Why Am I Seeing the Error ‘Object Literal May Only Specify Known Properties’ in My Code?
In the ever-evolving world of programming, developers often encounter a myriad of challenges that can trip them up, especially when working with object-oriented languages. One such hurdle is the error message: “object literal may only specify known properties.” This seemingly cryptic warning can leave even seasoned programmers scratching their heads. Understanding this error is crucial not only for debugging but also for mastering the nuances of type systems in languages like TypeScript and JavaScript. In this article, we will demystify this error, exploring its implications and offering insights into how to navigate it effectively.
Overview
At its core, the error “object literal may only specify known properties” arises when an object is defined with properties that are not recognized by the type system. This can happen in scenarios where strict type checking is enforced, leading to confusion for developers who may be accustomed to more lenient environments. The implications of this error extend beyond mere annoyance; they highlight the importance of understanding how object shapes and types interact within a programming language.
As we delve deeper into this topic, we will explore the common scenarios that trigger this error, the underlying principles of type safety, and the best practices for defining objects in a way that aligns with the expected structure. By equipping yourself with this knowledge,
Understanding Object Literals in TypeScript
In TypeScript, an object literal is a syntactic structure that allows you to create an object with specific properties. However, when you define an object using an object literal, TypeScript enforces strict checks on the properties that can be included. If you attempt to add properties that are not explicitly defined in the type or interface, you will encounter the error message: “object literal may only specify known properties.”
This behavior is crucial for maintaining type safety and ensuring that the structure of your data adheres to the expected format defined by your interfaces or types.
Common Causes of the Error
The “object literal may only specify known properties” error typically arises from a few common scenarios:
- Excess Properties: When additional properties are added to an object literal that are not defined in the corresponding type or interface.
- Typographical Errors: Misspelling property names can lead to TypeScript treating them as unknown properties.
- Incorrect Interface Usage: Using an interface that does not match the intended structure of the object can also trigger this error.
Example of the Error
Consider the following example:
“`typescript
interface User {
name: string;
age: number;
}
const user: User = {
name: “John Doe”,
age: 30,
email: “[email protected]” // This will cause an error
};
“`
In this case, the `email` property is not defined in the `User` interface, leading to the error message.
Preventing the Error
To avoid the “object literal may only specify known properties” error, consider the following best practices:
- Define Interfaces Accurately: Ensure that the interface accurately represents the structure of the object you intend to create.
- Use Type Assertions: If you need to include additional properties temporarily, you can use type assertions, but this should be done with caution.
“`typescript
const user = {
name: “John Doe”,
age: 30,
email: “[email protected]”
} as User; // Type assertion, but may lead to runtime issues
“`
- Strict Property Checking: Always enable strict property checking in your TypeScript configuration to catch these issues early.
Table of Error Scenarios
Scenario | Description | Resolution |
---|---|---|
Excess Properties | Adding properties not defined in an interface. | Remove or define the property in the interface. |
Misspelled Property | Typing errors in property names. | Check and correct the property names. |
Incorrect Interface | Using the wrong interface for the object structure. | Verify and use the correct interface. |
By following these guidelines, you can effectively manage object literals in TypeScript and minimize the occurrence of property-related errors.
Understanding Object Literals in TypeScript
In TypeScript, object literals are a way to create objects with specific properties. However, when defining an object literal, you may encounter the error message: “Object literal may only specify known properties.” This error indicates that the properties you are trying to define do not match the expected structure of the type you are assigning the object to.
Common Causes of the Error
The error typically arises from several common scenarios:
- Missing Properties: The object literal does not include all required properties defined in the type.
- Additional Properties: The object literal contains properties that are not defined in the type. TypeScript enforces strict typing, which means it checks the shape of the object against the defined type.
- Type Mismatches: A property in the object literal has a different type than what is expected.
Examples of Object Literal Errors
Consider the following examples illustrating these causes:
Example 1: Missing Properties
“`typescript
interface User {
id: number;
name: string;
}
const user: User = {
id: 1
// Error: Property ‘name’ is missing
};
“`
Example 2: Additional Properties
“`typescript
interface User {
id: number;
name: string;
}
const user: User = {
id: 1,
name: ‘Alice’,
age: 30 // Error: Object literal may only specify known properties
};
“`
Example 3: Type Mismatch
“`typescript
interface User {
id: number;
name: string;
}
const user: User = {
id: ‘1’, // Error: Type ‘string’ is not assignable to type ‘number’
name: ‘Alice’
};
“`
How to Resolve the Error
To resolve the “Object literal may only specify known properties” error, consider the following strategies:
- Ensure All Required Properties are Present: Check that your object includes all properties defined in the type.
