Why Can’t a Non-Static Variable Be Referenced from a Static Context?
Understanding the Non-Static Variable: The Challenge of ‘this’ in Static Contexts
In the world of object-oriented programming, the distinction between static and non-static variables is crucial for developers. One common error that programmers encounter, particularly in languages like Java, is the infamous message: “non-static variable this cannot be referenced from a static context.” This seemingly cryptic warning can be a source of confusion for both novice and experienced coders alike. But what does it really mean, and why is it so important to grasp this concept?
At its core, the issue stems from the fundamental differences between static and non-static contexts in programming. Static variables belong to the class itself rather than to any specific instance of that class, while non-static variables are tied to individual objects. This distinction leads to a critical understanding of how and when to access these variables. When a static method tries to reference a non-static variable, it creates a conflict, as the static method does not have access to instance-specific data.
Navigating this error requires a solid grasp of object-oriented principles, particularly the lifecycle of objects and the scope of variables. By understanding the implications of static and non-static contexts, developers can write more efficient, error-free code. In the following sections, we will
Understanding Non-Static Variables
In Java, the term “non-static variable” refers to instance variables that belong to an instance of a class. These variables are created when an object is instantiated and are unique to that object. Non-static variables can be accessed using the `this` keyword, which refers to the current object.
Key characteristics of non-static variables include:
- Instance-Specific: Each object has its own copy of non-static variables.
- Memory Allocation: Memory is allocated for non-static variables when an object is created.
- Access: Non-static variables can be accessed directly from instance methods but not from static methods.
The Role of the `this` Keyword
The `this` keyword is crucial in differentiating instance variables from parameters or local variables within methods. It provides a reference to the current object, allowing developers to clarify which variable is being referred to in cases of naming conflicts.
Consider the following code snippet:
“`java
public class Example {
private int value;
public Example(int value) {
this.value = value; // ‘this.value’ refers to the instance variable
}
}
“`
In this example, `this.value` refers to the instance variable, while `value` without `this` refers to the constructor’s parameter.
Static Methods and Variables
Static methods belong to the class itself rather than any particular instance. They can be called without creating an object of the class. However, static methods cannot directly access non-static variables or methods because they do not have a reference to an instance of the class.
This restriction is due to the fact that static methods do not have access to the `this` keyword, as there is no current object context.
Why the Error Occurs
The error message “non-static variable this cannot be referenced from a static context” occurs when you attempt to access a non-static variable from within a static method. This indicates that the method does not have a reference to an instance of the class.
Here’s an example that illustrates this error:
“`java
public class Example {
private int value;
public void instanceMethod() {
// This works fine
System.out.println(this.value);
}
public static void staticMethod() {
// This will cause a compile-time error
System.out.println(this.value); // Error: non-static variable this cannot be referenced from a static context
}
}
“`
Best Practices to Avoid the Error
To avoid this error, consider the following best practices:
- Use Static Variables and Methods: If a method needs to access a variable, consider making it static if no instance-specific data is required.
- Create an Instance: If static context access is necessary, create an instance of the class to access non-static variables.
Example of accessing non-static variables correctly:
“`java
public class Example {
private int value;
public Example(int value) {
this.value = value;
}
public static void staticMethod() {
Example example = new Example(10);
System.out.println(example.value); // Accessing non-static variable through an instance
}
}
“`
Comparison of Static and Non-Static Contexts
Feature | Static Context | Non-Static Context |
---|---|---|
Access to Instance Variables | No | Yes |
Access to Static Variables | Yes | Yes |
Reference to `this` | No | Yes |
Invocation Method | Class Name | Object Instance |
Understanding the Error
The error message “non-static variable this cannot be referenced from a static context” typically arises in Java programming when there is an attempt to access instance variables or methods from a static context. To grasp the implications of this error, it is essential to understand the differences between static and non-static members of a class.
- Static Members: These belong to the class itself rather than any specific instance. They can be accessed without creating an object of the class.
- Non-Static Members: These are tied to a specific instance of the class. To use them, an object of the class must be instantiated.
The error occurs because static contexts do not have access to the instance (`this`) reference.
Common Scenarios Leading to the Error
Several coding scenarios can lead to this error:
- Static Methods Accessing Instance Variables: When a static method tries to access instance variables directly without an object reference.
“`java
public class Example {
int instanceVar = 10;
static void staticMethod() {
// Error: Cannot reference instanceVar
System.out.println(instanceVar);
}
}
“`
- Static Blocks or Initializers: When static blocks attempt to use instance variables or methods.
