How Can You Resolve the ‘npm ERR! Maximum Call Stack Size Exceeded’ Issue?
If you’ve ever encountered the dreaded `npm err maximum call stack size exceeded` message while working on a Node.js project, you know just how frustrating it can be. This error often feels like a cryptic riddle, halting your development process and leaving you scrambling for answers. Whether you’re a seasoned developer or a newcomer to the world of JavaScript, understanding the intricacies of this error is essential for smooth sailing in your coding journey. In this article, we’ll unravel the mystery behind this common npm error, explore its causes, and provide you with practical solutions to get your project back on track.
Overview
At its core, the `maximum call stack size exceeded` error signifies that your application has run into a recursion problem, where functions call themselves too many times without a proper exit condition. This can occur in various scenarios, from circular dependencies in your code to issues with your npm packages. As your project grows in complexity, so does the likelihood of encountering this frustrating error, making it crucial to understand its origins and implications.
In addition to exploring the technical aspects of this error, we will also discuss best practices for preventing it in the first place. By adopting certain coding techniques and npm management strategies, you can minimize the risk of running into this
Understanding the Error
The error message “maximum call stack size exceeded” typically indicates that a function is calling itself recursively without a proper base case, leading to an infinite loop. In the context of npm, this can occur during various operations, such as installing packages, running scripts, or resolving dependencies.
Common causes of this error include:
- Circular dependencies between packages.
- Incorrect or overly complex npm scripts.
- A corrupted node_modules directory.
Troubleshooting Steps
To resolve the “maximum call stack size exceeded” error in npm, follow these troubleshooting steps:
- Clear the npm cache: Sometimes, a corrupted cache can cause issues. Use the following command to clear it:
“`bash
npm cache clean –force
“`
- Delete node_modules and package-lock.json: Removing the existing node_modules directory and the package-lock.json file can help reset your environment:
“`bash
rm -rf node_modules package-lock.json
“`
- Reinstall dependencies: After clearing the cache and removing the directories, reinstall the dependencies:
“`bash
npm install
“`
- Check for circular dependencies: Inspect your package dependencies to ensure there are no circular references. Use tools like `madge` to visualize the dependency tree.
- Review npm scripts: Examine the scripts defined in your package.json file for any recursive calls that may not terminate properly.
Preventive Measures
To avoid encountering the “maximum call stack size exceeded” error in the future, consider the following best practices:
- Limit recursive function calls: If you must use recursion, ensure there is a clear base case to terminate the function.
- Use stable versions of packages: Avoid using pre-release versions of packages that may have unresolved issues.
- Regularly update npm and Node.js: Keeping your environment updated can help avoid bugs that have been fixed in newer releases.
Common Npm Commands and Their Uses
Understanding npm commands can help prevent issues:
Command | Description |
---|---|
npm install | Installs dependencies listed in package.json |
npm update | Updates the packages to their latest versions |
npm uninstall |
Removes a package from node_modules and package.json |
npm run | Executes a script defined in package.json |
By following these guidelines and utilizing the commands effectively, you can minimize the chances of encountering the "maximum call stack size exceeded" error while using npm.
Understanding the Error
The `maximum call stack size exceeded` error in npm typically arises when there is an infinite loop in the code or when a function calls itself too many times without a base case. This can lead to excessive memory usage, ultimately resulting in the stack overflow.
Common causes include:
- Circular dependencies in modules.
- Recursive function calls without proper termination.
- Issues in configuration files such as `package.json`.
Troubleshooting Steps
To resolve this issue, follow these troubleshooting steps:
- Examine Recent Changes: Review any recent code changes that may have introduced circular dependencies or excessive recursion.
- Check Dependencies: Run `npm ls` to inspect your project's dependency tree. Look for any circular dependencies or outdated packages that may cause conflicts.
- Increase Stack Size: Temporarily increase the stack size by running your command with a higher limit:
```bash
node --stack-size=8192 your_script.js
```
- Use Debugging Tools: Utilize debugging tools such as Chrome DevTools or Node Inspector to pinpoint where the recursion or loop occurs.
- Clear npm Cache: Sometimes, corrupted cache files can lead to issues. Clear the npm cache using:
```bash
npm cache clean --force
```
Common Fixes
Here are some practical fixes that may help resolve the error:
- Fix Circular Dependencies: Refactor your code to eliminate circular dependencies. This may involve restructuring how modules are imported.
- Limit Recursion: Ensure that any recursive function has a clear base case to prevent infinite calls.
