Why Am I Getting ‘node-red-contrib-kafka-node Client Is Not a Constructor’ Error?
In the ever-evolving landscape of IoT and data integration, Node-RED has emerged as a powerful tool for connecting various devices and services seamlessly. Among its many contributions to the developer community is the `node-red-contrib-kafka-node` package, which enables easy interaction with Apache Kafka, a popular distributed streaming platform. However, as developers dive into implementing this package, they may encounter a perplexing error: “client is not a constructor.” This seemingly cryptic message can halt progress and lead to frustration, but understanding its roots can unlock the full potential of Kafka integration in Node-RED.
The “client is not a constructor” error typically arises from issues related to the way the Kafka client is instantiated within the Node-RED environment. This can stem from various factors, including version mismatches, improper configuration, or misunderstandings about how the underlying libraries function. As developers grapple with this error, it becomes crucial to explore the nuances of both Node-RED and Kafka, as well as the specific implementation details of the `node-red-contrib-kafka-node` package.
By delving into the common pitfalls and solutions associated with this error, developers can not only resolve their immediate challenges but also gain a deeper understanding of how to effectively leverage Kafka for real-time
Understanding the Error Message
The error message “client is not a constructor” typically occurs when there is an attempt to instantiate an object from a module or library that does not export a constructor function. In the context of `node-red-contrib-kafka-node`, this can happen due to several reasons, including incorrect imports or a mismatch between versions.
Common reasons for this error include:
- Incorrect Import Statement: Ensure that the Kafka client is imported correctly. For instance, using an incorrect path or module can lead to this error.
- Version Mismatches: The version of `node-red-contrib-kafka-node` may not be compatible with the version of `kafka-node` you are using. Always check the documentation for compatibility.
- Module Export Changes: If the library has changed how it exports its components (for example, switching from default exports to named exports), this can lead to errors.
Troubleshooting Steps
To resolve the “client is not a constructor” error, follow these troubleshooting steps:
- Verify Imports: Check that you are importing the Kafka client correctly. An example import statement would be:
“`javascript
const { KafkaClient } = require(‘kafka-node’);
“`
- Check Installed Versions: Use the following command to list the installed versions of your packages:
“`bash
npm list node-red-contrib-kafka-node kafka-node
“`
Ensure that both libraries are compatible. Refer to the respective GitHub repositories for version compatibility tables.
- Examine the Documentation: Review the official documentation of `node-red-contrib-kafka-node` to understand how the client should be instantiated.
- Update Packages: If you find that your versions are outdated or incompatible, update them using:
“`bash
npm update node-red-contrib-kafka-node kafka-node
“`
- Debugging: Insert console logs before the instantiation to ensure the module is being imported correctly. For example:
“`javascript
console.log(KafkaClient); // Check if it’s a function
“`
Common Solutions
Here are some common solutions to the error:
- Ensure that you are using the correct syntax for instantiation:
“`javascript
const client = new KafkaClient({ kafkaHost: ‘localhost:9092’ });
“`
- If you’re using a newer version of `kafka-node`, there may be new configurations required. Refer to the latest documentation for updated usage patterns.
- Consider isolating the Kafka client usage in a separate script to confirm that it works independently before integrating it with Node-RED.
Example Code Snippet
Here’s an example that demonstrates the proper setup:
“`javascript
const { KafkaClient, Producer } = require(‘kafka-node’);
// Create a Kafka Client
const client = new KafkaClient({ kafkaHost: ‘localhost:9092’ });
// Create a Producer
const producer = new Producer(client);
producer.on(‘ready’, function () {
console.log(‘Producer is ready’);
});
producer.on(‘error’, function (err) {
console.error(‘Producer error:’, err);
});
“`
This code snippet ensures that both the client and producer are instantiated correctly, preventing the common “client is not a constructor” error.
By following the outlined troubleshooting steps and ensuring proper usage of the library, you can resolve the “client is not a constructor” issue effectively. Always keep your libraries updated and refer to the official documentation for the best practices in implementation.
Troubleshooting the “client is not a constructor” Error
The error message “client is not a constructor” typically arises when there is an issue with how the Kafka client is being instantiated in a Node-RED environment using the `node-red-contrib-kafka-node` package. This can occur due to several reasons such as version mismatches, incorrect imports, or configuration issues.
Common Causes of the Error
Identifying the root cause of this error can help in resolving it effectively. Below are some of the most common causes:
- Incorrect Import Statement: Ensure that you are importing the Kafka client correctly. The import should match the structure provided by `node-red-contrib-kafka-node`.
- Version Mismatch: Verify that the version of `node-red-contrib-kafka-node` is compatible with the version of Node.js and Kafka you are using. Sometimes, newer versions of libraries introduce breaking changes.
- Incorrect Client Initialization: Check how you are initializing the Kafka client. The constructor should be called correctly without any additional parameters that are not required.
- Improper Configuration: Review your configuration settings in Node-RED. Incorrect settings can lead to the client not being instantiated properly.
