Why Am I Getting ‘Object Variable with Block Variable Not Set’ Error?
In the world of programming, encountering errors is an inevitable part of the development process. Among these, the error message “Object variable or With block variable not set” is one that often leaves developers scratching their heads. This cryptic warning, commonly associated with Visual Basic for Applications (VBA), can halt your code in its tracks, leading to frustration and wasted time. But fear not! Understanding the nuances of this error can empower you to troubleshoot and resolve issues with confidence, ultimately enhancing your coding skills and efficiency.
When you see this error message, it typically indicates that your code is trying to reference an object that hasn’t been properly instantiated or assigned. This can occur in various scenarios, such as when working with object variables, collections, or even when using the With statement in your code. The implications of this error can range from minor annoyances to significant roadblocks in your projects, making it crucial for programmers to grasp the underlying concepts that lead to this issue.
In this article, we will delve into the common causes of the “Object variable or With block variable not set” error, exploring how to identify and rectify the problem. We will also discuss best practices for managing object variables in your code to prevent this error from occurring in the first place. Whether you’re
Understanding the Error
The error message “Object variable or With block variable not set” commonly occurs in programming environments that utilize object-oriented concepts, particularly in languages like VBA (Visual Basic for Applications). This error indicates that a variable is being referenced without being properly initialized or set to an object instance.
In object-oriented programming, an object variable must point to an actual object before you can access its properties or methods. When this is not the case, the interpreter or compiler raises this error.
Common Causes
Several scenarios can lead to this error:
- Uninitialized Variables: When an object variable is declared but not instantiated.
- Out of Scope: When an object variable that was initialized goes out of scope.
- Incorrect Object Assignment: Attempting to assign a value to an object variable that does not support that operation.
How to Resolve the Error
To resolve the “Object variable or With block variable not set” error, consider the following strategies:
- Ensure Proper Initialization: Always initialize your object variables before use.
“`vba
Dim obj As Object
Set obj = New SomeObject
“`
- Check Object References: Verify that object references are valid and have been correctly assigned.
“`vba
If Not obj Is Nothing Then
‘ Proceed with using obj
End If
“`
- Scope Management: Keep track of the variable’s scope and ensure it remains valid throughout its intended use.
- Using With Statements: When using With statements, ensure that the object being referenced is properly initialized.
“`vba
With obj
‘ Ensure obj is set before this point
End With
“`
Example Code Snippet
Here is a simple example demonstrating how to correctly initialize and use an object variable:
“`vba
Dim workbook As Workbook
Set workbook = Workbooks.Open(“C:\Path\To\File.xlsx”)
If Not workbook Is Nothing Then
‘ Access workbook properties
MsgBox workbook.Name
End If
“`
Best Practices
To avoid encountering this error, adhere to the following best practices:
- Always initialize object variables.
- Use error handling to manage unexpected situations.
- Regularly check for `Nothing` before accessing object properties or methods.
- Encapsulate object creation in functions to maintain clarity and control.
Cause | Resolution |
---|---|
Uninitialized Variable | Ensure to instantiate the variable using `Set`. |
Out of Scope | Check variable scope and lifetime. |
Incorrect Assignment | Verify object compatibility and correct assignment. |
By following these guidelines, you can effectively manage object variables, reducing the likelihood of encountering the “Object variable or With block variable not set” error during development.
Understanding the Error
The error message “object variable or with block variable not set” typically occurs in Visual Basic for Applications (VBA) or similar environments when an object reference is not properly initialized. This can lead to runtime errors, causing the code to fail unexpectedly. The error often stems from attempts to access properties or methods of an object that hasn’t been instantiated or assigned.
Common Causes
Several factors can trigger this error:
- Uninitialized Object: Attempting to use an object variable that hasn’t been set using the `Set` keyword.
- Out of Scope Variables: Using an object variable that has gone out of scope, often due to the completion of a procedure or function.
- Improper Object Assignment: Assigning a non-object value to an object variable or failing to retrieve an object correctly from a collection.
- Late Binding Issues: Issues arising from late binding where the object type is not explicitly defined.
