How Can I Use ‘ORDER BY RANDOM’ in SQL to Shuffle My Query Results?
When it comes to querying databases, SQL (Structured Query Language) is the powerhouse that drives data manipulation and retrieval. Among its many capabilities, one intriguing feature is the ability to sort results in a seemingly chaotic manner—specifically, ordering records randomly. This technique can be particularly useful in various scenarios, from selecting a random sample for testing to creating dynamic content for applications and websites. But how does one effectively implement this seemingly whimsical ordering in SQL?
In this article, we will explore the concept of ordering results randomly in SQL, delving into the methods and functions that facilitate this process across different database systems. Whether you’re working with PostgreSQL, MySQL, or SQL Server, each platform offers unique ways to achieve random ordering, and understanding these nuances can enhance your data querying skills. We will also discuss practical applications of random ordering, shedding light on when and why you might want to use this technique in your own projects.
As we navigate through the intricacies of random ordering in SQL, you’ll gain insights into the underlying principles and best practices. By the end of this article, you’ll be equipped with the knowledge to implement random ordering effectively, adding a valuable tool to your SQL toolkit. So, let’s dive in and uncover the fascinating world of random data retrieval!
Understanding Random Ordering in SQL
In SQL, the ability to order records randomly can be particularly useful in various scenarios, such as selecting a random sample of data for analysis, testing, or even for presentation purposes. Different database management systems (DBMS) have specific functions or methods to achieve this random ordering.
Random Ordering Techniques by Database
Different SQL databases implement random ordering using varying functions. Here’s a breakdown of how to use random ordering in some popular SQL databases:
Database | Function | Example Query |
---|---|---|
PostgreSQL | RANDOM() | SELECT * FROM table_name ORDER BY RANDOM(); |
MySQL | RAND() | SELECT * FROM table_name ORDER BY RAND(); |
SQLite | RANDOM() | SELECT * FROM table_name ORDER BY RANDOM(); |
SQL Server | NEWID() | SELECT * FROM table_name ORDER BY NEWID(); |
Oracle | DBMS_RANDOM.VALUE | SELECT * FROM table_name ORDER BY DBMS_RANDOM.VALUE; |
Performance Considerations
When using random ordering, it is essential to consider the potential performance implications, especially with large datasets. Ordering by random functions can lead to significant performance overhead because:
- The entire dataset may need to be scanned and processed.
- Random ordering can prevent the use of indexes effectively, leading to full table scans.
- The performance can vary significantly depending on the size of the table and the specific database implementation.
To mitigate performance issues, you may opt for alternative approaches such as:
- Limiting the number of records returned using the `LIMIT` clause (where applicable).
- Using a temporary table or a Common Table Expression (CTE) to store random selections.
Use Cases for Random Ordering
Random ordering is employed in various practical applications, including:
- Sampling: Extracting random samples for statistical analysis.
- Testing: Creating randomized test cases for applications.
- Games and Applications: Randomly selecting items or users for promotions or rewards.
- User Experience: Displaying random items to enhance user engagement.
By understanding the appropriate functions and implications of random ordering in SQL, you can effectively utilize this feature in your database queries.
Using ORDER BY RANDOM in SQL
In SQL, retrieving records in a random order can be achieved using the `ORDER BY` clause in conjunction with a randomization function. The specific function varies by SQL database system. Below are examples demonstrating how to implement this in different SQL dialects.
PostgreSQL
In PostgreSQL, you can use the `RANDOM()` function to sort records randomly. The syntax is straightforward:
“`sql
SELECT * FROM your_table
ORDER BY RANDOM();
“`
This query will return all records from `your_table` in random order each time it is executed.
MySQL
For MySQL, you can utilize the `RAND()` function in a similar fashion:
“`sql
SELECT * FROM your_table
ORDER BY RAND();
“`
This command will also return the results in a different random order on each execution.
SQLite
SQLite supports the `RANDOM()` function as well, but it works slightly differently since it returns a random integer. You can use it like this:
“`sql
SELECT * FROM your_table
ORDER BY RANDOM();
“`
This effectively randomizes the order of the rows returned.
SQL Server
In SQL Server, the approach is slightly different as it does not support a direct random function in the `ORDER BY` clause. Instead, you can use `NEWID()`:
“`sql
SELECT * FROM your_table
ORDER BY NEWID();
“`
Using `NEWID()` generates a unique identifier for each row, resulting in a randomized order.
