Why Does Node Throw an Error for ‘Import Statement Outside a Module’?
In the ever-evolving landscape of JavaScript, the transition from traditional CommonJS modules to the modern ES6 module syntax has sparked a wave of excitement and innovation among developers. However, with this shift comes a set of challenges that can leave even seasoned programmers scratching their heads. One such conundrum is the notorious error message: “node cannot use import statement outside a module.” This seemingly cryptic warning can halt your development process, but understanding its root causes and solutions can empower you to harness the full potential of JavaScript’s modular capabilities.
As you delve into the intricacies of this error, you’ll discover that it stems from the fundamental differences between how Node.js and browsers handle modules. While ES6 modules offer a cleaner and more structured way to organize code, Node.js, by default, adheres to the CommonJS module system, leading to compatibility issues. This article will guide you through the nuances of module types, the configuration settings that can help you bridge the gap, and the best practices for a seamless development experience.
Whether you’re a newcomer eager to learn or a seasoned developer looking to refine your skills, understanding the implications of this error is crucial for navigating the modern JavaScript ecosystem. Join us as we unpack the complexities behind the “import statement outside a
Understanding the Error Message
The error message “Cannot use import statement outside a module” typically occurs in Node.js environments when attempting to use ES6 module syntax (the `import` statement) in a file that Node.js does not recognize as an ES module. By default, Node.js treats files as CommonJS modules unless specified otherwise.
To understand this error better, it’s essential to know the distinctions between CommonJS and ES modules. CommonJS uses `require()` for importing modules, while ES modules use `import`. This distinction is crucial when working with different module systems in a Node.js environment.
How to Resolve the Error
There are several ways to resolve this issue, depending on your project requirements and the version of Node.js you are using. Below are some common methods:
- Use .mjs Extension: Rename your file to have a `.mjs` extension. Node.js recognizes `.mjs` files as ES modules.
- Set “type”: “module” in package.json: If you want to use ES module syntax in `.js` files, add `”type”: “module”` to your `package.json` file. This informs Node.js to treat all `.js` files in the project as ES modules.
- Transpile with Babel: If you need to support both module systems or older Node.js versions, consider using Babel to transpile your code from ES6 to CommonJS.
- Dynamic Imports: If you need to import a module conditionally, consider using dynamic imports with the `import()` syntax, which works in both module types.
Example Configurations
Here are examples of how to configure your project for different approaches:
Method | Configuration |
---|---|
Using .mjs | Rename your file to example.mjs |
package.json Type Module |
{ "type": "module" } |
Babel Transpilation |
npm install --save-dev @babel/core @babel/preset-env // In .babelrc { "presets": ["@babel/preset-env"] } |
Dynamic Imports | Use const module = await import('./module.js'); |
Additional Considerations
When converting to ES modules, keep in mind the following:
- Ensure all dependencies support ES module syntax.
- Be aware of the differences in how modules are loaded and executed in ES modules compared to CommonJS.
- Test your application thoroughly after making changes to the module system to catch any issues early.
By carefully managing the transition between module types, developers can leverage the benefits of ES6 modules while maintaining compatibility with existing codebases.
Understanding the Error
The error message “Cannot use import statement outside a module” typically occurs in Node.js when attempting to use ES6 modules (import/export syntax) in an environment that does not support it by default. This is often due to the configuration of the JavaScript environment or the file extension used.
Common Causes
Several factors can lead to encountering this error:
- File Type: Node.js treats files with a `.js` extension as CommonJS modules by default. If you are using ES6 import/export syntax, you need to specify that the file is a module.
- Package Configuration: The `package.json` file may not be configured to support ES modules.
- Node.js Version: Some versions of Node.js do not support ES modules without specific configurations.
How to Resolve the Error
To resolve the error, consider the following solutions:
Change File Extension
Rename your file from `.js` to `.mjs`. Node.js automatically treats `.mjs` files as ES modules.
Update package.json
Add the following line to your `package.json` file:
“`json
{
“type”: “module”
}
“`
This configuration tells Node.js to treat all `.js` files in the package as ES modules.
Use Babel or a Module Bundler
If you need to support environments that do not understand ES modules, consider using Babel or a module bundler like Webpack or Rollup. These tools allow you to transpile ES6 code into a version compatible with CommonJS.
