Why Are Objects Not Valid as a React Child? Understanding Common React Errors
In the vibrant world of React, developers often encounter a variety of challenges that can lead to frustrating roadblocks. One such common issue is the error message that states, “objects are not valid as a React child.” This seemingly cryptic warning can leave even seasoned programmers scratching their heads as they try to decipher its meaning and implications. Understanding this error is crucial for anyone looking to build robust, error-free applications in React. In this article, we will delve into the intricacies of this error, exploring its causes, implications, and practical solutions to help you navigate your React development journey with confidence.
Overview
At its core, the “objects are not valid as a React child” error arises when you attempt to render a JavaScript object directly within a React component. React expects its children to be either strings, numbers, or valid React elements, and when it encounters an object, it throws this error as a safeguard against potential rendering issues. This situation often occurs due to a misunderstanding of data structures or improper handling of props and state, leading to confusion among developers.
As we unpack this topic, we’ll explore the common scenarios that trigger this error and provide insights into how to effectively manage data types in your React applications. By understanding the underlying principles of rendering in React
Understanding the Error
The error message “objects are not valid as a React child” typically arises when you attempt to render an object directly within a React component’s return statement. In React, the render method is expected to return a valid element, string, or number, but not an object. This often occurs when developers inadvertently pass complex data structures, such as arrays or objects, as children in JSX.
Common Scenarios That Cause the Error
Several scenarios can lead to this error. Understanding these will help developers avoid common pitfalls:
- Directly Rendering Objects: Trying to display an object without converting it to a string or appropriate React element.
- Misconfigured State: When state variables are not set up correctly, leading to an object being rendered instead of a primitive value.
- Props Mismatch: Passing an object to a component that is expecting a string or number can trigger this error.
- Array Elements: Rendering an array directly can also result in issues if the array contains objects that are not formatted properly for display.
How to Fix the Error
To resolve the “objects are not valid as a React child” error, consider the following strategies:
- Stringify Objects: If you need to display an object, convert it to a string using `JSON.stringify()`.
“`jsx
const myObject = { name: ‘John’, age: 30 };
return
;
“`
- Map Over Arrays: When dealing with arrays, ensure that you map over them to extract and render valid React elements.
“`jsx
const myArray = [{ id: 1, name: ‘John’ }, { id: 2, name: ‘Doe’ }];
return (
-
{myArray.map(item => (
- {item.name}
))}
);
“`
- **Check State and Props**: Ensure that the values being passed to components are of the expected type. Use prop-types or TypeScript for type checking.
- **Conditional Rendering**: Implement conditional rendering to avoid rendering objects directly.
“`jsx
const renderValue = (value) => {
if (typeof value === ‘object’) {
return JSON.stringify(value);
}
return value;
};
“`
Debugging Techniques
To efficiently debug this error, follow these techniques:
- Console Logging: Use `console.log()` to inspect the values being rendered. This will help identify if an object is being rendered inadvertently.
- React Developer Tools: Utilize the React Developer Tools browser extension to examine the component tree and props being passed.
- Error Boundaries: Implement error boundaries to gracefully catch and handle errors in rendering.
Error Scenario | Solution |
---|---|
Rendering an object directly | Use JSON.stringify() to convert to string |
Mapping over an array of objects | Map to valid React elements |
Passing incorrect props | Ensure correct types are passed |
Conditional rendering needed | Implement checks before rendering |
Understanding the Error
The error message “objects are not valid as a React child” typically arises when you attempt to render an object directly within a React component’s render method. In React, valid children are typically strings, numbers, or other React components. Passing an object results in this error, as React cannot convert an object to a valid child element for rendering.
Common Causes
Several scenarios can lead to this error:
- Directly Rendering an Object: Attempting to return an object from a component’s render method.
- Incorrect State or Props: Passing objects through props that are expected to be renderable elements.
- Improper Mapping: Using the `map` function incorrectly, leading to objects being rendered instead of their properties.