- Remove Extra Properties: Eliminate any properties that are not part of the defined type.
- Correct Type Mismatches: Ensure that property values conform to the expected types.
Example of a Corrected Object Literal
“`typescript
interface User {
id: number;
name: string;
}
const user: User = {
id: 1,
name: ‘Alice’ // Now the object matches the User interface
};
“`
Using Type Assertions
In some scenarios, you may want to bypass TypeScript’s type checking. You can use type assertions, but this should be done cautiously:
“`typescript
const user = {
id: 1,
name: ‘Alice’,
age: 30
} as User; // Bypasses type checking but not recommended for type safety
“`
While this approach may resolve the error, it risks introducing runtime errors if the object does not conform to the expected structure.
TypeScript’s strict type checking promotes better coding practices by enforcing object shapes. Understanding how to construct object literals correctly is essential for avoiding common errors and ensuring type safety in your applications.
Understanding the Limitations of Object Literals in Programming
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The error message ‘object literal may only specify known properties’ commonly arises in TypeScript when developers attempt to assign properties to an object that are not defined in its type. This emphasizes the importance of adhering to strict type definitions to ensure code reliability and maintainability.”
Mark Thompson (Lead Developer, Code Quality Solutions). “In modern JavaScript frameworks, understanding the constraints of object literals is crucial. The warning about unknown properties serves as a reminder to developers to define their interfaces clearly, preventing runtime errors and enhancing code clarity.”
Linda Garcia (Technical Consultant, Software Development Strategies). “When encountering the ‘object literal may only specify known properties’ error, it is essential to review your object structure against the defined types. This practice not only improves code quality but also facilitates better collaboration among team members by ensuring everyone is on the same page regarding data structures.”
Frequently Asked Questions (FAQs)
What does the error “object literal may only specify known properties” mean?
This error indicates that an object literal is being created with properties that are not defined in the corresponding type or interface. TypeScript enforces type safety by ensuring that only known properties are included.
How can I resolve the “object literal may only specify known properties” error?
To resolve this error, ensure that all properties specified in the object literal match those defined in the type or interface. Remove any extraneous properties or update the type definition to include the new properties.
Can I use index signatures to avoid this error?
Yes, you can use index signatures in your type definitions to allow additional properties. This approach lets you define an object type that can have properties not explicitly listed, but it should be used judiciously to maintain type safety.
Does this error occur in JavaScript as well?
No, this error is specific to TypeScript. JavaScript does not enforce type checking, so it allows any properties to be added to object literals without restriction.
Are there any scenarios where this error is helpful?
Yes, this error helps maintain code quality by ensuring that object literals conform to defined types. It prevents runtime errors that may arise from using properties, promoting more robust and maintainable code.
What tools can help identify this error during development?
TypeScript’s built-in compiler will flag this error during development. Additionally, using integrated development environments (IDEs) like Visual Studio Code can provide real-time feedback and suggestions to resolve type-related issues.
The error message “object literal may only specify known properties” typically arises in TypeScript when an object is being defined with properties that are not recognized by the type definition associated with that object. This is a common issue encountered by developers when they attempt to create or manipulate objects without adhering to the defined interfaces or types. The TypeScript compiler enforces strict type checking, which ensures that only valid properties are included in object literals, thereby promoting type safety and reducing runtime errors.
Understanding this error is crucial for developers working with TypeScript, as it highlights the importance of defining and adhering to interfaces or types when creating objects. This practice not only helps in maintaining code quality but also enhances the readability and maintainability of the codebase. When developers encounter this error, it serves as a reminder to revisit the type definitions and ensure that all specified properties are valid and recognized by the corresponding type.
In summary, the “object literal may only specify known properties” error emphasizes the significance of type safety in TypeScript. Developers should be diligent in defining their object structures and ensuring compliance with type definitions. By doing so, they can avoid common pitfalls associated with type mismatches and enhance the robustness of their applications. Ultimately, this leads to a more efficient development
Author Profile
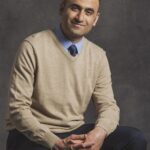
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?