, it is necessary to load the JDBC driver class explicitly using `Class.forName(“driver.class.name”)`. However, newer JDBC drivers support automatic loading, so this may not always be required.
What should I check if I still encounter the error after adding the driver?
If the error persists, check the connection URL for correctness, ensure that the driver version is compatible with your database, and confirm that there are no typos in the driver class name. Additionally, verify that the database service is running and accessible.
The error message “no suitable driver found for jdbc” typically indicates that the Java application is unable to locate the appropriate JDBC driver required to establish a connection to the specified database. This issue often arises due to several common factors, including the absence of the JDBC driver in the classpath, incorrect database URL syntax, or mismatched driver versions. Ensuring that the driver is correctly included and that the connection string is properly formatted is crucial for successful database connectivity.
To resolve this issue, developers should first verify that the JDBC driver for the specific database is included in the project’s dependencies. This can be done by checking the build configuration files, such as Maven’s `pom.xml` or Gradle’s `build.gradle`, to confirm that the driver is listed. Additionally, it is essential to ensure that the driver is compatible with the version of the database being used. If the application is running in an environment like a web server or application server, it may also be necessary to place the driver in the appropriate library directory.
Another important aspect to consider is the format of the JDBC URL. The URL must conform to the specific syntax required by the database being accessed. Any typographical errors or incorrect parameters can lead to the driver not being found. Developers
Author Profile
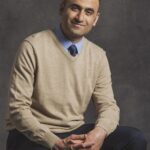
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?