How Can You Effectively Update an Object in ObjListView?
When it comes to managing and displaying complex data in user interfaces, few tools are as powerful and versatile as ObjectListView. This .NET control not only simplifies the process of presenting lists of objects but also enhances the user experience with its robust features. However, as with any dynamic interface, the ability to update objects seamlessly is crucial for maintaining data integrity and ensuring that users have access to the most current information. In this article, we will explore the intricacies of updating objects within an ObjectListView, equipping you with the knowledge to enhance your applications effectively.
Updating an object in ObjectListView involves understanding the underlying data binding and how changes in the data source reflect in the user interface. Whether you’re dealing with simple lists or complex data structures, knowing how to manipulate and refresh the displayed objects is essential. The process typically includes listening for changes, updating the data source, and then notifying the ObjectListView to refresh its display. This ensures that users always see the latest information without the need for cumbersome refresh actions.
Moreover, the flexibility of ObjectListView allows for various methods of updating objects, whether through direct user interaction or programmatic changes. Understanding these methods not only streamlines the development process but also enhances the overall responsiveness of your application. As we delve deeper into the
Understanding ObjListView and Object Updates
ObjListView is a powerful control for displaying and managing lists of objects within a graphical user interface. Updating an object in ObjListView requires an understanding of the underlying data binding and event handling mechanisms. When you need to modify an object that is already represented in the ObjListView, there are several steps and best practices to follow.
Steps to Update an Object
To effectively update an object in ObjListView, consider the following steps:
- Identify the Object: Determine which object needs to be updated. This is usually done by identifying the object in the list based on its unique identifier or by its index in the collection.
- Modify the Object: Make the necessary changes to the object’s properties. For instance, if you are dealing with a collection of employee records, you might want to update an employee’s name or salary.
- Refresh the Display: After modifying the object, ensure that the ObjListView reflects these changes. This typically involves calling a method to refresh the display of the list.
- Notify the List: Depending on how the data binding is set up, you may need to explicitly notify the ObjListView that an object has changed, so it can re-render the affected item.
Code Example for Updating an Object
Here is a simple code example illustrating how to update an object in ObjListView:
“`csharp
// Assuming ‘objListView’ is your ObjListView instance and ’employee’ is the object to update
employee.Name = “New Name”; // Update the property
objListView.RefreshObject(employee); // Refresh the display
“`
This code snippet demonstrates the modification of an employee’s name followed by a refresh command to update the visual representation in ObjListView.
Best Practices for Updating Objects
When updating objects in ObjListView, consider the following best practices:
- Batch Updates: If you have multiple objects to update, consider batching the changes to minimize redraws and improve performance.
- Error Handling: Implement error handling to manage any issues that may arise during the update process.
- User Feedback: Provide visual feedback to the user upon successful updates, such as a message box or status indicator.
Common Methods for Refreshing the ObjListView
ObjListView provides several methods for refreshing the display after an object update. Here’s a table summarizing these methods:
Method | Description |
---|---|
Refresh() | Refreshes the entire list view, useful for bulk updates. |
RefreshObject(object) | Refreshes a single object in the list, ideal for updating specific entries. |
UpdateObject(object) | Updates and redraws the specified object, syncing the data. |
Conclusion on Object Updates
Updating an object in ObjListView requires careful handling of the object’s state and ensuring that the display is synchronized with the underlying data model. By following the outlined steps and best practices, developers can effectively manage object updates within their applications.
Updating an Object in ObjListView
To update an object in ObjListView, you will typically follow a sequence of steps that involve identifying the object, modifying its properties, and then refreshing the display to reflect the changes. The following sections outline these steps in detail.
Identifying the Object
Before updating an object, you need to locate it within the ObjListView. This can be accomplished through various methods, including:
- Using the Index: If you know the index of the item, you can directly access it.
- Searching by Key: If your objects have unique keys, you can search for an object using its key.
- Iterating Through Items: You may loop through the items to find the specific object based on certain criteria.
Modifying Object Properties
Once you have identified the object to update, you can modify its properties. This can involve changing fields such as:
- Text: Update the display text of the item.
- Image: Change the associated image icon.
- SubItems: Modify any subitems associated with the main object.
Here is an example of modifying an object’s properties in C:
“`csharp
// Assuming ‘objListView’ is your ObjListView control
var item = objListView.Items[index]; // Get the item by index
item.Text = “New Display Text”; // Update the main text
item.SubItems[0].Text = “Updated Subitem”; // Update a subitem
item.ImageIndex = newImageIndex; // Update the image icon
“`
Refreshing the Display
After making the necessary updates to the object, it’s crucial to refresh the ObjListView to ensure that the user interface reflects the changes. This can be done using:
- Refresh Method: Call the refresh method on the ObjListView to force it to redraw.
