Why Am I Getting ‘Object of Type Set is Not JSON Serializable’ Error?
In the world of programming, particularly when working with Python, developers often encounter various data types that serve different purposes. One common challenge arises when trying to convert complex data structures into a format suitable for transmission or storage—namely, JSON (JavaScript Object Notation). Among the myriad of data types, the `set` type can sometimes throw a wrench in the works, leading to the frustrating error message: “object of type set is not JSON serializable.” This conundrum not only puzzles novice programmers but can also stump seasoned developers. Understanding why this error occurs and how to resolve it is crucial for anyone looking to effectively serialize data in Python.
At its core, the issue stems from the fundamental differences between Python’s data structures and the JSON format. While JSON supports arrays and objects, it does not recognize sets, which can lead to serialization errors. This incompatibility can arise in various scenarios, such as when attempting to store user preferences, configurations, or any other data that might naturally be represented as a set. Recognizing the limitations of JSON serialization is the first step in overcoming these hurdles.
Fortunately, there are several strategies to address this issue, allowing developers to convert sets into a JSON-compatible format. By exploring the nuances of data serialization and the alternatives
Understanding JSON Serialization
JSON (JavaScript Object Notation) is a lightweight data interchange format that is easy for humans to read and write and easy for machines to parse and generate. However, not all Python objects can be directly serialized into JSON format. This is particularly true for complex data types such as sets.
In Python, the `json` module provides a straightforward way to convert Python objects into JSON format using the `json.dumps()` function. However, when attempting to serialize a set, you will encounter the error message: “Object of type set is not JSON serializable.”
This error arises because the JSON format does not have a direct equivalent for the set data type. Consequently, the JSON encoder does not know how to handle it.
Common Solutions for Serializing Sets
To resolve the issue of sets not being JSON serializable, you can convert the set to a list or another serializable type before attempting to serialize it. Here are some common approaches:
- Convert to List: A straightforward method is to convert the set to a list, which is JSON serializable.
“`python
import json
my_set = {1, 2, 3}
json_data = json.dumps(list(my_set))
“`
- Convert to Dictionary: If the set contains unique values that serve as keys or can be paired with some values, converting it to a dictionary might be suitable.
“`python
my_set = {1, 2, 3}
json_data = json.dumps({x: None for x in my_set})
“`
- Custom Serialization: If you frequently need to serialize sets, consider creating a custom serialization function.
“`python
def serialize_set(obj):
if isinstance(obj, set):
return list(obj)
raise TypeError(f’Object of type {obj.__class__.__name__} is not JSON serializable’)
json_data = json.dumps(my_set, default=serialize_set)
“`
Example of Serializing Different Data Types
To better illustrate the serialization process, the following table presents various Python data types and their JSON serializability status:
Python Data Type | JSON Serializable |
---|---|
dict | Yes |
list | Yes |
tuple | Yes (converted to list) |
set | No |
str | Yes |
int | Yes |
float | Yes |
None | Yes (null) |
By employing these strategies, you can effectively handle sets and other non-serializable types when working with JSON in Python. This ensures that your data can be transmitted and stored in a format that is widely accepted and understood.
Understanding the Error
The error message “object of type set is not JSON serializable” typically arises in Python when attempting to convert a set data structure into JSON format using the `json` module. The `json` module can serialize several built-in types, but it does not support the `set` type directly. This limitation is important to understand when working with data serialization in Python.
Common Scenarios Leading to the Error
Several scenarios may lead to encountering this error:
- Direct Serialization: Attempting to serialize a set directly.
- Nested Data Structures: Using sets within lists or dictionaries that are being serialized.
- Returning Set from Functions: Functions returning a set that is subsequently serialized.
How to Resolve the Error
To resolve the “object of type set is not JSON serializable” error, consider the following approaches:
- Convert Set to List: The simplest solution is to convert the set to a list before serialization.
“`python
import json
my_set = {1, 2, 3}
json_data = json.dumps(list(my_set))
“`
- Custom Serialization: For more complex objects, you can define a custom serialization method.
“`python
import json
def serialize(obj):
if isinstance(obj, set):
return list(obj)
raise TypeError(f”Type {type(obj)} not serializable”)
my_set = {1, 2, 3}
json_data = json.dumps(my_set, default=serialize)
“`
- Using `default` Parameter: The `json.dumps()` function allows for a `default` parameter, which can be used to specify a function for handling unsupported types.