Example Configuration
Here is an example of a minimal `package.json` configuration that enables ES modules:
“`json
{
“name”: “my-app”,
“version”: “1.0.0”,
“type”: “module”,
“main”: “index.js”,
“scripts”: {
“start”: “node index.js”
},
“dependencies”: {}
}
“`
Using ES Modules in Node.js
When using ES modules, ensure you follow these practices:
– **Importing Modules**: Use the import statement as follows:
“`javascript
import express from ‘express’;
“`
– **Exporting Modules**: Use the export statement:
“`javascript
export const myFunction = () => {};
“`
- Top-Level Await: ES modules support top-level await. You can write:
“`javascript
const data = await fetchData();
“`
Fallback to CommonJS
If you prefer to continue using CommonJS syntax, you can:
- Use `require` to import modules:
“`javascript
const express = require(‘express’);
“`
- Use `module.exports` to export modules:
“`javascript
module.exports = myFunction;
“`
This approach avoids the complexities of handling ES modules while maintaining compatibility with existing Node.js codebases.
By understanding the error and implementing the appropriate configurations, you can effectively use ES modules in your Node.js applications without encountering the “Cannot use import statement outside a module” error.
Understanding the Import Statement Issue in Node.js
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Corp). “The error ‘node cannot use import statement outside a module’ typically arises when the Node.js environment is not configured to recognize ES modules. Developers must ensure that their package.json includes the ‘type’: ‘module’ field to enable this functionality.”
Michael Thompson (JavaScript Specialist, Code Academy). “This issue often confuses newcomers to Node.js. It is essential to understand that Node.js defaults to CommonJS modules. To utilize the import statement, one must either switch to using the .mjs file extension or adjust the package.json settings accordingly.”
Sarah Lee (Full-Stack Developer, Dev Solutions Inc.). “When encountering this error, it is advisable to check the Node.js version in use. ES module support has improved in recent releases, and ensuring compatibility can resolve many issues related to import statements.”
Frequently Asked Questions (FAQs)
What does the error “node cannot use import statement outside a module” mean?
This error indicates that the JavaScript file is attempting to use the `import` statement, which is only valid in ES modules, while the Node.js environment is treating the file as a CommonJS module.
How can I enable ES modules in Node.js?
To enable ES modules, you can either rename your JavaScript files with a `.mjs` extension or add `”type”: “module”` to your `package.json` file. This informs Node.js to treat the files as ES modules.
What is the difference between CommonJS and ES modules?
CommonJS is a module system used primarily in Node.js, utilizing `require()` and `module.exports`. ES modules, introduced in ECMAScript 6, use `import` and `export` syntax, allowing for more flexible and optimized module loading.
Can I mix CommonJS and ES modules in the same project?
While it is technically possible to mix both module systems, it is generally discouraged due to potential compatibility issues. If you must mix them, ensure that you use appropriate import/export syntax and be aware of the limitations.
What should I do if I want to use `import` statements in a Node.js project?
If you want to use `import` statements, configure your project to use ES modules by following the steps mentioned earlier. Alternatively, consider using a transpiler like Babel to convert ES module syntax into CommonJS.
Is there a way to run scripts with ES module syntax without changing the project structure?
Yes, you can run scripts using the `–experimental-modules` flag in Node.js versions prior to 14. However, this approach is not recommended for production environments, as it may lead to unexpected behavior.
The error message “node cannot use import statement outside a module” typically arises when attempting to use ES6 module syntax (import/export) in a Node.js environment that is not configured to recognize it. By default, Node.js treats files as CommonJS modules, which utilize require() for module imports. To resolve this issue, developers must ensure that their Node.js environment is set up to support ES6 modules by either using the .mjs file extension or by specifying “type”: “module” in the package.json file.
Another important consideration is the version of Node.js being used. ES6 module support was introduced in Node.js 12, but it is more stable and fully featured in later versions. Therefore, it is advisable for developers to use the latest stable version of Node.js to take advantage of all the enhancements and features related to module handling.
Furthermore, when working with mixed module types, such as importing CommonJS modules into ES6 modules, developers should be aware of the differences in syntax and behavior. This can lead to additional complications, and careful handling of imports and exports is essential to avoid runtime errors. Understanding the module system in Node.js is crucial for effective development and to prevent common pitfalls associated with module imports.
Author Profile
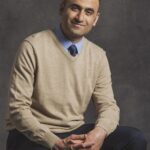
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?