How Can You Get Notified When a Custom Resource in Kubernetes Changes?
In the dynamic world of Kubernetes, managing custom resources effectively is crucial for ensuring the stability and performance of your applications. As developers and operators strive to build resilient systems, the ability to monitor changes in these custom resources becomes increasingly vital. Imagine receiving timely notifications when a critical configuration is altered or when a resource’s status changes unexpectedly. This capability not only enhances your operational awareness but also empowers you to respond swiftly to potential issues, minimizing downtime and maintaining service quality.
Understanding how to set up notifications for changes in custom resources is essential for anyone working with Kubernetes. Custom resources allow users to extend Kubernetes capabilities, enabling the creation of tailored solutions that meet specific needs. However, with great flexibility comes the responsibility of monitoring these resources effectively. By implementing notification mechanisms, you can stay informed about any modifications, ensuring that your applications run smoothly and your infrastructure remains robust.
In this article, we will explore various strategies and tools that can help you achieve effective notification systems for Kubernetes custom resources. From leveraging built-in Kubernetes features to utilizing external monitoring solutions, we’ll provide insights into how you can enhance your observability and responsiveness. Whether you are a seasoned Kubernetes administrator or just starting your journey, understanding how to notify when custom resources change will empower you to take control of your Kubernetes environment like never before.
Understanding Kubernetes Custom Resource Definitions (CRDs)
Kubernetes Custom Resource Definitions (CRDs) extend Kubernetes capabilities by allowing users to define their own resource types. This enables the addition of new APIs tailored to specific application needs without modifying the core Kubernetes code. CRDs are essential for building operators and managing complex applications.
Key components of CRDs include:
- Spec: Defines the desired state of the resource.
- Status: Reflects the current state of the resource.
- Validation: Ensures the data conforms to specified schemas.
Monitoring Changes in Custom Resources
To notify users when a custom resource changes, Kubernetes provides several mechanisms. The primary method involves leveraging the Kubernetes API and its watch functionality, which allows clients to receive notifications about resource changes in real-time.
Watch Functionality
The watch feature in Kubernetes allows clients to subscribe to updates for specific resources. When a change occurs, a notification is sent to the client. This can be beneficial for custom resources managed via CRDs.
- Event Types: Notifications can include various event types such as `ADDED`, `MODIFIED`, and `DELETED`.
- Implementation: Using the Kubernetes client libraries (available for multiple programming languages), you can set up a watch on your custom resource.
Example Code Snippet
Here’s an example of how to set up a watch on a custom resource using Python:
“`python
from kubernetes import client, config, watch
config.load_kube_config()
v1 = client.CustomObjectsApi()
w = watch.Watch()
for event in w.stream(v1.list_cluster_custom_object, group=”mygroup.example.com”, version=”v1″, plural=”myresources”):
print(f’Event: {event[“type”]} – {event[“object”]}’)
“`
Using Informers for Change Notifications
Informers are higher-level abstractions built on top of the watch mechanism. They provide a more efficient way to manage and respond to changes in Kubernetes resources, including custom resources.
Benefits of Informers:
- Caching: Informers cache the state of resources to reduce the load on the API server.
- Event Handling: They provide a way to handle events through callback functions.
Sample Informer Implementation
Here is a simple example of how to create an informer for a custom resource:
“`python
from kubernetes import client, config, watch
from kubernetes.client import ApiClient
from kubernetes.client.rest import ApiException
config.load_kube_config()
v1 = client.CustomObjectsApi()
def on_add(obj):
print(f’Added: {obj}’)
def on_update(old_obj, new_obj):
print(f’Updated: {new_obj}’)
def on_delete(obj):
print(f’Deleted: {obj}’)
Set up an informer (pseudo-code)
informer = CustomResourceInformer(v1, “mygroup.example.com”, “v1”, “myresources”)
informer.on_add(on_add)
informer.on_update(on_update)
informer.on_delete(on_delete)
informer.start()
“`
Best Practices for Notification Systems
When implementing notifications for custom resource changes, consider the following best practices:
- Debouncing: Avoid overwhelming consumers with notifications by implementing a debounce mechanism.
- Filtering Events: Only notify about significant changes relevant to consumers to reduce noise.
- Logging: Keep logs of notifications for auditing and debugging purposes.
Event Type | Description |
---|---|
ADDED | A new custom resource has been created. |
MODIFIED | An existing custom resource has been updated. |
DELETED | A custom resource has been deleted. |
By applying these strategies, organizations can effectively monitor and respond to changes in Kubernetes custom resources, enhancing their operational efficiency and reliability.
Methods to Notify When a Custom Resource Changes
Kubernetes offers several approaches to monitor changes in custom resources. These methods can be tailored based on your specific use case and infrastructure.
Using Kubernetes Watch API
The Kubernetes API provides a watch mechanism that allows you to listen for changes to resources, including custom resources. This is typically done using the following steps:
- Establish a connection to the Kubernetes API server.
- Specify the resource you want to watch, which can include namespaces or specific resource types.
- Handle the events that the API server sends when changes occur.
Example code snippet in Go to watch a custom resource:
“`go
import (
“context”
“k8s.io/client-go/kubernetes”
“k8s.io/client-go/tools/clientcmd”
“k8s.io/apimachinery/pkg/watch”
)
func watchCustomResource() {
config, _ := clientcmd.BuildConfigFromFlags(“”, kubeconfigPath)
clientset, _ := kubernetes.NewForConfig(config)
watcher, _ := clientset.CustomResources(“default”).Watch(context.TODO())
for event := range watcher.ResultChan() {
switch event.Type {
case watch.Added:
// Handle added resource
case watch.Modified:
// Handle modified resource
case watch.Deleted:
// Handle deleted resource
}
}
}
“`
Using Kubernetes Operators
Operators are a powerful way to extend Kubernetes functionality. By creating an operator, you can automate the monitoring and response to changes in custom resources.