Example Code Snippet
Here’s a more comprehensive example demonstrating these solutions:
“`python
import json
def serialize(obj):
if isinstance(obj, set):
return list(obj)
raise TypeError(f”Type {type(obj)} not serializable”)
data = {
“name”: “Alice”,
“age”: 30,
“hobbies”: {“reading”, “hiking”, “coding”}
}
Attempting to serialize directly will fail
try:
json_data = json.dumps(data)
except TypeError as e:
print(e)
Correctly serialize using the custom function
json_data = json.dumps(data, default=serialize)
print(json_data)
“`
Best Practices for JSON Serialization
When working with JSON serialization in Python, adhere to the following best practices:
- Use Supported Types: Stick to JSON-supported types such as dict, list, str, int, float, and bool.
- Avoid Sets: If using sets, convert them to lists before serialization.
- Custom Encoder Classes: For projects requiring extensive custom serialization, consider implementing a subclass of `json.JSONEncoder`.
Supported Types | Unsupported Types |
---|---|
dict | set |
list | complex |
str | custom classes |
int | |
float | |
bool |
By following these practices, you can minimize serialization errors and ensure compatibility with JSON standards.
Understanding JSON Serialization Issues with Sets
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The error ‘object of type set is not JSON serializable’ arises because the JSON format does not support Python’s set data type. To resolve this, one should convert the set to a list before serialization, as lists are compatible with JSON.”
Mark Thompson (Data Scientist, Analytics Solutions Group). “When encountering the ‘object of type set is not JSON serializable’ error, it is crucial to understand that JSON serialization is limited to certain data types. Utilizing the built-in functions to convert sets to lists or dictionaries can effectively circumvent this issue.”
Linda Garcia (Lead Developer, CodeCraft Technologies). “To avoid the common pitfall of ‘object of type set is not JSON serializable’, developers should implement custom serialization methods that handle sets appropriately, ensuring that data structures are compatible with JSON standards.”
Frequently Asked Questions (FAQs)
What does “object of type set is not JSON serializable” mean?
This error indicates that you are trying to convert a Python set object into a JSON format, which is not supported by the built-in JSON encoder in Python. JSON supports data types like lists, dictionaries, strings, numbers, and booleans, but not sets.
How can I convert a set to a JSON serializable format?
To convert a set to a JSON serializable format, you can convert the set to a list using the `list()` function. For example, `json.dumps(list(my_set))` will successfully serialize the data.
What are common scenarios that lead to this error?
Common scenarios include attempting to serialize a set directly when using `json.dumps()` or when a set is included as part of a larger data structure, such as a dictionary, that is being serialized.
Can I use other data structures instead of sets?
Yes, you can use lists or dictionaries instead of sets if you need to serialize the data to JSON. Lists are ordered and allow duplicates, while dictionaries store key-value pairs.
Is there a way to customize JSON serialization for sets?
Yes, you can create a custom JSON encoder by subclassing `json.JSONEncoder` and overriding the `default` method to handle sets specifically, allowing you to define how sets should be converted to a JSON-compatible format.
What libraries can help with JSON serialization of complex data types?
Libraries such as `simplejson` or `marshmallow` provide enhanced capabilities for JSON serialization and deserialization, allowing for better handling of complex data types, including sets and other non-standard types.
The error message “object of type set is not JSON serializable” typically arises in Python when attempting to convert a set data structure into a JSON format using the `json` module. JSON, or JavaScript Object Notation, is a widely used data interchange format that only supports certain data types, including dictionaries, lists, strings, numbers, and booleans. However, it does not natively support sets, which can lead to this serialization issue.
To resolve this error, developers must convert the set into a compatible data type before serialization. A common approach is to transform the set into a list using the `list()` function. This conversion allows the data to be serialized successfully, as lists are supported by the JSON format. It is essential to ensure that any data structure being serialized adheres to the limitations of JSON to avoid similar issues in the future.
Additionally, understanding the underlying reasons for this error can enhance a developer’s ability to work effectively with JSON in Python. It emphasizes the importance of data type compatibility when dealing with serialization processes. By being mindful of the data structures used in applications, developers can prevent errors and ensure smooth data interchange between systems.
Author Profile
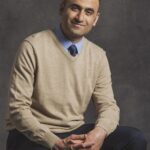
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?