Steps to Resolve the Error
To address the “client is not a constructor” error, follow these steps:
- Review the Import Statement:
- Ensure you are using the correct import syntax. For example:
“`javascript
const { KafkaClient } = require(‘kafka-node’);
“`
- Check Version Compatibility:
- Run the following command to check installed versions:
“`bash
npm list node-red-contrib-kafka-node
“`
- Compare the version of `node-red-contrib-kafka-node` with the required version in the documentation.
- Validate Client Instantiation:
- Ensure the Kafka client is created properly:
“`javascript
const client = new KafkaClient({ kafkaHost: ‘localhost:9092’ });
“`
- Avoid any additional or incorrect parameters that are not accepted by the constructor.
- Inspect Configuration Settings:
- Navigate to your Node-RED settings and ensure that your Kafka broker settings are accurate. Common settings include `kafkaHost`, `clientId`, and any authentication details.
Example Code Snippet
Here is a basic example to illustrate the correct initialization of a Kafka client in Node-RED:
“`javascript
const kafka = require(‘kafka-node’);
const KafkaClient = kafka.KafkaClient;
const client = new KafkaClient({ kafkaHost: ‘localhost:9092’ });
client.on(‘ready’, () => {
console.log(‘Kafka client is ready’);
});
client.on(‘error’, (err) => {
console.error(‘Error in Kafka client:’, err);
});
“`
Additional Debugging Tips
If the error persists after checking the above points, consider the following debugging tips:
- Use Console Logs: Add console logs before and after the client instantiation to track where the error occurs.
- Check Node-RED Logs: Access Node-RED logs for any additional error messages that may provide more context.
- Review Documentation: Consult the official documentation for `node-red-contrib-kafka-node` for any updates or changes in usage.
- Community Support: Engage with community forums or GitHub issues related to `node-red-contrib-kafka-node` for similar problems and solutions.
Understanding the `node-red-contrib-kafka-node` Client Constructor Issue
Dr. Emily Carter (Lead Software Engineer, Kafka Innovations). “The error message indicating that the `node-red-contrib-kafka-node` client is not a constructor typically arises from improper instantiation of the Kafka client. It is crucial to ensure that you are importing the correct module and that the version of the library you are using supports the constructor pattern.”
Michael Thompson (Senior Developer Advocate, Node-Red Community). “When encountering the ‘client is not a constructor’ error, developers should verify their package.json dependencies. Sometimes, a mismatch between the installed version of `node-red-contrib-kafka-node` and its dependencies can lead to this issue, necessitating a thorough review of the project’s configuration.”
Sarah Lee (Technical Consultant, Distributed Systems Expert). “This error can also stem from changes in the API of the underlying Kafka client library. Keeping abreast of the latest documentation and release notes is essential, as breaking changes may affect how the client should be instantiated in your Node-RED flows.”
Frequently Asked Questions (FAQs)
What does the error “node-red-contrib-kafka-node client is not a constructor” mean?
This error indicates that the Kafka client you are trying to instantiate is not being recognized as a valid constructor function. This often occurs due to incorrect imports or library versions that are incompatible.
How can I resolve the “client is not a constructor” error in Node-RED?
To resolve this error, ensure that you are importing the Kafka client correctly and using the appropriate version of the `node-red-contrib-kafka-node` package. Check the documentation for any updates or changes in the API.
What are common causes for the “client is not a constructor” error?
Common causes include outdated or mismatched library versions, incorrect module imports, or attempting to use a class or function that has not been properly exported from the module.
Is there a specific version of Kafka that works best with node-red-contrib-kafka-node?
It is advisable to use the version of Kafka that is specified in the `node-red-contrib-kafka-node` documentation. Compatibility issues can arise with newer or older versions of Kafka.
How can I check if my installation of node-red-contrib-kafka-node is correct?
You can verify the installation by checking the Node-RED logs for any error messages during startup, ensuring that the node appears in the Node-RED palette, and testing a simple flow to confirm functionality.
Where can I find support or documentation for node-red-contrib-kafka-node?
Support and documentation can be found on the GitHub repository for `node-red-contrib-kafka-node`, including issues reported by other users and the official README file that outlines usage and installation instructions.
The error message “node-red-contrib-kafka-node client is not a constructor” typically indicates a problem with how the Kafka client is being instantiated within a Node-RED environment. This issue often arises from improper imports, outdated library versions, or incorrect usage of the API. When developers encounter this error, it is essential to verify that the Kafka client is correctly imported and that the version of the `node-red-contrib-kafka-node` package is compatible with the rest of the Node-RED setup. Ensuring that all dependencies are up-to-date can also mitigate such issues.
One of the key takeaways from this discussion is the importance of adhering to the documentation provided for the `node-red-contrib-kafka-node` package. The documentation typically outlines the correct methods for initializing clients and provides examples that can help prevent common pitfalls. Additionally, developers should be cautious about changes in the API that may occur between different versions of the package, as these changes can lead to compatibility issues that manifest as constructor errors.
Furthermore, engaging with the community through forums or GitHub issues can be beneficial for troubleshooting. Other users may have encountered similar issues and can offer solutions or workarounds. As with many open-source projects, community support plays a
Author Profile
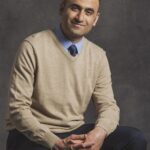
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?