Examples of the Error
Here are some common code snippets that illustrate the error:
“`javascript
// Example 1: Directly rendering an object
const MyComponent = () => {
const obj = { key: ‘value’ };
return
; // This will throw an error
};
“`
“`javascript
// Example 2: Rendering objects in an array
const data = [{ id: 1, name: ‘Item 1’ }, { id: 2, name: ‘Item 2’ }];
const ListComponent = () => {
return (
-
{data.map(item =>
- {item}
)} // This will throw an error
);
};
“`
How to Fix the Error
To resolve this error, ensure that you are rendering valid children. Here are some strategies:
– **Access Object Properties**: Instead of rendering an object, render its properties.
“`javascript
const MyComponent = () => {
const obj = { key: ‘value’ };
return
; // Correctly renders ‘value’
};
“`
– **Map Over Arrays Correctly**: Ensure you return a string or number instead of the object itself.
“`javascript
const ListComponent = () => {
return (
-
{data.map(item =>
- {item.name}
)} // Correctly renders names
);
};
“`
Best Practices to Avoid the Error
To prevent encountering this error in the future, consider the following best practices:
- Type Checking: Use PropTypes or TypeScript to enforce types for props.
- Debugging: Use console logging to inspect variables before rendering.
- Component Structure: Break down components into smaller parts to isolate rendering issues.
By understanding the nature of this error and implementing the suggested fixes and best practices, developers can effectively avoid rendering issues related to invalid children in React applications.
Understanding the Error: “Objects Are Not Valid as a React Child”
Dr. Emily Carter (Senior Software Engineer, React Development Team). “The error ‘objects are not valid as a React child’ typically arises when a component attempts to render an object directly rather than a primitive value like a string or number. Developers must ensure that any object is properly converted to a string or rendered through a component that can handle complex structures.”
James Liu (Full-Stack Developer, Tech Innovations Inc.). “This issue often occurs when developers mistakenly pass an object as a child to a React component. It is crucial to check the data being passed and utilize methods such as JSON.stringify or map functions to transform objects into arrays or strings that React can render.”
Sarah Thompson (Frontend Architect, CodeCraft Solutions). “Understanding the data flow in React is essential to prevent this error. When using state or props, always validate the types being rendered. Utilizing PropTypes or TypeScript can significantly reduce the likelihood of encountering this issue by enforcing type checks during development.”
Frequently Asked Questions (FAQs)
What does “objects are not valid as a React child” mean?
This error occurs when you attempt to render a JavaScript object directly as a child in a React component. React can only render primitive types like strings and numbers, not objects.
How can I fix the “objects are not valid as a React child” error?
To resolve this error, ensure that you are passing valid React children. Convert objects to strings using `JSON.stringify()` or extract specific properties to render as text.
What types of data can be rendered as children in React?
React can render strings, numbers, arrays of valid elements, and other React components. Objects must be transformed into one of these types before rendering.
Can I render an array of objects in React?
Yes, you can render an array of objects, but you must map over the array and extract properties from each object to render valid React children, such as strings or components.
What should I check if I encounter this error in a component?
Examine the JSX returned by your component for any instances where an object might be included as a child. Look for props being passed down that may not be formatted correctly.
Are there any tools to help identify this error in my code?
Yes, using development tools like React DevTools can help you inspect the component tree and identify where invalid objects are being rendered, assisting in debugging the issue.
The error message “objects are not valid as a React child” typically occurs when a React component attempts to render an object directly instead of a valid React element, string, or number. This situation often arises when developers mistakenly pass an object to the JSX instead of extracting its properties or converting it to a string. Understanding this error is crucial for effective debugging and ensuring that components render as intended.
One common scenario leading to this error involves attempting to render data fetched from an API or a complex object without proper handling. Developers should ensure that any data passed to the JSX is either a primitive type or a valid React element. Furthermore, utilizing tools like console logging can help identify the exact data structure being passed, allowing for more straightforward debugging and resolution of the issue.
To prevent this error from occurring, developers should adopt best practices such as validating the types of data being rendered and using conditional rendering to handle cases where the data may not be in the expected format. Additionally, leveraging TypeScript or PropTypes can enhance type checking and provide early warnings about potential issues in the code. By following these strategies, developers can create more robust React applications that handle data more effectively.
Author Profile
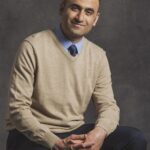
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?