How to Resolve the ‘Operand Type Clash: Int is Incompatible with Date’ Error?
In the world of programming and database management, encountering errors can be a frustrating yet enlightening experience. One such common error that developers often face is the “operand type clash: int is incompatible with date.” This seemingly cryptic message can halt progress and lead to confusion, especially for those new to data manipulation and querying. Understanding the nuances behind this error is crucial for anyone working with databases, as it not only highlights the importance of data types but also emphasizes the need for precision in coding.
When working with databases, every data type serves a specific purpose, and mixing them can lead to unexpected results or outright failures. The error in question typically arises when an integer value is mistakenly used in a context where a date is expected. This can happen in various scenarios, such as during comparisons, calculations, or function calls. By delving into the mechanics of data types and their interactions, developers can not only resolve this error but also enhance their overall coding practices.
Moreover, the implications of such operand type clashes extend beyond mere error messages. They can affect data integrity, application performance, and user experience. Recognizing the root causes of these clashes and implementing best practices for data type management can significantly improve the robustness of applications. In the following sections, we will explore the intric
Understanding Operand Type Clash
Operand type clash errors commonly occur in programming and database management systems when there is an attempt to perform operations between incompatible data types. Specifically, the error message “operand type clash int is incompatible with date” indicates a mismatch between an integer (int) and a date data type during a comparison or operation.
This type of error is prevalent in SQL queries, particularly when dealing with conditions that involve both numeric and date values. For example, attempting to compare an integer representing an ID with a date column will trigger this type of error.
Common Causes of Operand Type Clash
Several scenarios can lead to this type of error:
- Improper Data Type Usage: Using an integer where a date is expected, such as in a WHERE clause or function.
- Function Mismatches: Applying a function designed for dates to an integer value or vice versa.
- Implicit Conversions: Relying on the database to implicitly convert types can result in unexpected type clashes.
Examples of Operand Type Clash
Consider the following SQL query:
“`sql
SELECT * FROM orders WHERE order_id = 2023-01-01;
“`
In this case, `2023-01-01` is interpreted as a date, while `order_id` is likely an integer. This will raise an operand type clash error.
Another example is:
“`sql
SELECT * FROM events WHERE event_date < 2023;
```
Here, `event_date` is a date type, and `2023` is treated as an integer. The comparison fails due to incompatible types.
Resolving Operand Type Clash Errors
To address operand type clash errors, consider the following strategies:
- Explicit Casting: Convert the integer to a date or vice versa using appropriate functions.
- Check Data Types: Ensure that the variables or columns being compared are of compatible types.
- Use Correct Functions: Utilize functions that are designed to handle the specific data types involved.
Data Type | Example | Resolution Strategy |
---|---|---|
Integer | 2023 | Convert to Date using CAST or CONVERT |
Date | ‘2023-01-01’ | Ensure comparison with a Date type |
Date | current_date() | Use for comparisons with Date types |
By implementing these strategies, developers can effectively handle operand type clashes and avoid potential runtime errors in their applications.
Understanding Operand Type Clash
Operand type clash errors occur when there is an attempt to perform operations on incompatible data types. In the context of databases and programming languages, this often arises when a numeric type, such as an integer, is used where a date type is expected.
Common Causes of Operand Type Clash
Several scenarios may lead to this type of error:
- SQL Queries: Trying to compare or manipulate a date field with an integer.
- Function Arguments: Passing an integer to a function that expects a date.
- Data Manipulation: Inserting or updating records where data types do not align.
Examples of Operand Type Clash
Scenario | Example | Explanation |
---|---|---|
SQL Comparison | `SELECT * FROM orders WHERE order_date = 20230101;` | The integer `20230101` should be a date type, e.g., `DATE ‘2023-01-01’`. |
Function Usage | `DATEDIFF(20230101, order_date)` | The first argument needs to be a date, not an integer. |
Data Insertion | `INSERT INTO events (event_date) VALUES (12345);` | The value `12345` must be formatted as a date. |
Resolving Operand Type Clash Issues
To address operand type clashes, consider the following strategies:
- Check Data Types: Ensure that all operands in expressions or functions are of compatible types.