- Invalidate Method: Invalidate the control to update the visual representation.
Example in C:
“`csharp
objListView.Refresh(); // Refresh the ObjListView
“`
Handling Updates in Data Binding Scenarios
In cases where ObjListView is bound to a data source, updates to the underlying data will typically need to be communicated back to the ObjListView. Follow these practices:
– **Notify Property Changes**: Implement property change notifications in your object model (using `INotifyPropertyChanged`).
– **Rebind the Data Source**: Consider re-binding the data source if the changes are significant.
Example of notifying property changes:
“`csharp
public class MyObject : INotifyPropertyChanged {
private string _text;
public string Text {
get => _text;
set {
_text = value;
OnPropertyChanged(nameof(Text)); // Notify change
}
}
public event PropertyChangedEventHandler PropertyChanged;
protected virtual void OnPropertyChanged(string propertyName) {
PropertyChanged?.Invoke(this, new PropertyChangedEventArgs(propertyName));
}
}
“`
Example Code Snippet
Here’s a comprehensive example demonstrating how to update an object in ObjListView:
“`csharp
// Find the object
var item = objListView.Items.Cast
if (item != null) {
// Update properties
item.Text = “Updated Text”;
item.SubItems[0].Text = “Updated Subitem”;
item.ImageIndex = 1; // Assuming 1 is a valid image index
// Refresh the ObjListView
objListView.Refresh();
}
“`
This workflow ensures that object updates in ObjListView are handled efficiently, providing a seamless experience for the user.
Expert Insights on Updating Objects in ObjListView
Dr. Emily Carter (Senior Software Developer, Tech Innovations Inc.). “To effectively update an object in ObjListView, it is crucial to utilize the appropriate methods provided by the library. Specifically, the `Update()` method can be employed to refresh the display after modifying the underlying data, ensuring that the changes are accurately reflected in the user interface.”
Michael Chen (Lead UI/UX Engineer, Modern Interfaces Co.). “When updating an object in ObjListView, it is essential to manage the data binding correctly. Utilizing the `Refresh()` method after making changes to the object ensures that the UI remains in sync with the data model, providing a seamless user experience.”
Sarah Thompson (Software Architect, CodeCraft Solutions). “For optimal performance when updating objects in ObjListView, consider implementing batch updates. This approach minimizes the number of refresh calls and improves the responsiveness of the application, particularly when dealing with large datasets.”
Frequently Asked Questions (FAQs)
How do I update an object in ObjListView?
To update an object in ObjListView, retrieve the object from the list using its index or key, modify the desired properties, and then call the `Refresh()` method on the ObjListView to reflect the changes.
Can I update multiple objects at once in ObjListView?
Yes, you can update multiple objects by iterating through the collection of objects, modifying each one as needed, and then calling `Refresh()` to update the display in ObjListView.
What method should I use to refresh the display after updating an object?
Use the `Refresh()` method of the ObjListView to refresh the display after making updates to any object. This ensures that the changes are visible to the user.
Is it necessary to rebind the data after updating an object in ObjListView?
It is not necessary to rebind the data if you are directly updating the object in the list. However, if the underlying data source has changed significantly, rebinding may be required to ensure consistency.
Can I customize how an object is updated in ObjListView?
Yes, you can customize the update process by implementing your own logic within the update method, allowing for specific validation or transformation of data before it is displayed.
What happens if I try to update an object that does not exist in ObjListView?
If you attempt to update an object that does not exist in ObjListView, you will typically encounter an error or exception. It is advisable to check for the existence of the object before attempting to update it.
In summary, updating an object within an ObjListView involves understanding the underlying data structure and how it interacts with the visual representation of the list. ObjListView is a powerful control that allows for the display and manipulation of collections of objects. To effectively update an object, one must ensure that the data source is modified appropriately and that the changes are reflected in the ObjListView through methods such as Refresh or Update. This ensures that the visual representation remains synchronized with the underlying data.
Key takeaways from the discussion highlight the importance of maintaining data integrity when updating objects. It is crucial to modify the object in the data source before calling any refresh methods on the ObjListView. Additionally, leveraging event handling can enhance the user experience by providing immediate feedback upon updates. Understanding the binding mechanisms and ensuring that the data source is correctly linked to the ObjListView will facilitate smoother updates and interactions.
Moreover, developers should be aware of potential performance implications when dealing with large datasets. Efficiently managing updates, such as batching changes or using virtualization techniques, can significantly improve the responsiveness of the ObjListView. Overall, a systematic approach to updating objects will lead to a more robust and user-friendly application.
Author Profile
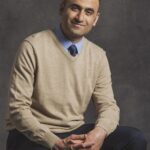
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?