Why Am I Seeing ‘Nonetype Object is Not Iterable’ Error and How Can I Fix It?
Have you ever encountered the perplexing error message “nonetype object is not iterable” while coding in Python? If you have, you’re not alone. This common pitfall can leave even seasoned developers scratching their heads, as it often arises unexpectedly and can disrupt the flow of your programming. Understanding this error is crucial for anyone looking to enhance their coding skills and streamline their debugging process. In this article, we will unravel the mystery behind this message, explore its causes, and equip you with the tools to prevent it from derailing your projects.
Overview
At its core, the “nonetype object is not iterable” error signifies that you’re attempting to iterate over an object that is of type `None`. In Python, `None` is a special value that represents the absence of a value or a null reference. When you try to loop through or unpack a `None` object, Python raises this error, indicating that it cannot perform the operation you intended. This often occurs in scenarios where functions or methods return `None` instead of a list, tuple, or other iterable types.
Understanding the context in which this error arises is essential for effective troubleshooting. Common culprits include functions that lack a return statement or those that inadvertently return `None` due to conditional logic
Understanding the Error Message
The error message “NoneType object is not iterable” typically occurs in Python when an operation attempts to iterate over a variable that is `None`. This situation often arises in contexts where a function or method is expected to return an iterable object, such as a list or a tuple, but instead returns `None`.
Common scenarios that lead to this error include:
- Attempting to loop through the result of a function that does not return a value.
- Trying to unpack values from a function that returns `None`.
- Using list comprehensions or generator expressions with variables that are not properly initialized.
To illustrate, consider the following code snippet:
“`python
def get_items():
This function is expected to return a list of items
return None Returns None instead of a list
for item in get_items():
print(item)
“`
In this example, the function `get_items` returns `None`, leading to the error when trying to iterate over its result.
Identifying the Source of the Error
To effectively troubleshoot this error, follow these steps:
- Check Function Returns: Ensure that all functions expected to return iterable objects actually do so. Validate the return statements.
- Print Debugging: Use print statements to output the values of variables before the iteration to confirm their types.
- Type Checking: Implement type checks to verify that the variable is indeed an iterable before attempting to iterate.
Here’s a simple way to check if a variable is iterable:
“`python
from collections.abc import Iterable
def is_iterable(obj):
return isinstance(obj, Iterable)
result = get_items()
if is_iterable(result):
for item in result:
print(item)
else:
print(“The result is not iterable.”)
“`
Common Fixes
To resolve the “NoneType object is not iterable” error, consider the following approaches:
- Ensure Functions Return Valid Iterables: Modify the function to return an empty list or another appropriate iterable if there are no items to return.
“`python
def get_items():
Logic to retrieve items
return [] Return an empty list instead of None
“`
- Use Default Values: When functions can return `None`, use default values to handle such cases gracefully.
- Error Handling: Implement try-except blocks to manage exceptions and provide informative error messages.
Approach | Description |
---|---|
Return an Empty List | Modify the function to return `[]` instead of `None`. |
Default Values | Use default values in function parameters to avoid `None` returns. |
Error Handling | Utilize try-except blocks to catch and handle the error gracefully. |
By following these guidelines, you can effectively address and prevent the “NoneType object is not iterable” error in your Python code.
Understanding the Error
The error message “NoneType object is not iterable” typically arises in Python when attempting to iterate over an object that is `None`. This can occur in various scenarios, particularly when a function that is expected to return a list or similar iterable type instead returns `None`.
Common scenarios leading to this error include:
- Forgetting to return a value from a function.
- Assigning the result of a function that may not return an iterable.
- Using an assignment operation that mistakenly overrides an iterable variable with `None`.
Common Causes
Identifying the root cause of this error is essential for debugging. Below are some frequent reasons why this error may occur:
- Function Returns None: A function intended to return a list might not have a return statement, or it might have a conditional that leads to no return under certain circumstances.
- Incorrect Variable Assignment: A variable that is expected to hold an iterable may be assigned `None` due to previous operations or conditions.
- List Comprehensions: Using a list comprehension with a function that can potentially return `None` can lead to this error.