“`java
public class Example {
int instanceVar = 20;
static {
// Error: Cannot reference instanceVar
System.out.println(instanceVar);
}
}
“`
- Static Nested Classes: When static nested classes attempt to access non-static members of the enclosing class without an instance.
Resolving the Error
To fix the “non-static variable this cannot be referenced from a static context” error, follow these strategies:
- Use an Instance of the Class: Create an object of the class within the static method or block to access instance members.
“`java
public class Example {
int instanceVar = 30;
static void staticMethod() {
Example example = new Example();
System.out.println(example.instanceVar); // Now valid
}
}
“`
- Make Methods Static: If the functionality does not require instance variables, consider making the method static.
“`java
public class Example {
static int staticVar = 40;
static void staticMethod() {
System.out.println(staticVar); // Now valid
}
}
“`
- Refactor Code: Sometimes, the design may need reconsideration. Evaluate if a method should be static or if the logic can be contained within an instance method instead.
Best Practices to Avoid the Error
To prevent encountering this error, adhere to the following best practices:
- Clearly Distinguish Between Static and Instance Contexts: Understand when to use static methods versus instance methods.
- Encapsulation: Keep non-static variables private and provide public getters/setters if needed.
- Code Review: Regularly review code to identify potential static context issues before compilation.
- Testing: Implement unit tests to ensure that instance-dependent logic is correctly structured.
By following these strategies, developers can effectively navigate and resolve the “non-static variable this cannot be referenced from a static context” error in their Java applications.
Understanding Static Contexts and Non-Static Variables
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The error ‘non-static variable this cannot be referenced from a static context’ arises because static methods belong to the class itself rather than to any specific instance. Therefore, they cannot access instance variables or methods directly, which require an object reference.”
Johnathan Lee (Java Programming Instructor, Code Academy). “When you encounter this error, it is crucial to remember that static methods do not have access to the instance variables of the class. To resolve this, you can either create an instance of the class or convert the method to a non-static one, depending on your design requirements.”
Sarah Thompson (Lead Developer, Future Tech Solutions). “Understanding the distinction between static and non-static contexts is essential for effective Java programming. This error serves as a reminder to developers about the scope of variables and methods, emphasizing the need for proper object-oriented principles.”
Frequently Asked Questions (FAQs)
What does the error “non-static variable this cannot be referenced from a static context” mean?
This error indicates that you are trying to access an instance variable or method from a static context, such as a static method or a static block. In Java, static members belong to the class itself, while non-static members belong to instances of the class.
How can I resolve the “non-static variable this cannot be referenced from a static context” error?
To resolve this error, you can either make the variable or method static if it does not depend on instance-specific data, or create an instance of the class to access the non-static member.
Can you provide an example of this error in Java?
Certainly. For instance, if you have a class with a non-static method `display()` and you try to call it from a static method like `main`, you will encounter this error unless you create an instance of the class to call `display()`.
What is the difference between static and non-static variables in Java?
Static variables are shared among all instances of a class and can be accessed without creating an object, while non-static variables are unique to each instance and require an object to be accessed.
Is it possible to access non-static variables from a static method without creating an object?
No, it is not possible to access non-static variables from a static method without creating an instance of the class. Static methods do not have access to instance-specific data.
What are some best practices to avoid this error in Java programming?
To avoid this error, use static methods only for operations that do not require instance data. If instance data is necessary, consider refactoring the code to use instance methods or passing the required instance as a parameter.
The error message “non-static variable this cannot be referenced from a static context” is a common issue encountered in Java programming. This error arises when a static method or block attempts to access instance variables or methods, which are tied to a specific object instance. In Java, static members belong to the class itself rather than any particular instance, which creates a fundamental distinction that developers must understand to avoid this error.
To resolve this issue, developers can either instantiate the class to access its non-static members or change the static method to an instance method if the context allows. Understanding the difference between static and non-static contexts is crucial for effective object-oriented programming in Java. This knowledge not only aids in preventing compilation errors but also enhances the overall design and architecture of the code.
Moreover, this error emphasizes the importance of proper class design and the appropriate use of static methods. Static methods are best utilized for utility functions or operations that do not require access to instance-specific data. By adhering to these principles, developers can create more robust and maintainable Java applications, minimizing the likelihood of encountering similar errors in the future.
Author Profile
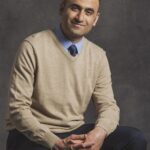
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?