- Type Conversion: Use casting functions to convert data types where necessary. Common SQL functions include:
- `CAST(value AS DATE)`
- `CONVERT(DATE, value)`
- Validation Before Operations: Implement checks to validate that data types align prior to executing commands.
Best Practices to Avoid Operand Type Clash
Adopting best practices can significantly reduce the occurrence of operand type clashes:
- Consistent Data Types: Maintain uniform data types in tables for fields that will be compared or manipulated together.
- Use Descriptive Naming: Label fields clearly to indicate their data types, such as `event_date` for date fields.
- Implement Error Handling: Use try-catch blocks in your code to gracefully handle type mismatches.
Tools and Functions to Assist with Data Type Management
Utilizing built-in tools and functions can help manage data types efficiently:
Tool/Function | Description |
---|---|
`TRY_CAST()` | Attempts to cast a value to a specified data type and returns NULL if it fails. |
`ISDATE()` | Checks if a value can be converted to a date, returning a boolean result. |
Database Schema Design Tools | Use these tools to enforce data type constraints at the design stage. |
By implementing these strategies and practices, developers can effectively avoid and resolve operand type clash errors, leading to smoother database operations and programming workflows.
Understanding Operand Type Clashes in Database Management
Dr. Emily Carter (Database Systems Analyst, Tech Innovations Inc.). “The error message indicating an operand type clash between ‘int’ and ‘date’ typically arises in SQL queries when an integer value is being compared or assigned to a date field. It is crucial to ensure that the data types align correctly to prevent such conflicts.”
Mark Thompson (Senior Software Engineer, Data Solutions Corp.). “When encountering an operand type clash, developers should review their SQL statements for any implicit conversions that may be taking place. Using explicit type casting can often resolve these issues and maintain data integrity.”
Linda Garcia (Database Administrator, Global Tech Services). “To effectively troubleshoot the ‘int is incompatible with date’ error, it is advisable to analyze the schema of the database. Ensuring that the data types of the columns involved in the operation match the expected types is essential for smooth query execution.”
Frequently Asked Questions (FAQs)
What does “operand type clash int is incompatible with date” mean?
This error indicates that there is an attempt to perform an operation between an integer and a date type in a database query or programming context, which is not allowed due to type incompatibility.
What causes this error in SQL queries?
This error typically arises when a query tries to compare or manipulate an integer value with a date field, such as using a mathematical operation or a direct comparison that is not type-compatible.
How can I resolve the operand type clash error?
To resolve this error, ensure that both operands in the operation are of the same data type. You may need to convert the integer to a date or vice versa, depending on the intended logic of your query.
Are there specific functions to convert data types in SQL?
Yes, SQL provides functions like `CAST()` and `CONVERT()` that can be used to change data types. For example, you can use `CAST(your_integer AS DATE)` to convert an integer to a date format.
Can this error occur in programming languages other than SQL?
Yes, similar type clash errors can occur in various programming languages when operations involve incompatible data types, such as trying to perform arithmetic on a date object and an integer.
How can I prevent operand type clashes in my code?
To prevent operand type clashes, always ensure that you are aware of the data types being used in your operations. Implement type checks and conversions as necessary before performing operations that involve multiple data types.
The error message “operand type clash int is incompatible with date” typically arises in database operations, particularly when using SQL. This error indicates that there is an attempt to perform an operation or comparison between an integer and a date data type, which are fundamentally incompatible. Such conflicts often occur in queries involving WHERE clauses, JOIN conditions, or during data manipulation where the expected data types do not align. Understanding the context of this error is crucial for effective troubleshooting and resolution.
To resolve this issue, it is essential to ensure that the data types in your SQL statements match. This can be achieved by explicitly converting data types using functions such as CAST or CONVERT, depending on the database system in use. For instance, if you need to compare an integer value representing a date (like a timestamp) with a date column, converting the integer to a date format would be necessary. Conversely, if the operation requires the date to be compared as an integer, the date can be cast to an integer format.
Key takeaways from this discussion include the importance of data type compatibility in SQL queries. It is advisable to always check the data types of the columns involved in operations to prevent such clashes. Additionally, utilizing proper data conversion functions can help maintain the integrity
Author Profile
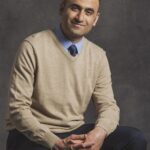
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?