- Update Dependencies: Regularly update your dependencies to their latest versions. Use:
```bash
npm outdated
npm update
```
- Check for Global Packages: If the error occurs when running global npm commands, check if any globally installed packages are causing the issue. You can list global packages with:
```bash
npm ls -g --depth=0
```
Example Code Analysis
Here is an example of problematic recursive code that could lead to this error:
```javascript
function recursiveFunction() {
return recursiveFunction();
}
```
In the above example, the function `recursiveFunction` calls itself indefinitely, leading to a stack overflow. To correct it, ensure there is a termination condition:
```javascript
function recursiveFunction(n) {
if (n <= 0) return;
return recursiveFunction(n - 1);
}
```
This version includes a base case that stops further calls when `n` reaches zero.
Preventive Measures
To avoid encountering this error in the future, consider the following practices:
- Code Reviews: Implement regular code reviews to catch potential circular dependencies early in the development process.
- Testing: Write unit tests for recursive functions to ensure that they behave correctly under all conditions.
- Monitoring Dependencies: Use tools like `npm-check-updates` to keep track of outdated packages and update them regularly.
- Documentation: Maintain clear documentation on module dependencies and the intended structure of your codebase to minimize confusion and errors.
By adhering to these practices, you can significantly reduce the likelihood of facing the `maximum call stack size exceeded` error in your npm projects.
Understanding the npm Error: Maximum Call Stack Size Exceeded
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The ‘maximum call stack size exceeded’ error in npm typically indicates a recursion issue or an infinite loop within your code. It is crucial to review your function calls and ensure that they have proper exit conditions to prevent this from happening.”
Mark Thompson (Lead Developer, Open Source Solutions). “This error can also arise from circular dependencies in your JavaScript modules. It is advisable to analyze your module imports and exports to eliminate any circular references that could lead to this stack overflow.”
Linda Nguyen (Technical Consultant, Code Quality Experts). “When encountering this npm error, one effective strategy is to increase the stack size using Node.js flags, such as `--stack-size`. However, this should be a temporary fix while you investigate the underlying code issues that are causing the stack overflow.”
Frequently Asked Questions (FAQs)
What does the error "maximum call stack size exceeded" mean in npm?
This error indicates that a function has exceeded the JavaScript call stack limit, typically due to excessive recursion or circular references in your code.
What are common causes of the "maximum call stack size exceeded" error in npm?
Common causes include circular dependencies between modules, excessive recursion in functions, or issues within npm scripts that lead to infinite loops.
How can I troubleshoot the "maximum call stack size exceeded" error?
Begin by reviewing the stack trace provided with the error message. Identify any recursive functions or circular dependencies. Use debugging tools or add console logs to trace the execution flow.
Can updating npm or Node.js resolve this error?
Yes, updating npm or Node.js can resolve the error if it is caused by bugs in the current version. Ensure you are using the latest stable versions to benefit from fixes and improvements.
Are there specific npm commands that are more prone to this error?
Yes, commands like `npm install` or `npm run` can trigger this error if your project has complex dependencies or incorrect scripts that lead to recursion.
What steps can I take to prevent the "maximum call stack size exceeded" error in the future?
To prevent this error, maintain clean code practices, avoid circular dependencies, limit recursion depth, and regularly update your npm packages to minimize compatibility issues.
The error message "npm ERR! Maximum call stack size exceeded" typically indicates that there is a recursion problem or an infinite loop occurring within the Node Package Manager (npm) process. This issue can arise during various operations, such as installing packages or running scripts, and is often linked to problems in the project's dependencies or configuration files. It is essential to identify the root cause of the error to resolve it effectively.
Common causes of this error include circular dependencies in the package.json file, excessive nesting of dependencies, or issues with specific npm scripts. Additionally, outdated npm versions or corrupted cache can contribute to the problem. To troubleshoot, developers can try clearing the npm cache, updating npm to the latest version, or examining the package.json file for any circular references or conflicts.
Key takeaways from addressing this error include the importance of maintaining a clean and organized project structure, as well as regularly updating dependencies to avoid compatibility issues. It is also advisable to utilize tools such as npm audit to identify vulnerabilities and potential conflicts within the project's dependencies. By following best practices and performing regular maintenance, developers can minimize the likelihood of encountering the "maximum call stack size exceeded" error in their npm workflows.
Author Profile
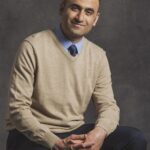
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?