How to Resolve Operand Type Clash: Why Is Date Incompatible with Int?
In the world of programming and database management, errors can often feel like insurmountable obstacles, particularly when they arise from seemingly simple operations. One such error that developers frequently encounter is the “operand type clash: date is incompatible with int.” This cryptic message can halt progress and lead to frustration, especially for those who may not fully understand the underlying causes. But fear not! By unraveling the complexities of data types and their interactions, we can demystify this error and equip you with the knowledge to tackle it head-on.
Overview
At its core, the “operand type clash” error serves as a reminder of the importance of data types in programming. When performing operations, especially in languages that enforce strict type checking, mismatched data types can lead to conflicts that prevent code execution. This specific error highlights a common scenario where a date type is incorrectly paired with an integer, leading to confusion and disruption in the flow of data manipulation. Understanding the nature of these types and their intended use is crucial for any developer aiming to write efficient and error-free code.
Moreover, this error often arises in database queries, where developers might inadvertently mix date and integer values in a condition or calculation. By exploring the intricacies of data types, such as how they
Understanding Operand Type Clash
Operand type clash errors occur when an operation attempts to combine incompatible data types. In the context of databases and programming, a common instance arises when trying to perform arithmetic or logical operations between a date type and an integer type. This can lead to confusion and runtime errors, especially when the underlying logic assumes compatibility between these types.
Common Causes of Type Clashes
Several scenarios can trigger operand type clashes:
- Direct Comparisons: When a date is compared to an integer, such as in a conditional statement.
- Arithmetic Operations: Attempting to add, subtract, or manipulate dates with integer values.
- Function Arguments: Passing incompatible types to functions expecting specific data types.
Understanding these triggers is crucial to effectively debugging and resolving these errors.
Examples of Operand Type Clash
Here are some illustrative examples that showcase operand type clashes:
- **Date Comparison with Integer**:
“`sql
SELECT * FROM Orders WHERE OrderDate > 20230101;
“`
In this query, the date field `OrderDate` is being compared to an integer, which can lead to a type clash.
- Arithmetic Operation:
“`sql
SELECT DATEADD(DAY, 5, OrderDate) FROM Orders WHERE OrderID = 1 + 2;
“`
Here, adding the integers `1 + 2` directly to a date without proper handling could result in an error.
Resolving Operand Type Clash
To effectively resolve operand type clashes, consider the following strategies:
- Type Conversion: Explicitly convert one of the operands to ensure compatibility.
- Using Appropriate Functions: Utilize functions designed to handle specific data types, like `CAST` or `CONVERT` in SQL.
- Validation: Implement checks to ensure data types match before performing operations.
Operation | Correct Usage | Incorrect Usage |
---|---|---|
Date Comparison | WHERE OrderDate > ‘2023-01-01’ | WHERE OrderDate > 20230101 |
Date Addition | SELECT DATEADD(DAY, 5, OrderDate) | SELECT OrderDate + 5 |
By adhering to these practices, developers can avoid operand type clashes and ensure smoother execution of queries and operations involving dates and integers.
Understanding Operand Type Clash
Operand type clash errors occur when there is an attempt to perform operations between incompatible data types in programming or database queries. Specifically, the error message “operand type clash: date is incompatible with int” indicates that an operation is trying to use a date type in a context where an integer is expected, or vice versa.
Common Scenarios Leading to Operand Type Clashes
Several scenarios can trigger this error:
- Date Comparisons: Attempting to compare a date column with an integer value. For example:
“`sql
SELECT * FROM orders WHERE order_date = 20230101; — Incorrect
“`
- Arithmetic Operations: Trying to perform arithmetic on date fields and integers, such as adding an integer to a date, without proper conversion.
“`sql
SELECT order_date + 5 FROM orders; — Incorrect
“`
- Function Misuse: Using functions that expect a certain data type, but passing an incompatible type.
“`sql
SELECT DATEDIFF(order_date, 5) FROM orders; — Incorrect
“`
How to Resolve Operand Type Clash Errors
To resolve these errors, consider the following approaches:
- Type Casting: Explicitly convert data types to ensure compatibility.
- Use `CAST` or `CONVERT` functions in SQL.
“`sql
SELECT * FROM orders WHERE order_date = CAST(20230101 AS DATE); — Correct
“`
- Correct Data Types: Ensure that the correct type is used in operations.
- Always use date formats when working with date fields.
“`sql
SELECT * FROM orders WHERE order_date = ‘2023-01-01’; — Correct
“`
- Review Function Inputs: Verify that all function inputs are of the expected type.