Examples of the Error
Here are some common scenarios where this error may arise:
Scenario | Example Code | Explanation |
---|---|---|
Uninitialized Object | `Dim obj As Object` `obj.Method` |
`obj` was declared but not set to any object, leading to an error. |
Out of Scope Variable | `Dim obj As Object` `Set obj = New Class` `End Sub` `obj.Method` |
Trying to access `obj` outside of its defined scope. |
Improper Object Assignment | `Dim obj As Object` `obj = “string”` |
Assigning a string to an object variable results in an error. |
Late Binding Issues | `Dim obj As Object` `Set obj = CreateObject(“Excel.Application”)` `obj.Visible` |
If the object cannot be created, any method call will fail. |
Troubleshooting Steps
To resolve the “object variable or with block variable not set” error, follow these troubleshooting steps:
- Check Initialization: Ensure all object variables are initialized before use.
- Use `Set` Keyword: Always use `Set` when assigning object references.
- Scope Management: Verify that the object variable is within scope when accessed.
- Debugging Tools: Utilize debugging tools to step through the code and identify where the object fails to set.
- Error Handling: Implement error handling to gracefully manage potential runtime errors.
Best Practices
To avoid encountering this error, consider the following best practices:
- Explicit Object Types: Declare object variables with explicit types whenever possible to improve readability and reduce errors.
- Initialize Objects: Always initialize your objects before accessing their properties or methods.
- Clear Object References: Set object variables to `Nothing` after use to release resources and prevent memory leaks.
- Comment Your Code: Use comments to clarify the purpose of object variables, especially in complex procedures.
Understanding the nuances of object management in VBA is crucial for effective programming. By adhering to best practices and employing diligent debugging strategies, developers can minimize the occurrence of “object variable or with block variable not set” errors, leading to more robust and reliable code.
Understanding the ‘Object Variable with Block Variable Not Set’ Error in Programming
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The ‘Object variable with block variable not set’ error typically arises in Visual Basic when an object is referenced before it has been instantiated. It is crucial to ensure that all objects are properly initialized before use, which can often be achieved through rigorous debugging practices.”
Michael Chen (Lead Developer, CodeCraft Solutions). “This error serves as a reminder of the importance of scope and object lifetime in programming. Developers should be vigilant about the context in which objects are created and accessed, as failing to do so can lead to runtime errors that disrupt application flow.”
Sarah Thompson (Technical Consultant, Software Quality Assurance Group). “Addressing the ‘Object variable with block variable not set’ issue often involves reviewing the code for proper object instantiation. Implementing error handling can also provide insights into where the failure occurs, allowing for more robust application development.”
Frequently Asked Questions (FAQs)
What does “object variable with block variable not set” mean?
This error typically occurs in programming environments like Visual Basic when a variable that is expected to reference an object is not initialized or set to an object instance.
What are common causes of the “object variable with block variable not set” error?
Common causes include attempting to access properties or methods of an object that hasn’t been instantiated, or using a variable that has gone out of scope or has not been assigned a value.
How can I resolve the “object variable with block variable not set” error?
To resolve this error, ensure that all object variables are properly initialized before use. Check for any missing `Set` statements or ensure that the object is created using the appropriate constructor.
What is the difference between an object variable and a block variable?
An object variable is a reference to an object instance, while a block variable is a local variable defined within a block of code, such as a loop or conditional statement. Object variables can persist beyond the block if not declared locally.
Can this error occur in other programming languages?
Yes, similar errors can occur in other programming languages, often with different terminology. For instance, in languages like Cor Java, it may manifest as a null reference exception when attempting to access a method or property of a null object.
Are there any best practices to avoid this error?
Best practices include always initializing object variables, using error handling to manage potential null references, and following coding conventions that promote clear variable scope and lifecycle management.
The phrase “object variable with block variable not set” typically refers to a runtime error encountered in programming environments such as Visual Basic for Applications (VBA). This error occurs when a code attempts to use an object that has not been properly initialized or assigned. The term “block variable” signifies that the variable is defined within a specific block of code, and if it has not been set to reference an object, any attempt to access its properties or methods will result in an error. Understanding this error is crucial for debugging and ensuring that code runs smoothly.
One of the primary causes of this error is the failure to instantiate an object before its use. Developers must ensure that all object variables are assigned to a valid instance of an object. This can be achieved by using the ‘Set’ keyword in VBA when assigning an object reference. Additionally, programmers should implement error handling techniques to gracefully manage situations where an object might not be set, thus preventing abrupt crashes and improving user experience.
Another key takeaway is the importance of scope and lifetime of variables in programming. Block variables exist only within the confines of the block in which they are declared. Therefore, if an object variable is declared within a block and is attempted to be accessed outside of that block without
Author Profile
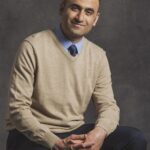
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?