Examples
Here are examples illustrating how the error occurs:
“`python
def get_numbers():
Missing return statement
numbers = [1, 2, 3]
return numbers Uncommenting this line will fix the issue.
result = get_numbers()
for number in result:
print(number) Raises “TypeError: ‘NoneType’ object is not iterable”
“`
“`python
def fetch_data(condition):
if condition:
return [1, 2, 3]
Implicitly returns None if condition is
data = fetch_data()
for item in data: Raises “TypeError: ‘NoneType’ object is not iterable”
print(item)
“`
Debugging Techniques
To address the “NoneType object is not iterable” error, consider the following debugging strategies:
- Check Function Returns: Ensure that all code paths in a function return an iterable. Add print statements to verify the output of functions.
- Use Type Checking: Implement type checks to confirm that a variable is not `None` before iterating over it.
“`python
if data is not None:
for item in data:
print(item)
else:
print(“Data is None, cannot iterate.”)
“`
- Utilize Assertions: Use assertions to enforce that certain variables must not be `None`.
“`python
assert data is not None, “Data must not be None”
“`
Preventative Measures
To avoid encountering this error in the future, follow these best practices:
- Always Return Values: Ensure functions that are expected to return iterables do so on all execution paths.
- Initialize Variables: Initialize variables with an empty iterable (e.g., `[]`) rather than `None` when they are declared.
- Thorough Testing: Implement unit tests to cover edge cases where functions might return `None`.
Technique | Description |
---|---|
Return Statements | Ensure all branches return a valid iterable. |
Initialization | Set variables to empty lists when appropriate. |
Type Checking | Verify variable types before iteration. |
Assertions | Enforce preconditions for functions and variables. |
By applying these practices, developers can minimize the risk of encountering the “NoneType object is not iterable” error in their codebases.
Understanding the ‘NoneType Object is Not Iterable’ Error
Dr. Emily Carter (Software Engineer, Tech Innovations Inc.). “The ‘NoneType object is not iterable’ error typically arises when a function returns None instead of a list or other iterable object. Developers must ensure that they are handling return values correctly and implementing checks to avoid attempting to iterate over None.”
Michael Chen (Data Scientist, Analytics Solutions Group). “In data processing, this error can indicate that an expected dataset is missing or not properly initialized. It’s crucial to validate input data and confirm that all necessary data structures are in place before performing iterations.”
Sarah Thompson (Python Developer, CodeCraft Academy). “To prevent the ‘NoneType object is not iterable’ error, developers should adopt defensive programming techniques. This includes using assertions and exception handling to catch potential issues early in the code execution process.”
Frequently Asked Questions (FAQs)
What does the error ‘NoneType object is not iterable’ mean?
This error indicates that you are attempting to iterate over a variable that is `None`, which is not a valid iterable type in Python.
What causes a variable to be None in Python?
A variable may be assigned `None` explicitly, or it may be the result of a function that does not return a value. Additionally, it can occur if an operation fails to produce a valid result.
How can I troubleshoot the ‘NoneType object is not iterable’ error?
To troubleshoot, check the variable causing the error to ensure it is not `None`. Use print statements or debugging tools to trace where the variable is assigned and confirm it holds an iterable value.
What types of objects are considered iterable in Python?
In Python, iterable objects include lists, tuples, dictionaries, sets, and strings. These objects can be looped over using constructs like for-loops.
Can I prevent the ‘NoneType object is not iterable’ error?
Yes, you can prevent this error by validating the variable before iteration. Use conditional statements to check if the variable is not `None` and is indeed iterable.
What should I do if I need to handle a None value in a loop?
If you need to handle a `None` value in a loop, you can use a conditional check within the loop to skip the iteration or provide a default value to avoid the error.
The error message “NoneType object is not iterable” typically occurs in Python when an operation attempts to iterate over an object that is of type None. This situation often arises when a function that is expected to return an iterable, such as a list or a tuple, instead returns None. Understanding the context in which this error occurs is crucial for effective debugging and code correction.
Common scenarios leading to this error include forgetting to return a value from a function, mistakenly assigning None to a variable intended to hold an iterable, or misusing conditional statements that result in None being returned. Developers can mitigate this issue by ensuring that functions consistently return the expected data types and by implementing checks to validate the type of variables before attempting to iterate over them.
Key takeaways include the importance of thorough testing and debugging practices to identify the source of the NoneType error. Utilizing tools such as print statements or debuggers can help trace the flow of data and confirm that functions are returning the correct values. Additionally, employing type hints and assertions can enhance code clarity and prevent such errors from occurring in the first place.
Author Profile
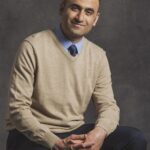
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?