“`sql
SELECT DATEDIFF(order_date, ‘2023-01-01’) FROM orders; — Correct
“`
Best Practices to Avoid Operand Type Clashes
To minimize the risk of encountering operand type clashes, adhere to the following best practices:
- Data Type Awareness: Familiarize yourself with the data types used in your database schema.
- Use Parameterized Queries: When dealing with input values, always use parameterized queries to prevent type mismatches.
- Consistent Formatting: Maintain consistent date formatting in your application and database interactions.
- Regular Code Reviews: Conduct code reviews to identify and rectify potential type mismatches before deployment.
Example Cases of Operand Type Clash
Below is a table showcasing examples of common operand type clashes and their resolutions:
Scenario | Cause | Resolution |
---|---|---|
Comparing date with integer | `WHERE order_date = 20230101` | Use date format: `WHERE order_date = ‘2023-01-01’` |
Adding integer to date | `SELECT order_date + 5` | Use date functions: `SELECT DATEADD(DAY, 5, order_date)` |
Incorrect function argument | `SELECT DATEDIFF(order_date, 5)` | Use proper type: `SELECT DATEDIFF(order_date, ‘2023-01-01’)` |
Implicit casting issues | Using mixed types in queries | Ensure consistent types across all fields |
Conclusion on Operand Type Clash
Understanding and resolving operand type clash errors is essential for maintaining data integrity and ensuring the smooth operation of applications. By following best practices and being aware of type compatibility, developers can effectively manage and prevent these errors in their code.
Understanding Operand Type Clash in Data Management
Dr. Emily Carter (Data Integrity Specialist, TechSolutions Inc.). “The error message ‘operand type clash: date is incompatible with int’ typically arises in database queries when there is an attempt to perform operations between mismatched data types. It is crucial to ensure that date fields are correctly formatted and not mistakenly treated as integers during calculations.”
Michael Chen (Senior Database Administrator, CloudData Corp.). “In my experience, this type of error often occurs when developers overlook type casting in SQL statements. Always validate the data types of the operands involved in your queries to avoid such clashes, especially in complex joins or aggregations.”
Laura Patel (Software Engineer, DataWorks Solutions). “When encountering an ‘operand type clash’ error, it is essential to revisit the schema definitions of your database. Ensuring that the data types align with the expected input can prevent runtime errors and improve overall application reliability.”
Frequently Asked Questions (FAQs)
What does the error “operand type clash: date is incompatible with int” mean?
This error indicates that there is an attempt to perform an operation between a date type and an integer type, which is not permissible in SQL or programming languages that enforce strict type checking.
How can I resolve the “operand type clash: date is incompatible with int” error?
To resolve this error, ensure that you are using compatible data types in your operations. Convert the integer to a date or vice versa, depending on the context of your operation.
What are common scenarios that trigger this error?
Common scenarios include trying to compare a date column with an integer value, performing arithmetic operations on dates using integers, or incorrectly passing parameters in a function that expects a date.
Can this error occur in both SQL and programming languages?
Yes, this error can occur in both SQL databases and programming languages that enforce type safety, such as Java, C, and Python, when there is an attempt to mix incompatible types.
Is there a way to check data types in SQL to prevent this error?
Yes, you can use the `SELECT DATA_TYPE` query on your table schema to review the data types of your columns, which helps ensure that you are using the correct types in your operations.
What best practices can help avoid this error in the future?
Best practices include consistently using the correct data types in your queries, validating input data types before performing operations, and utilizing type conversion functions when necessary.
The error message “operand type clash: date is incompatible with int” typically arises in database management systems, particularly when dealing with SQL queries. This error indicates that there is an attempt to perform an operation that involves incompatible data types, specifically a date type and an integer type. Such clashes often occur in expressions that involve comparisons, calculations, or function calls where the expected data types do not match the provided values.
To resolve this issue, it is crucial to ensure that the data types being used in operations are compatible. This can be achieved by explicitly converting data types using appropriate functions provided by the database system. For instance, one can use functions like CAST or CONVERT in SQL to change the data type of a variable or column to match the expected type in the operation. Additionally, careful examination of the query structure and the data types of the involved columns can help identify and rectify the source of the error.
It is also important to consider the context in which the error occurs. Understanding the logic of the query and the intended operations can aid in determining the correct data types to use. Moreover, thorough testing and validation of queries before execution can prevent such errors from occurring in production environments. By adhering to best practices in data type management, one
Author Profile
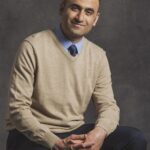
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?