How Can I Identify an Unknown C Struct Type in Objective-C?
In the ever-evolving landscape of programming languages, Objective-C stands out as a powerful tool for developing applications on Apple’s platforms. However, as developers dive deeper into the intricacies of this language, they often encounter peculiar challenges that can leave them scratching their heads. One such conundrum is the infamous “unknown C struct type” error, a cryptic message that can disrupt the flow of even the most seasoned programmers. Understanding the nuances of this error not only enhances your coding skills but also opens the door to mastering more complex data structures in Objective-C.
As we explore the realm of Objective-C, we will unravel the mystery behind unknown C struct types, shedding light on the common pitfalls that lead to this frustrating issue. This article will guide you through the fundamental concepts of C structs, their integration with Objective-C, and the scenarios that typically trigger such errors. By gaining insight into the underlying causes, you will be better equipped to tackle these challenges head-on, ensuring a smoother development experience.
Moreover, we will delve into best practices for defining and using structs in Objective-C, providing you with the tools to avoid potential errors and enhance your code’s robustness. Whether you’re a novice programmer or a seasoned developer looking to refine your skills, understanding how to navigate the complexities
Understanding Unknown C Struct Types in Objective-C
When working with Objective-C, it is not uncommon to encounter situations where you need to interact with C structs. If you find yourself facing an “unknown C struct type” error, it typically indicates that the compiler cannot recognize the struct type you are trying to use. This may happen for several reasons, including missing definitions or improper scope.
To effectively manage C structs in Objective-C, consider the following key points:
- Struct Definition: Ensure that the struct is properly defined before you attempt to use it. A struct definition should look like this:
“`c
typedef struct {
int x;
int y;
} Point;
“`
- Include Headers: If the struct is defined in a separate header file, make sure to include that header at the top of your Objective-C file:
“`objective-c
import “Point.h”
“`
- Scope and Visibility: Verify that the struct is in the correct scope. If the struct is defined within a function, it will not be accessible outside of that function.
- Forward Declarations: If you are using pointers to structs without defining the struct completely, consider using forward declarations:
“`c
typedef struct Point Point; // Forward declaration
struct Point {
int x;
int y;
};
“`
Common Causes of “Unknown C Struct Type” Errors
Understanding the causes of these errors can help in troubleshooting:
- Typographical Errors: A simple misspelling of the struct type name can lead to confusion. Double-check the struct name in your code.
- Improper Typedef Usage: If you have defined a typedef for your struct, ensure you are using the typedef name and not the struct tag name.
- Circular Dependencies: If your code structure leads to circular dependencies, the compiler might not recognize the struct definition when needed.
- Compiler Settings: Ensure that your project settings are configured correctly to recognize C code, especially if you are mixing C and Objective-C.
Example of Struct Usage in Objective-C
Below is a simple example that demonstrates how to define and use a C struct in Objective-C:
“`objective-c
import
typedef struct {
int width;
int height;
} Rectangle;
int main(int argc, const char * argv[]) {
@autoreleasepool {
Rectangle rect;
rect.width = 10;
rect.height = 20;
NSLog(@”Rectangle width: %d, height: %d”, rect.width, rect.height);
}
return 0;
}
“`
In this example, a struct `Rectangle` is defined and used within the `main` function. The values are assigned and logged to the console, demonstrating proper usage without triggering any “unknown C struct type” errors.
Best Practices for Managing C Structs in Objective-C
To avoid common pitfalls and ensure smooth integration between C structs and Objective-C, adhere to the following best practices:
- Keep Structs Simple: Limit the complexity of your structs. Avoid nesting structs or including pointers to Objective-C objects within structs.
- Documentation: Clearly document your structs, including their purpose and usage, to enhance code maintainability.
- Consistency: Be consistent in naming conventions and struct usage throughout your codebase.
- Testing and Validation: Regularly test your code to catch any issues related to struct usage early in the development process.
Best Practice | Description |
---|---|
Keep Structs Simple | Avoid unnecessary complexity in struct definitions. |
Documentation | Provide clear comments on struct definitions and their intended use. |
Consistency | Use uniform naming conventions across your codebase. |
Testing and Validation | Implement regular testing to identify issues early. |
Understanding Unknown C Struct Types in Objective-C
When working with Objective-C, you may encounter scenarios where you need to utilize C structs that are not explicitly defined in your codebase. This can lead to confusion regarding the type and structure of these unknown C structs. The following details how to effectively manage and utilize unknown C structs within Objective-C.
Identifying Unknown C Struct Types
To work with unknown C struct types, you can use several strategies:
- Check Headers: Often, struct definitions are found in header files. Look for relevant `.h` files that may declare the struct.
- Compiler Errors: Pay attention to compiler error messages which may provide hints about the struct’s expected type or members.
- Debugging Tools: Utilize debugging tools such as LLDB or Xcode’s built-in debugger to inspect memory and identify struct layouts.
Defining C Structs in Objective-C
If you determine the structure of the unknown C struct, you can define it in your Objective-C code. Here’s how to define a simple struct:
“`objc
typedef struct {
int id;
float value;
char name[50];
} MyStruct;
“`
You can then declare variables of this struct type and use them within your Objective-C methods.