Performance Considerations
Using `ORDER BY RANDOM()` or its equivalents can lead to performance issues, particularly with large datasets. Here are some key points to consider:
- Full Table Scan: Sorting by a random function often requires a full scan of the table, making it resource-intensive.
- Limitations: If you only need a subset of random records, consider using the `LIMIT` clause to retrieve fewer rows.
Example for MySQL:
“`sql
SELECT * FROM your_table
ORDER BY RAND()
LIMIT 10;
“`
This retrieves 10 random records instead of the entire table.
Alternative Methods
For large datasets, consider using alternative strategies such as:
- Sampling: Use sampling techniques or create a temporary table with a random selection of IDs.
- Random Number Generation: Generate random numbers in application logic and fetch corresponding records.
These methods can enhance performance while still achieving random selection.
Utilizing `ORDER BY RANDOM()` or equivalent functions allows for effective randomization in SQL queries. However, it is essential to consider performance implications, especially with larger datasets. Employing strategies to limit the number of records processed can significantly improve execution time.
Expert Insights on Using Order By Random in SQL
Dr. Emily Carter (Database Architect, Tech Innovations Inc.). “Using ‘ORDER BY RANDOM()’ in SQL can be an effective way to retrieve random records from a database. However, it is essential to be cautious with performance implications, especially with large datasets, as this method can lead to significant overhead.”
Michael Chen (Data Analyst, Insight Analytics). “When implementing ‘ORDER BY RANDOM()’, one must consider alternative methods such as using a random number generator in combination with filtering, which can enhance efficiency and reduce execution time in larger tables.”
Lisa Patel (Senior SQL Developer, Cloud Solutions Corp.). “While ‘ORDER BY RANDOM()’ is straightforward, it’s crucial to understand that it may not be the best choice for every application. For instance, in high-traffic environments, exploring indexed approaches or sampling techniques could yield better performance.”
Frequently Asked Questions (FAQs)
What does “order by random” do in SQL?
“Order by random” is a SQL clause used to sort the results of a query in a random order, ensuring that the output is different each time the query is executed.
How do I use “order by random” in different SQL databases?
The syntax for ordering by random varies by database. For example, in PostgreSQL, use `ORDER BY RANDOM()`, while in MySQL, use `ORDER BY RAND()`. SQLite also uses `ORDER BY RANDOM()`.
Can “order by random” affect performance?
Yes, using “order by random” can significantly impact performance, especially on large datasets, as it requires sorting all rows randomly before returning results.
Is there a way to limit the number of random results returned?
Yes, you can combine “order by random” with the `LIMIT` clause to restrict the number of rows returned. For example, `SELECT * FROM table ORDER BY RANDOM() LIMIT 10;`.
Are there any alternatives to “order by random” for selecting random rows?
Alternatives include using a random number generator to select specific rows or using sampling methods, such as `TABLESAMPLE` in SQL Server, which can be more efficient.
Can “order by random” be used with other SQL clauses?
Yes, “order by random” can be combined with other clauses like `WHERE`, `GROUP BY`, and `JOIN` to filter and organize data while still returning results in a random order.
In SQL, the ability to order results randomly can be achieved using specific functions that vary by database management system. For instance, in MySQL, the `ORDER BY RAND()` clause is commonly used to randomize the order of rows returned by a query. Similarly, PostgreSQL employs the `ORDER BY RANDOM()` function, while SQL Server utilizes the `NEWID()` function to achieve a similar outcome. Understanding these variations is crucial for developers and database administrators when working across different platforms.
Utilizing random ordering can be particularly beneficial in scenarios such as selecting random samples from a dataset, displaying random promotional items, or generating varied user experiences. However, it is important to note that random ordering can have performance implications, especially on large datasets, as these functions may require a full table scan to generate the random order. Therefore, it is advisable to consider the size of the dataset and the specific use case when implementing this feature.
Overall, ordering by random in SQL is a powerful tool that enhances the flexibility and dynamism of data presentation. By leveraging the appropriate functions for their specific database systems, developers can effectively introduce randomness into their queries, thereby enriching user interaction and engagement with the data. As with any SQL operation, careful consideration of performance and
Author Profile
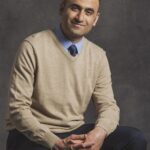
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?