- Controller Logic: Implement a controller that watches for events related to your custom resource.
- Event Handling: Define specific actions to take when your resource changes, such as sending notifications or updating other resources.
Example of a simple controller structure:
“`yaml
apiVersion: apps/v1
kind: Deployment
metadata:
name: my-operator
spec:
replicas: 1
template:
spec:
containers:
- name: my-operator
image: my-operator-image
“`
Webhooks for Notifications
Integrating webhooks can facilitate notifications outside of the Kubernetes cluster. By setting up a webhook that listens for changes, you can trigger actions in external systems.
- Setup a Webhook: Define a service that listens for HTTP requests.
- Notification Logic: Implement logic to process the incoming requests and take appropriate actions, such as sending an email or an alert.
Using Third-Party Tools
Several tools can help monitor Kubernetes resources and notify you about changes:
Tool | Description |
---|---|
Prometheus | Monitors Kubernetes metrics and can alert on changes. |
ArgoCD | Continuous delivery tool that can monitor changes in GitOps. |
KubeEvents | A tool for managing events in a Kubernetes cluster. |
These tools often come with built-in notification systems that can integrate with messaging platforms like Slack, email, or custom webhooks.
Custom Scripts with CronJobs
For simpler use cases, consider using Kubernetes CronJobs to periodically check the state of custom resources.
- Script Logic: Write a script that queries the custom resource and checks for changes.
- CronJob Configuration: Set up a CronJob in Kubernetes to run your script at specified intervals.
Example CronJob configuration:
“`yaml
apiVersion: batch/v1
kind: CronJob
metadata:
name: custom-resource-check
spec:
schedule: “*/5 * * * *” Every 5 minutes
jobTemplate:
spec:
template:
spec:
containers:
- name: check-script
image: my-check-image
args: [“sh”, “-c”, “check-custom-resource.sh”]
restartPolicy: OnFailure
“`
Implementing these methods can ensure that you are promptly informed about any changes to your custom resources, enabling you to take immediate action as necessary.
Expert Insights on Monitoring Changes in Custom Kubernetes Resources
Dr. Emily Chen (Kubernetes Specialist, Cloud Innovations Inc.). “To effectively notify when a custom resource in Kubernetes changes, leveraging Kubernetes’ built-in watch functionality is essential. This allows developers to listen for changes in real-time, ensuring that any modifications trigger appropriate notifications or actions within the system.”
Michael Thompson (DevOps Engineer, Tech Solutions Group). “Implementing a custom controller that utilizes the Kubernetes API can provide a robust solution for monitoring changes in custom resources. By integrating this with a notification system, teams can achieve seamless alerts for any updates, enhancing operational awareness and responsiveness.”
Sarah Patel (Cloud Native Architect, FutureTech Labs). “Utilizing tools like Prometheus along with Alertmanager can significantly simplify the process of notifying changes in custom resources. By setting up appropriate metrics and alerting rules, organizations can ensure they are promptly informed of any critical changes that may impact their applications.”
Frequently Asked Questions (FAQs)
How can I monitor changes to a custom resource in Kubernetes?
You can monitor changes to a custom resource in Kubernetes by using the Kubernetes API to watch for events related to that resource. This can be done through client libraries available for various programming languages, or by using tools like `kubectl` with the `–watch` flag.
What tools can I use to get notified when a custom resource changes?
You can use tools like Kubernetes event exporters, Prometheus with Alertmanager, or custom controllers that implement the Kubernetes operator pattern to receive notifications when custom resources change.
Is it possible to set up webhooks for custom resource changes?
Yes, you can set up webhooks by creating a custom controller that listens for changes to your custom resource and triggers a webhook to notify an external service when a change occurs.
Can I use Kubernetes operators to manage notifications for custom resources?
Absolutely. Kubernetes operators can be designed to manage the lifecycle of custom resources and can include logic to send notifications whenever a resource changes state.
What programming languages are suitable for implementing notifications on custom resource changes?
Common programming languages for implementing notifications include Go, Python, and Java, as they have robust client libraries for interacting with the Kubernetes API and handling events.
Are there any built-in Kubernetes features for notifying changes in custom resources?
Kubernetes does not have built-in notification features specifically for custom resources. However, you can leverage the watch functionality of the API server to implement custom notification logic in your applications.
In Kubernetes, monitoring changes to custom resources is essential for maintaining system integrity and responsiveness. Custom resources extend the Kubernetes API, allowing users to define their own resource types that can be managed like built-in resources. To effectively notify users or systems when these custom resources change, various approaches can be employed, including the use of Kubernetes controllers, webhooks, and external monitoring tools.
One of the most effective methods for tracking changes is through the implementation of a Kubernetes controller that watches for events related to custom resources. This controller can be programmed to trigger notifications based on specific events, such as creation, updates, or deletions. Additionally, leveraging Kubernetes’ built-in event system allows for real-time notifications, which can be integrated with external systems for further processing or alerting.
Another approach involves using webhooks that can send HTTP callbacks to specified endpoints whenever a change occurs. This method is particularly useful for integrating with external systems or services that require immediate notification of changes. Furthermore, utilizing monitoring and observability tools that support Kubernetes can provide a comprehensive view of resource changes and facilitate alerting based on defined criteria.
In summary, effectively notifying stakeholders of changes to custom resources in Kubernetes involves a combination of leveraging Kubernetes’ native capabilities and integrating
Author Profile
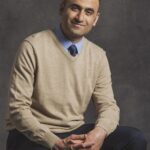
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?