Interfacing C Structs with Objective-C
When interfacing C structs with Objective-C classes, consider the following points:
- Objective-C Properties: You can encapsulate C structs within Objective-C classes by defining properties:
“`objc
@interface MyClass : NSObject
@property (nonatomic) MyStruct myStruct;
@end
“`
- Initialization: Ensure you initialize structs properly before use. For example:
“`objc
MyStruct myStructInstance;
myStructInstance.id = 1;
myStructInstance.value = 3.14;
strcpy(myStructInstance.name, “Example”);
“`
Handling Struct Pointers
When dealing with pointers to C structs, you can manipulate memory more efficiently. Here’s how you can do this:
– **Allocating Memory**: Use `malloc` to allocate memory for a struct pointer:
“`objc
MyStruct *myStructPtr = (MyStruct *)malloc(sizeof(MyStruct));
myStructPtr->id = 2;
myStructPtr->value = 2.71;
strcpy(myStructPtr->name, “Pointer Example”);
“`
- Freeing Memory: Always free allocated memory to prevent leaks:
“`objc
free(myStructPtr);
“`
Common Pitfalls and Solutions
When working with unknown C structs in Objective-C, be aware of these common issues:
Issue | Solution |
---|---|
struct type | Ensure the struct is defined in an included header. |
Incorrect memory allocation | Check size calculations when using `malloc`. |
Memory leaks | Always pair `malloc` with `free`. |
Type mismatches | Use `void *` for generic pointers and cast accordingly. |
By understanding how to identify, define, and manipulate unknown C struct types in Objective-C, you can enhance your ability to manage complex data structures effectively.
Understanding Objective-C and Unknown C Struct Types
Dr. Emily Chen (Senior Software Engineer, Tech Innovations Inc.). “When encountering an ‘unknown C struct type’ in Objective-C, it is crucial to ensure that the struct is properly defined and included in the relevant header files. Often, this issue arises from missing imports or incorrect visibility of the struct definition, which can lead to compilation errors.”
Mark Thompson (Lead iOS Developer, Mobile Solutions Group). “In Objective-C, the integration of C structs can be tricky, especially when dealing with complex data types. Developers should verify that the struct is not only declared but also that its memory layout is compatible with Objective-C’s object model, as this can lead to unexpected behaviors.”
Sarah Patel (Technical Consultant, CodeCraft Consulting). “To resolve issues related to unknown C struct types in Objective-C, I recommend utilizing the Clang compiler’s diagnostic tools. These tools can provide insights into type definitions and help identify where the struct might be going out of scope or not being recognized.”
Frequently Asked Questions (FAQs)
What does “unknown C struct type” mean in Objective-C?
The “unknown C struct type” error occurs when the compiler encounters a struct that has not been defined or declared in the current scope. This typically happens when the struct definition is missing or not properly imported.
How can I resolve the “unknown C struct type” error in my Objective-C code?
To resolve this error, ensure that the struct is defined before it is used. Include the necessary header files where the struct is defined, or define the struct in the same file if appropriate.
Are there specific scenarios where this error commonly arises?
This error commonly arises when using third-party libraries, where the struct is defined in a separate header file that has not been imported. It can also occur when there is a typo in the struct name or when the struct is defined within a conditional compilation block that is not active.
Can I use forward declarations for structs in Objective-C?
Yes, you can use forward declarations for structs in Objective-C. This allows you to declare a struct type without defining its members, enabling you to reference it in function signatures or other declarations before its full definition.
Is there a difference between C structs and Objective-C classes regarding this error?
Yes, C structs are defined using a different syntax and do not have the same object-oriented features as Objective-C classes. The “unknown C struct type” error specifically pertains to C structs, while Objective-C classes would typically generate different types of errors if not properly defined or imported.
What best practices can I follow to avoid the “unknown C struct type” error?
To avoid this error, always ensure that struct definitions are included in the appropriate header files and imported correctly. Maintain clear organization of your code and use consistent naming conventions to minimize the risk of typos and other issues.
In Objective-C, encountering an “unknown C struct type” error typically indicates that the compiler cannot recognize a struct definition at the point where it is being referenced. This situation often arises due to issues such as missing header files, improper forward declarations, or scope-related problems. Structs in C and Objective-C must be defined before they are used, and any attempt to reference a struct that has not been declared will lead to this error.
To resolve this issue, it is essential to ensure that all relevant struct definitions are included in the appropriate header files. Developers should also verify that the struct is declared in the correct scope, particularly when dealing with nested structs or when using them across different files. Utilizing forward declarations can help in certain scenarios, but it is crucial to follow up with a complete definition before any usage.
Moreover, understanding the organization of code and the compilation process in Objective-C can aid in preventing such errors. Properly structuring code, including header files, and maintaining clear dependencies between files can significantly reduce the likelihood of encountering unknown struct types. By adhering to these best practices, developers can enhance code maintainability and minimize compilation errors.
Author Profile
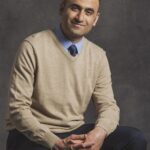
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?